This content originally appeared on Level Up Coding - Medium and was authored by Matteo Tasinato
Were you confused during your last interview by capturing and bubbling? Discover the rules which govern this world and master it!
Javascript is a versatile language and it can be used in many different environments. The most common environment is the browser but it can also be used on servers, mobile, and many others.
When you are using Javascript in a browser you have access to a special set of methods. These methods provide, for example, methods to manipulate the DOM or its events.
Starting from now we’ll use the most powerful human power to set the theory in our mind: abstraction.
The DOM desert
Imagine an infinite desert. This impressive mass of sand is the root of the DOM, the container of everything.
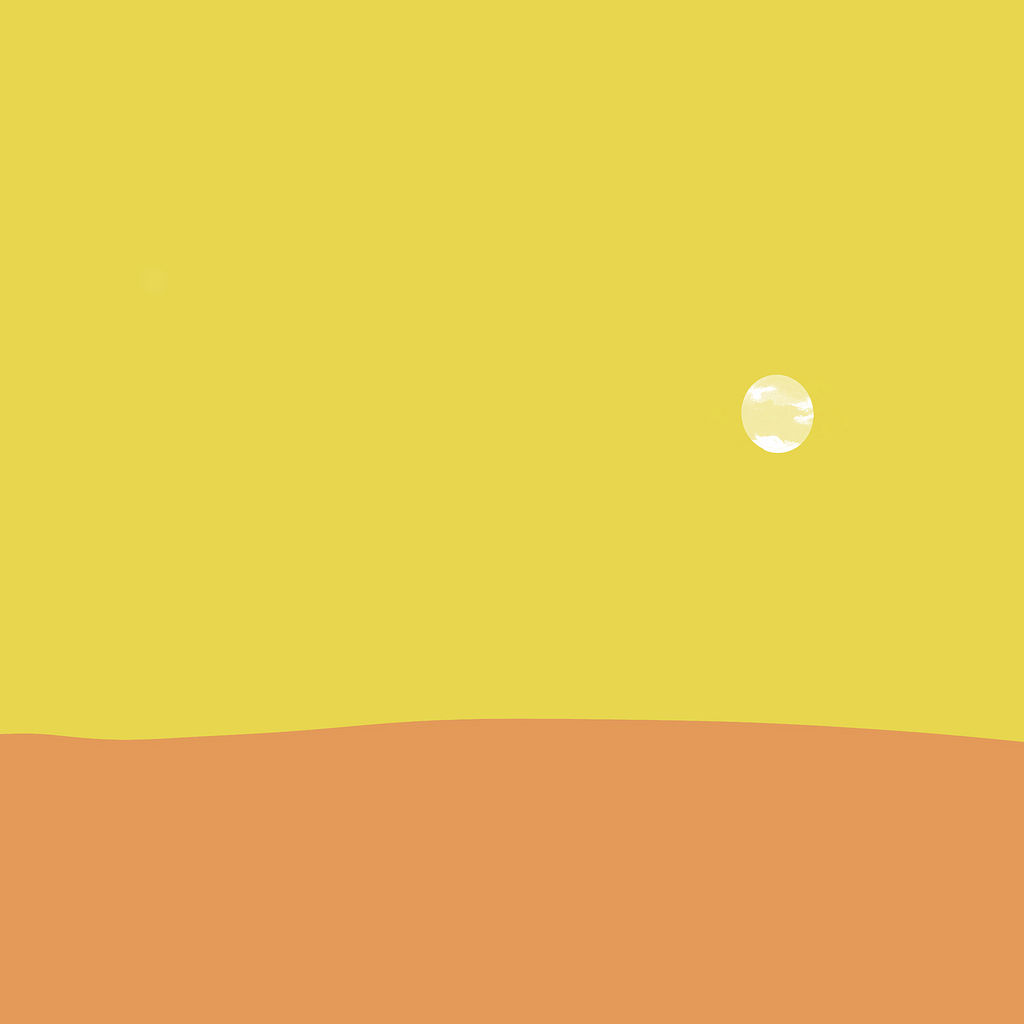
As a real desert, it can be immense and it contains every child on your HTML page. Your only limit is the power of your customer’s machine, which has to draw this large amount of components.
