This content originally appeared on Bits and Pieces - Medium and was authored by Shane Tarleton
Functional programming in JavaScript is your friend
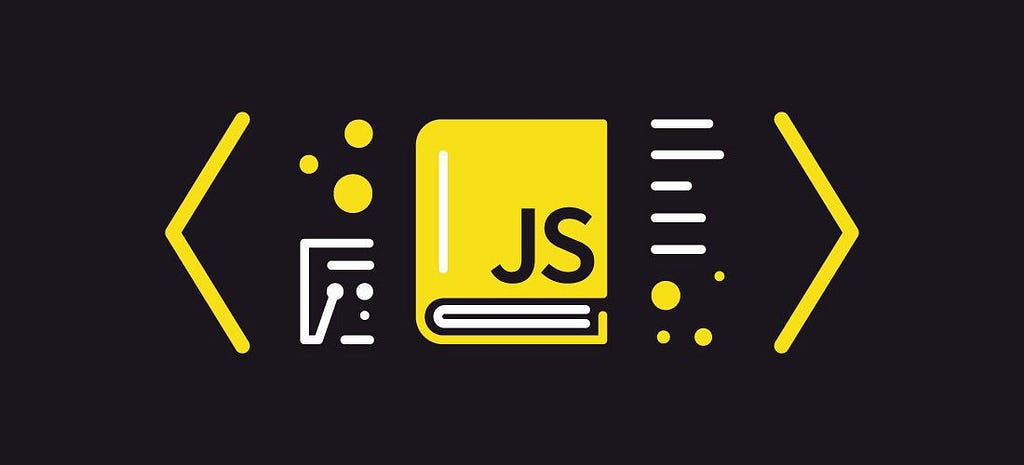
Pre-Requisite Knowledge
Before I get started, this is going to teach you how the .map() function works. If you only have knowledge of for() loops in JavaScript, this article will require you to understand the Arrow Function Expression syntax (a.k.a. “fat arrow” functions).
If there is enough demand, I can create a separate tutorial to explain those further. Let me know in the comments.
Let’s Get Started
Oh, how I long for the days when learning a for loop was a foreign concept to me. Those were simple times back before trying to take programming concepts and data structures and turn them into usable, real-world software.
But I digress.
In every programmer’s career, there is a point in time where we were taught that Imperative Programming (usually as a precursor to Object-Oriented Programming) was the definitive method of building usable software.
In some cases, such as mine, you slowly begin to change your perception of such things. My perceptions and “programming style” have morphed ever since a friend introduced me to functional programming around 6 years ago.
I do not claim to fully-understand Monads or some of the other concepts from that paradigm, but one thing has become clear to me:
Pure functions make code significantly-easier to read and write automated tests against.
Alright, enough talk. Let’s get into some actual code, shall we?
You’re chugging along with your code and are provided with an array of objects, where each object represents a Person. Each Person contains 3 properties: id, name, and emailAddress. You need to create an array that contains only the id values from each Person within the original array.
Here is likely what you wrote, assuming you were using an Imperative Programming approach:
The principle concept that stands out in every similar scenario is this: you want to create a new array with the exact same length as the previous array, but the data in the new array is different (in some way or another) and you do not want to make any changes to the original array. You have now discovered the perfect scenario for Functional Programming.
Array.prototype.map()
Let’s take a look at how we would write the exact same code above using the Array.prototype.map() function (not to be confused with the Map object).
The first time I saw someone use the .map() function as a replacement for how I would’ve written the for() loop above, I was lost. There is actually another way that you could write the .map() function, which you wouldn’t do in a real-world situation, but it does help explain what is going on here.
Now, I am sure you will recognize the listOfPeople[i].id piece of the code here from the for() loop example. To explain this further, the .map() function is “looping” over each item in the array and assigning the item (i.e. person in the second and third examples) and the current index (i.e. i in the first and third examples) as parameters to the function that we define.
The .map() function requires that the function return a value (i.e. person.id in the second example and listOfPeople[i].id in the third example) for the index so that it is able to assign that value to the same index in the new array (i.e. listOfIds). This is, essentially, the same thing that is being done in the first example when performing the listOfIds.push(listOfPeople[i].id) function.
