This content originally appeared on DEV Community and was authored by Ahmed Gouda
Selection Statements
Selection statements are used to choose among alternative actions.
if
if( Condition )
{
Action;
}
if(grade >= 60)
{
puts("Passed");
}
if .. else
if(Condition)
{
Action_1;
}
else
{
Action_2;
}
if(grade >= 60)
{
puts("Passed");
}
else
{
puts("Failed");
}
if .. elseif
if(Condition_1)
{
Action_1;
}
else if(Condition_2)
{
Action_2;
}
else if(Condition_3)
{
Action_3;
}
else
{
Action_4;
}
if(grade >= 90)
{
puts("A");
}
else if(grade >= 80)
{
puts("B");
}
else if(grade >= 70)
{
puts("C");
}
else if(grade >= 60)
{
puts("D");
}
else
{
puts("F");
}
switch
switch(Condition)
{
case 1:
Action_1;
break;
case 2:
Action_2;
break;
case 3:
Action_3;
break;
default:
Action_4;
break;
}
switch(mark)
{
case 'A':
puts("Student got excellent grade");
break;
case 'B':
puts("Student got very good grade");
break;
case 'C':
puts("Student got good degree grade");
break;
case 'D':
puts("Student got fair grade");
break;
default:
puts("Student should retake the exam");
break;
}
Conditional Operator
This is the only C ternary operator. It is used in place where if .. else
can't.
Condition ? True Action : False Action;
grade >= 60 ? puts("Pass") : puts("Fail");
//or
puts(grade >= 60 ? "Pass" : "Fail");
Iteration Statements
An iteration statement (repetition statement or loop) allows you to specify that an action is to be repeated while some condition remains true.
while
while(Continuation_Condition)
{
Action;
}
while(grade < 60)
{
puts("Retake the exam");
}
do .. while
do{
Action;
}while(Continuation_Condition);
do{
//do the action once at least before check the condition
puts("Start the Exam");
}while(grade < 60>);
for
for(Initialization; Continuation_Condition; Increment)
{
Action;
}
for(int i = 1; i <= 10; i++)
{
printf("%d ", i);
}
Iteration Types
- Counter-Controlled
- Sentinel-Controlled
Counter-Controlled Iteration
We know in advance exactly how many times the loop will be executed.
Sentinel-Controlled Iteration
We don't know in advance how many times the loop will be executed. We use sentinel value (flag value) to indicated "End of Data" entry.
This content originally appeared on DEV Community and was authored by Ahmed Gouda
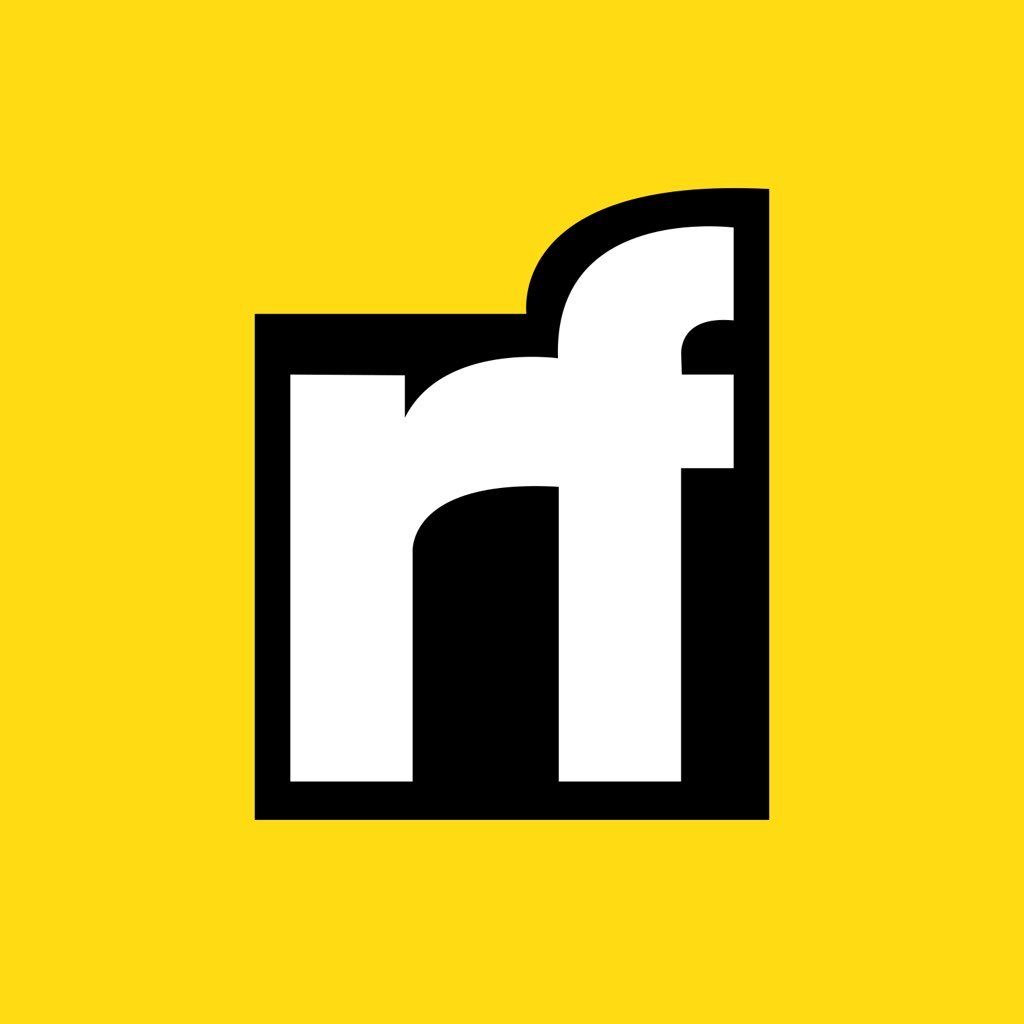
Ahmed Gouda | Sciencx (2022-03-28T22:44:44+00:00) Selection and Iteration in C. Retrieved from https://www.scien.cx/2022/03/28/selection-and-iteration-in-c/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.