This content originally appeared on Level Up Coding - Medium and was authored by Alex Powell

The Python Standard Library makes our lives as programmers a lot easier by providing a breadth of functionalities. We select a few libraries spanning data structures, filesystems, data persistence, and functional programming — which we deem most vital— to go over. Whether you are prepping for an interview or a simply trying to become a more proficient Python programmer, these are our top 7 must-know libraries.
1. Collections
The Collections module defines some useful data structures, including extensions of arrays and dictionaries.
Collections.Counter
Collections.Counter is useful because it sets default values of a non-existent key to zero. One can delete a key in the same manner one would delete a key in a Dictionary.
>>> from collections import Counter
>>> c = Counter()
>>> c['nonexistent_key']
0
>>> c['a']=3
>>> c['b']=4
>>> c
Counter({'a': 3, 'b':4})
>>> del c['a']
>>> c
Counter({'b'}:4)
Collections.deque
A deque is a doubly-ended queue. The most common operations on a deque are append, appendleft, pop, and popleft. All of these functions run in O(1) time, because the underlying data structure is a linked list.
>>> from collections import deque
>>> d.append('c')
>>> d.appendleft('b')
>>> d.appendleft('a')
>>> print(d)
['a', 'b', 'c']
Collections.defaultDict
A defaultDict allows you to set the values of a dictionary to some pre-set value. A default dict can be initialized using a type such as a list, in which case the value will be set to the
from collections import defaultdict
s = [('a', 1), ('a', 2), ('b', 3)]
>>> d = defaultdict(list)
>>> for k, v in s:
... d[k].append(v)
...
>>> sorted(d.items())
>>> [('a':[1, 2]), ('b':[3])]
Collections.orderedDict
In a regular Python Dict object, the keys are not ordered. An OrderedDict, on the other hand, maintains key order.
>>> form collections import OrderedDict
>>> d = OrderedDict.fromkeys('xyz')
>>> d.move_to_end('x')
>>> ''.join(d)
'yzx'
>>> d.move_to_end('y', last=False)
>>> ''.join(d)
'zxy'
2. Itertools
You can use Itertools instead of defining your own iterators when working with operations like permutations and cartesian products. Though it’s not hard to create your own iterator, using Itertools makes your code more concise and is considered more idiomatic.
Itertools.accumulate
The accumulate function takes prefix sums of an array.
>>> from itertools import accumulate
>>> x = [1, 2 ,3]
>>> res = accumulate(x)
>>> for item in result:
... print(item)
[1, 3, 6]
Itertools.product
The product function returns an iterator that iterates over the cartesian product of two or more lists.
>>> from itertools import product
>>> x = [1, 2]
>>> y = ['a', 'b']
>>> res = product(x, y)
>>> for item in result:
... print(item)
(1, 'a')
(2, 'a')
(1, 'b')
(2, 'b')
Itertools.permutations
Itertools.permutations gives you all permutations of a list.
>>> from itertools import permutations
>>> x = [1, 2, 3]
>>> res= permutations(x)
>>> for each in res:
... print(each)
(1, 2, 3)
(1, 3, 2)
(2, 1, 3)
(2, 3, 1)
(3, 1, 2)
(3, 2, 1)
Itertools.repeat
Itertools.repeat returns an iterator that yields a certain value a set number of times.
>>> from itertools import product
>>> for i in itertools.repeat("a", 3):
... print(i)
a
a
a
3. Bisect
bisect_left & bisect_right
Bisect gives you super useful binary search functions: bisect_left and bisect_right. Bisect_left, for example, returns the left-most index at which to insert an item (pushing other elements to the right), to make the array sorted. Bisect_right inserts at the right-most index.
Binary search is a common interview topic — you can use this built-in function as a subroutine so you don’t have to implement your own binary search from scratch!
>>> from bisect import bisect_left
>>> x = [1, 2, 3, 3, 7, 9]
>>> bisect_left(x, 3)
2
4. Heapq
A heap is one of the most common data structures. By default, a heap created using heapify is a min-heap. Heap push and pop operations have logarithmic time complexity. Min-heap is also a commonly-used data structure in coding interviews.
>>> from heapq import heappush, heappop, heapify
>>> x = [6, 2, 1, 4, 5]
>>> heapify([x)
>>> x[0]
1
>>> heappop(x)
1
>>> x[0]
2
5. os
The os module provides functionalities relating to the operating system, including functions for manipulating filesystems.
os.listdir
This function lists all the files and directories in a path (to a directory).
>>> import os
>>> os.listdir("/my_project")
.git
.gitignore
src
tests
os.mkdir
This function lets you create a new directory.
>>> import os
>>> os.mkdir("/my_new_project")
os.rename
This function renames a file or directory from src to dst.
>>> import os
>>> os.rename("/note1.txt", "/note2.txt")
os.is_dir & os.is_file
These functions let you find out if a path corresponds to a file or directory.