Hopefully this explanation helps someone out there understand this concept a little bit better. If there is anything you think I should change, explain further, or update, then I would love to hear about it in the comments. I wish you well!
Build component-driven. It’s better, faster, and more scalable.
Forget about monolithic apps, start building component-driven software. Build better software from independent components and compose them into infinite features and apps.
OSS Tools like Bit offer a great developer experience for building component-driven. Start small and scale with many apps, design systems or even Micro Frontends. Give it a try →
Learn more
- Building a React Component Library — The Right Way
- 7 Tools for Faster Frontend Development in 2022
- The Composable Enterprise: A Guide
Use map() instead of for() loops was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Shane Tarleton
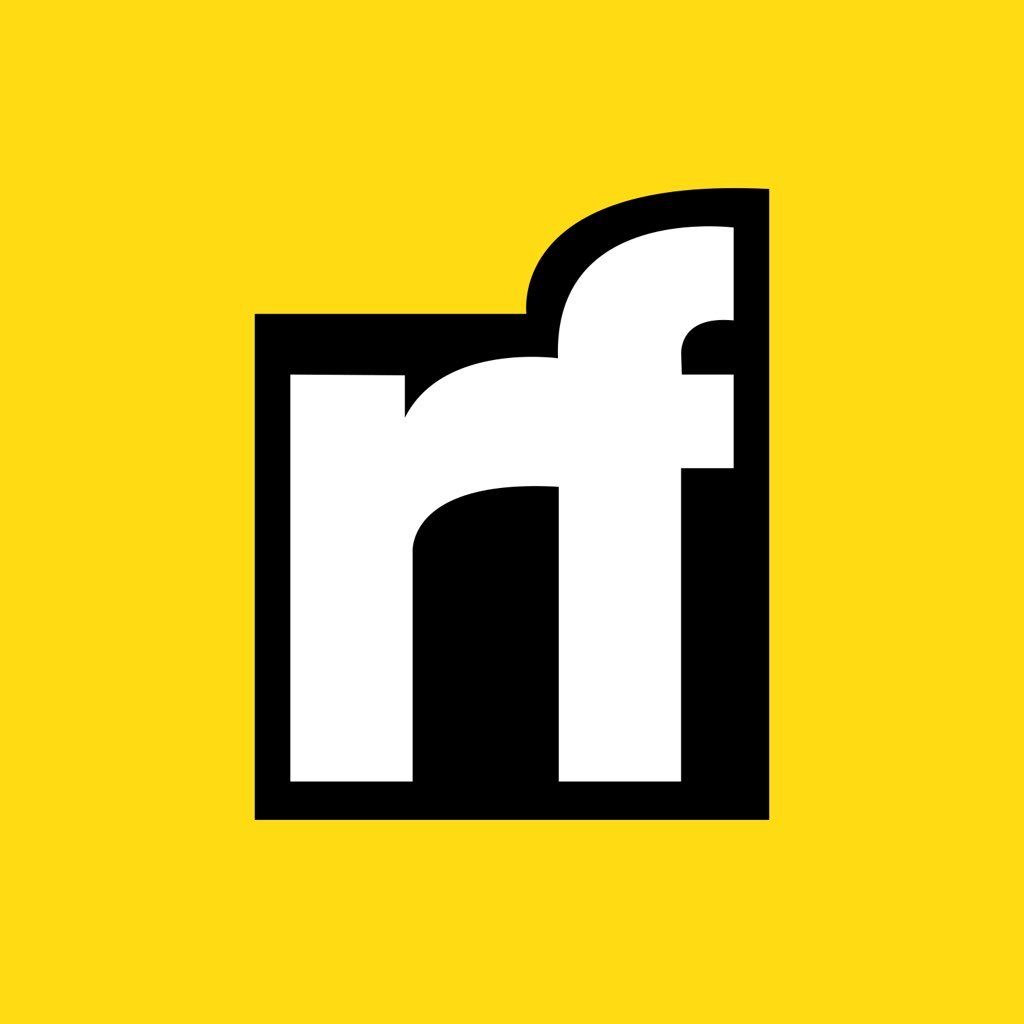
Shane Tarleton | Sciencx (2022-03-01T16:29:21+00:00) Use map() instead of for() loops. Retrieved from https://www.scien.cx/2022/03/01/use-map-instead-of-for-loops/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.