This content originally appeared on Bits and Pieces - Medium and was authored by Tony
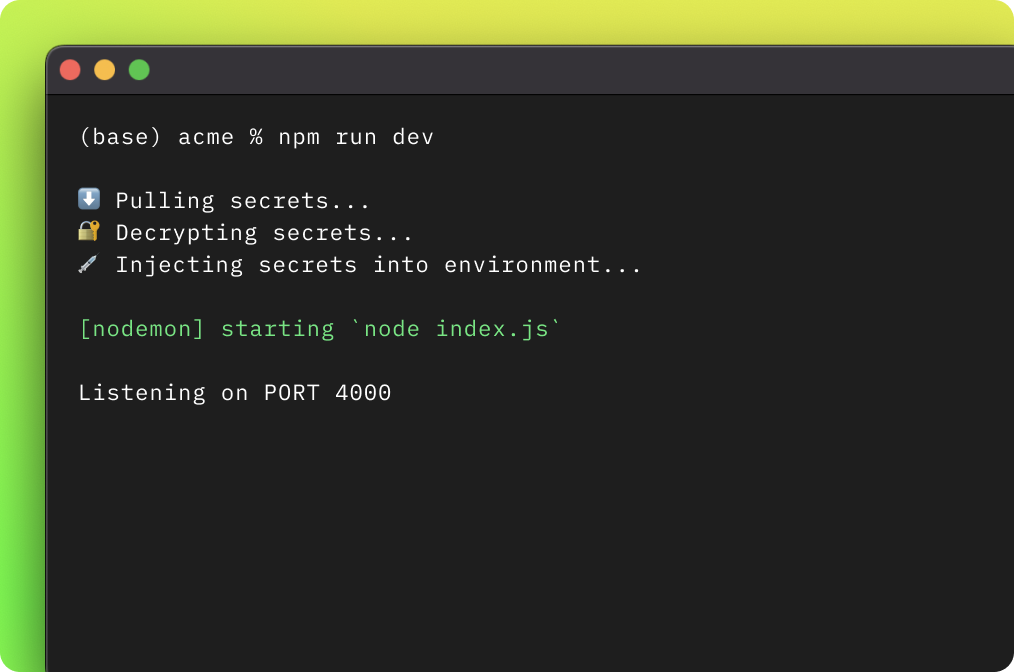
I ran into a post by Suhail recently about engineers at Twitter building in production environments. It captured a lot of attention and inspired me to write this blog about the importance of using multiple environments and how to do so with Node.js.
For context, software development teams use different (deployment) environments with unique properties/configurations for their apps and programs. They are useful for enabling apps to access variations of resources like internal development/production databases and external services like Stripe or Twilio. The most common environments are:
- Development: The environment in which a developer should operate in whilst writing code.
- Testing: The environment where testing teams can check code quality via automated and non-automated techniques.
- Staging: The environment that simulates the production environment as closely as possible.
- Production: The “live” environment that users interact with.
Using multiple environments ensures that ongoing software developments are well battle-tested through various stages before being deployed to production for users to interact with.
Unless you’re working alone on a small side-project that’s not going to be used by many people, then I’d recommend using at least two environments being development and production to mitigate ongoing development work from corrupting user experiences in production.
Left unchecked, bad things can also happen to developers too. Imagine developing Stripe or Twilio functionality in a production environment; you’d run the risk of losing money developing out the feature. Obvious tip: Don’t do it. Test credentials exist for a reason and should be grouped into the set of development environment variables.
That said, for those of you who are developing in production, please for your sake, take a few minutes out of your day to set up a development database and use development tokens for the external resources you’re using.
In Node.js, working with multiple environments is pretty straightforward. Developers maintain multiple .env files containing environment variables for each respective environment that can be loaded into their programs using a package like dotenv with the following code:
require('dotenv').config()
Given multiple .env files like .env.local or .env.production, it’s possible to specify a desired file path to load. Here’s how you might achieve that with dotenv:
dotenv.config({ path: '.env.local' });
dotenv.config();
Inside a .env file, you’d typically find environment variable key-pairs with NODE_ENV specifying the intended environment as follows:
NODE_ENV=development
SOME_CONFIG_X=XXX
SOME_SERVICE_API_KEY_X=XXX
Now here’s how you’d go about accessing the environment variables in code:
// check if operating in the development environment
if (process.env.NODE_ENV === "development") {
// execute some code
some_service({
apiKey: process.env.SOME_SERVICE_API_KEY
})
}
Unfortunately, it’s common to see developers in small teams group development and production API keys into one .env file. This, however, makes it difficult for teams to manage developer access to different environments and often produces code with ternary operators everywhere.
