This content originally appeared on Level Up Coding - Medium and was authored by shivam bhatele
Java is a powerful, robust, secure and highly approachable programming language. Thus, it is no wonder that even after 28 years of its release, it is one of the most used and popular programming languages available today.
And thanks to its popularity, skilled Java developers are highly sought after. As such, it is not uncommon to see amateur developers hone their java programming skills to leverage the various professional, social and financial benefits that come with being a java programmer.
However, simply learning a programming language is not enough. You also need to learn certain coding tips and best practices to become an excellent programmer.
To ease your burden of hunting around on the internet for coding tips, we have compiled a list of a few java coding practices. These coding best practices are regularly used by experienced Java programmers and will help you immensely in writing great code.
The 10 Crucial Java Coding Practices For Budding Developers
Here we have 10 essential java coding standards and best practices that every beginner should habituate themselves to.
1. Employ Appropriate Naming Conventions
While programming in Java, or for that matter, any programming language, the key to writing efficient code is to ensure that it not only works well but is also readable. The higher the readability of a piece of code, the more convenient it is for you and your teammates to understand its purpose.
And one of the most consistent ways to improve a code’s readability is to abide by certain naming conventions. Naming conventions are fundamentally a collection of rules that enforce a consistent naming standard for all identifiers (classes, variables, interfaces, methods, etc.).
Since this article is for Java developers, here we have mentioned some typical Java naming conventions.
- For naming classes, one should only use nouns and the names should begin with uppercase letters.
- Package names should entirely be in lowercase.
- Programmers should use as few acronyms as possible.
- Interfaces should be named while following Camel Case.
- Method names should be verbs. Also, programmers should capitalise the first letter of every word in a method name except the first word.
- Variable names should follow the mixed case convention (Camel Case but with the first letter not capitalised).
- All names used should be precise and relevant to the program.
2. Use Java Libraries Ergonomically
One of the most prominent reasons behind the popularity of Java is its support for vast code libraries. Also called Java Class Libraries, these collections of pre-written code are a handy tool for many programmers, regardless of experience.
Using libraries, programmers can put together efficient pieces of code within a short time frame as they do not have to write code for each function in a program.
However, using too many libraries can prove detrimental in the long run. Apart from hogging system memory, they can also reduce an application’s load times. And let us not talk about how some libraries ship with buggy code that can seriously harm a program.
Furthermore, managing and updating several libraries at once gradually becomes too cumbersome.
Hence, programmers should only use libraries that fulfil multiple purposes instead of installing one for a single function
3. Do not Leave Catch Blocks Vacant
The try…. catch couple is an essential Java utility that programmers use for handling exceptions.
The try block encapsulates the dubious code that might give an exception, while the catch block houses the definition of the exception.
The consensus among experienced programmers is that the catch block should not be left empty unless needed. This is because excluding any explanation for an exception in the catch block can prevent a bad piece of code from getting addressed. This, in turn, makes rectifying the code even more difficult.
4. Avoid Redundant Initialization
A common java coding practice that sets apart beginner programmers from veterans is how and when they initialise variables.
Programmers fresh out of school are more used to initialising every variable in a program. And while this is a good practice, at times, it results in redundancies when an initialised variable gets another value assigned to it without using its initial value even once.
Examine the following piece of code, for example:
The variable “c” in the above code has zero has its initial value. However, it gets overwritten when the user inputs a different number. Thus, the program never utilizes the “zero” value of variable c, resulting in a redundant initialisation.
Redundant initialisations are frowned upon as they do nothing more than occupying memory.
Hence, initialise variables only if you plan to use their initial values in the program.
5. Declare Class Members Private Wherever Possible
If you ask veteran Java programmers, they will tell you that instances of one class altering the values of another class’s variables are not uncommon. To counter such situations, these programmers employ the concept of data hiding or data encapsulation.
