This content originally appeared on Level Up Coding - Medium and was authored by David Bethune
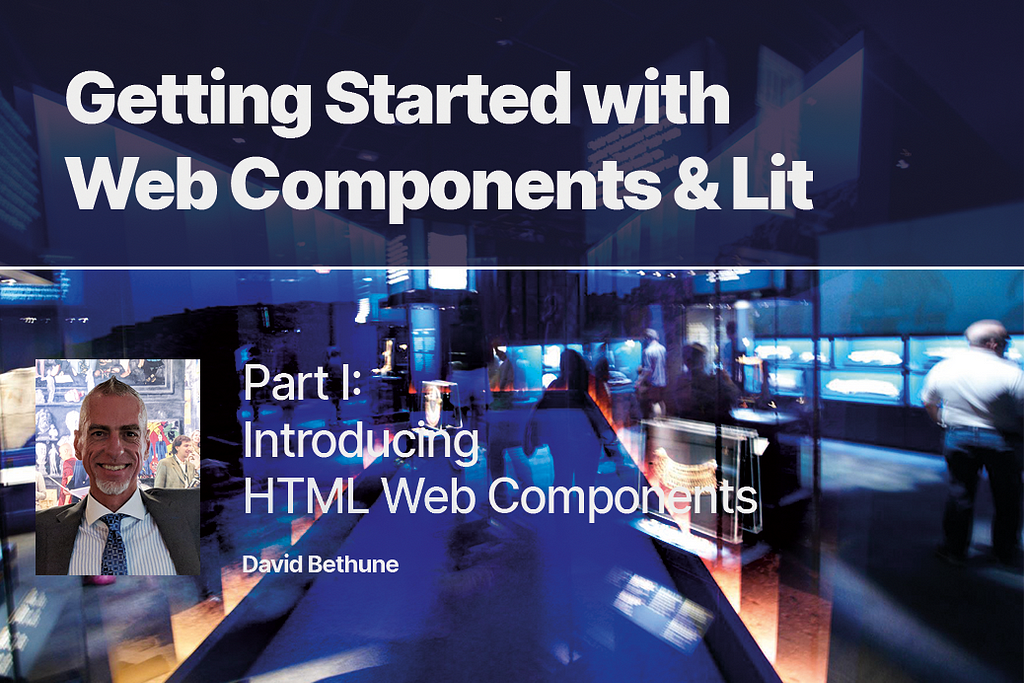
Part 1 | Intro to HTML Web Components
Lit, from Google, is a simple way to start working with web components. These are an upgraded form of HTML that solves many of the problems addressed by frameworks like Angular, React, and Vue. What’s different is that Lit also solves many of the problems those frameworks create. Read on to see why I call Lit the anti-framework and why I think you should strongly consider it for your next clean-sheet project.
Quick Index
Part 1: Introducing Web Components
Part 2: Setting Up Lit and Vite
Part 3: Building a Single Page App
The Problem of Interactivity
Since the earliest days of using JavaScript in web pages, many frameworks have tried to overcome the raw ugliness of dealing with the DOM. The first web pages didn’t have any interactivity precisely because this was so awful. Any JS program that has to inspect the actual HTML elements like <input> or <button> and change their content or behavior on the fly is going to look like spaghetti code immediately, because that’s what it is.
The earliest popular solution, jQuery, made it easier to address the individual elements of a page programmatically, but still left users writing a lot of raw, one-off code. The first true framework that gave programmers a way to treat web pages as applications was AngularJS. It had a huge set of noble goals. And it achieved them, at a heavy price.
Going Up?
Angular went beyond just talking to elements on the page. It came with a model for data binding, updating some behind-the-scenes data to reflect what was happening on the page. That model, called MVC or Model-View-Controller, was deeply baked into Angular’s design. It allowed for two-way dataflow between the model (a server or local data source) and the view (the page’s appearance to the user).
In theory, having data go up as easily as it comes down was terrific. A programmer’s dream, right? Susan changes her availability on a calendar and instantly the database on the back end has the new date. And AngularJS, the first iteration, worked this way.
In practice, two-way data was untenable. It was too easy to break your own stuff and preventing that breakage required too much scaffolding. Just as before, apps of any complexity quickly looked like spaghetti — and they were slow.
