This content originally appeared on Level Up Coding - Medium and was authored by Kiran Suvarna
Two best friends with a clear separation

I want to thank you for stopping by and reading this blog instead of scrolling through Instagram and swiping right. The real reason I’m writing this blog is to brush up on my knowledge of concurrency and parallelism.
I recently discovered a learning method by reading about Richard Feynman. The method states,
Write the idea down as simply as you can and consider how you would explain it to someone who has little or no prior knowledge of the idea.
So I’m giving it my all here. Before we get into the definitions, consider some practical examples.
Concurrency is having only one hand and doing something with it, stopping, starting something else, stopping, and returning to the first thing. You’re splitting one hand between several activities.
Parallelism is when you use both of your hands to brush your teeth with one hand while styling your hair with the other.
Concurrency is two lines of customers ordering from a single cashier (lines take turns ordering); Parallelism is two lines of customers ordering from two cashiers (each line gets its own cashier).
Definitions:
By Rob Pike, famous programmer, and author
Concurrency is dealing with multiple things at the same time while Parallelism is doing multiple things at the same time.
In the book The Pragmatic Programmer
Concurrency is when the execution of two or more pieces of code act as if they run at the same time. Parallelism is when they do run at the same time.
On the surface, both seem similar and also I’ve seen some developers use these words interchangeably but both have clear separations.
Let’s dive a little deeper with some diagrams
Concurrency:
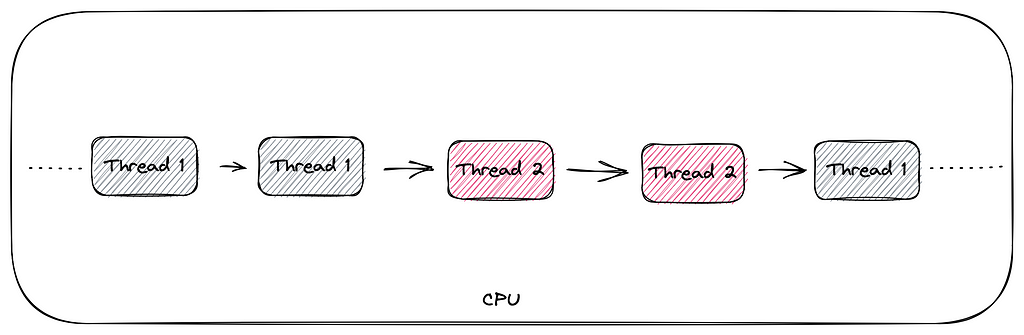
Under modern terminology, the CPU and processor mean more or less the same thing. So consider the above scenario in a single CPU / processor. A thread of execution is the smallest sequence of programmed instructions that can be managed independently.
A computer with only one CPU cannot perform more than one task at a time. When given multiple tasks, such as making requests to the Google, Twitter, and Medium websites, it simply switches between them. This switching is so quick and seamless that it appears to the user to be multitasking.
Parallel execution:
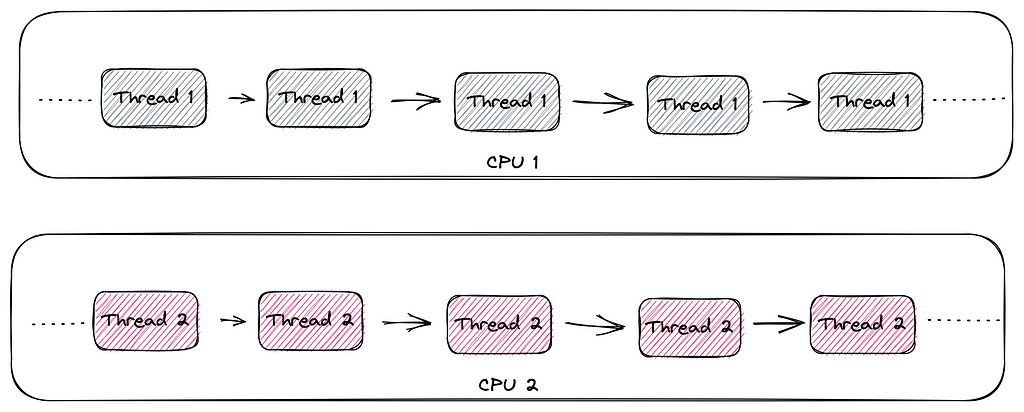
We looked at a single CPU / processor in concurrency, but most computers now have more than one processor, and most processors have more than one core. Parallel execution occurs when a computer has more than one CPU or CPU core and works on multiple tasks at the same time.
Let’s go a little further with some code samples in Go:
Go was designed to take advantage of multiple cores natively, and it knows how to do so effectively. To support concurrency, Golang provides goroutines.
A goroutine is a lightweight thread managed by the Go runtime.
A goroutine requires only 2kB of stack space to initialize. A standard thread, on the other hand, can take up to 1MB of memory, so creating a thousand goroutines requires far fewer resources than creating a thousand threads.
Concurrency

As seen in the above example, a method in Go may be made run concurrently by simply prepending the method call with the “go” keyword. The pingWebsite method is called from main method concurrently and prints the website’s status to the console. Run and debug it once on your PC to better understand it.
Parallelism

