This content originally appeared on DEV Community and was authored by Fesobi omoyemi
Error handling is an important part of any code you write. For example, if you are developing a library to be used by other developers, they don't want to deal with errors that might crop up. Those errors would either cause the library to break or would require extra effort on their part to resolve.
It's a good idea to plan for how you will handle errors before writing your software in the first place, but it's even more important to actually follow through and handle errors effectively once the software has been written. In this post, we'll examine a (contrived) hypothetical scenario and look at ways that we can handle errors in JavaScript.
Error syntax
When an exception occurs, the browser stops executing your script and displays an error message. The try...catch statement catches and handles these exceptions so that they don't cause the browser to stop running your program.
In general JavaScript error syntax is as shown below:
try {
code block to try
}
catch(err) {
code to handle the catched error
}
finally {
Block of code to be executed regardless of the try / catch result
}
The try block executes first and if an exception occurs or throws during the execution, the catch block is executed. The Finally block is executed whether an exception is thrown or not.
If there is no catch block in your program, then the program will terminate with an error message, whenever there is an error.
Using the Error Object
The Error Object is a special error handler that is used to handle runtime errors in JavaScript. This object allows you to identify the type of error that occurred, as well as its source and other information about it.
The runtime error can be a logic error, undefine variable or functions, or division by zero.
Consider the following scenario: if you're utilizing an external script in your software, you'll need to use try and catch in your code to handle any errors that may occur when the external script is updated, as shown below.
try {
username = doument.getElementById("username").value
password = doument.getElementById("password").value
if(validate(username, password)== true){
//login successfully
}else{
// username/password not correct
}
} catch (e) {
console.error(e.name + ': ' + e.message)
alert("an error occur, we are working to fix it!!!")
}
If the function validate in the external script is renamed or has an error, and it was not updated in the main script, it will generate a custom message for the user. Instead of throwing errors and terminating the program execution.
If the Validate() is been modified in the external script and you have not
Utilize Error Events
Error Events is a function that lets you handle errors that may occur when loading external HTML files in your program. It attaches a handler to the window object's error event. That way the script can be informed whenever an external resource fails.
Error events are used for handling HTML elements such as img, video, audio, object, frame, etc when their resource fails to load. Each element must have onerror attribute, containing a function to handle the error, as demonstrated below.
<img src="image.jpg" onerror="error_function()">
Whenever the image.jpg fails to load, the function inside the onerror attribute will be triggered for us to handle the error in our code as shown below:
function error_function() {
console.log("The image could not be loaded.");
}
We can also use the HTML DOM to assign an onerror event to an element. let's take for instance, a video element, if a video fails to load or its URL is invalid, we can display a custom message to the users as shown below:
<!DOCTYPE html>
<html>
<body>
<p>Handling javascript errors</p>
<img id="myImg" src="image.png">
<span id="demo"></span>
<script>
document.getElementById("myImg").onerror = function() {error_function()};
function error_function() {
document.getElementById("demo").innerHTML = "The image could not be loaded.";
}
</script>
</body>
</html>
Create Custom Errors
Javascript allows developers to throw an error exception if certain conditions are not met or to throw an exception on a particular line.
You can control your program flow and generate custom error messages by using throw state inside try statement. This gives you the opportunity to define your own error types.
throw "error message"
When the execution reaches throw, the custom exception message is thrown. Consider an example of how to use it.
function myFunction() {
const message = document.getElementById("p01");
message.innerHTML = "";
let x = document.getElementById("demo").value;
try {
if(x == "") throw "empty";
if(isNaN(x)) throw "not a number";
x = Number(x);
if(x < 5) throw "too low";
if(x > 10) throw "too high";
}
catch(err) {
message.innerHTML = "Input is " + err;
}
}
Conclusion
In this article we've learnt what errors are in JavaScript and how to handle errors in your programs in a way that makes it easy to maintain later.
If you have any comments, please feel free to leave them. Thank you for reading, and I hope you found the article useful.
This content originally appeared on DEV Community and was authored by Fesobi omoyemi
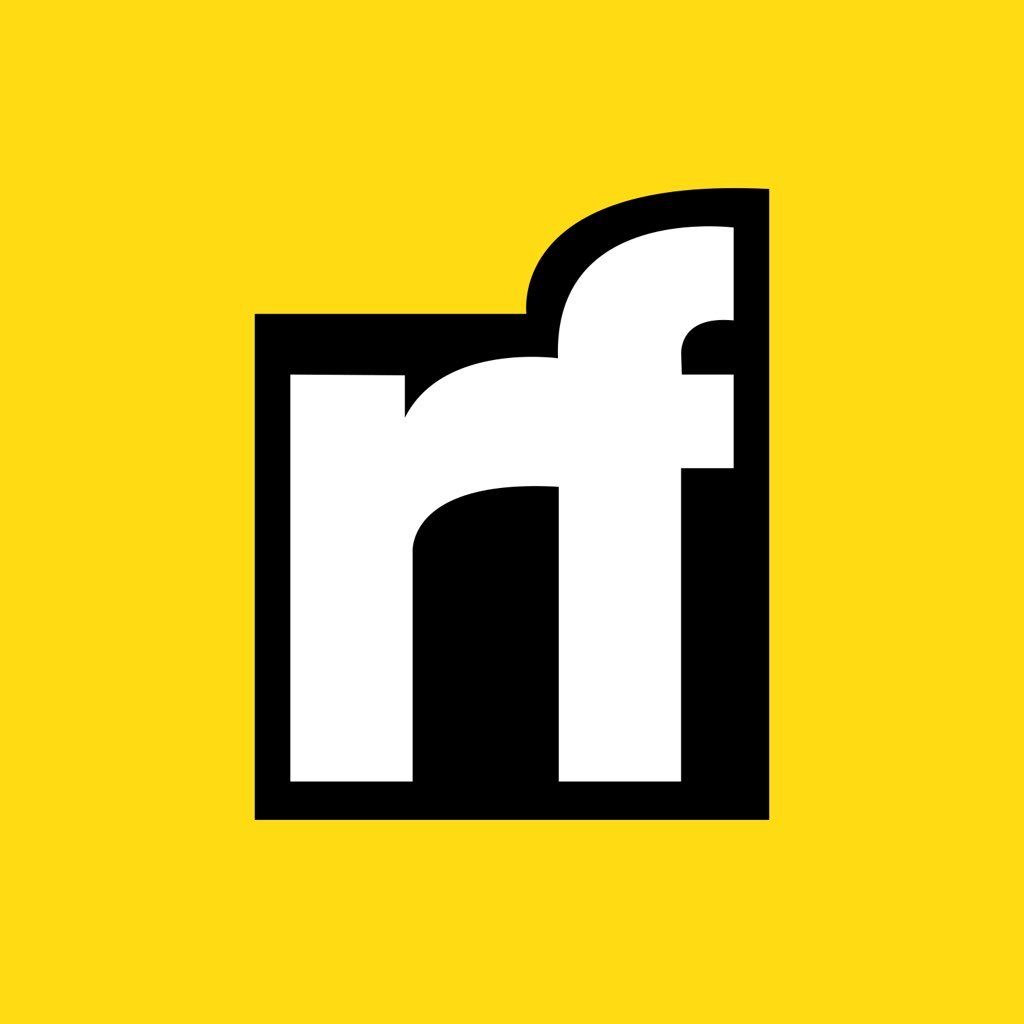
Fesobi omoyemi | Sciencx (2022-05-12T23:36:14+00:00) Handling Errors In JavaScript. Retrieved from https://www.scien.cx/2022/05/12/handling-errors-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.