This content originally appeared on DEV Community and was authored by Code Courses
FOR MORE FREE AND DETAIL COURSES, PLEASE GO TO HTTPS://CODECOURSES.SITE
Table of Contents
No. | Topics |
---|---|
1 | About Code Courses |
2 | Live Demo |
3 | Takeaway Skills |
4 | Course Overview |
5 | Prerequisites |
5.1 | Softwares |
5.2 | Technical Skills |
6 | Creating the React Project |
6.1 | Create React Counter App |
7 | Structuring the Project |
7.1 | Project Structure |
7.2 | Setting Up CSS |
7.3 | Build the Counter Component |
8 | Pushing the Project to Github |
9 | Creating a new Vercel Account |
10 | Deploying the Project to Vercel |
11 | Conclusion |
1. About Code Courses
Code Courses is a website where people learn about coding and different technologies/frameworks/libraries. For the purpose of helping people learn, all of the courses are FREE and DETAIL. For this reason, Code Courses believe that you do not need to buy any courses out there. Hopefully, after following the content on Code Courses, you will find your dream jobs, and build any applications that you want.
2. Live Demo
After we finish this course, the final output will be like this:
If you want to find the full source code, you can refer to this Github link.
3. Takeaway Skills
We can build the Counter application and understand how to apply React to create a real-life project. We can include this project in our profiles. It would help us a lot in finding a software engineer job. Aside from that, we can build other related applications with the skills we will get from this course.
4. Course Overview
As we see from the above image, we will have two buttons - increase and decrease. On the other hand, we also have a label that will be used to demonstrate the current result. After the users click on the increase button, the current result will be increased by 1. Aside from that, if the users click on the decrease button, the current result will be decreased by 1.
After finishing this course, we can understand how to apply React to build a real-life project.
5. Prerequisites
5.1. Softwares
- Install NodeJS.
- An IDE or a text editor (VSCode, Intellij, Webstorm, etc).
5.2 Technical Skills
- Basic programming skills.
- Basic HTML, CSS, JS, React skills.
6. Creating the React Project
In fact, we have several ways to create a new React project such as importing the React library from CDN links or using existing boilerplates/templates out there. In this case, we will create our React project by using the Create React App
Create React App is a comfortable environment for learning React and is the best way to start building a new single-page application in React. It sets up your development environment so that you can use the latest JavaScript features, provides a nice developer experience, and optimizes your app for production.
6.1. Create React Counter App
In this situation, to create our React counter app project, we need to follow the below steps:
Step 1: We'll need to have Node >= 14.0.0 and npm >= 5.6 on our machine. In case, If we have not installed Node.js, please click on the above link and follow its documentation.
Step 2: In order to make sure that we have installed Node.js on our computer. Hence, please open your terminal/cmd/power shell and run the following statement.
node -v
The result should be like this v16.10.0
.
16.10.0 is the Node.js version on my computer. Nevertheless, it may be different on your computer, depending on which version you have installed.
- Step 3: After we have installed Node.js on our computer. On our terminal, we need to use the below statements.
npm install -g create-react-app
create-react-app your-app-name
In addition, we need to replace
your-app-name
with the real name of our application. In this case, we want to build a counter app. For this reason, we will replaceyour-app-name
withcounter-app
. In conclusion, now our final statement should look like this:
create-react-app counter-app
- Step 4: Otherwise, we need to wait until the process is finished. After that, our result should look like this:
- Step 5: Now we can try to run our application. On the same terminal, please
cd
to your project folder.
cd counter-app
- Step 6: Following that, please run the below statement to start our React project.
npm run start
Our result should look like this
7. Structuring the Project
In general, we will talk about how we structure our project. In some other tutorials, we may see that those tutorials will tell you to store every component in the src
folder or develop everything in those files without caring about some best practices and principles. For example, we don't want to violate the DRY principle, DRY stands for don't repeat yourself
. For the most part, It means that we should avoid duplication in the business logic. Hence, to avoid that, we create some common files and folders. Therefore, we can reuse them in different places. With this purpose in mind, doing that helps us increase the readability, maintainability, and scalability of our code.
7.1. Project Structure
In this section, we talk about how to structure our project.
- Step 1: Firstly, please create a folder which is called
components
inside thesrc
folder.
The
components
folder will contain all of the components in our application. For example, the login component, the register component, the home component, and so on.