Now consider this small piece of HTML:
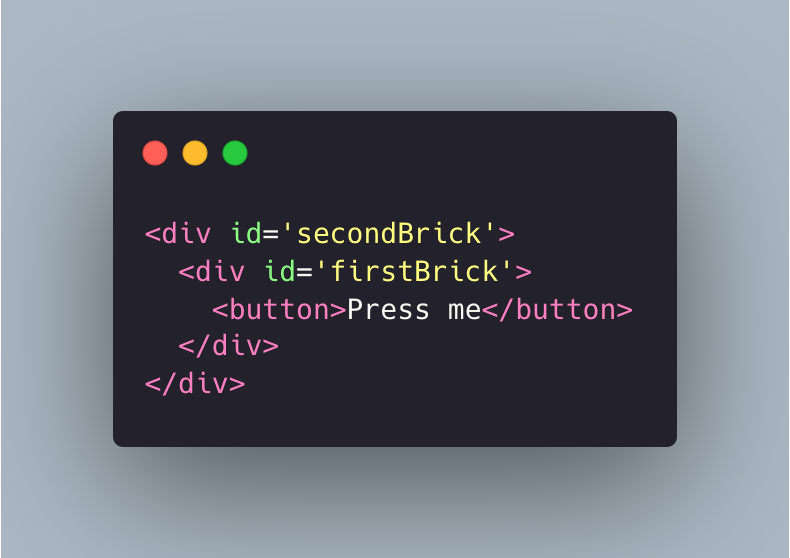
Now change your perspective:
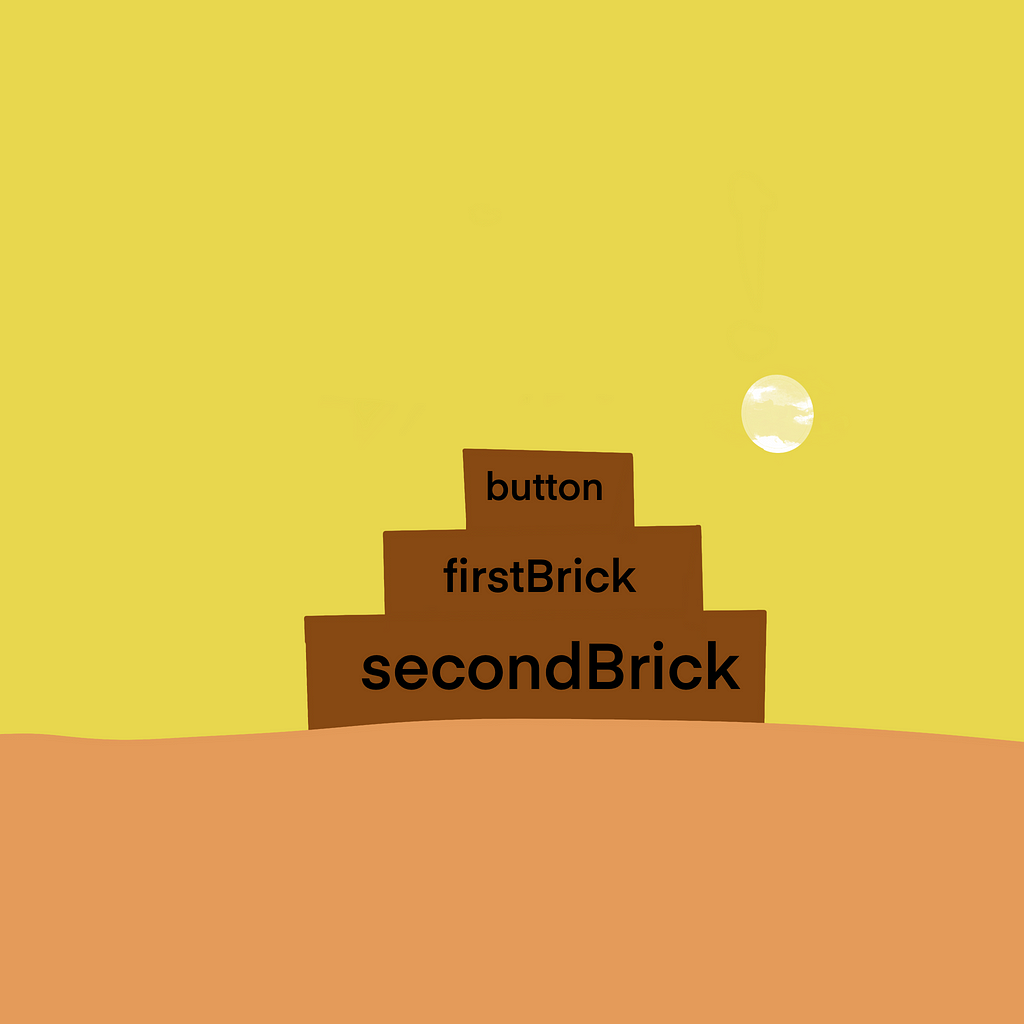
This pyramid represents your interface but we have a problem: these are not interactive. We need to bind some events to the blocks, we need some callbacks.
What is a callback?
Imagine a function as an envelope that contains instructions to execute something. For example, we have this function that gets you a unicorn:
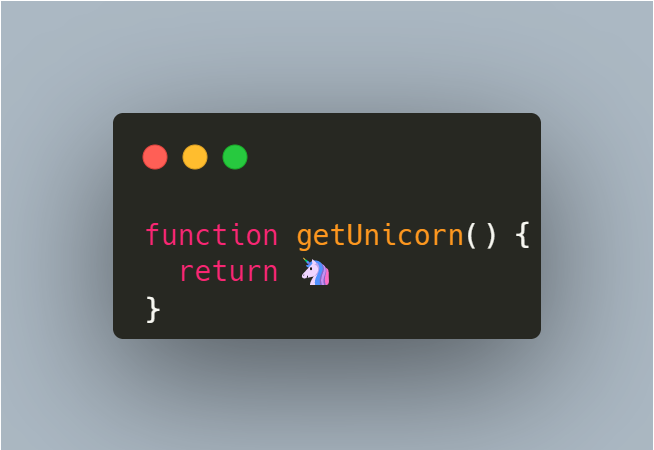
I noticed that many new developers have many difficulties distinguishing the difference between a function and its execution. When you have a doubt ask yourself: could a unicorn stay in an envelope?

A function is an object which can’t contain the actual unicorn, but just the instruction to build (or find, or create) it.
