This content originally appeared on Level Up Coding - Medium and was authored by StackZero
Hi reader, as you can see from my profile I usually write about cybersecurity stuff.
Even though it might not seem like it I like every aspect of programming.
In this article I’m excited to show how to write a tale generator.
To be honest, the final result is a text generator that will complete your incipit, whatever it is.
By the way “AI tale generator” was my primary use of the resulting script.
What are we going to learn?
The entire project it’s simply an excuse to hone our skills.
In detail, we are going to see how:
- Print a beautiful banner by using ASCII Art
- Manage CLI arguments
- Use a pre-trained model from HuggingFace
I guess you can’t wait to get your hands dirty, so let’s start!
Prerequisites
I will assume the presence of Python 3 on your system, so on be operative we need to install some libraries:
We can do everything with just one line on the terminal:
pip install tensorflow transformers pyfiglet click
It could take a while, get a quick break and maybe a coffee!
After the completion, we are ready to code!
Warming up and preparing the imports and global stuff!
The first lines we are going to write, as usual, are the imports.
However, they are not the only lines we are going to write.
In fact, the libraries we are going to use are a bit more verbose for our tastes!
You can see that all the warnings of tensorflow and transformers are turned off (2nd and 7th lines).
import os
os.environ["TF_CPP_MIN_LOG_LEVEL"] = "2"
import transformers
import click
from pyfiglet import Figlet
transformers.utils.logging.set_verbosity(transformers.logging.FATAL)
MAX_LENGTH = 250
MAX_LENGTH is just a global variable indicating the default value of the maximum length of the generated text.
Set up our wonderful banner
Every Command Line application needs a wonderful banner.
Let’s see how we can do it in a few lines by using pyfiglet and ASCII Art.
def print_banner(text, font='graffiti'):
figlet = Figlet(font=font)
print(figlet.renderText(text))
The function we just defined will come to our aid soon, but for now, let’s continue with our code!
GPT-2 How to use a pre-trained Transformer model
Although it is not a specific article on neural networks, I would like to show you how to use a pre-trained model from HuggingFace.
In this tutorial we are going to use a relatively old model, however the results will be satisfactory in many cases.
The model of interest is GPT-2 (Generative Pretrained Transformer) and it was the state of the art in 2019.
It is a Deep Neural Network which uses the attention mechanism to generate text.
By reading the documentation here it’s obvious how easily we can use a so complex model!
The high-level interface provided by transformers library allows us to focus just on the final result.
This is the code on how to create the model and then generate the text with a given incipit!
generator = transformers.pipeline('text-generation', model='gpt2')
out = generator(beginning,
max_length=max_length,
num_return_sequences=1)
The result is a list of dictionaries ( in this case we set num_return as 1).
Every dictionary contains a different text completion and the generated text is stored under the key generated_text .
The parameter max_length indicates the largest possible length of the output.
How to get arguments from the command line
In this step we want to pass the variables in two ways:
- The length should come from the terminal
- The beginning should come from user input after launching the application
To do that, we can rely on the click library which allows us to do that just with a few decorators.
These are the ones we are going to use:
@click.command()
@click.option("--beginning", prompt="Incipit", help="The incipit of your story")
@click.option("--length", default=MAX_LENGTH, help="The max length of the story", type=click.INT)
The first option gets the variable either from the terminal with the option “ — beginning” or from user input.
The second option’s value “ — length” is set as int and comes from the terminal.
Easy, isn’t it?
Now it’s time to create the main and an auxiliary method for generating the story.
Latest functions main and generate_story
Before putting everything together we need two more operations.
First we want a method that with a given generator will return the generated text.
And here are the two lines of code that will do the task
def get_story(generator, beginning, max_length):
out = generator(beginning, max_length=max_length, num_return_sequences=1)
return out[0]['generated_text']
After that, we need to define the main method. Is preferable to do that so that using the decorators will be easier and cleaner.
The main should just generate and print the story by getting parameters from the terminal, as we saw previously.
def main(beginning, length):
story = get_story(generator, beginning, length)
print(story)
Put all together
The final step is to sort everything out and call the functions in the right order, let’s see how:
if __name__=="__main__":
print_banner("Welcome on AI Story Generator!\n\n\n")
main()
Everything seems quite simple, but just for the laziest, let’s see the complete code.
import os
os.environ["TF_CPP_MIN_LOG_LEVEL"] = "2"
import transformers
import click
from pyfiglet import Figlet
transformers.utils.logging.set_verbosity(transformers.logging.FATAL)
generator = transformers.pipeline('text-generation', model='gpt2')
MAX_LENGTH = 250
def get_story(generator, beginning, max_length=MAX_LENGTH):
out = generator(beginning, max_length=max_length, num_return_sequences=1)
return out[0]['generated_text']
def print_banner(text, font='graffiti'):
figlet = Figlet(font=font)
print(figlet.renderText(text))
@click.command()
@click.option("--beginning", prompt="Incipit", help="The incipit of your story")
@click.option("--length", default=MAX_LENGTH, help="The max length of the story", type=click.INT)
def main(beginning, length):
story = get_story(generator, beginning, length)
print(story)
if __name__=="__main__":
print_banner("Welcome on AI Story Generator!\n\n\n")
main()
Et voilà, now we can try to run the script (in a file named “main.py”) with a max_length of 150 and see the result:
python main.py --length 150
And this is a my result!
