This content originally appeared on DEV Community and was authored by DEV Community
Do you know the differences between var let and const ? If not, this article is made for you.
Var
Variables declared with var will be available in the parent scope unless it is declared inside a function.
var age = 15;
function getFirstName() {
var firstName = "John";
}
getFirstName();
console.log(age); // Logs 15
console.log(firstName); // Logs "firstName is not defined"
They can also be re-declared.
var color = "Blue";
var color = "Red";
console.log(color); // Logs "Red"
Let
You can declare a variable and initialize it later :
let firstName;
console.log(firstName); // Returns "undefined"
firstName = "John"; // Returns "John"
But you can't re-declare it.
let firstName = "John";
let firstName = "David";
console.log(firstName); // Logs "Identifier 'firstName' has
already been declared"
And they can only be accessed inside their scope.
function getFirstName() {
if (true) {
let firstName = "John";
console.log( firstName); // Logs "John"
firstName = "David";
console.log( firstName); // Logs "David"
}
console.log( firstName); // Logs "firstName is not defined"
}
getFirstName();
Const
Const variables can't be updated, nor re-declared.
const firstName = "John";
firstName = "David";
console.log(firstName); // Returns "Assignment to constant variable"
const color = "Blue";
const color = "Red";
console.log(color); // Logs "Identifier 'color' has already been declared"
This means you have to initialize them when declared, and you can't use the same name twice either.
const firstName;
console.log(firstName); // Logs "Missing initializer in const declaration"
Just like let, const is block scoped.
function getFirstName() {
if (true) {
const firstName = "John";
console.log( firstName); // Logs "John"
}
console.log( firstName); // Logs "firstName is not defined"
}
getFirstName();
⚠️ Even if the variable can't be reassigned, this doesn't mean the value is immutable. If the value is an object or an array you can still change its content.
const array = []
array.push(1);
console.log(array); // Returns [1]
const object = { firstName: "Red" };
object.firstName = "Blue";
console.log(object); // Returns { firstName: 'Blue' }
Photo by Pankaj Patel on Unsplash
This content originally appeared on DEV Community and was authored by DEV Community
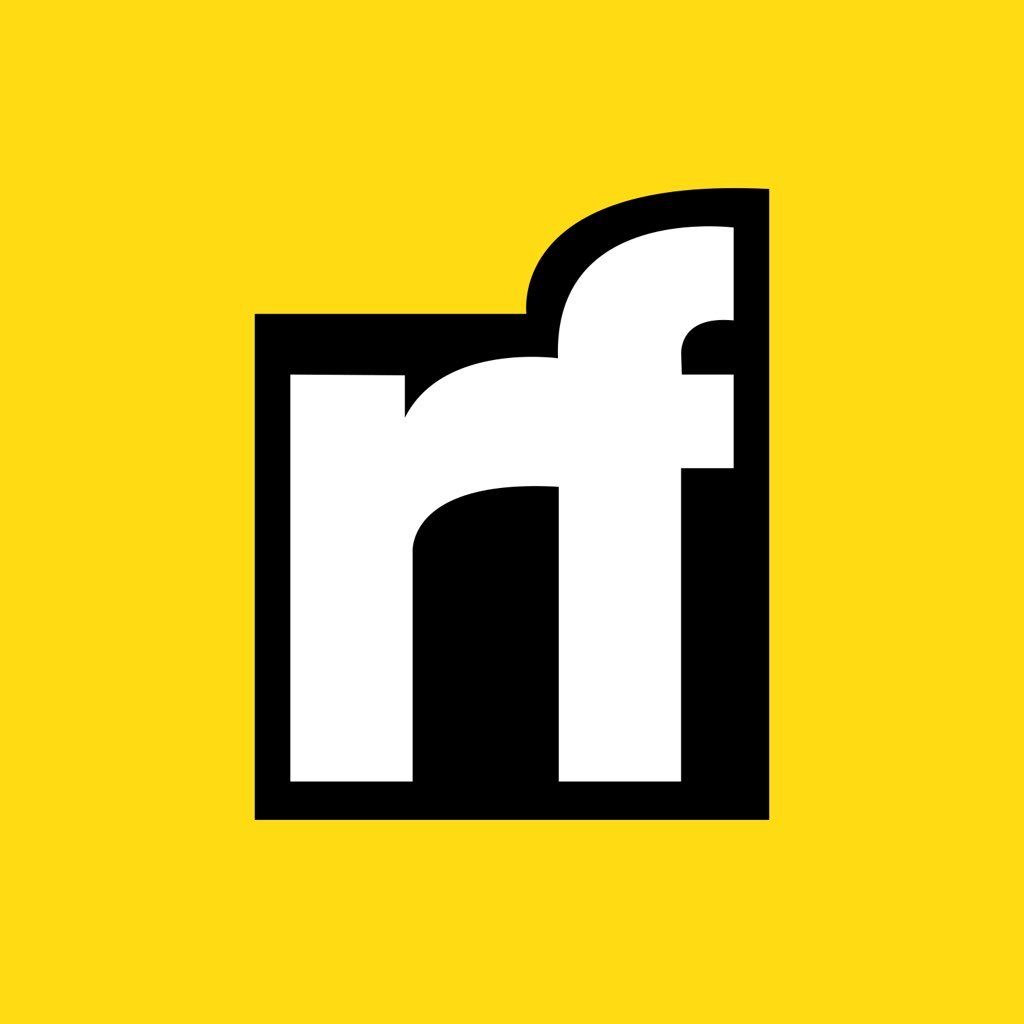
DEV Community | Sciencx (2022-03-02T19:55:24+00:00) Var, Let and Const Explained. Retrieved from https://www.scien.cx/2022/03/02/var-let-and-const-explained/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.