So, talking about the code:
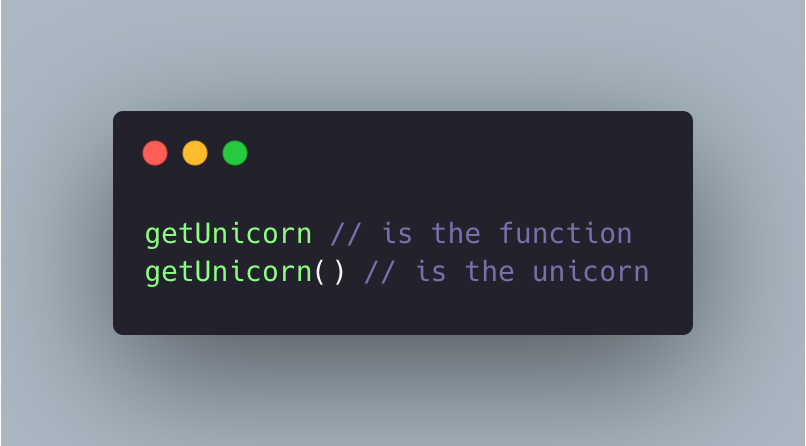
When you are passing the envelope (not the 🦄) to another element, then: this function is friendly called Callback.
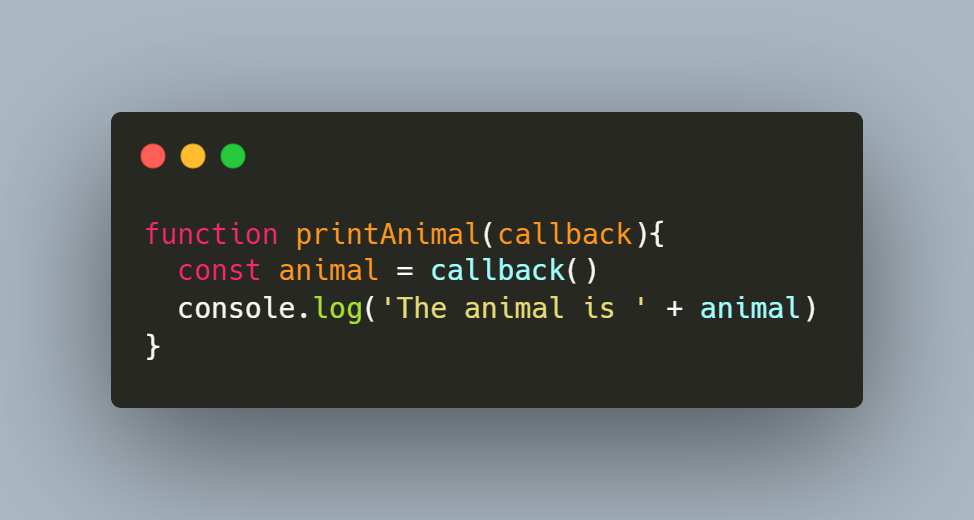
Now that we have an idea about what a callback is, we can go back to our Event Listener. If you would like a full article about callbacks, please let me know in a comment.
How to register a callback on a DOM element
We are walking close to our pyramids and we can note that every brick has a very long row of mailboxes (for my sanity I just drew three of them as examples).


Every mailbox is specialized in accepting a specific Event type.
Registering a callback in an HTML element basically means putting an envelope in one of this brick’s mailbox.
How to register an Event
Concerning the code, you have many ways to put an envelope in this mailbox. This is not the topic of this article, so I will just mention the recommended way to register an event listener:addEventListener.

This method allows you to put more than a single envelope into a mailbox. You cannot do this using the other methods. If you want to go deeper into this topic, please leave a comment.
JavaScript (HTML DOM) EventListener
Next to the desert, there is an office: the EventListener.
