This content originally appeared on Level Up Coding - Medium and was authored by Joel Chi
React Context and the useContext Hook
React is a JavaScript library for building user interfaces. If you are not familiar with JavaScript, then check out this article — JavaScript, a brief introduction.
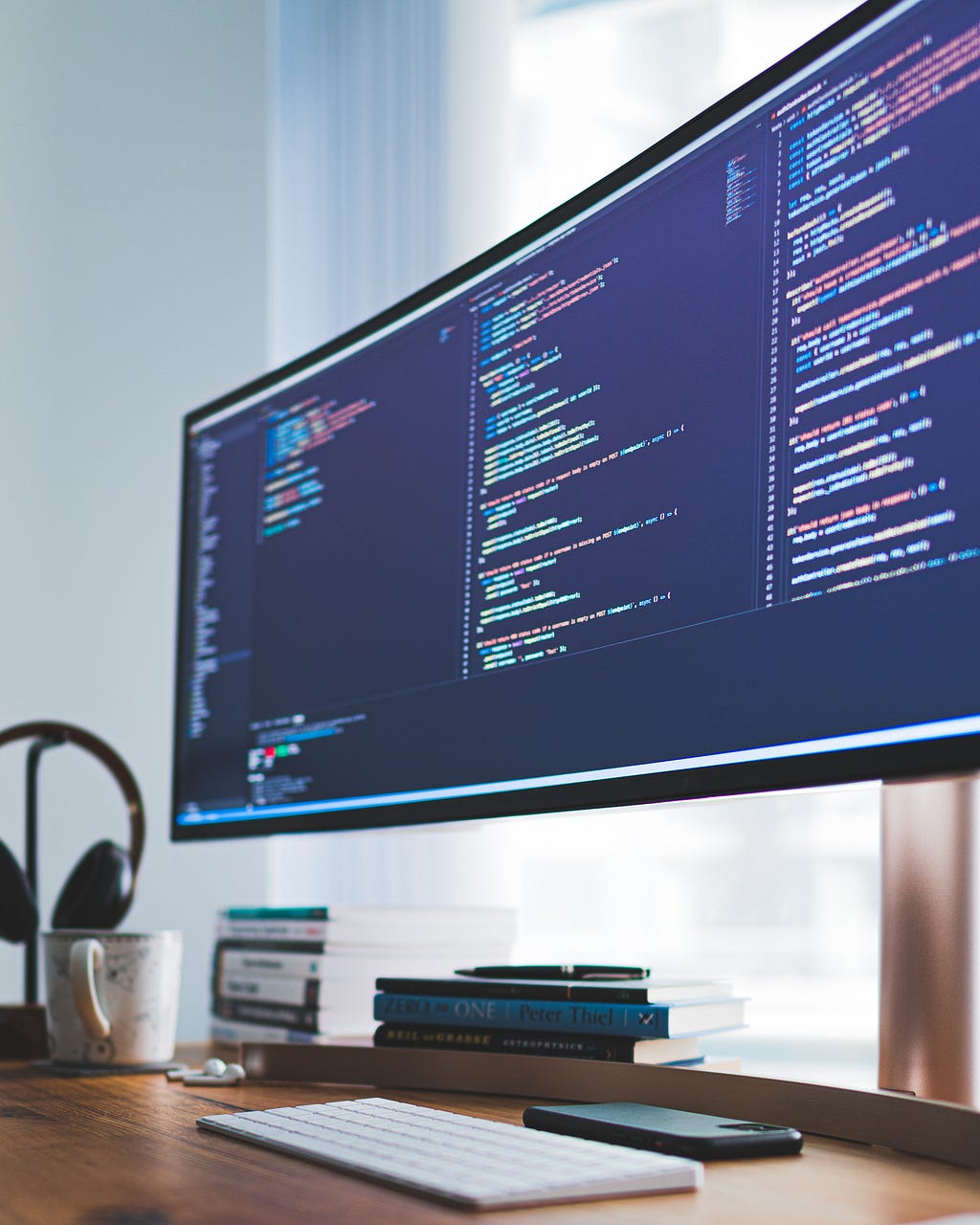
This article assumes you are familiar with the JavaScript framework React.
In this article, we will focus our attention on React Context — what it is and how to use it.
React Context
React Context provides a way to pass data through the component tree without having to pass props down manually at every level.
Context is designed to share data that can be considered “global” for a tree of React components, such as the current authenticated user, theme, or preferred language.
How do we use React Context?
There are three main steps in using React Context namely:
- Use the createContext method to create a context.
const MyContext = React.createContext();
2. Wrap the Provider method from the created context around our component tree passing to it the data to be consumed by our components as value.
export default function App() {
return (
<MyContext.Provider value="Some data to pass down">
<MyCompenent />
</MyContext.Provider>
)
}
3. Read the passed value within any child component by using the context consumer.
function ChildComponent() {
return (
<MyContext.Consumer>
{value => <h1>{value}</h1>}
</MyContext.Consumer>
)
}
Note: We can pass an initial value to our value prop when we call React.createContext.The created context is an object with properties: Provider and Consumer, which are components as seen above.
