This content originally appeared on DEV Community and was authored by Kai Wenzel
Introduction
Typescript is gaining more followers day-by-day. The type-safe cousin of Javascript is getting massively adopted and so does the projects and lines of TypeScript code written. This trend has been documented many times during the last years with the most important achievement being TypeScript voted as the 2nd most loved programming language for the year 2020 in the well-known Stack overflow developers survey.
The situation described above has lead many organizations to make a shift to TypeScript. Thus, large amounts of legacy code written in pure Javascript are being converted to TypeScript.
Recently, I took part in a migration project from Javascript to TypeScript for an established Software product with thousands of live users. The code base was large and complicated, so many things were refactored during the process but the final result was achieved without any serious drawbacks. This is because my colleagues and I, followed a very strict methodology and plan.
So let’s make a walkthrough over the process we followed in discrete steps.
The process
Step 1: Install TypeScript compiler and loader
You can do so by running the following command in the root directory of your project.
npm install --save-dev typescript ts-loader
Step 2: Replace any dependencies with the respective TypeScript version.
Most of the packages that are publicly available through package managers like npm or yarn, offer a TypeScript version as well. Most of times, TypeScript versions of some known libraries are prefixed with @types
. For example, the TypeScript version of lodash is the @types/lodash
package.
Step 3: Create a configuration file
As the official TypeScript documentation mentions:
TypeScript uses a file called
tsconfig.json
for managing your project’s options, such as which files you want to include, and what sorts of checking you want to perform.
The file should have the structure presented below (assuming that all the code of the project is located under the /src directory):
{
"compilerOptions": {
"outDir": "./dist/",
"noImplicitAny": true,
"sourceMap": true,
"module": "es6",
"target": "es5",
"jsx": "react",
"allowJs": true,
"moduleResolution": "node"
},
"include": ["./src/**/*"]
}
Step 4: Setup Webpack
Webpack is a static module bundler that will handle the process of combining all the .ts and .tsx source files and export only the needed .js files. The webpack.config.js file is needed in order to describe to webpack the way in which it will go through and process the source files. Below, you can see an example of a webpack config file that exports the processed code to bundle.js file (output key).
const path = require('path');
module.exports = {
entry: './src/index.ts',
module: {
rules: [
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/,
},
],
},
devtool: 'inline-source-map',
resolve: {
extensions: ['.tsx', '.ts', '.js'],
},
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
};
Step 5: Convert .js files to .ts and .jsx files to .tsx
Now it is time to do the actual conversion. You need to simply change the suffix / file type of every source file from .js to .ts and for the .jsx files, you need to change their suffix to .tsx (in case we have to to the conversion for a React project that uses JSX). Pretty straightforward uh ?
Step 6: Declare interfaces and types
If you have completed all the steps above correctly, you are done with most of the conversion process. In this point, the project should run correctly when you run the classic npm start
command on the root directory. However, you are not taking any advantage that TypeScript offers, as you have not still declared types and interface for your objects and variables.
Now, it is time to write your own types for complex objects and / or explicitly declare types for constants and variables that you are sure about the kind of value that they will get.
interface Options {
color: string;
volume: number;
}
TypeScript offers a variety of basic types that you can use with boolean
, string
, number
and enum
among them. You can view an extensive list on this link.
Troubleshooting
In case you face any issue during the process, do not hesitate to have a look on the following links:
https://www.typescriptlang.org/docs/handbook/migrating-from-javascript.html — Official migration guide
https://webpack.js.org/guides/typescript/ — Webpack documentation for TypeScript
TypeScript good practices
The following 2 practices will help you get the most out of this technology and avoid errors before they reach to production environment.
- Avoid the use of
any
andobject
types
These types are too generic and offer very limited value to error prevention.
- Make your custom interfaces as detailed as possible and use nesting
By using nesting in the interface level, you are making the application type-safe by keeping a modular architecture too. This practice will pay off as the size of the code base increases and you need to reuse the types that you declared.
Conclusion
TypeScript has changed many things in the web development world since its appearance. It seems that gradually, most of anything that “lives” on web will be refactored to TypeScript in order to take advantage of its enormous capabilities. I hope that this article will help you achieve a smoother transition to this new era for your projects.