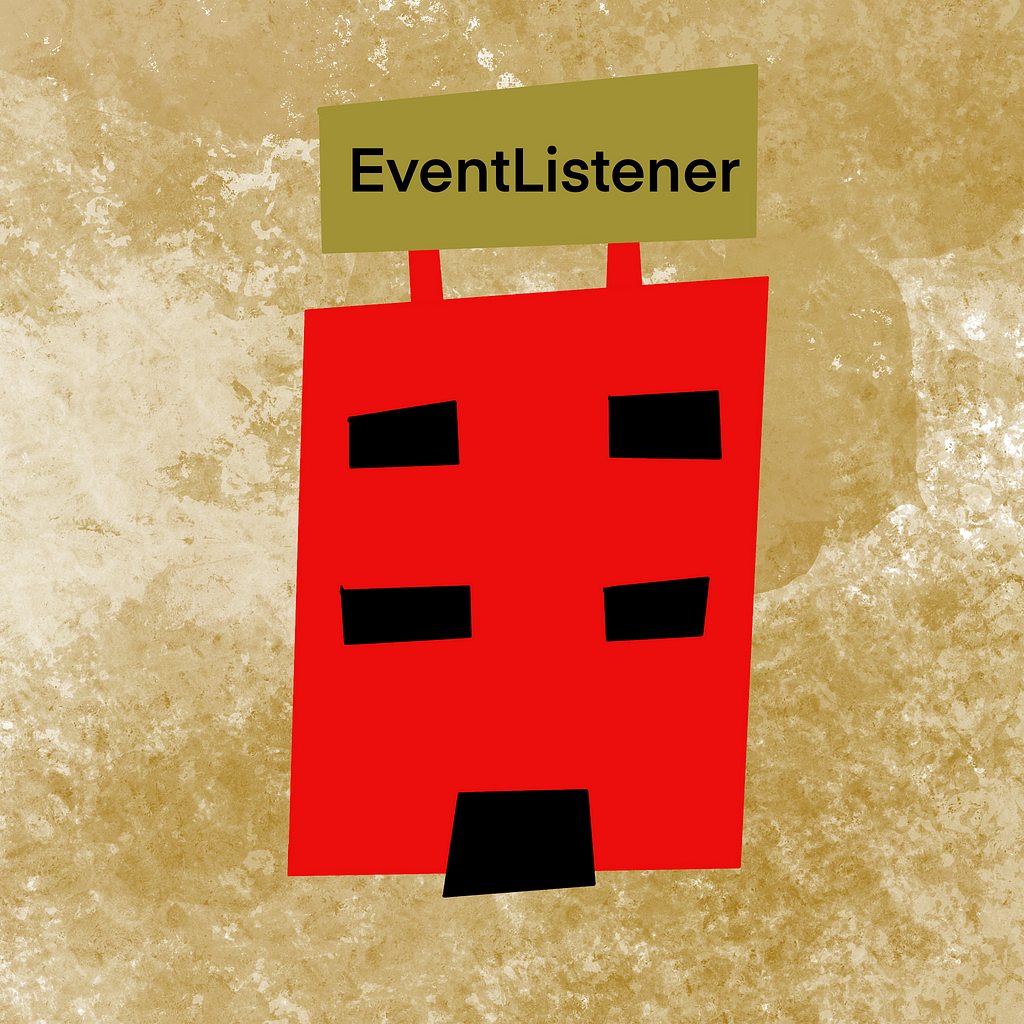
Inside of it a group of minions is working: the Event Handlers.

This office is in charge of receiving notifications about any event triggered in the Browser and delegates its best minion to climb the pyramid by executing the correct registered callback.
Example of base scenario
Suppose the user clicks the button on the top of our pyramid. The EventListener receives a notification from the browser. It always contains two important voices:
- Event type (in this case a click event).
- Target element (in this case the button).
Then the EventListener picks the Click EventListener and sends it to climb the pyramid, get the button on the top and come back.