- Step 2: Moreover, we need to remove some unused files in this course. They are the
App.css
,App.test.js
,logo.svg
,reportWebVitals.js
,setupTests.js
.
- Step 4: In this situation, we are importing the
logo.svg
file in theApp.js
. For that reason, we need to remove it from theApp.js
file.
import React from "react";
const App = () => {
return <React.Fragment>Hello, Counter App</React.Fragment>;
};
export default App;
In the
App.js
file, we removed all of the dependencies and the current JSX elements. After that, we returned a React fragment. Inside that fragment, we showedHello, Counter App
.
- Step 5: In fact, We are importing
reportWebVitals
in theindex.js
file. However, we removedreportWebVitals
in step 3. Therefore, we need to remove it from theindex.js
file.
import React from "react";
import ReactDOM from "react-dom";
import "./index.css";
import App from "./App";
ReactDOM.render(<App />, document.getElementById("root"));
Now we can get back to our browser and the UI should be like this.
The full source code of the index.js
file will be like this:
import React from "react";
import ReactDOM from "react-dom";
import "./index.css";
import App from "./App";
ReactDOM.render(<App />, document.getElementById("root"));
The full source code of the App.js
file will be like this:
import React from "react";
const App = () => {
return <React.Fragment>Hello, Counter App</React.Fragment>;
};
export default App;
7.2. Setting Up CSS
In fact, we need to make our UI attractive. Therefore, in this section, we will set up CSS for our application. Hence, we do not need to care about styling because CSS was pre-prepared.
In the index.js
file, we are importing the index.css
file. This file contains all CSS for the application. We have different ways to set up styling for a React application, we can use the styled-components
library, or we can use the TailwindCSS
library, and so on.
Therefore, we will write all CSS in the index.css
file.
Please replace the current content of the index.css
file with the following content:
body {
margin: 0;
font-family: -apple-system, BlinkMacSystemFont, "Segoe UI", "Roboto", "Oxygen",
"Ubuntu", "Cantarell", "Fira Sans", "Droid Sans", "Helvetica Neue",
sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
background-color: #112b3c;
}
code {
font-family: source-code-pro, Menlo, Monaco, Consolas, "Courier New",
monospace;
}
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
input {
outline: none;
border: none;
}
::-webkit-scrollbar {
display: none;
}
.counter__container {
align-items: center;
display: flex;
gap: 1rem;
justify-content: center;
min-height: 100vh;
width: 100%;
}
.counter__container span {
color: #fff;
font-size: 5rem;
font-weight: 600;
}
.counter__container button:first-child {
padding-bottom: 0.25rem;
}
.counter__container button {
align-items: center;
background: #205375;
border-radius: 50%;
border: none;
color: #fff;
display: flex;
font-size: 3rem;
height: 5rem;
justify-content: center;
outline: none;
width: 5rem;
}
7.3. Build the Counter Component
As mentioned above, we will have two buttons - increase and decrease. On the other hand, we also have a label that will be used to demonstrate the current result. After the users click on the increase button, the current result will be increased by 1. Aside from that, if the users click on the decrease button, the current result will be decreased by 1.
To develop the counter component, please follow the below steps:
- Step 1: Please create the
Counter.js
file inside thecomponents
folder.
The component needs to update the current value whenever the users click on the increase or decrease buttons. To make the component show the updated result, we need to store the current result in a state. Whenever that state is updated, the component will be re-rendered and show the latest result.
- Step 2: To define a state, we need to import
useState
fromreact
in theCounter.js
file.
import { useState } from "react";
- Step 3: In the
Counter.js
file, we need to define a functional component that returns an empty fragment.
import { useState } from "react";
const Counter = () => {
return <></>;
};
export default Counter;
Currently, we just return an empty fragment, we will develop the UI in the next steps.
- Step 4: In the
Counter.js
file, we define a state which is calledcount
. thecount
state will store the current result.
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
return <></>;
};
export default Counter;
The default value for the
count
state is 0. It means that when we go to the application first time. If we reload the page, the current value will be 0.
- Step 5: In the
Counter.js
file, we update thereturn
statement as follows:
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div className="counter__container">
<button>-</button>
<span>{count}</span>
<button>+</button>
</div>
);
};
export default Counter;
We are using the
count
state in thereturn
statement. For this reason, whenever the users click on the increase or decrease buttons, we will update thecount
state, and the UI will be updated as well and it will show the latest result.As we can see from the above code snippet, we have not handled the events for the increase and decrease buttons, yet. We will do that in the below step.
