This content originally appeared on Level Up Coding - Medium and was authored by George Argyrousis
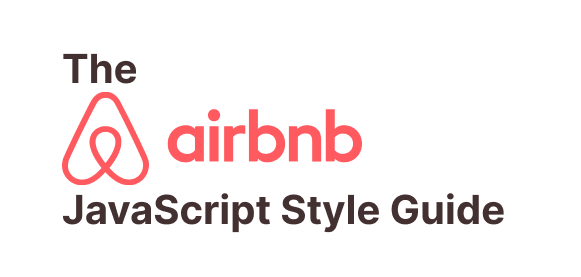
Airbnb has one of the most popular style guides for writing concise, clean, and readable JavaScript code. Their goal is to advocate stylistic rules for writing a consistent code base that is immediately understandable by your engineering organisation.
Each of the following points offers a small summary of the extracted rule along with a JavaScript snippet showcasing the formatted code in practise. Controversial issues such as tabs versus spaces or the usage of semicolon have been completely avoided.
Use const and let
By using const we ensure we do not re-assign variables and all of our declarations are block scoped. When a variable will need to be re-assigned, we opt for let instead of var.
// ❌ bad
var firstName = 'George';
// ✅ good
const firstName = 'George';
-----------------------------
// ❌ bad
var shirtSize = 'large';
shirtSize = 'medium';
// ✅ good
let shirtSize = 'large';
shirtSize = 'medium';
Use literal declarations
Literal declarations allow us to declare all properties of an object or an array in one location.
// ❌ bad
const person = new Object();
object.firstName = 'George';
// ✅ good
const person = {
firstName: 'George',
};
-----------------------------
// ❌ bad
const people = new Array();
// ✅ good
const people = [];
Furthermore, we have the added benefit of using object property assignments for variables having the same name as the matching key of the object.
// ❌ bad
const firstName = 'George';
const person = {
firstName: firstName
};
// ✅ good
const firstName = 'George';
const person = { firstName };
Destruct objects properties
We destruct object properties when we will need to repeatedly use them across the scope of our code.
// ❌ bad
const getFullName = (user) => {
const firstName = user.firstName;
const lastName = user.lastName;
// ...
return `${firstName} ${lastName}`;
};
// ✅ good
const getFullName = (user) => {
const { firstName, lastName } = user;
// ...
return `${firstName} ${lastName}`;
};
In addition, we can retrieve trimmed versions of our objects without having to delete or copy properties. Below we want all other user related properties excluding the shirtSize.
const user = { firstName: 'George', shirtSize: 'small' };
// ❌ bad - mutate user object
delete user.shirtSize;
// ✅ good
const { shirtSize, ...restOfUser } = user;
Use the spread (…) operator for shallow copying objects
The spread operator is the preferred way of shallow copying objects or properties. Unlike Object.assign it is concise without mutating* the original object.
// ❌❌ very bad
const person = { firstName: 'George' };
// Mutating the person object
const copy = Object.assign(person, { shirtSize: 'small' });
// ❌ bad
const person = { firstName: 'George' };
// (*) We are not mutating person here
const copy = Object.assign({}, person, { shirtSize: 'small' });
// ✅ good
const person = { firstName: 'George' };
const copy = {
shirtSize: 'small',
...person
};
Use template literals for string concatenation
Template strings provide a concise and readable syntax for string concatenation. They also provide multiline support without the need of a new line character \n.
const firstName = 'George';
// ❌ bad
const greeting = ['How are you, ', firstName, '?'];
// ❌ bad
const greeting = 'How are you, ' + firstName + '?';
// ✅ good
const greeting = `How are you, ${firstName}?`;
Use arrow functions for inline callbacks
When using anonymous functions as a parameter of another function, it is recommended to use the concise arrow function notation. However, if the logical operations are complex, it is suggested to extract the logic into its own function with a lexical name.
Always wrap the parameter(s) in parenthesis.
// ❌ bad
[1, 2, 3].map(function(entry) {
return entry ^ 2;
});
// ✅ good
[1, 2, 3].map((entry) => {
return entry ^ 2;
});
Use default parameters for function arguments
Argument defaults allows us to assign predefined values when the parameter is undefined. We no longer need to mutate passed arguments within the function scope.
It is highly recommended to declare optional arguments last.
// ❌ bad
function(firstName, options) {
options = options ?? {};
// ...
}
// ✅ good
function(firstName, options = {}) {
// ...
