This content originally appeared on Bits and Pieces - Medium and was authored by Akash Yadav
This will be a four-part blog series to understand how threading works and get some insights on knowledge of performing concurrent programming using different principles.
In this blog, we will first understand how code is executed in single-threaded and multi-threaded environments.
Sequential Execution
Single-Threaded
The first model we will look into is a “single-threaded sequential model”. This is the simplest style of programming. Each task is performed one at a time, with one finishing before another is started. It’s like you have only one worker to complete all three tasks, and the worker if given a task will complete it till its termination before picking up any other task.
Let’s consider a person cooking. He wants to cook bread-omelet. He starts to boil an egg and first waits for it till it’s boiled, then started to toast the bread and then consumes it.
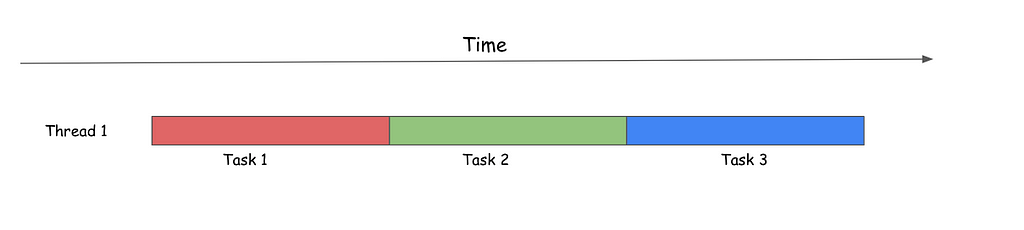
Multi-Threaded
Another model we will look into is a multi-threaded sequential model. In this model, the main thread is assigning tasks to child threads/workers but at the same time blocking over them to finish executing before assigning another task to some other worker thread.
Using the same analogy of cooking bread omelet above, Let’s say a person starts to boil an egg, after it is boiled her mom will toast the bread. The tasks are performed one after another and by different persons(threads).
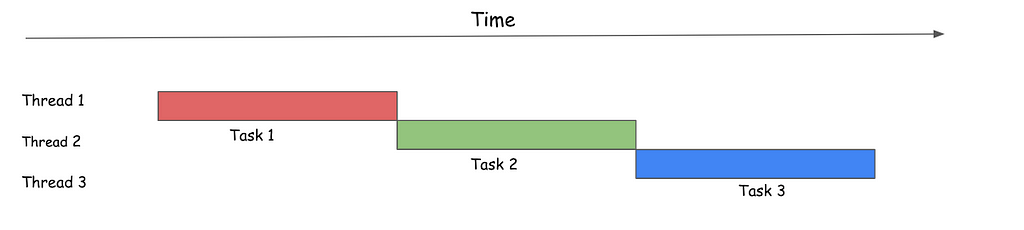
A similar and more modern code using flatMap to perform future composition:
Concurrent or Interleaved Execution
Concurrency is the capability to deal with lots of things at once. Let me take a simple analogy to explain.
Let’s consider a person jogging. During his jog, let’s say his shoelaces got untied. He halts for a moment to tie his shoelaces and then starts running again. This is a classic example of concurrency as the person is able to deal with both running and tying shoelaces at the same time.
Single-Threaded
Traditionally we have been using multiple threads to make progress on more than one task at a time, with each thread-making progress on its own individual task.
Single-threaded Concurrency means making progress on more than one task at a time from within a single thread. This pattern was made popular by Node.js for performing truly non-blocking I/O and achieving much high concurrency with less memory footprint. In a later blog, we will understand the motivation and advantages of using this model.

Coming back to the same bread-omelet analogy, I put the egg to boil and set a timer, I put the bread to toast and start another timer, when they are done, I can eat.
Now we can see what we mean by synchronous model and asynchronous model.
Synchronous execution means the thread that launched the task needs to wait for the task to complete to continue to work and asynchronous execution means that the task is executed at some point in the future and that there is no need to wait for anything for it to complete.
Asynchronous does not mean in another thread. Let’s take an example
List<String> li = new ArrayList<>(Arrays.asList("z","y","x"));
li.sort(Comparator.naturalOrder());
We are sorting the list of strings in ascending order, by providing an inbuilt comparator. The questions are
When does this comparator get executed? Sometime in the future.
In what thread is it executed? Main or same thread in which it is called.