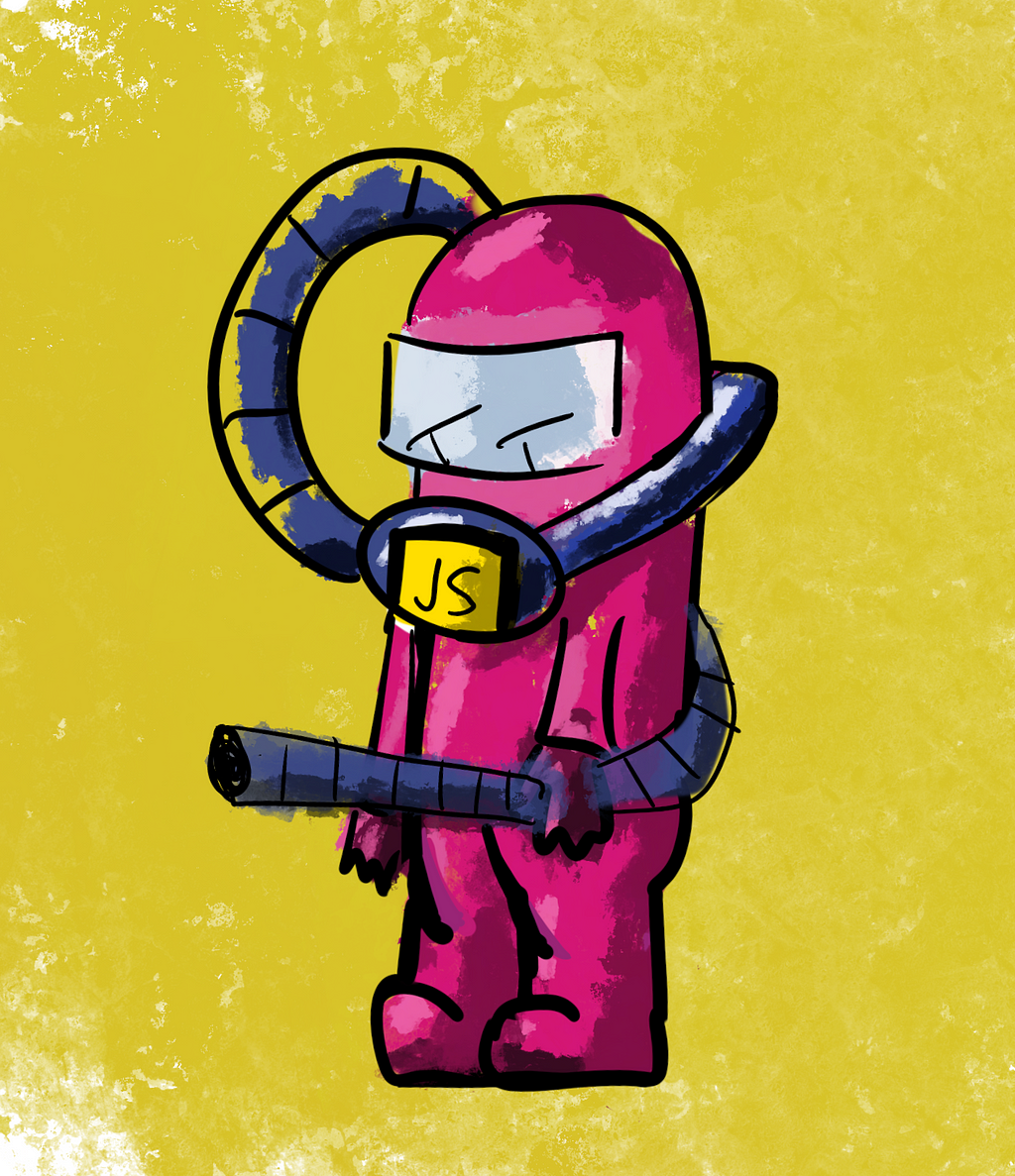
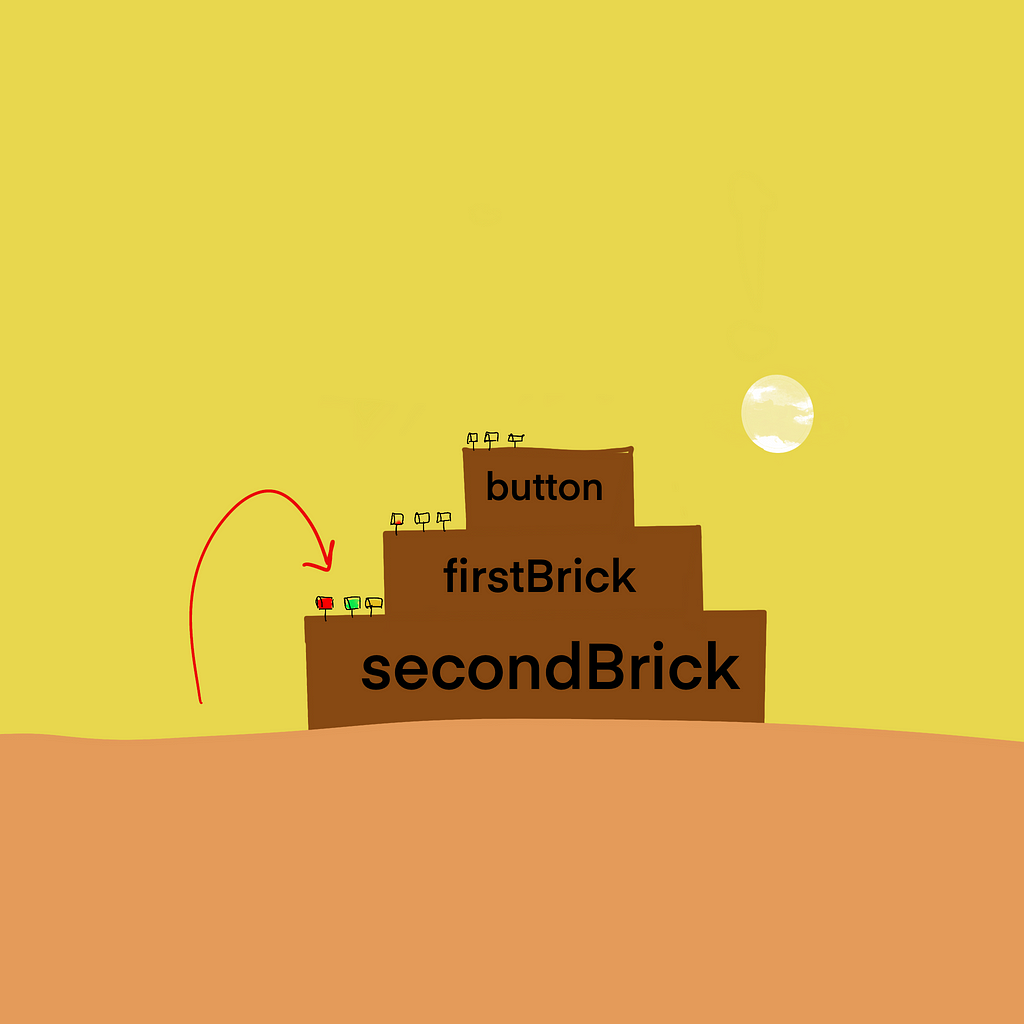
The Event Handler reads the goal of its mission (reach the target element and go back) and then jumps over the first brick. Here he’ll find the secondBrick’s mailboxes, open the ‘event click’ one and check if there is a callback inside. If there is anything to execute, it executes it and then jumps over the next brick.
It does that for each brick until he reaches his target element. Now, he checks the callback and then starts to jump down passing through the other side of the pyramid.