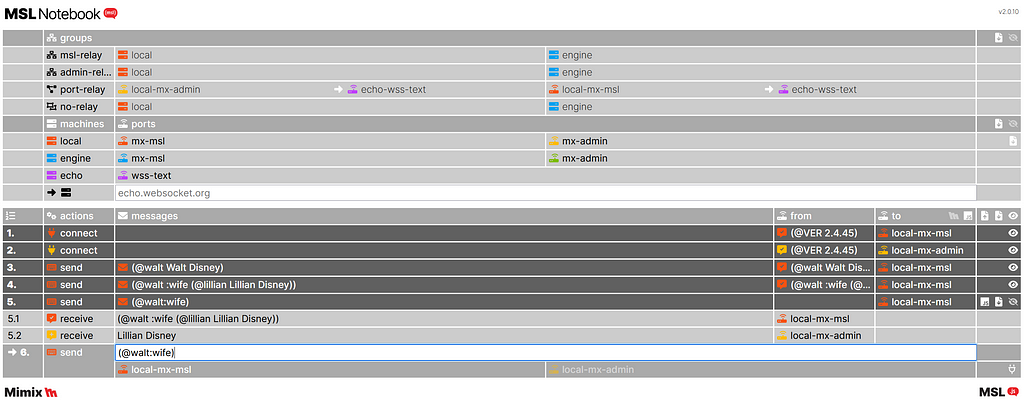
Angular and Friends: The Series
Before AngularJS became too wildly popular, Google realized these problems and killed it off by introducing a new version, named just Angular, and building all the later releases on the changed framework. The two are incompatible because new Angular does not allow two-way data binding.
Around this same time, Facebook (who could not really be seen using a Google framework), released their own MVC framework which they had created for internal use. That’s React. Vue was created by a former Google employee who had worked on Angular.
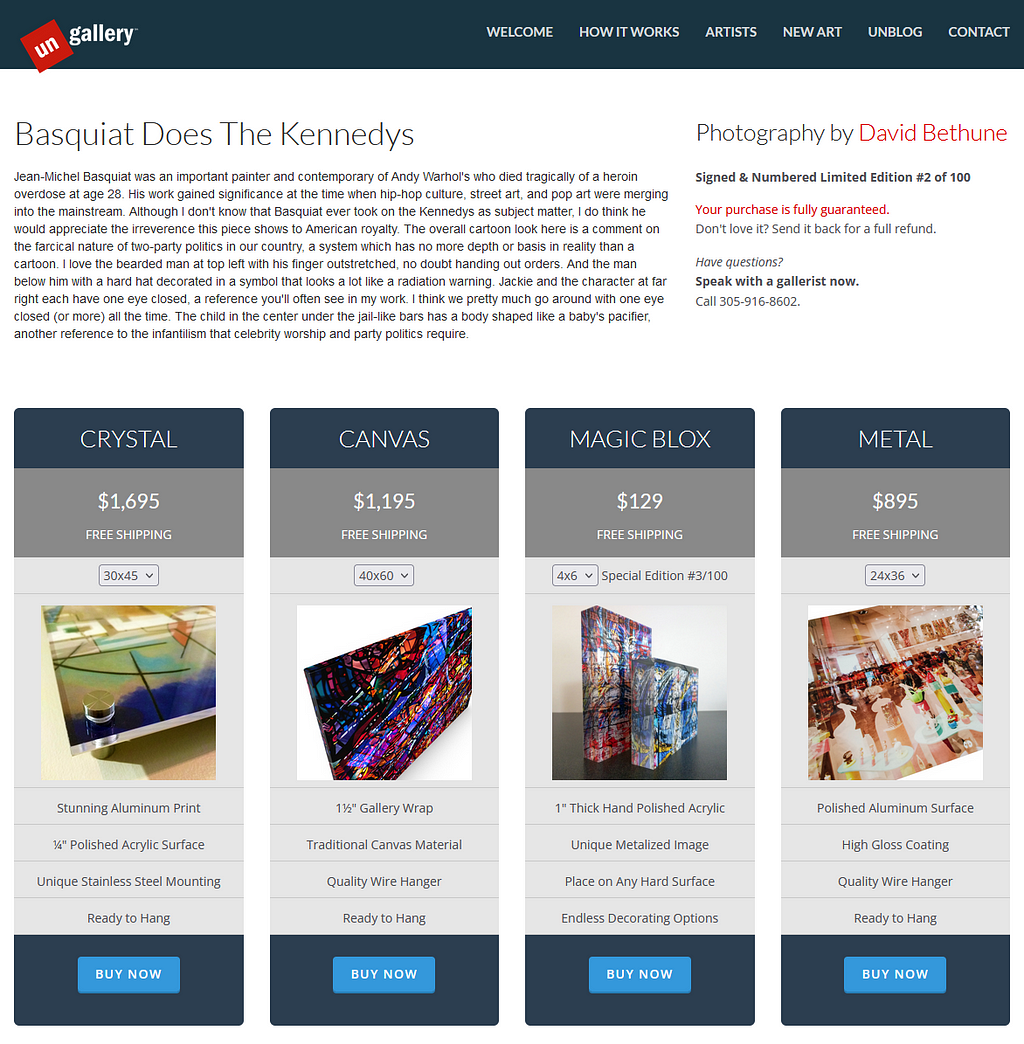
Enter Web Components
Seeing the profusion of frameworks which were all trying to address the same problems (ugly, inflexible DOM; no data binding), the W3C sought to improve the HTML standard itself by allowing programmers to write all of their own elements in a standardized way. These are web components.
In other words, I could decide that my pages would be made of <dta-page> elements filled with <dta-panel> content, not <div> and <p> elements. My custom elements would layout in any way that I choose. Because the attributes and values of these custom elements could also be set programmatically (in a standard syntax where different frameworks wouldn’t clobber each other), these HTML extensions would enable any kind of data binding.
A further goal of web components was to cleanup the problems of CSS style inheritance in the DOM. The cascading part of CSS (that “C” in the name) turns out to be difficult to debug. Sometimes, you just want your own CSS where you want it, without any cascading from above. In a terrible choice of names, the W3C selected shadow DOM for this concept of non-inherited CSS. In fact, it extends not only to styling but to the entire DOM, as the name implies. It’s good programming practice to stay away from the DOM, but I thought you should know.
