This content originally appeared on DEV Community and was authored by Rasaf Ibrahim
Rendering JSX conditionally is a very common and essential work in React. There are 4 ways in which we can render JSX conditionally in React.
4 ways of conditional rendering:
Ternary Operator
Logical Operator
if, else, else if
Switch Statement
Generally, developers don't use if else
or switch statement
inside JSX for conditional rendering. Because it takes more lines of code with if else
or switch statement
than ternary operator
or logical operator
. But when you have more than two conditions to deal with, you have to use if else
or switch statement
.
Ternary Operator
return (
<div>
{
'a'==='a' ? <p>Hi</p> : <p>Bye</p>
}
</div>
)
Note:
Only if the condition
'a'==='a'
is true,<p>Hi</p>
will be rendered in the screen. Otherwise,<p>Bye</p>
will be rendered on the screen.
Logical Operator - AND &&
return (
<div>
{
'a'==='a' && <p>Hi</p>
}
</div>
)
Note:
Only if the condition
'a'==='a'
is true,<p>Hi</p>
will be rendered in the screen.
Logical Operator - OR ||
export default function LogicalOperatorExample({name, labelText}) {
return (
<div>
{labelText || name}
</div>
)
}
Note:
If
labelText
andname
both props are passed into this component, thenlabelText
will be rendered on the screen. But if only one of them (name
orlabelText
) is passed as a prop, then that passed prop will be rendered on the screen.
if, else, else if
return (
<div>
{
(() => {
if('a'==='b') {
return (
<p>Hi</p>
)
} else if ('b'==='b') {
return (
<p>Hello</p>
)
} else {
return (
<p>Bye</p>
)
}
})()
}
</div>
)
Note:
Have to use an anonymous function (also need to immediately invoke the function )
Switch Statement
return (
<div>
{
(() => {
switch(true) {
case('a'==='b'): {
return (
<p>Hello</p>
)
}
break;
case('a'==='a'): {
return (
<p>Hi</p>
)
}
break;
default: {
return (
<p>Bye</p>
)
}
break;
}
})()
}
</div>
)
Note:
Have to use an anonymous function (also need to immediately invoke the function )
That's it.😃 Thanks for reading.🎉
This content originally appeared on DEV Community and was authored by Rasaf Ibrahim
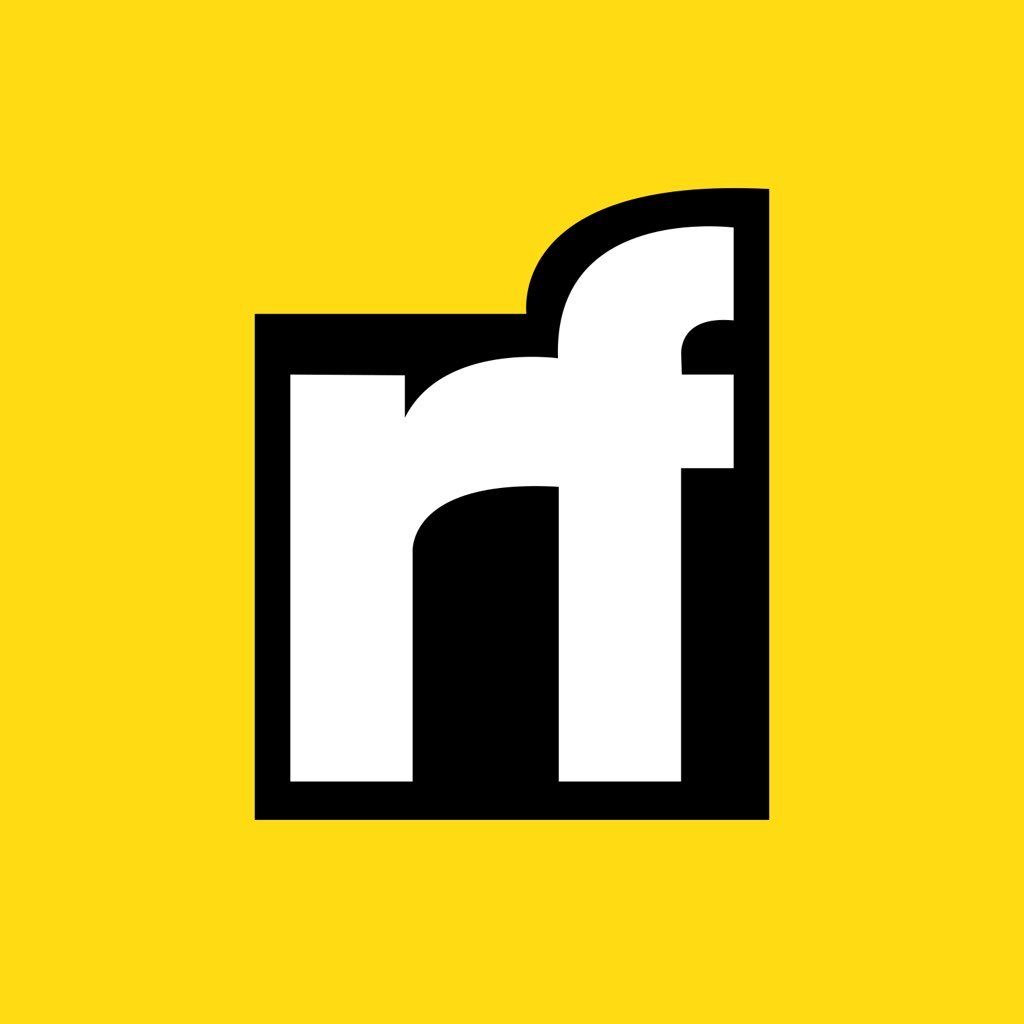
Rasaf Ibrahim | Sciencx (2022-06-24T16:58:44+00:00) 4 Ways to Render JSX Conditionally in React. Retrieved from https://www.scien.cx/2022/06/24/4-ways-to-render-jsx-conditionally-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.