This content originally appeared on DEV Community and was authored by Andrii Fedyk
When it's came to exceptions, try...catch
is the way to go in JS. With introducing async/await
, we can use try...catch
statement:
let greeting
try {
greeting = hello("World")
}
catch(err) {
greeting = "Hello World"
}
console.log(greeting)
async function hello(name: string): string {
if (name === "") {
throw new RangeError("`name` can't be empty")
}
return `Hello ${name}`
}
The code is not the most elegant. When you have more errors to handle in your code, it become hell with try...catch
. As a result, it's easier just assume that code is "perfect" and don't use try...catch
. Also, many of JS developers are scared to throw new Error()
.
After spending some time with golang
, I'm amazed how well the language is designed in this matter. Inspired by golang
, I have created a simple utility called go
which drastically simplify the error handling:
function go<T>(promise: T) {
return Promise.resolve(promise)
.then(result => [null, result] as const)
.catch(err => [err, null] as const)
}
Here the example from the begging:
let [err, greeting] = await go(hello("Andrii"))
if (err) {
greeting = "Hello World"
}
console.log(greeting)
Perhaps, this is the reason why most of JS
developers are optimists and assume that their code never fail.
Error handling in async JS. In JS it's difficult and code is not tidy - as a result we just skip this part.
Inspiration from golang and explicit errors.
Example of code with difficult errors handling.
Example of solution with go helper
let [err, message] = await go(hello("Andrii"))
if (err) {
message = "Hello world"
}
console.log(message)
async function hello(name: string): string {
if (name === "") {
throw new RangeError("`name` can't be empty")
}
return `Hello ${name}`
}
function go<T>(promise: T) {
return Promise.resolve(promise)
.then(result => [null, result] as const)
.catch(err => [err, null] as const)
}
This content originally appeared on DEV Community and was authored by Andrii Fedyk
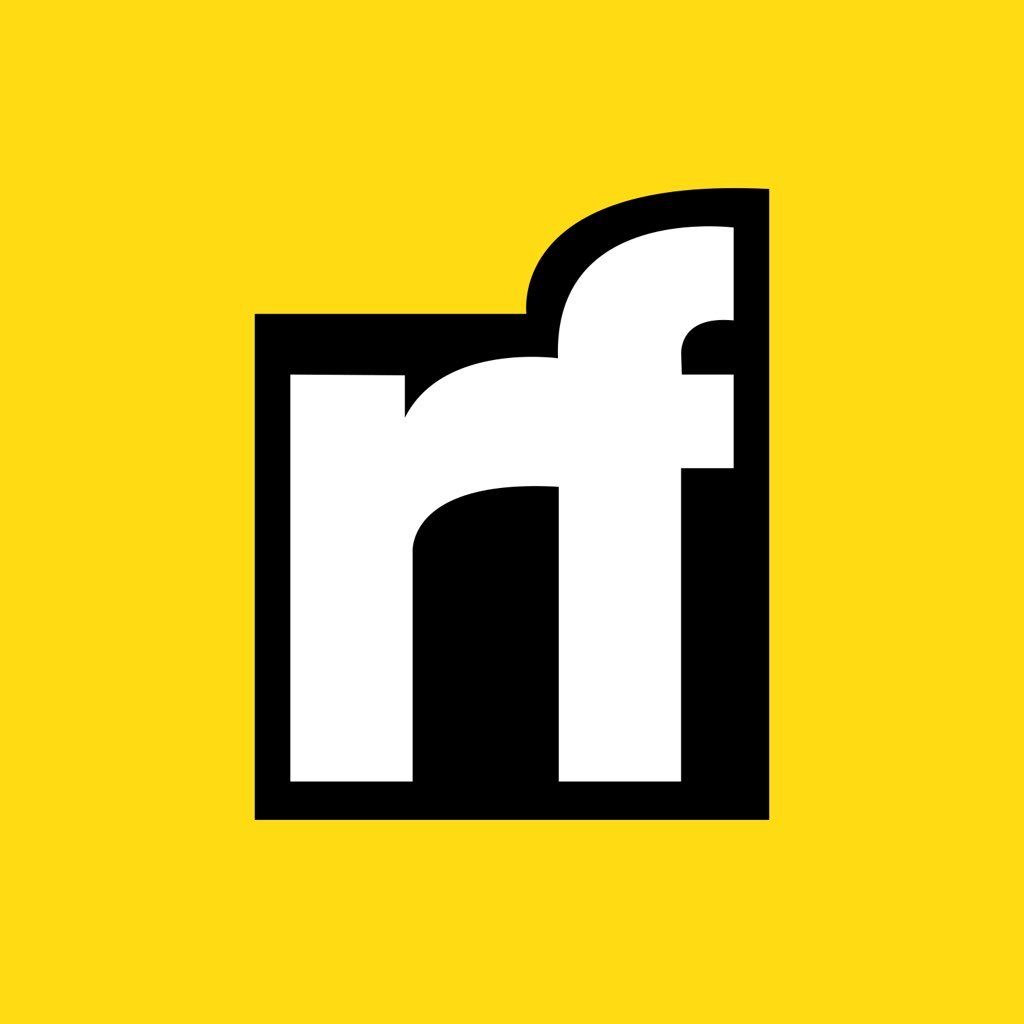
Andrii Fedyk | Sciencx (2022-04-22T14:17:37+00:00) Golang errors handing in JavaScript. Retrieved from https://www.scien.cx/2022/04/22/golang-errors-handing-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.