To use our passed down value, we use what is called the render props pattern, which is just a function that the consumer component gives us as a prop. In the return of that function, we can return and use value.
The useContext hook
Another way of consuming context became available in React 16.8 with the arrival of React hooks.
Simply put, hooks are a feature in React that let us use state and other React features without writing a class.
Thus, we can now consume context with the useContext hook as follows:
function ChildComponent() {
const value = React.useContext(MyContext)
return <h1>{value}</h1>}
}
We can clearly see how the useContext hook eases our life by making our components more concise and easier to read.
Conclusion
If we are not updating state, but merely passing it down our component tree, we do not need a global state management library like Redux. We can simply use React Context in such a case.
While it is possible to combine React context with a hook like useReducer and create a makeshift state management library without any third-party library, it is generally not recommended for performance reasons.
—-
If you like this article, please leave a few claps (or a lot) and make sure to follow me on Medium if you are not already. You can also subscribe to get an email whenever I publish. Thanks.
React Context and the UseContext hook was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Joel Chi
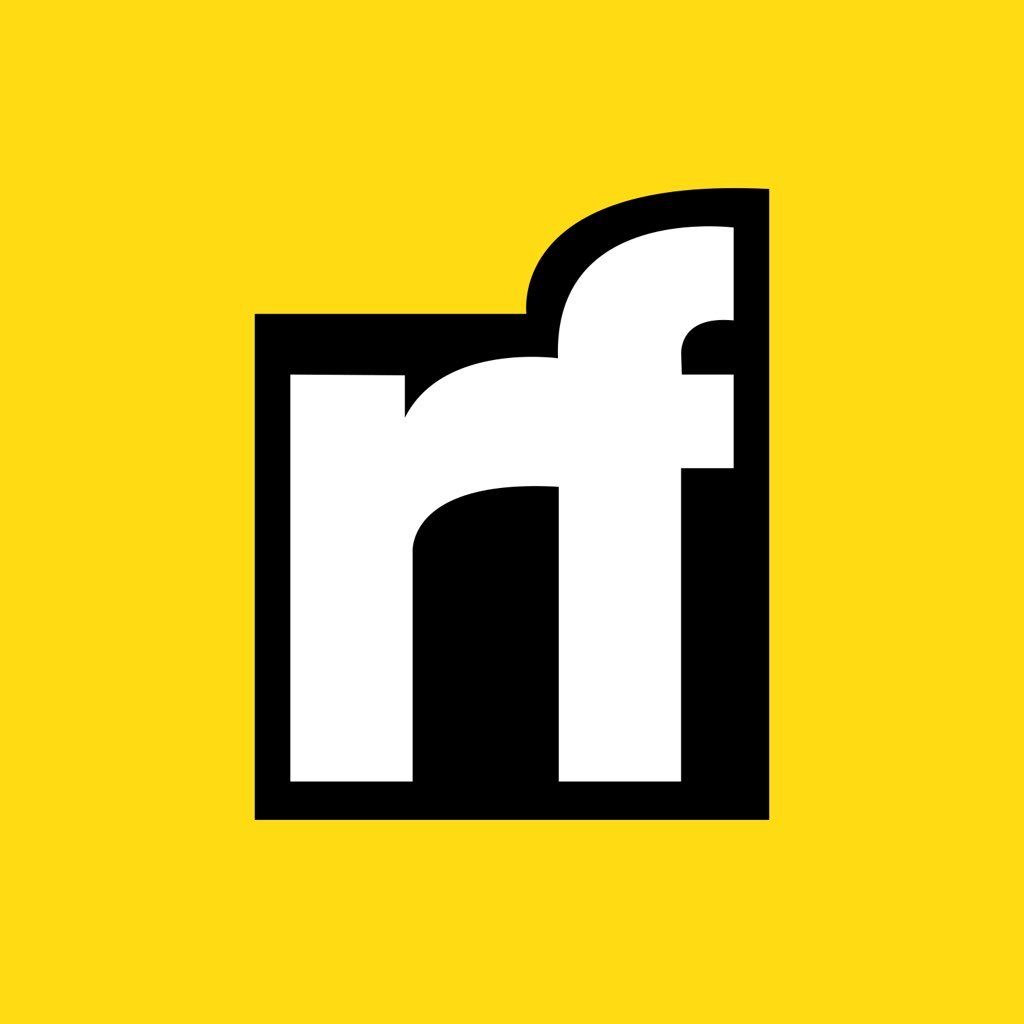
Joel Chi | Sciencx (2022-06-23T20:16:22+00:00) React Context and the UseContext hook. Retrieved from https://www.scien.cx/2022/06/23/react-context-and-the-usecontext-hook/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.