- Step 6: In the
Counter.js
file, we associate the events for the increase and decrease buttons.
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div className="counter__container">
<button onClick={decrease}>-</button>
<span>{count}</span>
<button onClick={increase}>+</button>
</div>
);
};
export default Counter;
If the users click on the decrease button, the
decrease
function will be executed. Following that, if the users click on the increase button, theincrease
function will be executed.We have not defined the
increase
anddecrease
functions. We will do that in the next step.
- Step 7: In the
Counter.js
file, we define theincrease
function.
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
const increase = () => {
setCount((count) => count + 1);
};
return (
<div className="counter__container">
<button onClick={decrease}>-</button>
<span>{count}</span>
<button onClick={increase}>+</button>
</div>
);
};
export default Counter;
Inside the
increase
function, we calledsetCount
to update thecount
state, thesetCount
function accepts a callback function as its parameter. That callback function will have one parameter (the current count value). Therefore, we just need to get the current count value and increase it by 1
setCount((count) => count + 1);
- Step 8: In the
Counter.js
file, we define thedecrease
function.
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
const increase = () => {
setCount((count) => count + 1);
};
const decrease = () => {
setCount((count) => count - 1);
};
return (
<div className="counter__container">
<button onClick={decrease}>-</button>
<span>{count}</span>
<button onClick={increase}>+</button>
</div>
);
};
export default Counter;
The
decrease
function is similar to theincrease
function. > Inside thedecrease
function, we calledsetCount
to update thecount
state, thesetCount
function accepts a callback function as its parameter. That callback function will have one parameter (the current count value). Therefore, we just need to get the current count value and decrease it by 1.
The full source code of the Counter.js
file will be like this:
import { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
const increase = () => {
setCount((count) => count + 1);
};
const decrease = () => {
setCount((count) => count - 1);
};
return (
<div className="counter__container">
<button onClick={decrease}>-</button>
<span>{count}</span>
<button onClick={increase}>+</button>
</div>
);
};
export default Counter;
- Step 9: In the
App.js
file, we need to import theCounter
component that we defined above.
import Counter from "./components/Counter";
- Step 10: We need to use the imported counter component by updating the
return
statement in theApp.js
file.
import Counter from "./components/Counter";
function App() {
return <Counter />;
}
export default App;
The full source code of the App.js
file will be like this:
import Counter from "./components/Counter";
function App() {
return <Counter />;
}
export default App;
Now if we run our code, the UI will be like this:
8. Pushing the Project to Github
In this part, we will push our project to Github. GitHub is where over 73 million developers shape the future of software, together. Contribute to the open-source community, manage your Git repositories, and so on.
To push our project to Github, we need to follow the below steps:
- Step 1: Create the
.gitignore
file in your root directory with the following content.
node_modules
We do not want to push the
node_modules
folder to Github.
- Step 2: Go to this link, and then log in to Github with your created account.
Step 3: After we've logged in to Github, please go to this Link to create a new repository.
Step 4: We need to input the repository name and then click on the `Create Repository button.
- Step 5: We need to open the terminal,
cd
to the project folder, and follow the guidelines on Github
If everything is fine, you should see the below UI
9. Creating a New Vercel Account
In this section, we will create a new Vercel Account because we want to deploy our application on Vercel.
Vercel combines the best developer experience with an obsessive focus on end-user performance.
The platform enables frontend teams to do their best work. Vercel is the best place to deploy any frontend app. Start by deploying with zero configuration to our global edge network. Scale dynamically to millions of pages without breaking a sweat.
To create a new Vercel account, please follow the below steps:
- Step 1: Please go to this Link and click on the
Login
button.
- Step 2: Please log in with your Github account.
- Step 3: After logging in to the Vercel platform successfully, we will be on this page
10. Deploying the Project to Vercel
In this part, we will deploy our project to the Vercel platform.
As mentioned above, Vercel combines the best developer experience with an obsessive focus on end-user performance.
The platform enables frontend teams to do their best work. Vercel is the best place to deploy any frontend app. Start by deploying with zero configuration to our global edge network. Scale dynamically to millions of pages without breaking a sweat.
To deploy the project to Vercel, please follow the below steps:
Please make sure that we've logged in to the Vercel platform.
- Step 1: Please go to this link and click on the
Create Project
button.
- Step 2: Please click on the
Import
button to import our repository to Vercel.
