This content originally appeared on Level Up Coding - Medium and was authored by Anjan Shomodder
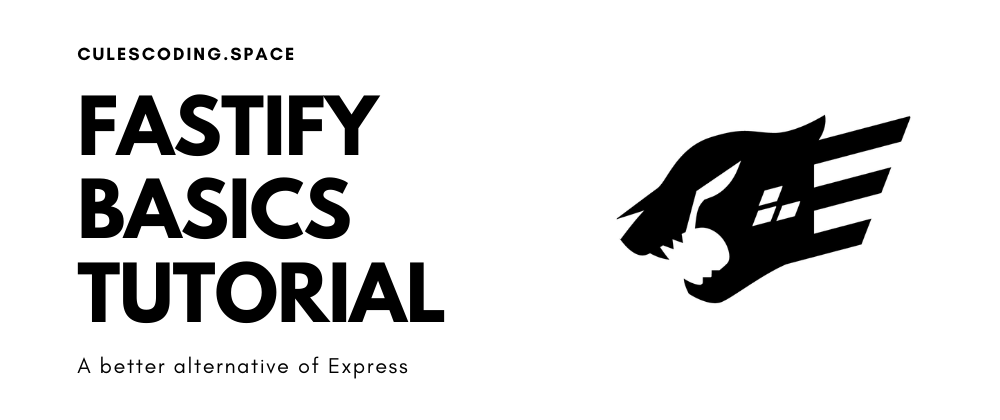
In this blog, we will learn everything you need to know to get started with Fastify.
Video tutorial
What is Fastify?
Fastify is a web framework for Node.js. It is a lightweight, fast, and flexible framework for building modern server-side web applications.
It is pretty similar to Express. But it has some features that make it standout from the others.
- Schema Validation
- Plugin System
Setup
npm init -y
npm i fastify
Create a basic node server
const fastify = require('fastify')
const app = fastify({ logger: true })
const PORT = process.env.PORT || 8000
app.listen(PORT).catch(error => {
app.log.error(error)
process.exit()
})
Explanation:
- app is the instance of Fastify. Fastify has a separate login system. We are enabling the logger by passing the object.
- PORT is the port number.
- app.listen is the function that starts the server. If any error occurs, it will log the error and exit the process.
Start the server
node <file>
Or, you can use nodemon to restart the server whenever you make a change.
npm i -g nodemon
nodemon <file>
You can see it is pretty similar to Express. Let’s create some simple api.
I will use the following data.
[
{
"id": 1,
"name": "Innis Gladeche",
"email": "igladeche0@yellowbook.com",
"gender": "Male",
"country": "North Korea"
},
{
"id": 2,
"name": "Woodman Haylands",
"email": "whaylands1@addtoany.com",
"gender": "Male",
"country": "Russia"
},
{
"id": 3,
"name": "Caleb Galbraith",
"email": "cgalbraith2@last.fm",
"gender": "Male",
"country": "Brazil"
},
{
"id": 4,
"name": "Earlie Beddie",
"email": "ebeddie3@nsw.gov.au",
"gender": "Genderqueer",
"country": "Ukraine"
},
{
"id": 5,
"name": "Marcellus Cloake",
"email": "mcloake4@opensource.org",
"gender": "Male",
"country": "Sweden"
},
{
"id": 6,
"name": "Mada Poll",
"email": "mpoll5@washington.edu",
"gender": "Female",
"country": "Sweden"
},
{
"id": 7,
"name": "Ashly Goodrum",
"email": "agoodrum6@photobucket.com",
"gender": "Female",
"country": "United States"
},
{
"id": 8,
"name": "Obed Mabbs",
"email": "omabbs7@lycos.com",
"gender": "Male",
"country": "China"
},
{
"id": 9,
"name": "Margalo Weild",
"email": "mweild8@freewebs.com",
"gender": "Female",
"country": "Sweden"
},
{
"id": 10,
"name": "Seth Jex",
"email": "sjex9@deliciousdays.com",
"gender": "Male",
"country": "France"
}
]
GET route
const getUsers = (request, reply) => users
app.get('/getUsers' getUsers)
- To handle a request use the HTTP method from app. Useapp.get for GET requests andapp.post for POST requests and so on.
- The function takes two arguments. api endpoint and callback function.
- The handler function takes two arguments. request and reply.
- To return a response, just return data from the function. We are returning the users array.
Query parameter
You can send additional information with the URL as query parameters.
Example:
http://localhost:8000/getUsers?gender=female
After the question mark, we have key-value pairs. This is what we call query parameters. Let’s use that in our /getUsers route. We will get users of specific gender with a query parameter.
const getUsers = (request, reply) => {
const { gender } = request.query
if (!gender) return users
const filteredUsers = users.filter(
(user) => user.gender.toLowerCase() === gender.toLowerCase()
)
return filteredUsers
}
app.get('/getUsers' getUsers)
Explanation:
- We are getting gender from the request.query object.
- If gender doesn’t exist, we will send users.
- Else we will filter users based on gender and return that as the response.
Post Route
Let’s create a new user.
const addUser = request => {
const id = users.length + 1
const newUser = { ...request.body, id }
users.push(newUser)
return newUser
}
app.post('/addUser', addUser)
Explanation:
- This time we will use app.post instead of app.get.