}
Final thoughts
There are far more than 8 points to consider if you want to adhere towards AirBnb’s JavaScript style guide. Of course, you can always augment these rules to fit the engineering culture and preset definitions of code styling within your organisation.
You can automate the process of checking code formatting using the eslint-config-airbnb npm package.
Thank you for reading! If you found this post useful, please share it with others. Some claps 👏🏻 below help a lot!
By clapping, you help others discover this content and motivate the writing of more articles about accessibility, design, React and JavaScript!
Level Up Coding
Thanks for being a part of our community! More content in the Level Up Coding publication.
Follow: Twitter, LinkedIn, Newsletter
Level Up is transforming tech recruiting 👉 Join our talent collective
8 Noteworthy Points from AirBnb’s JavaScript Style Guide was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by George Argyrousis
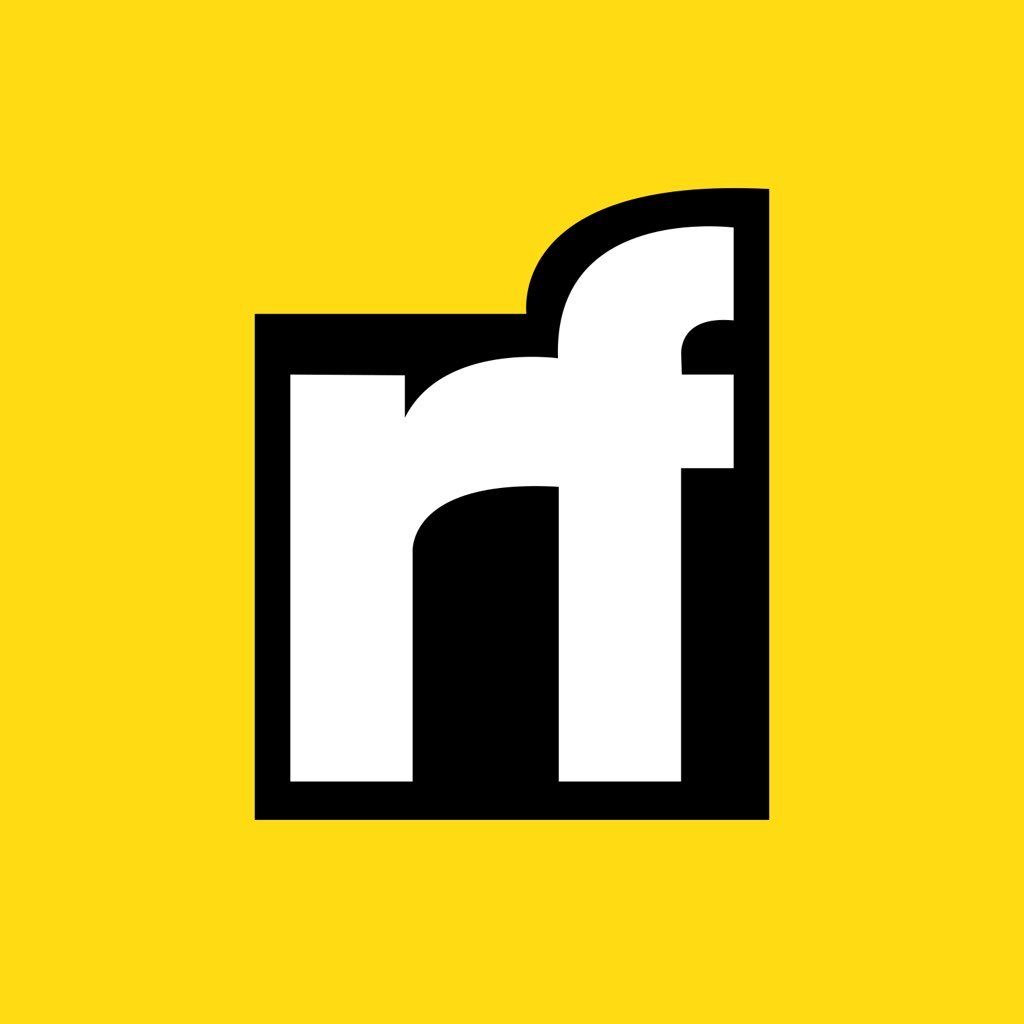
George Argyrousis | Sciencx (2022-07-18T15:01:36+00:00) 8 Noteworthy Points from AirBnb’s JavaScript Style Guide. Retrieved from https://www.scien.cx/2022/07/18/8-noteworthy-points-from-airbnbs-javascript-style-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.