What they do is declare the members of a class as private using the “private” access modifier. Doing so helps limit the scope of a class member to its parent class and protects its data from any sort of externally influenced changes.
Data hiding is more of a precautionary step that should be a part of every java coder’s books.
6. Fix Memory Leaks
Java is a high-level programming language and, as such, has an automated memory management feature administered by the garbage collector.
Now, while the garbage collector does a great job of periodically clearing system memory, there are times when it cannot remove a few unused objects. This is primarily because, although not in use, those objects are still being referenced in the program.
Programmers call this situation a memory leak.
Memory leaks are usually not harmful as the JVM has enough memory to run programs even with a leak. However, things can get serious if your program returns the OutOfMemoryError error.
To prevent memory leaks:
- avoid running System.gc()
- close any idle or unused sessions
- use StringBuilder for string concatenation
- avoid creating too many static objects as they occupy memory for the entire duration of an application
- close database connections by calling the close() method
7. Incorporate Underscores
As mentioned in the first pointer, readability is at the core of a well-written piece of code.
Typically, the big numbers in a Java application look something like these:
int r= 2454781
int x= 784512389
The underscore feature, introduced first with JAVA SE 7 and included in subsequent versions, allows programmers to write big numbers in a much more conventional manner.
Thus, with the underscores, the above numbers will look something like this:
int r= 2_454_781
int x= 784_512_389
Tell us they aren’t more readable now.
8. Replacing the “+” with StringBuilder for Concatenating Strings
Many programmers use the “+” operator for concatenating strings as a standard java coding practice. And there is nothing wrong with doing so.
However, as seen in the above pointers, memory in Java is of great concern. As such, if a programmer uses the “+” operator for concatenating a large number of strings, the amount of memory consumed will be quite high, along with drops in performance. This is because every time the “+” operator links two strings together, it creates an intermediate object for the concatenation process. And the more the number of strings, the more the number of intermediate objects.
Look at the following code, for example:
This code will print “Hey, 0Hey, 0, 1Hey, 0, 1, 2Hey, 0, 1, 2, 3Hey,….” until the loop is executed 50 times. Now you can imagine how many intermediate objects will arise in the process.
To deal with this, programmers use the append() method under the StringBuilder class to concatenate strings. The reason: StringBuilder is faster, does not create intermediate objects and thus, takes up relatively less memory.
Here we have a barebones example of the StringBuilder class and the append() method in action:
The output of the above code will be: Hello Bye.
PS: You can try running and tinkering with this code on Interviewbit compiler for a better understanding of how things work.
9. Get used to Logging
It fundamentally refers to the process of generating logs for every event happening within an application during its execution.
Programmers then use these logs to trace any changes in the application code and further perform functions such as debugging the application.
Java comes with the Java Logging API out of the box. It consists of the java.util.logging package using which programmers carry out logging in a program.
To do this, they first import the java.util.logging package using the import java.util.logging.level; syntax.
Importing the java.util.logging package allows programmers to call the logger object which logs the changes occurring in the application during run time.
Logging is an essential java coding best practice that experienced programmers exercise regularly. And as a beginner, you should also incorporate it into your programming routine.
10. Write Relatable Code Comments
Beginners often overlook code comments. But, they are a highly useful coding element that can elevate the readability of a code to new heights. And veteran programmers know it the best.
Code comments become a norm when programmers have to work with a team. They enable programmers to define, explain, notify and suggest the purpose behind specific lines of code.
For example, the following code converts temperature values from Celsius to Fahrenheit.
The comment written above the code with a // notifies the uninitiated viewers what the code does.
Now, the example case above is quite basic, but it does give you a guess of how useful comments can be.
Programmers should take note to not write overly long comments as they only make the code more obscure.
Conclusion
Java is a highly versatile programming language. And to cope with this versatility, or rather, take advantage of it, programmers need to learn to not just code but code smartly.
The Java coding practices mentioned above, although not exhaustive, offer a great starting point to anyone looking to become a better Java Programmer.