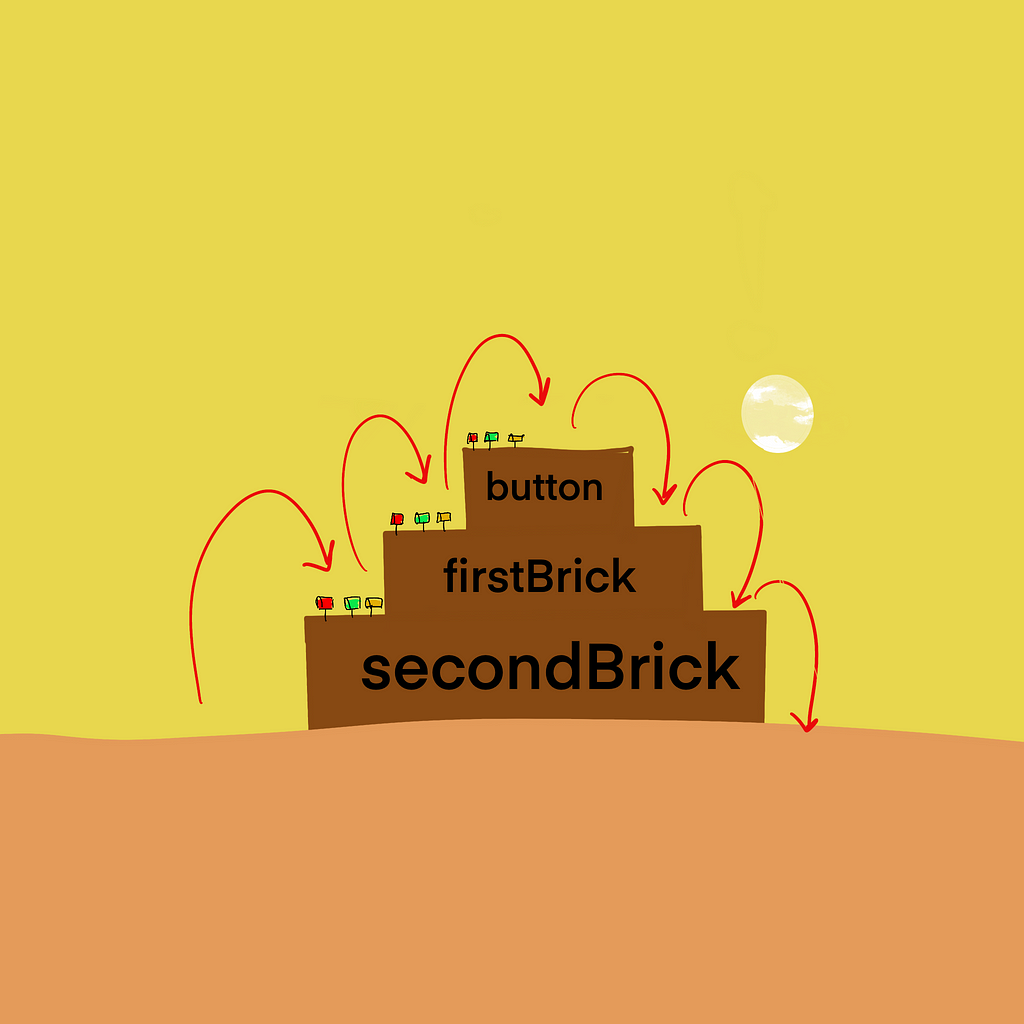
Note: the target element can be any element! For example, if the target element is secondBrick, the minion will just do that:
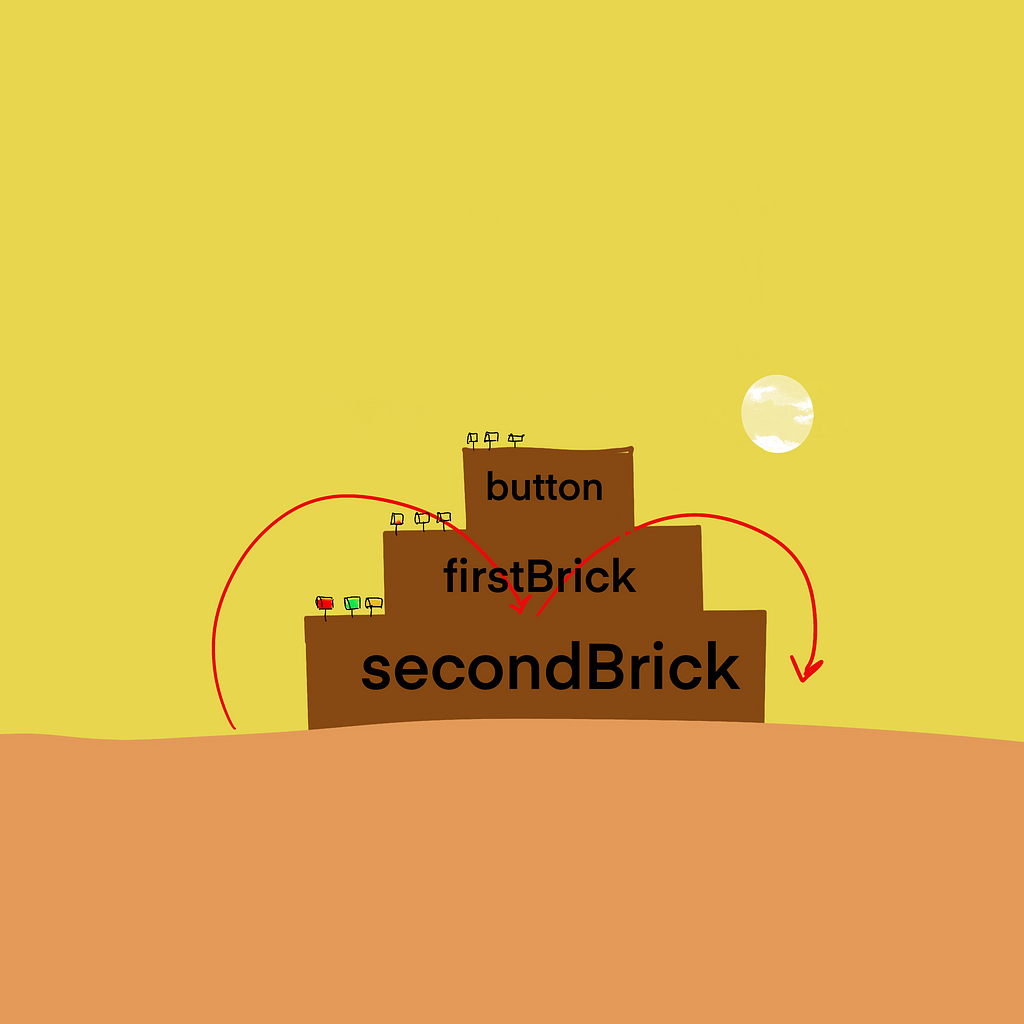
Now, if we assume that we registered a callback for each brick which prints the name of the brick we’ll get what follows:
- Event Handler jumps over secondBrick, finds a callback, and executes it.