Please note that the attached image is the screenshot at the time of writing this course. Your UI may be different. However, the purpose is the same, we want to import our repository to the Vercel platform.
- Step 3: Please input the environment variables, we just need to input all of the environment variables in our
.env
file. However, we do not have any environment variable in this project. Therefore, we can ignore that. After that, we need to click on theDeploy
button.
If everything is fine, we should see the below UI
11. Conclusion
Congratulation! We have finished the counter application by using React. In conclusion, we have known about the purposes of this course, and created and structured the project. Following that, we also developed the Counter component and deployed the application to Vercel. Thank you so much for joining this course, you can find many courses on Code Courses.
This content originally appeared on DEV Community and was authored by Code Courses
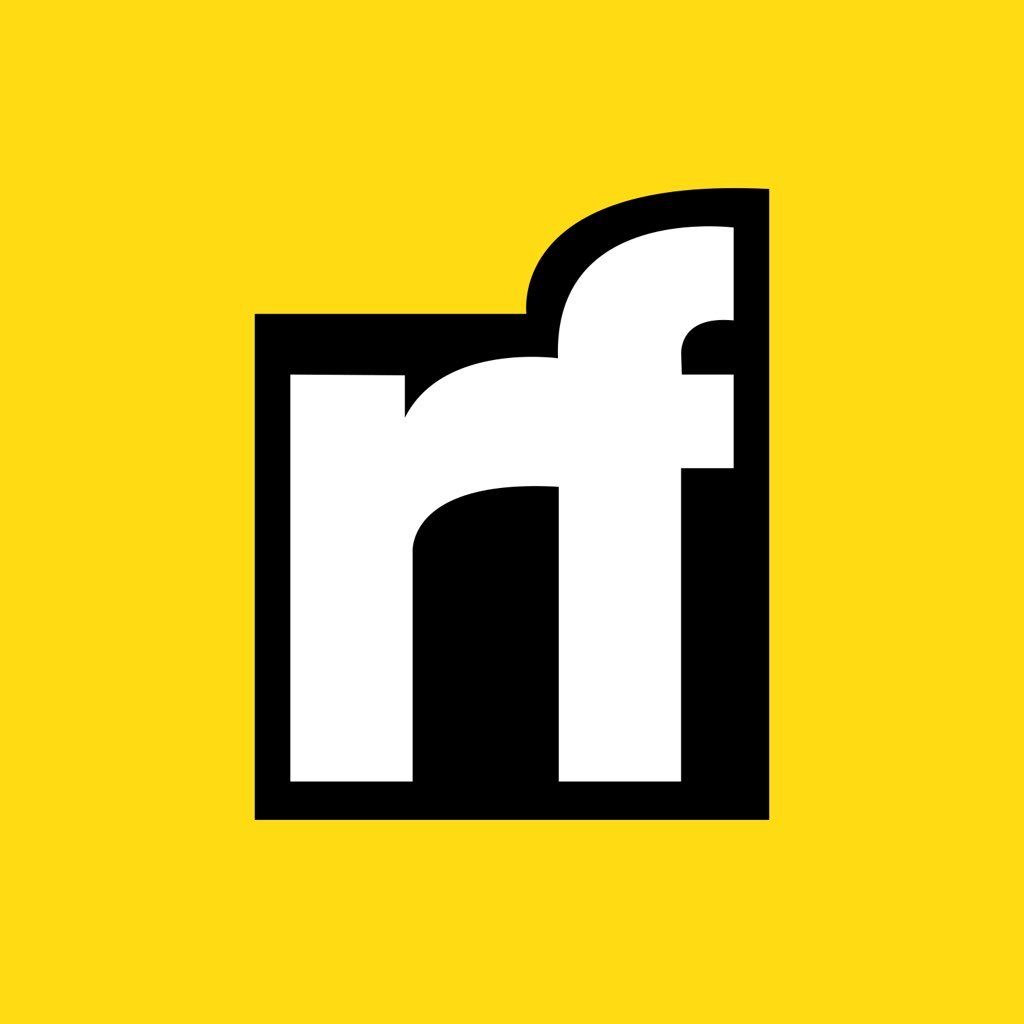
Code Courses | Sciencx (2022-05-10T11:36:28+00:00) How to Build Counter App in React [DETAIL COURSE]. Retrieved from https://www.scien.cx/2022/05/10/how-to-build-counter-app-in-react-detail-course/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.