- We are getting the request body from the request object.
- Then we are creating a new user with the information of the request body and adding it to the users array.
Schema validation
A schema is a structural representation of some kind of data. In a schema, you can specify what preterites the data will have and what values will be stored.
Fastify has a built-in schema validation. You can have schema validation for request body, query parameters, response, and headers.
It is my favorite feature of Fastify. Let’s use that in our /addUser route.
const addUserOptions = {
schema: {
body: {
type: 'object',
properties: {
name: {
type: 'string',
},
age: {
type: ['number', 'string'],
},
gender: {
type: 'string',
enum: ['male', 'female', 'others'],
},
},
required: ['name', 'gender'],
},
},
}
const addUser = request => {
const id = users.length + 1
const newUser = { ...request.body, id }
users.push(newUser)
return newUser
}
app.post('/addUser', addUserOptions, addUser)
Explanation:
- We are padding options object as 2nd argument.
- We are creating a schema for the request body.
- Include the necessary properties inside the properties object and their type.
- Include the required properties inside the required array.
- To learn more about schema validation please check the video tutorial.
So that’s all I wanted to teach you. To learn more about Fastify please check the video tutorial.
I have tried to explain things simply. If you get stuck, you can ask me questions.
By the way, I am looking for a new opportunity in a company where I can provide great value with my skills. If you are a recruiter, looking for someone skilled in full stack web development and passionate about revolutionizing the world, feel free to contact me. Also, I am open to talking about any freelance project.
See my work from here
Fastify basics tutorial | A better alternative to Express was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anjan Shomodder
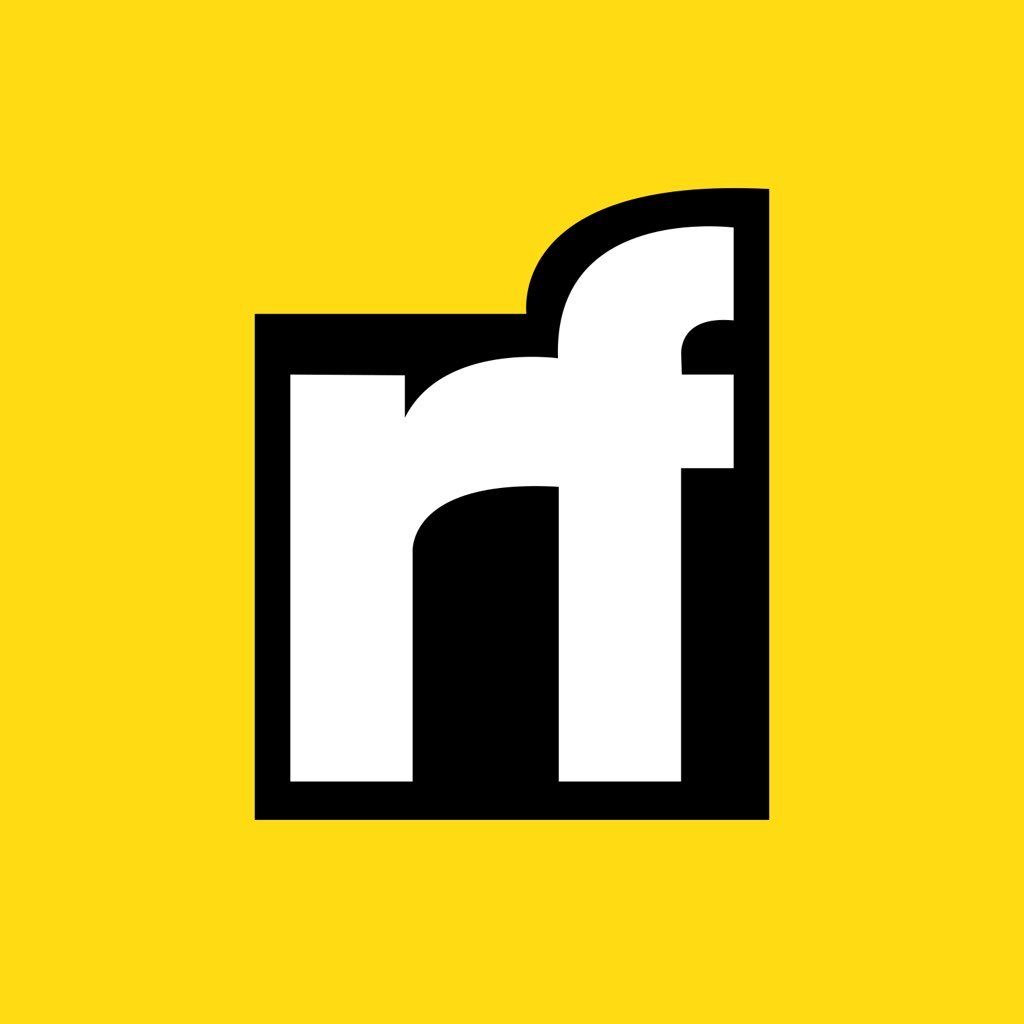
Anjan Shomodder | Sciencx (2022-04-27T01:18:16+00:00) Fastify basics tutorial | A better alternative to Express. Retrieved from https://www.scien.cx/2022/04/27/fastify-basics-tutorial-a-better-alternative-to-express/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.