- Event Handler jumps over firstBrick, finds a callback, and executes it.
- Event Handler jumps over button, finds a callback, and executes it.
- Event Handler jumps down over button.
- Event Handler jumps down over firstBrick.
- Event Handler jumps down over secondBrick.
The printed result will be:
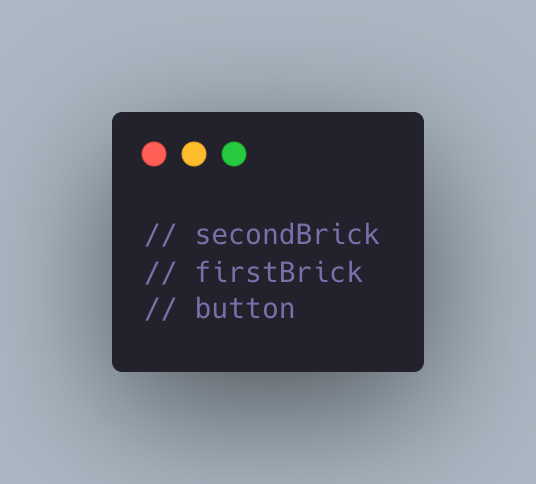
Cool, it’s easy! Now there is just a last small thing: the mailboxes can be on the right side of the pyramid as well!
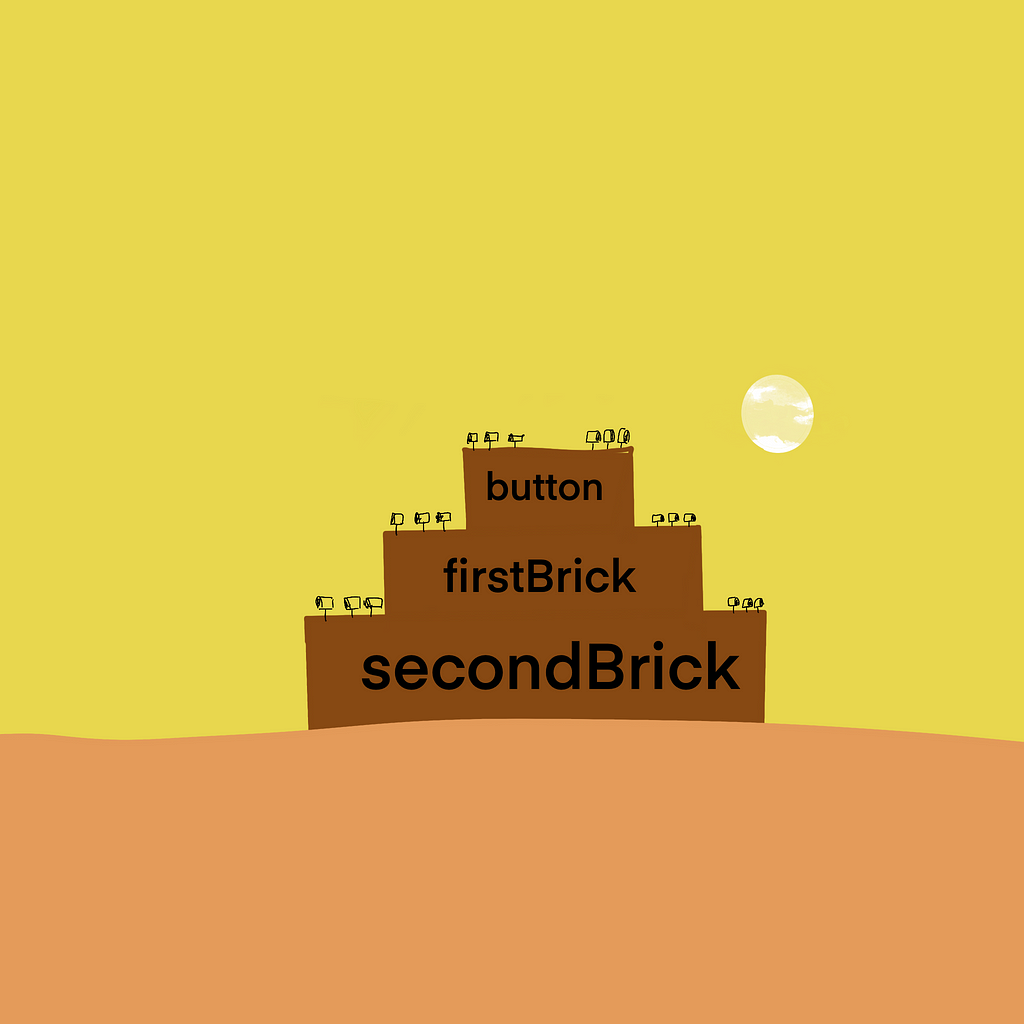
Bubbling: the pyramid descent
We saw that our minion (Event Handler) was executing the callback on the left side of the pyramid.