Instead of this:
// suboptimal
some_service({
apiKey: process.env.NODE_ENV === "production"
? process.env.SOME_SERVICE_API_KEY_PROD
: process.env.SOME_SERVICE_API_KEY_DEV
)}
Do this:
// more optimal
some_service({
apiKey: process.env.SOME_SERVICE_API_KEY
})
Ideally, you’d like to maintain separate .env files, so you can directly substitute environment variables in without needing to use ternary operators everywhere as in the latter code block above.
To summarize: Create multiple .env files like .env.local and .env.production for each environment, modify the file path in your dotenv configuration, and start directly substituting variables into your program. That’s all it takes to start using multiple environments in Node.js!
As icing on top, I wanted to talk about an alternative to .env files, for feeding environment variables into your local process. This is relevant because .env files can be mishandled and troublesome to keep in sync, especially if you’re dealing with multiple environments. You can read more about that discussion in my blog here.
If possible, you should use a secret manager to inject environment variables into your local processes during development. Such a handy solution can store your environment variables and pull them back into your local process automatically.
A secret manager can give your dev team a centralized view of all your environment variables across various environments, impose relevant access management controls so certain users may not have access to certain environments or variables, and inject the right set of variables into your local process.
That said, many solutions like Vault can be cumbersome and overkill to set up depending on the size of your projects.
Seeing this gap, we’ve made a simple solution called Infisical that’s compatible with most leading JS tools and frameworks including Express, Fastify, Koa (+ nodemon) as well as Create-React-App, Next.js, NestJS, and Gatsby; you can check out a demo for it here.
It’s an end-to-end encrypted platform that dev teams can use to upload their environment variables and enable their devs to pull and inject back values into their local processes all by adding 1 line of code to their dev scripts. We’ve even released a complete step-by-step guide for it here!
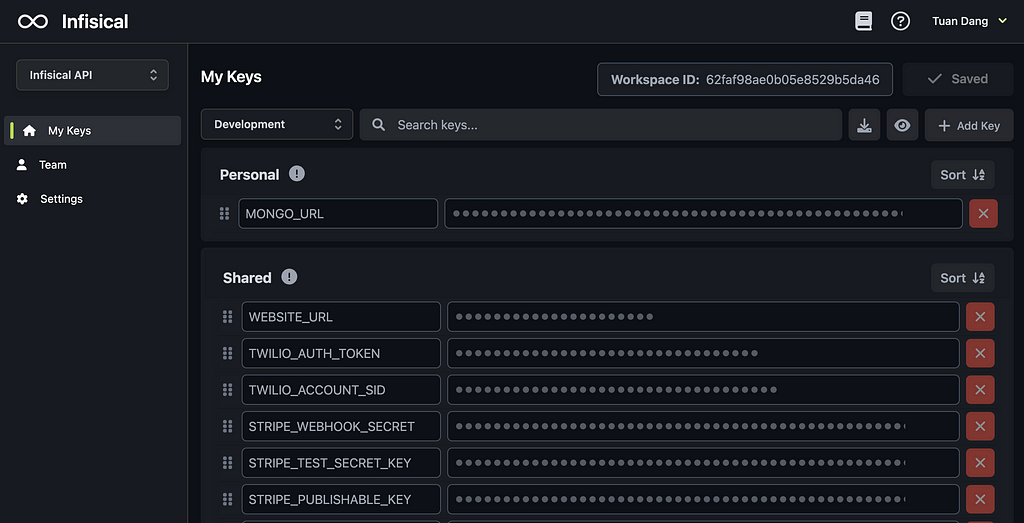
Thank you for reading and I hope you enjoyed this article on why to use multiple environments and how to implement them in Node.js.