*That’s it and if you found this article helpful, please hit the ❤️, 🦄 button and share the article, so that others can find it easily :) *
If you want to see more of this content you can support me on Patreon!
Thanks for reading and hope you enjoyed!
This content originally appeared on DEV Community and was authored by Kai Wenzel
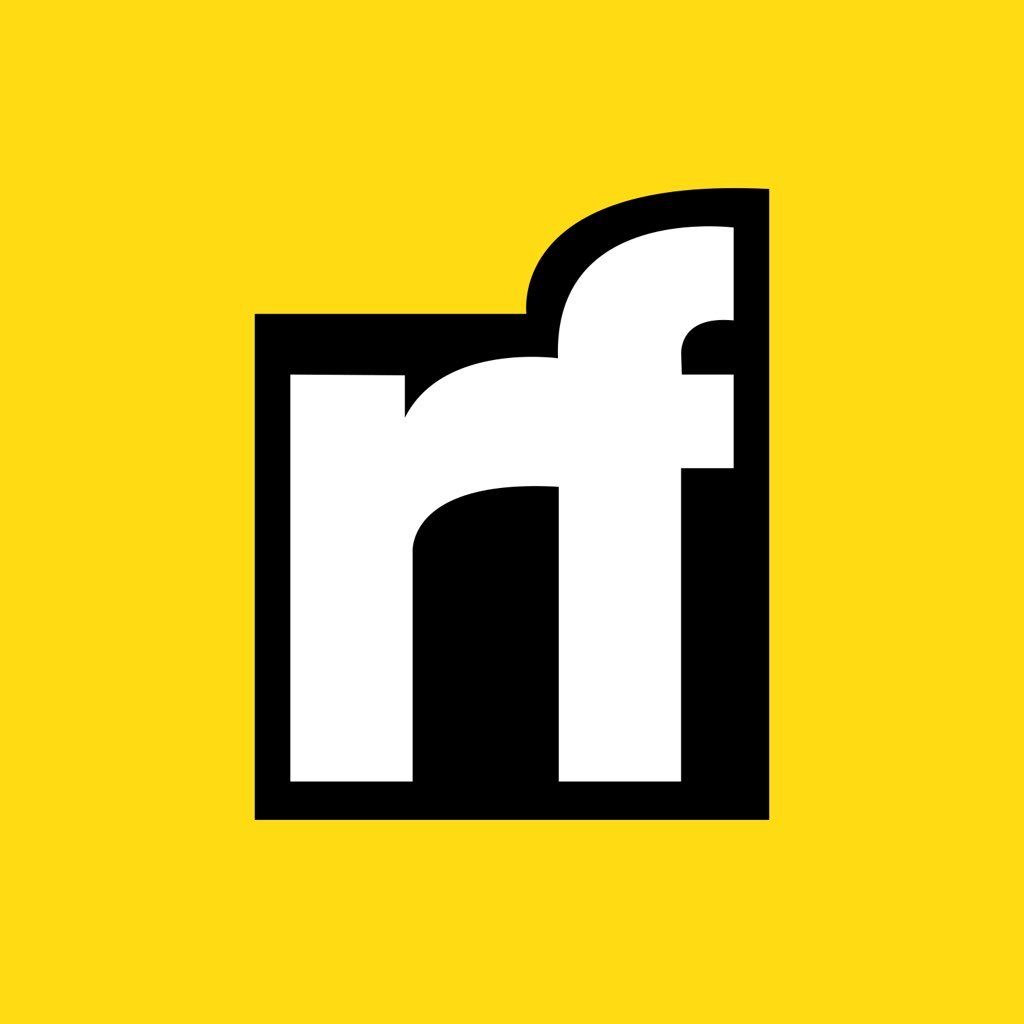
Kai Wenzel | Sciencx (2022-07-05T18:00:18+00:00) Converting a Javascript project to Typescript. Retrieved from https://www.scien.cx/2022/07/05/converting-a-javascript-project-to-typescript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.