A standard was also necessary to stop the walled garden effect that the giant frameworks were creating. React has hundreds of web elements that you can choose from and countless apps are full of these components. All kinds of dialogs, controls, sliders, calendars, etc. React has all of that. And that’s great, but… You have learn their way of doing everything. Should you want an input box or dropdown that doesn’t behave like React’s, well, you’re in for a world of hurt. Whatever you write must at least sort of conform to React because the whole rest of your app is in React, right?

Down to the Bare Metal
The opposite of a framework is bare metal HTML. That is, only stuff that’s in the MDN standard documentation for HTML, JS, CSS, and SVG — and nothing that’s controlled by a single vendor. A bare metal web component doesn’t require any import statements and doesn’t have any dependencies. It will work the same way in every browser on every device, from the smallest phone to the largest TV. It will not need upgrading in the future just because a platform said so.
Before I forget, nothing about web components or Lit precludes your using any other framework or any parts of the HTML spec. You can’t clobber anything with custom components, which is part of their design. This means you can use Lit to evolve an app from static HTML into more dynamic elements, without rewriting it from scratch. You can also use Lit to upgrade individual components from a different framework to custom ones you made in Lit without changing anything else about your page. You can even add Lit behavior to out-of-the box components from other libraries.
Now that web components are part of the bare metal, we can truly start to write apps that fix some of the problems we’ve had all along. They can be fully custom but also clean and modular. They can have as few dependencies as possible, yet be full of highly reusable code that we wrote. We can have our cake and eat it, too. So let’s get started!