In Javascript, the climb is called capturing phase.
So, when the minion is going down on the right side
the descend is called bubbling phase.
Nothing more, now it’s just a matter of putting the envelopes on the correct side.
As you may remember, earlier we used this function to register the firstBrick’s callback:

addEventListener registers by default the callback on the bubbling phase, which is the right side of our pyramid.
Its third parameter isuseCapture (false by default) and you need to explicitly set it as true if you want to register your callbacks on the capturing phase, the left side of your pyramid.
You can decide in which side you want to register your callback by setting the third parameter of addEventListener with true (capturing) or false (bubbling).
Now that you know enough theory you won’t waste too much time with drawings, you just have to think about this pyramid, in which mailbox you registered the callback and make the minion jump!
You can find my first article (about drag and drop) here. If you liked this one follow me on medium, I’ll drop an article per week, stay tuned!
Learn Javascript EventListener while traveling in the desert was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Matteo Tasinato
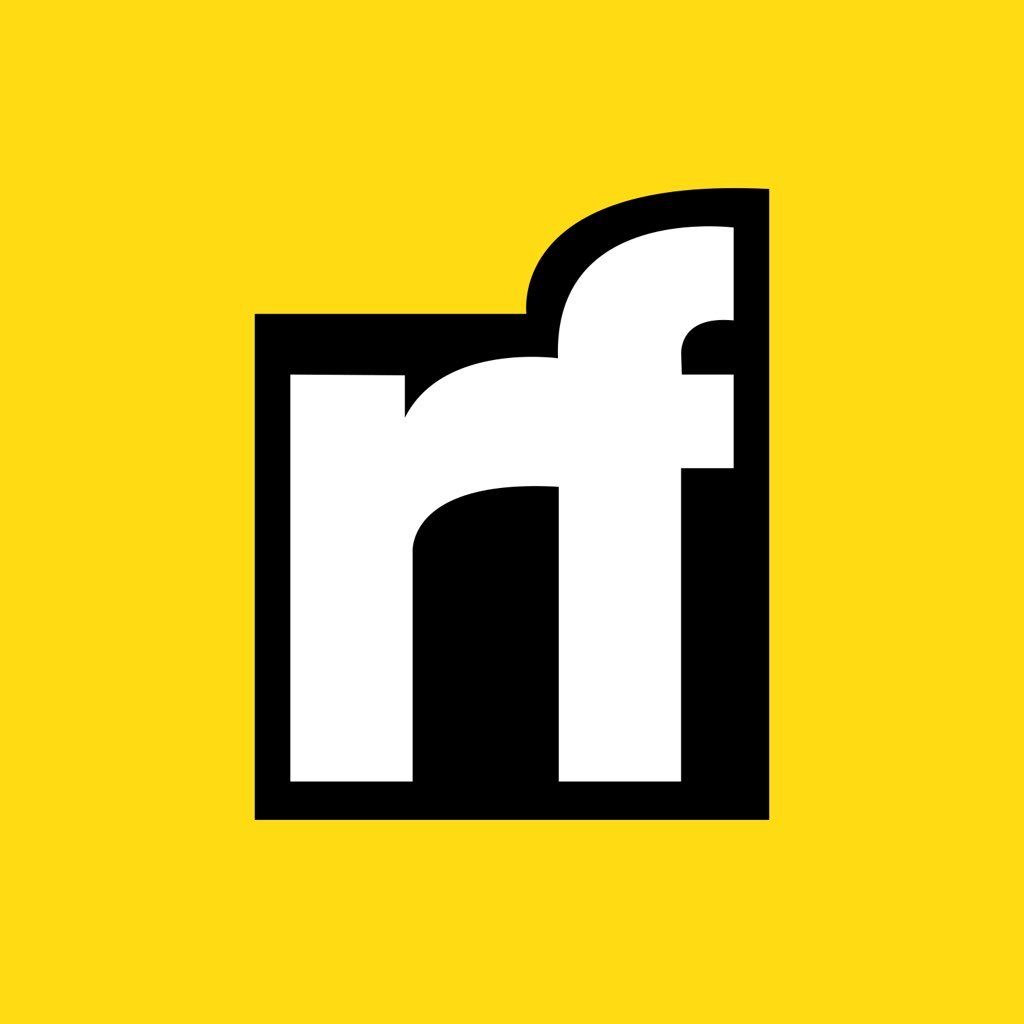
Matteo Tasinato | Sciencx (2022-04-27T01:17:56+00:00) Learn Javascript EventListener while traveling in the desert. Retrieved from https://www.scien.cx/2022/04/27/learn-javascript-eventlistener-while-traveling-in-the-desert/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.