So go through these tips and tricks, look around for some more (the internet is your best friend) and upskill the programmer in you. Good luck!
Top 10 Java Coding Best Practices for Beginners was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by shivam bhatele
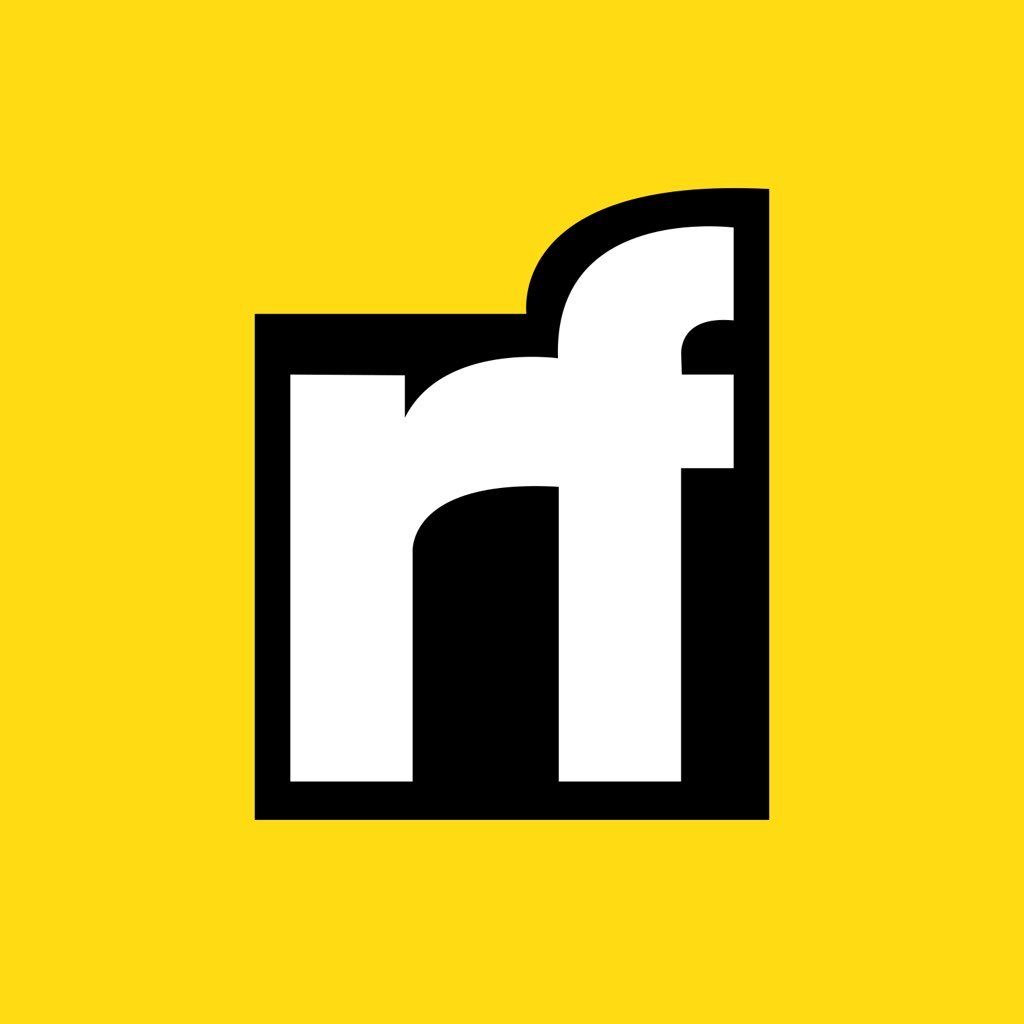
shivam bhatele | Sciencx (2022-04-05T01:09:19+00:00) Top 10 Java Coding Best Practices for Beginners. Retrieved from https://www.scien.cx/2022/04/05/top-10-java-coding-best-practices-for-beginners/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.