This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by simc dev CSM®
1. Shorthand for if with multiple OR(||) conditions
if (car === 'audi' || car === 'BMW' || car === 'Tesla') {
//code
}
In a traditional way, we used to write code in the above pattern. but instead of using multiple OR conditions we can simply use an array and includes. Check out the below example.
if (['audi', 'BMW', 'Tesla', 'grapes'].includes(car)) {
//code
}
2. Shorthand for if with multiple And(&&) conditions
if(obj && obj.tele && obj.tele.stdcode) {
console.log(obj.tele .stdcode)
}
Use optional chaining (?.) to replace this snippet.
console.log(obj?.tele?.stdcode);
3. Shorthand for checking null, undefined, and empty values of variable
if (name !== null || name !== undefined || name !== '') {
let second = name;
}
The simple way to do it is...
const second = name || '';
4. Shorthand for switch case to select from multiple options
switch (number) {
case 1:
return 'Case one';
case 2:
return 'Case two';
default:
return;
}
Use a map/ object
const data = {
1: 'Case one',
2: 'Case two'
};
//Access it using
data[num]
5. Shorthand for functions for single line function
function example(value) {
return 2 * value;
}
Use the arrow function
const example = (value) => 2 * value
6. Shorthand for conditionally calling functions
function height() {
console.log('height');
}
function width() {
console.log('width');
}
if(type === 'heigth') {
height();
} else {
width();
}
Simple way
(type === 'heigth' ? height : width)()
7. Shorthand for To set the default to a variable using if
if(amount === null) {
amount = 0;
}
if(value === undefined) {
value = 0;
}
console.log(amount); //0
console.log(value); //0
Just Write
console.log(amount || 0); //0
console.log(value || 0); //0
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by simc dev CSM®
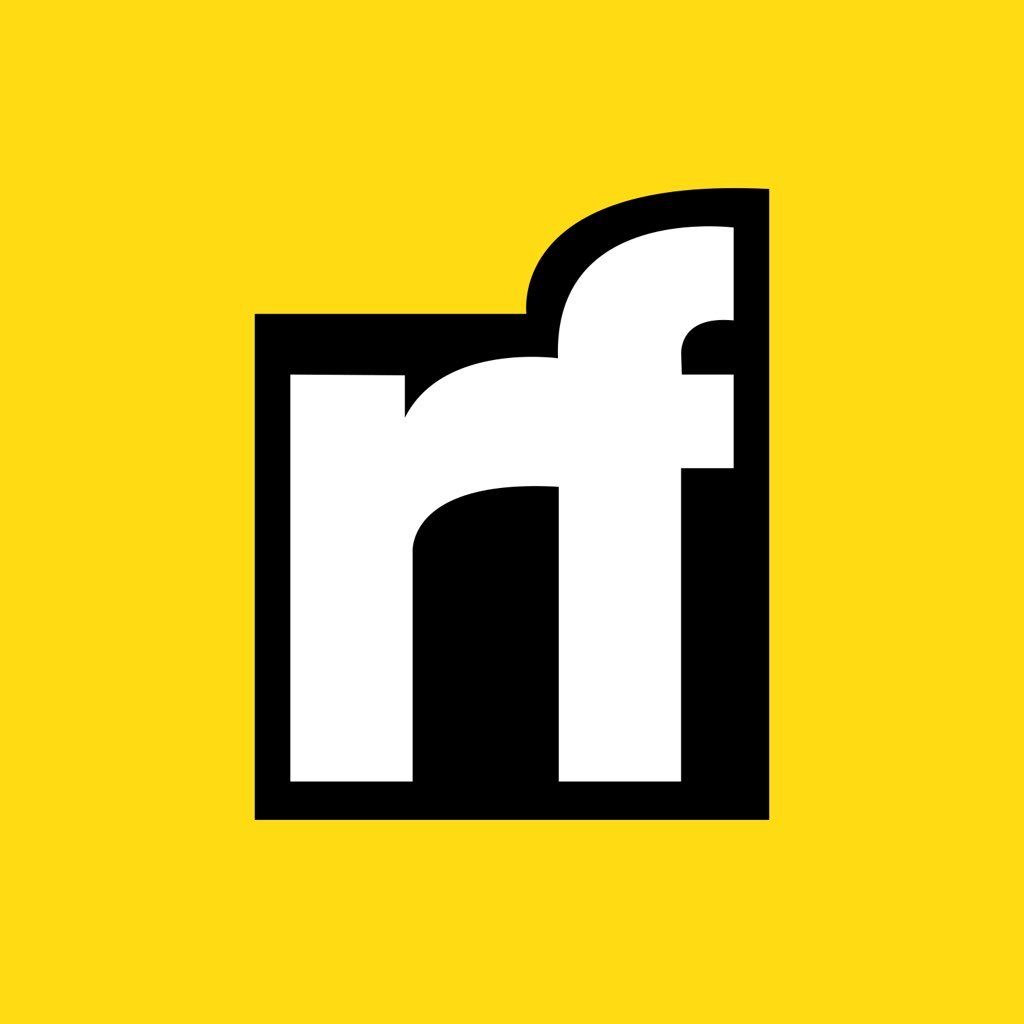
simc dev CSM® | Sciencx (2022-10-05T12:51:29+00:00) Change your old methods for writing a JavaScript Code – Shorthand’s for JavaScript Code. Retrieved from https://www.scien.cx/2022/10/05/change-your-old-methods-for-writing-a-javascript-code-shorthands-for-javascript-code/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.