Now go out there and build cool stuff safely 🚀
Build apps with reusable components like Lego
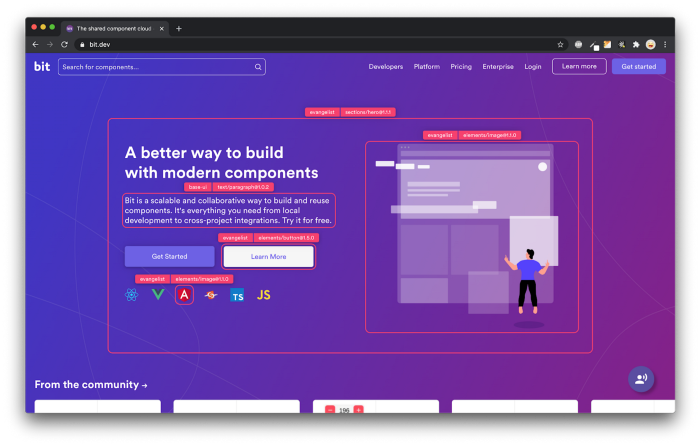
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- The Bit Blog
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Why, When, and How to Use Multiple Environments for Node.js was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Tony
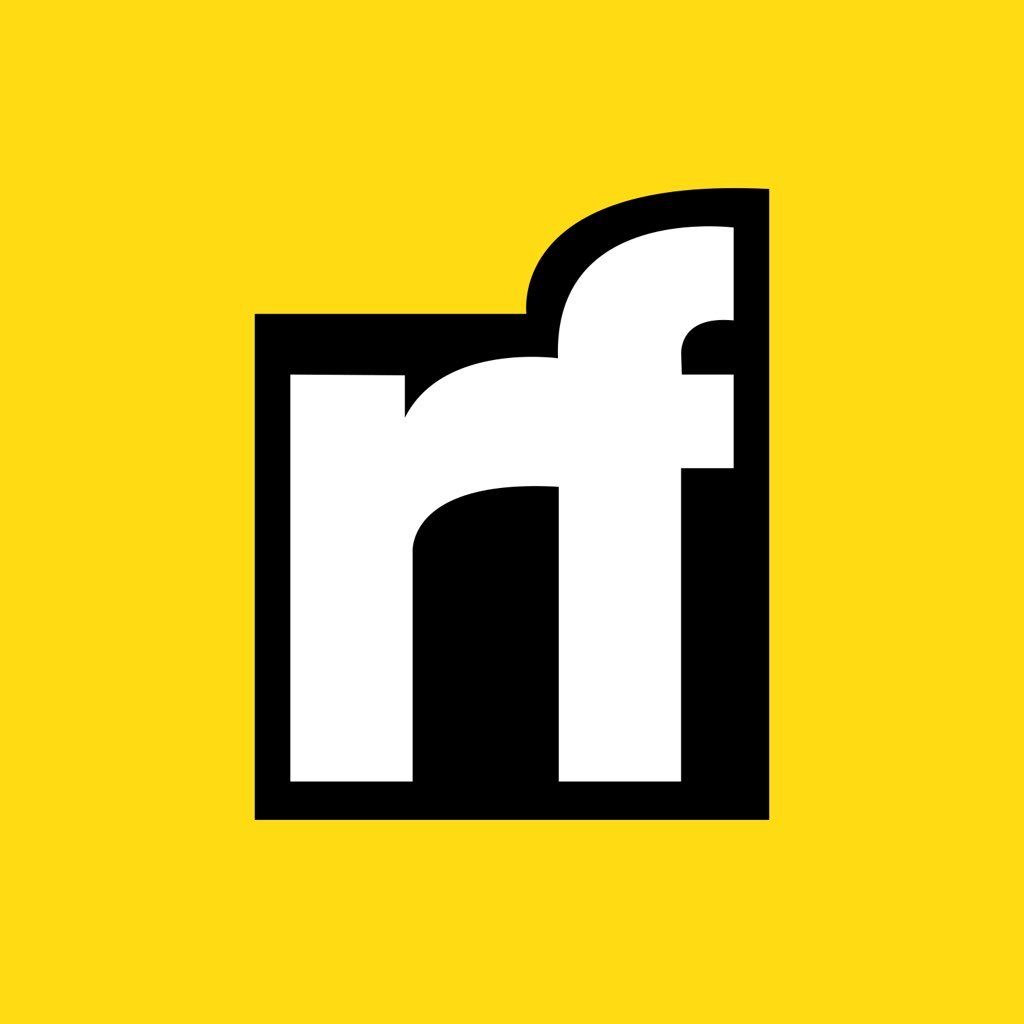
Tony | Sciencx (2022-10-05T11:01:51+00:00) Why, When, and How to Use Multiple Environments for Node.js. Retrieved from https://www.scien.cx/2022/10/05/why-when-and-how-to-use-multiple-environments-for-node-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.