This content originally appeared on DEV Community and was authored by jgifford82
In the first phase of my coding boot camp, I learned how to do a fetch request. It was confusing at first, but after a bit of practice, I realized how useful it can be.
A fetch request is a way of retrieving data from a database. The data can be added to the DOM after a page loads, which helps reduce rendering time. Fetch is a global method called on the window object.
There are a few different types of fetch requests, such as GET, POST, and DELETE. I’m focusing on the GET request, which was what I used for my phase 1 project. All you need to get started is a URL link to the database of your choice.
A basic way to set up a fetch request is to pass the URL string in as an argument, and add a couple of .then() methods that will convert the data to JSON and log it in the console. The data needs to be converted into JSON so you can work with it.
fetch(“URL of your choice")
.then(response => response.json())
.then(data => console.log(data))
You should see the data in the console log returned as arrays you can work with. This screenshot shows 3 nested arrays returned under object key results:
If you were to set up the request without converting the data to JSON, you’d only log the response from the fetch request in the console. This doesn’t provide data we can easily work with, so be sure to include a .then method that converts the fetch response data to JSON. Here’s an example of console logged data without being converted to JSON:
fetch("https://www.dnd5eapi.co/api/spells/")
.then(data => console.log(data))
From the basic fetch layout, we can build out what we want to happen with the fetched data within the second .then. In my project, I set up code to display the fetched data on the web page by creating and appending elements using forEach on the nested results arrays (see below). The entire fetch request was built within a function, which is invoked when a user submits something in a search form.
function fetchSpells() {
return fetch(`https://www.dnd5eapi.co/api/spells/?name=${document.getElementById("search-spells-form").value}`)
.then(response => response.json())
.then(data => data.results.forEach((element) => {
console.log(element)
let obj = element
const spellsDiv = document.getElementById('spells-container')
const ul = document.getElementById('spell-name')
const h3 = document.createElement('h3')
h3.innerHTML = obj.name
const a = document.createElement('a')
a.innerHTML = `https://www.dnd5eapi.co${obj.url}`
a.href = `https://www.dnd5eapi.co${obj.url}`
spellsDiv.append(ul)
ul.append(h3)
ul.append(a)
}))
When I was trying to set up the forEach method on the fetched data, I got stuck because I set it up like this:
.then(data => data.forEach(element => console.log(element)))
It returned an error “data.forEach is not a function.” After a couple hours of searching online, trial and error, and frustration, I figured out the issue after talking through it with someone. We realized that the data I wanted to use was nested in the object key “results.” I just needed to add “results.” before forEach to get it to work.
I also learned that it’s not necessary to state “method: GET” in that type of fetch request since that’s what it does by default. The method only needs to be stated for the other types of fetch requests.
Hopefully this helps others learning about fetch requests. Happy fetching!
Resource:
“Fetch API - Web Apis: MDN.” Web APIs | MDN, https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API.
This content originally appeared on DEV Community and was authored by jgifford82
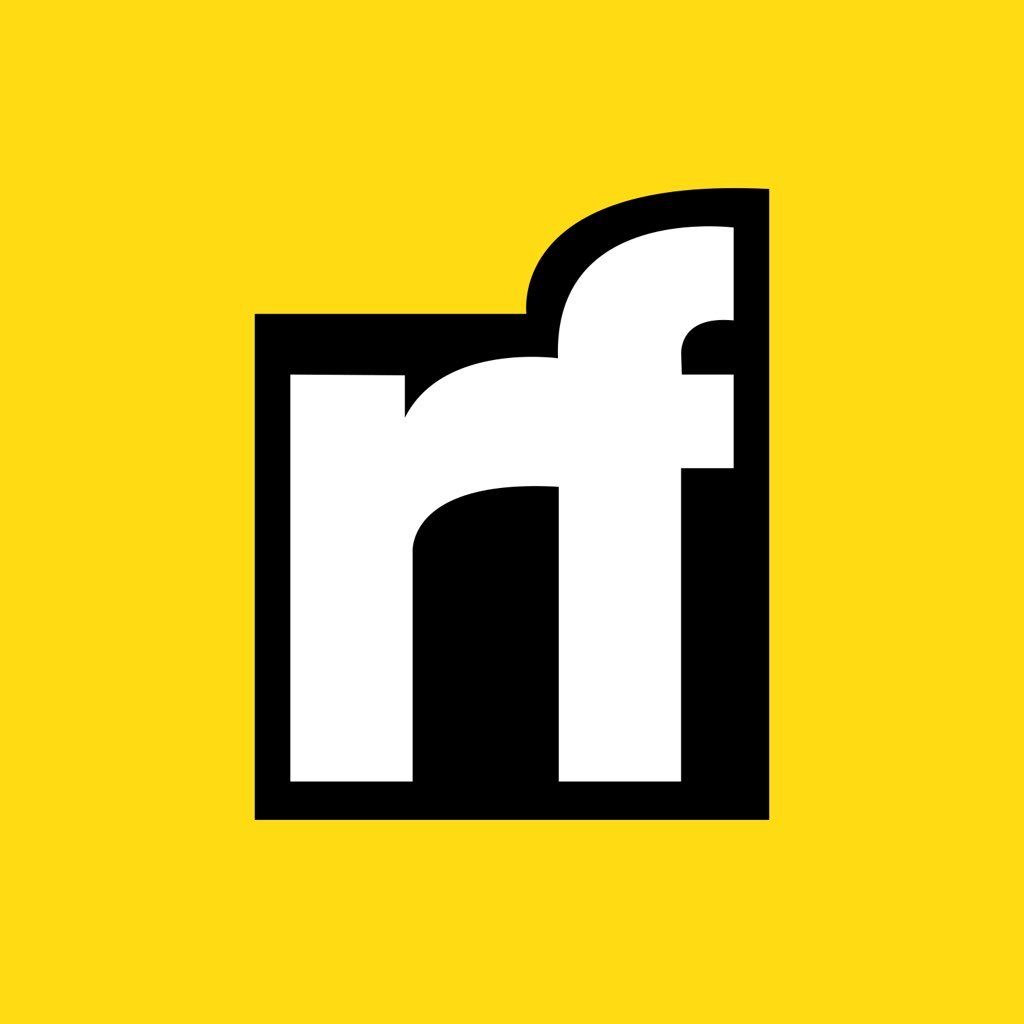
jgifford82 | Sciencx (2022-07-14T17:28:47+00:00) How to make a fetch request. Retrieved from https://www.scien.cx/2022/07/14/how-to-make-a-fetch-request/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.