Asynchronous and concurrent are two different notions. We can be asynchronous without being concurrent and being concurrent does not necessarily mean that we are asynchronous.
Multi-threaded
In this model, each task is performed in a separate thread of control. The thread is managed by the operating system in the case of kernel-level threads or by language runtime in the case of user-level threads aka green threads or co-routines. We will talk about this more in our successive blogs.
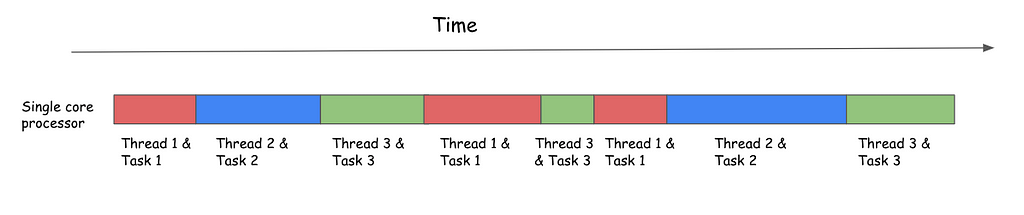
Coming back to the same bread-omelet analogy, I hire 2 cooks and they will boil the egg and toast the bread for me.
They can do it at the same time, and neither they have to wait for one to finish in order for the other one to start but they are cooking in the same kitchen using one resource:
Equivalent code by using Java future object and the callable pattern which is used to get data from one thread to another thread. This is a bit similar to channels in the go programming language which is used to communicate between go-routines.
Truly Concurrent or Parallel Execution
Multi-threaded
Parallelism is doing lots of things at the same time. It might sound similar to concurrency but it’s actually different. Let’s take an example of you getting a call from a friend you were talking to him and at the same time you got another call from another friend. You put both of them on a conference call and now you are talking to both of them at the same time.
For parallelism to occur we need hardware support, in this case, multiple CPU cores.
The same goes for the bread-omelet analogy, you hire 2 cooks and they will boil the egg and toast the bread for you. They can do it at the same time, and neither they have to wait for one to finish in order for the other one to start but they are now cooking in the different kitchens consuming different resources
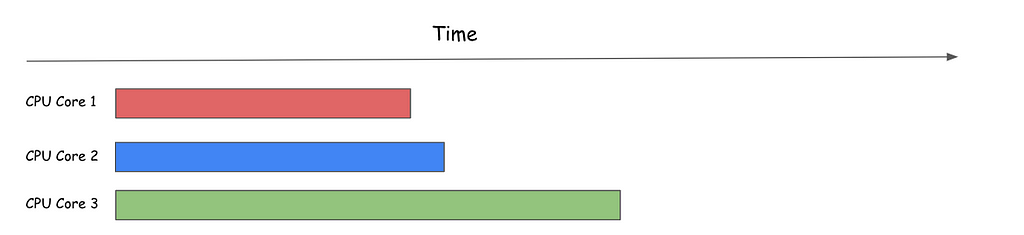
Thanks for reading this post. The next part can be found here.
I would love to hear from you
You can reach me for any query, feedback, or just want to have a discussion through the following channels:
Drop me a message on Linkedin or email me on akash.codingforliving@gmail.com
Build composable web applications
Don’t build web monoliths. Use Bit to create and compose decoupled software components — in your favorite frameworks like React or Node. Build scalable and modular applications with a powerful and enjoyable dev experience.
Bring your team to Bit Cloud to host and collaborate on components together, and speed up, scale, and standardize development as a team. Try composable frontends with a Design System or Micro Frontends, or explore the composable backend with serverside components.