The above example is almost similar to the previous example but a major change is we have introduced a lock mechanism to mimic the parallel execution.
The line
runtime.GOMAXPROCS(runtime.NumCPU())
sets the number of CPUs that can be used, if not set it uses all available CPUs
GOMAXPROCS sets the maximum number of CPUs that can be executed simultaneously and returns the previous setting. It defaults to the value of runtime.NumCPU.
The line
runtime.LockOSThread()
prevents goroutines from reusing existing threads by locking the thread and unlocking it after the task is completed in the following line.
runtime.LockOSThread().
The pingWebsite method is called from main method parallelly and prints the website’s status and the number of threads before and after the execution to the console. The number of threads after the code execution is greater than before the execution. And remember Go can automatically create the required number of threads dynamically.
To better understand it, please run and debug both examples on your computer.
That is how we can implement parallel execution in Go. By default, the Go scheduler reuses threads that are not blocked.
You can find the code samples here.
Final thoughts
Concurrency and parallelism have a very distinct meanings in the realm of computers and engineering and I hope you are crystal clear on what each one exactly means. So if you ask which one is better, there is no single answer, you have to figure out which one is better for your use case. What do you think? Is concurrency better than parallelism? or is parallelism better than concurrency? Are apples better than oranges?
Thank you so much if you’ve made it this far. You are now free to scroll through random cat videos on the internet. If you enjoyed this blog, please give it a clap, leave a comment, and follow for more.
References:
- https://jenkov.com/tutorials/java-concurrency/concurrency-vs-parallelism.html
- https://pkg.go.dev/runtime#GOMAXPROCS
- https://levelup.gitconnected.com/how-to-implement-concurrency-and-parallelism-in-go-83c9c453dd2
- https://www.youtube.com/watch?v=oV9rvDllKEg
- https://stackoverflow.com/questions/1050222/what-is-the-difference-between-concurrency-and-parallelism
A Short Intro on Concurrency and Parallelism was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Kiran Suvarna
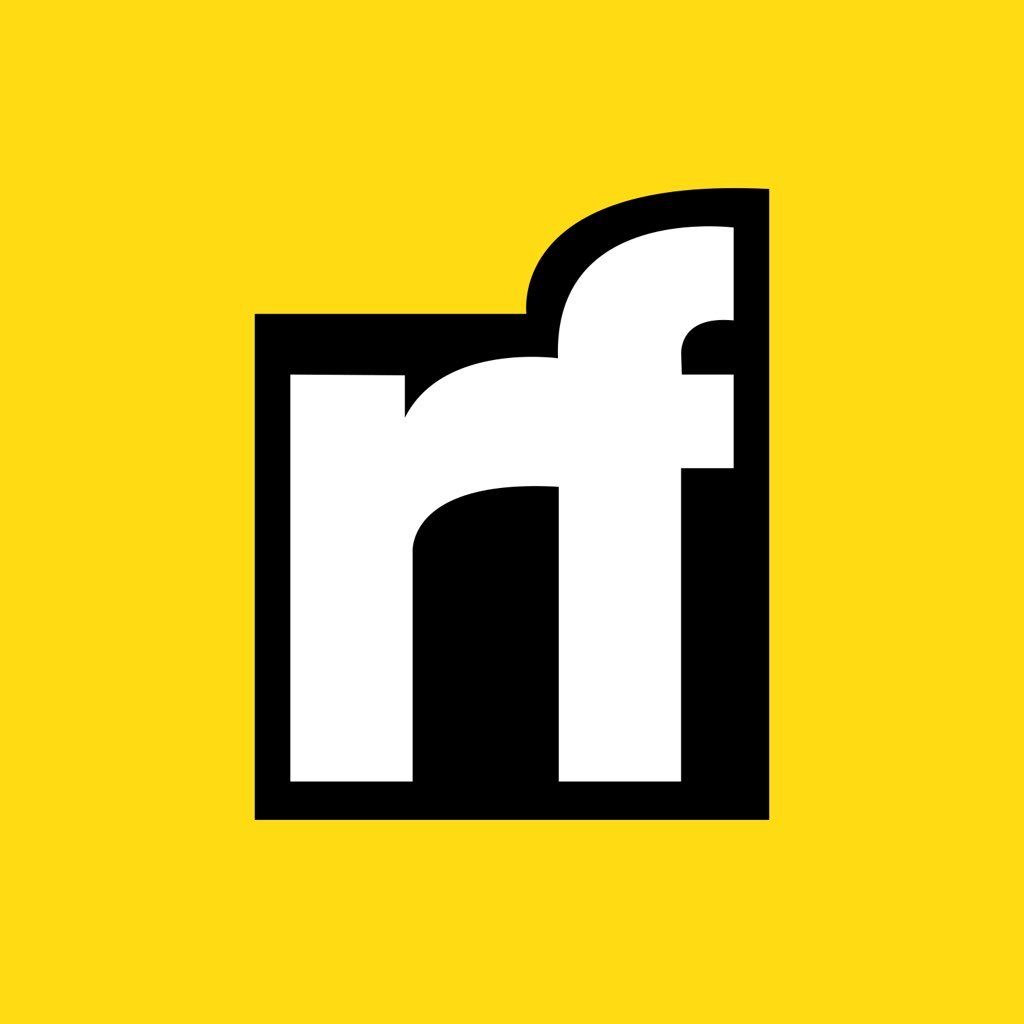
Kiran Suvarna | Sciencx (2022-10-06T14:50:19+00:00) A Short Intro on Concurrency and Parallelism. Retrieved from https://www.scien.cx/2022/10/06/a-short-intro-on-concurrency-and-parallelism/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.