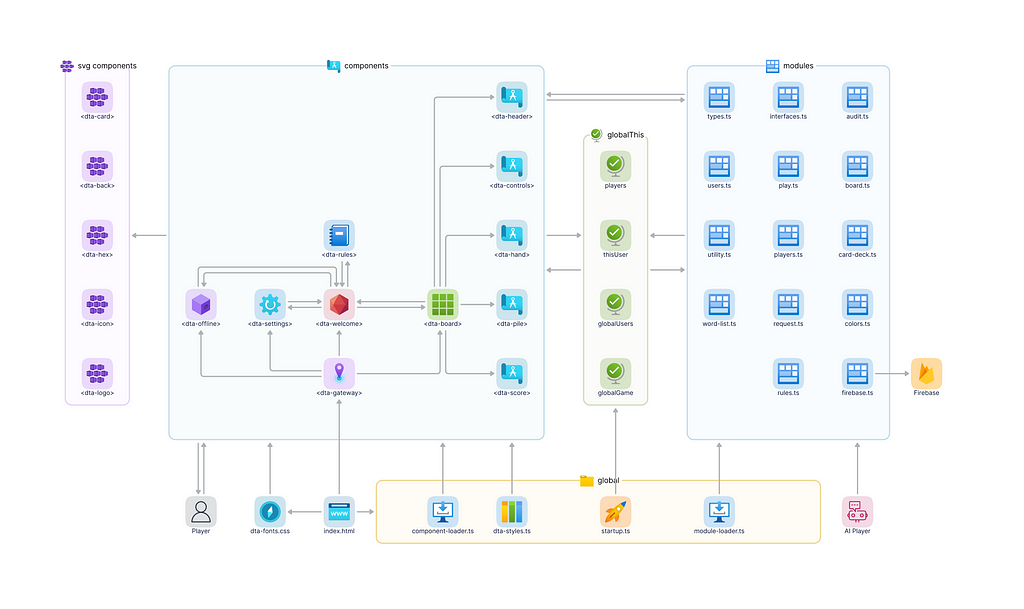
Lit is the Anti-Framework
Google’s latest HTML framework is as close to bare metal as you can get. While it does add data binding and event binding for you, in contrast with React’s huge component library, Lit offers zero components to choose from. You’ll be writing those. How about routing? Did you need that? Hope you can write it. (You can.) Does your app need any kind of security, identity, or storage? Wire it up, my friend!
If this sounds terrible, clearly you haven’t worked with React or Angular yet. Because, in reality, writing your own is often 5x better than learning the way one of these big frameworks does things. Instead of figuring out how to make what you want work within the “framework,” you simply write it the way you want it to work!
In practice, this is very ennobling. It’s also very productive because it’s easy to debug. Let’s take routing, for example. Google around for ways to do routing in Vue, or Angular, or React. Get coffee!

Now, in bare metal JS, old skool style, what is routing? It means taking something off the URL and going to a page by showing different content. Ok, in JavaScript that’s about 3 lines of code. Really. You parse the URL and then change the value of whatever variable is determining the page content being shown. This is not rocket science. Learning to use someone else’s routing framework, however, may take as long as learning rocket science!
If Lit will do the data binding for you, which is the secret sauce it adds to your bare metal web components, then when the “page content” variable changes, your app will automatically update so that all the HTML elements contain the correct new content. Congratulations! You’ve just written a single page app without a framework.
Yes, In Your Backyard
The airline business has a terrible automation problem. When pilots become too reliant on software, they make mistakes with the basics of flying the plane. The same can be said of developers. Lit encourages you to take ownership for all the parts of your app, which means understanding all the parts.
You cannot go back to Google and say, “I am having a problem with Lit” because it’s very unlikely that you are. You cannot say, “I don’t know enough about Lit to write my app,” because you can learn that very quickly. Thinking in web components and Lit forces you to think about the real architecture of your app and how it comes to life on the page. It also gives you deep debugging instincts when there are problems, as there will always be — because the problems are in what you are doing, not what Lit is doing.
This is why I recommend Lit for clean-sheet applications where you are starting from scratch. I also think it’s an excellent choice for new developers learning JavaScript (or, hopefully, Typescript) for the first time. Might as well start out right!

Thinking In Web Components
Writing an app with web components requires a different kind of thinking because all of the updated data must come top-down and it must flow through each component and its children without ever “reaching up” into data from anywhere else. When a component needs to tell another component about something (one that’s not its direct child), the first component must send a message asynchronously to the second component through the JS event system.