>>> import os
>>> os.is_file("/note.txt")
true
>>> os.is_directory("/note.txt")
6. shutil
The shutil module provides high-level operations on files and directories.
shutil.copyfile
Copyfile copies a file src to a file dst.
>>> import shutil
>>> src = "/tmp/note.txt"
>>> dst = "/usr/tom/note.txt"
>>> shutil.copyfile(src, dst)
shutil.copy
Copy copies a file src to a new file or directory dst.
7. Pickle
Data serialization and deserialization is important in any programming language. Pickle lets you turn a Python data structure into a byte stream.
>>> import pickle
>>> owner_to_pets = { "fred": "bunny", "dawson": "dog" }
>>> pickle.dump(owner_to_pets, open( "save.p", "wb" ) )
To load a pickle file, we use load:
>>> owner_to_pets = pickle.load(open( "save.p", "rb"))
>>> owner_to_pets
{ "fred": "bunny", "dawson": "dog" }
The “wb” and “rb” mean write binary and read binary.
Thanks for reading!
Level Up Coding
Thanks for being a part of our community! More content in the Level Up Coding publication.
Follow: Twitter, LinkedIn, Newsletter
Level Up is transforming tech recruiting 👉 Join our talent collective
7 Must-Know Python Built-In Libraries was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Alex Powell
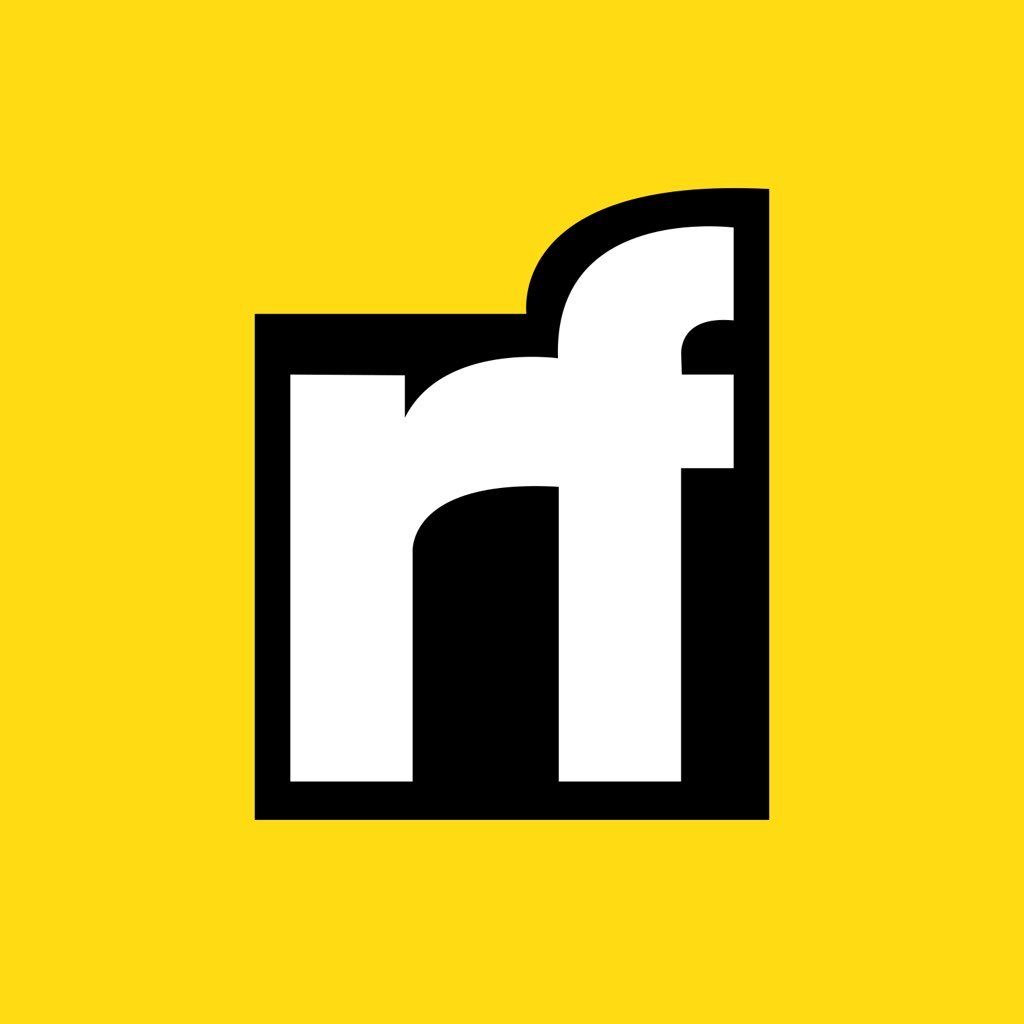
Alex Powell | Sciencx (2022-07-18T15:01:32+00:00) 7 Must-Know Python Built-In Libraries. Retrieved from https://www.scien.cx/2022/07/18/7-must-know-python-built-in-libraries/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.