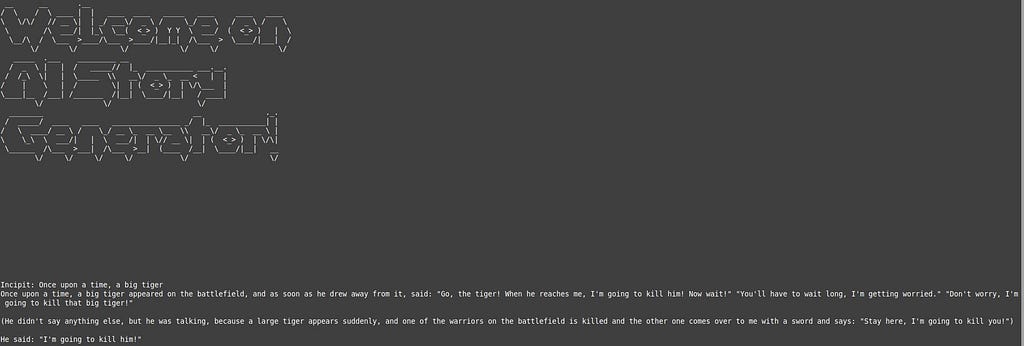
There are many possible improvements:
- Give the possibility to keep generating with a loop
- Use a more advanced model like GPT-3
- Fine-tuning the model in order to produce better tales
- Insert a loading animation while it’s generating the tale
etc…
I hope you like this article, and if you liked my work you can follow me on medium or maybe visit StackZero.
How To Build an AI tale generator for your kids was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by StackZero
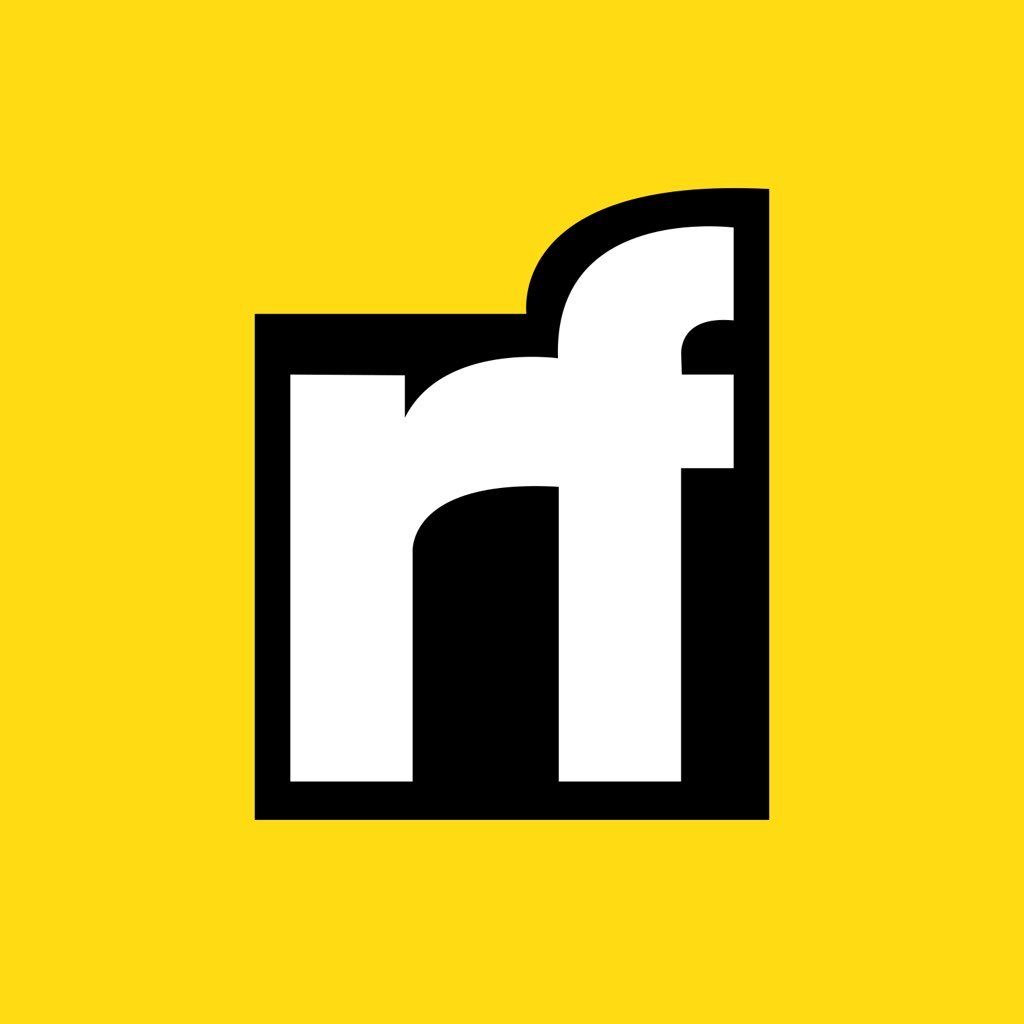
StackZero | Sciencx (2022-09-13T15:41:01+00:00) How To Build an AI tale generator for your kids. Retrieved from https://www.scien.cx/2022/09/13/how-to-build-an-ai-tale-generator-for-your-kids/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.