The components must not communicate in any other way other than by these messages. When a message is received, a component can redraw itself. When a parent component redraws, so do its children. This design constraint is the result of trying to write and debug apps which don’t work this way. It prevents many errors that are common in other design patterns. When architected correctly, web component apps are magical and beautiful because they appear to automatically update themselves on-screen with all the correct data.
Next Up… Setting Up Lit & Vite
In Part II of this series, we’ll setup Lit to work with VSCode. We also need a development server so we can see our app in progress, and for that I recommend Vite.
As always, thank you for reading and see you next time!
— D
Getting Started with Web Components & Lit | Part 1 was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by David Bethune
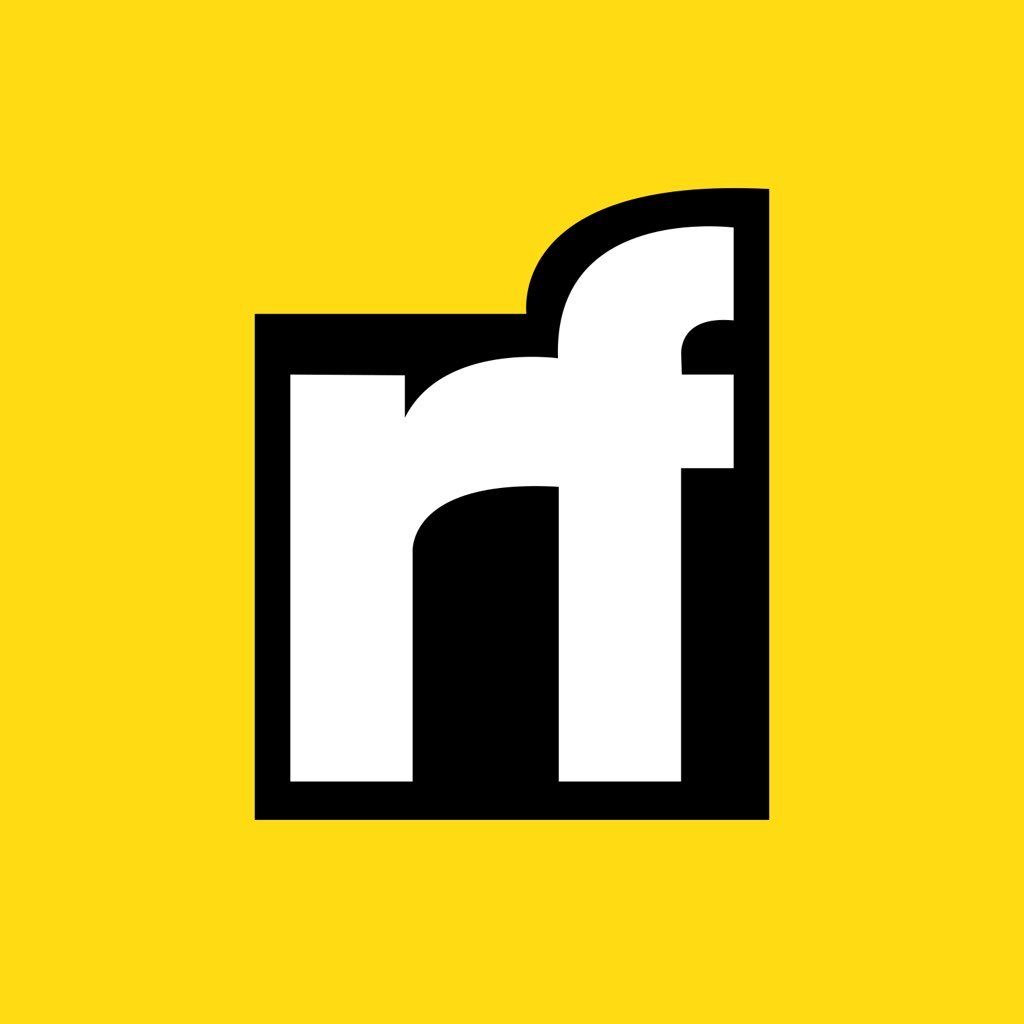
David Bethune | Sciencx (2022-07-05T12:18:00+00:00) Getting Started with Web Components & Lit | Part 1. Retrieved from https://www.scien.cx/2022/07/05/getting-started-with-web-components-lit-part-1/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.