This content originally appeared on DEV Community 👩💻👨💻 and was authored by Mrigendra Prasad
So, now that you are familiar with iterators right? if not then
Iterators
Iterators are one of the fundamental concepts of programming. It is used to loop through all the elements of an array and perform the same operation on all of them or you can perform an operation number of times like printing a text 100 times with just a few lines of code with the help of iterators. In Python iterators are "for loop" and "while loop". Let's take an example of factorial using for loop.
def fact(n):
f = 1
if n == 0:
return 1
elif n == 1:
return 1
else:
for i in range(1, n+1):
f *= i
return f
print(fact(5))
>>> 120
But there is one more way to do that and that is recursion.
Recursion
Recursion is a technique by which a function calls itself but in its simpler form. It is an important concept and most of the time it is used in data structures. Let's take the example of factorial using recursion:
def fact_re(n):
if n == 1:
return 1
elif n == 0:
return 1
else:
return n * fact_re(n -1)
print(fact_re(5))
>>> 120
So, here you can see that fact_re() is calling itself but the argument passed is less than that of the initial function. Hence, every time the function calls itself the argument becomes smaller and smaller, and at some point, it will become 1 since we have already provided a condition that if the argument is equal to 1 it will return 1 and the function will stop calling itself after reaching that condition.
Here, if you compare both functions, the iterator one and the recursion one, you will find that the recursion one contains fewer lines of code and that's what programmers want.
Programmers are so lazy.
Recursion helps to develop logical thinking and improves problem-solving skills.
It is easier to write and debug code for data structures using recursion.
It reduces time complexity for larger codes.
Types of recursion
Yes, recursion also has different types. It is of three types basically:
- Linear recursion: If a recursive function is invoking itself at most one time during its execution then it is known as linear recursion. It can be a useful tool for processing a data sequence like lists or tuples in Python. For example:- The factorial function that we have seen above. Let's take another example to illustrate it better
def power(x, n):
if n == 0:
return 1
else:
return x * power(x, n - 1)
print(power(3, 4))
>>> 81
In the above example "x" is a number and "n" is the power. Here is the illustration:-
Note I will add more content to this article.
This content originally appeared on DEV Community 👩💻👨💻 and was authored by Mrigendra Prasad
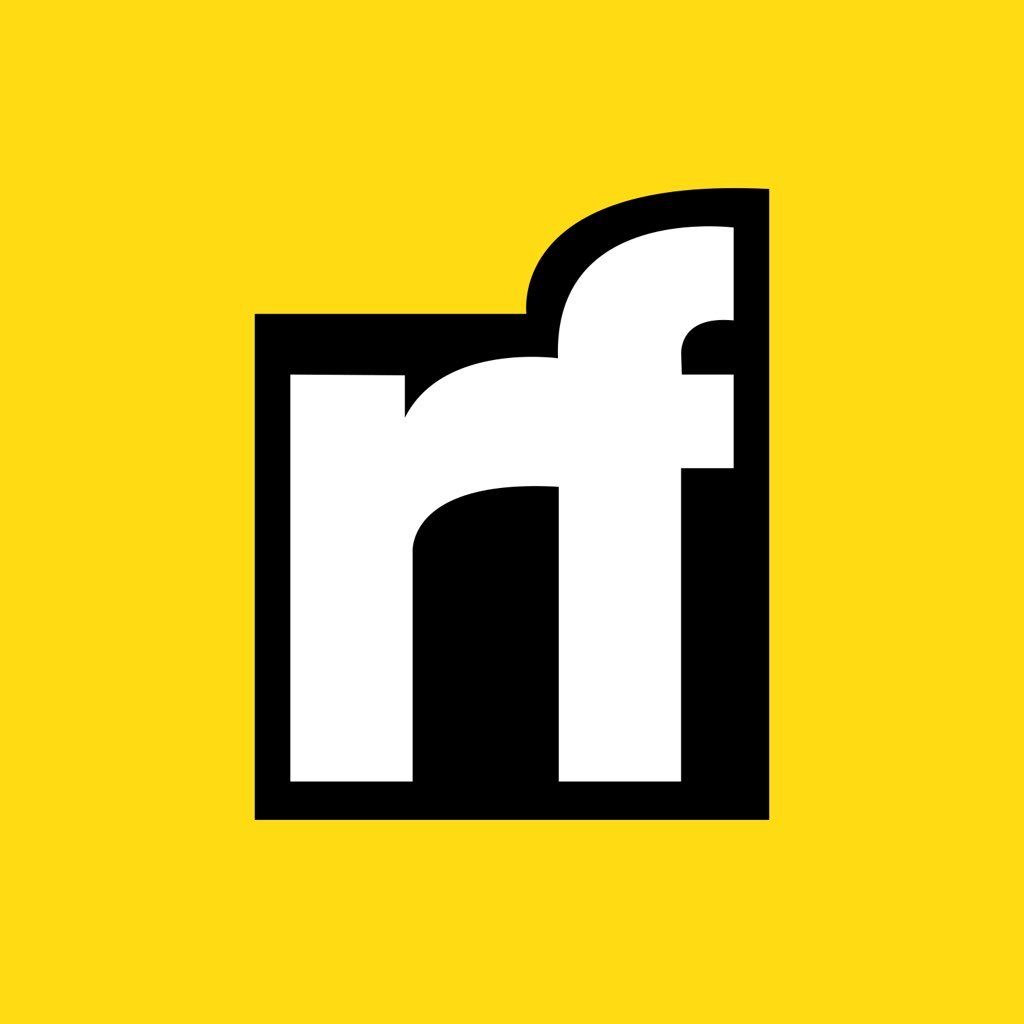
Mrigendra Prasad | Sciencx (2022-09-23T19:04:13+00:00) Recursion: One more way.. Retrieved from https://www.scien.cx/2022/09/23/recursion-one-more-way/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.