This content originally appeared on Level Up Coding - Medium and was authored by Joshua Etim

In this short tutorial, we will build a random user generator that runs on only client-side technology. This tool will be useful for users who want to register on websites for temporary usage without giving away their real details. It will also link to a temporary email generator where they can receive emails.
With the increasing number of API services available, it is possible to build simple and useful tools without ever having to work on the backend. This project fetches data from the Random User API, performs a quick password generation operation and date formatting, and returns user profile data to the user. Let’s get started!
For the styling, I’ll be using Bootstrap and Vue.js will be used to handle the client-side scripting. We will be using Vue from a CDN instead of the usual CLI tool. This is to maintain simplicity for the first part of the project.
Step 1: Create the layout
If you’re familiar with bootstrap, this part should be quite easy for you. I made use of simple bootstrap classes and a few custom styles I got from the internet.
(Run on codepen: https://codepen.io/redwolf333/pen/dyJRmWr)
Here, I used tables to style the user data, primarily used bootstrap styles and some custom styling. I also added Google fonts link, and a script source to the Vue3 library. We also have an app.js where we will write the Vue code for the app. You can take some time to study the markup and understand the layout.
Step 2: Create Vue app
We need to create a Vue app instance and bind it to the element with id “app”. This has been created in the html layout. To create the Vue app, we will bind initial data that will be loaded into the html template:
Also, we update the html layout to display data defined in the JavaScript code:
Step 3: Create required functions
I always like to break my code down into unit functions to encourage reuse and extension. For this project, we need a small number of functions to perform the following tasks:
- Fetch data from the Random User API
- Update the fields we defined in the Vue data() section
- Generate passwords
We will define the three functions in the code snippet below:
The fetchDataAPI uses the async keyword to both return a Promise (asynchronous execution) and ensure the fetch request and json functions runs in sequence. Consequently, the 2 functions run like normal functions and returns concrete values.
For the updateFields function, I passed the ‘that’ parameter, and did the same in the fetchDataAPI function. This is because these functions affect data in another scope, so we pass the ‘this’ keyboard to set the scope to that of the caller. We will implement it in a later stage for better understanding.
Step 4: Send request when app loads
For now, all we have is the default values we set in the data() function. What we want, however, is that on loading the app, the user should get a new profile loaded, in addition to the option of generating another profile.
To achieve this, we will use the Vue’s mounted function. This function is called as soon as the Vue’s mounted event is triggered (when the app is completely mounted on the “app” id). Here’s how it fits in the Vue.createApp() call:
After the data() function, we inserted the mounted() function (line 21). This calls the previously defined fetchDataAPI function which fetches the data, and calls the updateFields function to update the current data. Take note of the scope recognition using ‘this’.
Step 5: Create the method to Generate Data
From the html template, you will notice a button that says “Generate New User”. This uses a Vue event handler that triggers a method named getUser() when clicked. We will define this method in the Vue createApp function:
Step 6: Run your application
That’s all for now. You can run your application and see how it works. Let me know if you run into any issues.
Here’s the complete code:
HTML code:
JavaScript code:
Links
GitHub repository for the project: https://github.com/joshuaetim/vue-user-generator
Live demo of project: https://joshuaetim.github.io/vue-user-generator/
Random User API: https://randomuser.me/
Random User Generator with API + Vue.js was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Joshua Etim
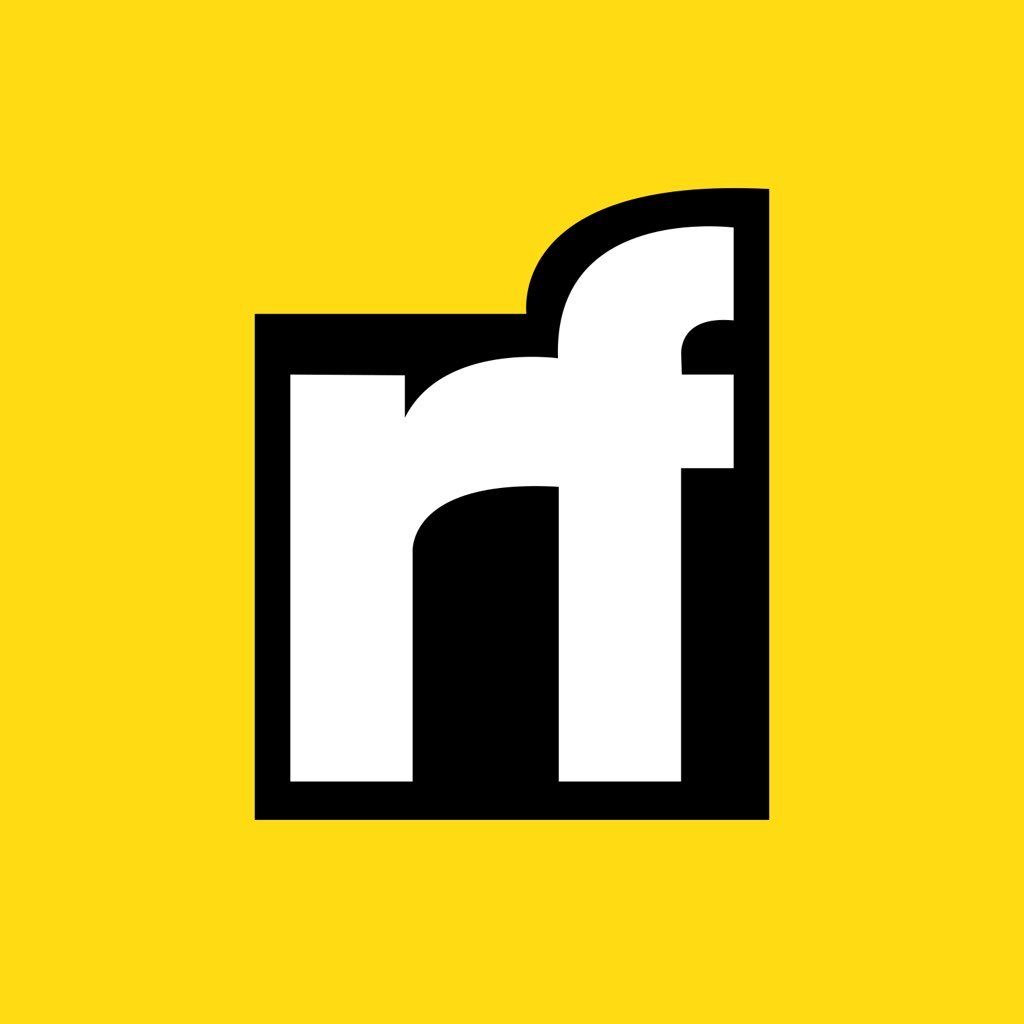
Joshua Etim | Sciencx (2022-04-01T13:23:46+00:00) Random User Generator with API + Vue.js. Retrieved from https://www.scien.cx/2022/04/01/random-user-generator-with-api-vue-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.