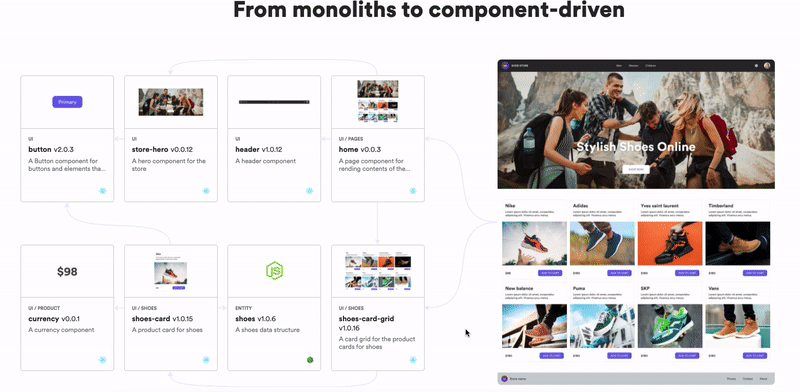
Learn more
- Building a Composable UI Component Library
- How We Build Micro Frontends
- How we Build a Component Design System
- How to build a composable blog
- The Composable Enterprise: A Guide
- Meet Component-Driven Content: Applicable, Composable
- Sharing Components at The Enterprise
Sequential vs Concurrent vs Parallelism was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Akash Yadav
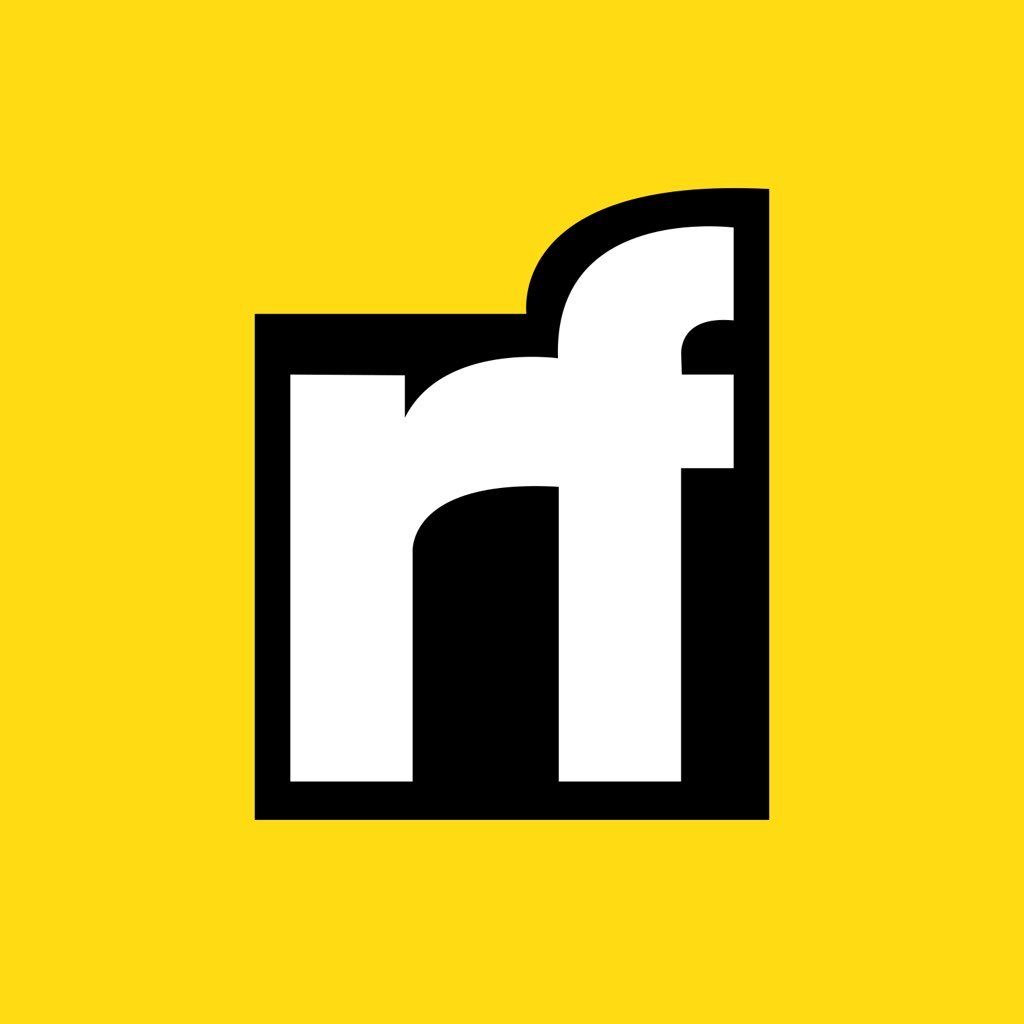
Akash Yadav | Sciencx (2022-06-03T11:43:58+00:00) Sequential vs Concurrent vs Parallelism. Retrieved from https://www.scien.cx/2022/06/03/sequential-vs-concurrent-vs-parallelism/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.