This content originally appeared on Level Up Coding - Medium and was authored by Maxwell
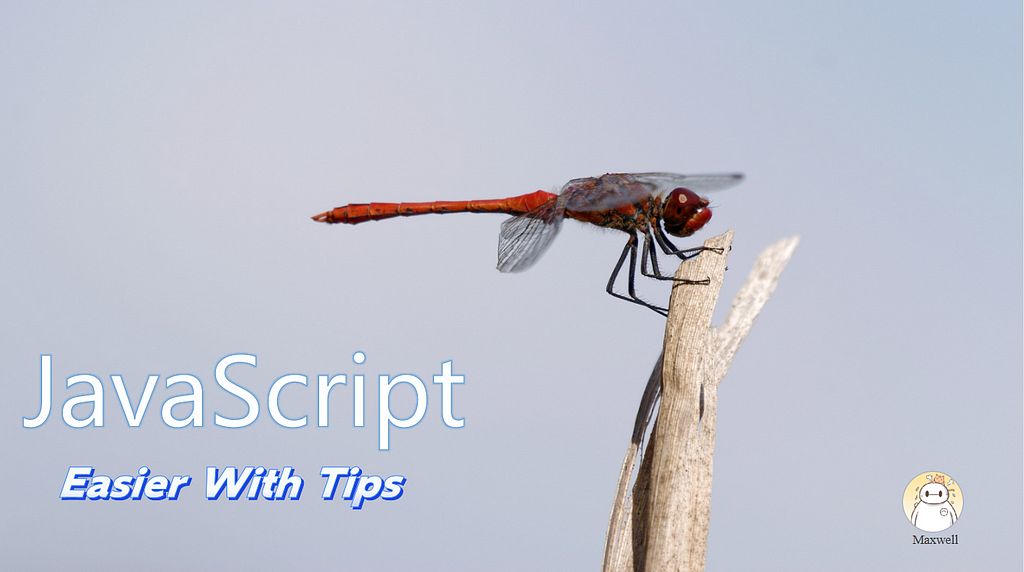
Bookmark these 21 super useful JS tips and use them in your development to make your job easier!
1. If Statement With Multiple Conditions
Put multiple values in an array and then call the includes method of the array.
2. Simplify if true…else with conditional expressions
3. False value (undefined, null, 0, false, NaN, empty string) check
When we create a new variable, sometimes we want to check if the referenced variable is not a falsy value such as null or undefined or an empty string. JavaScript does have a nice shortcut for this kind of checking — the logical OR operator (||)
|| will return the right operand if the left operand is false only if the left side is: empty or NaN or null or undefined or false, The logical or operator (||) will return with the right side the value of.
4. null/undefined checking and default assignment
5. Get the last item in the list
In other languages this functionality is made into a method or function that can be called on an array, but in JavaScript you have to do some work yourself.
6. Return after comparison
7. Use the optional chaining operator -?.
? Also known as the chain judgment operator. It allows developers to read property values deeply nested in object chains without having to verify every reference. When the reference is empty, the expression stops evaluating and returns undefined.
8. && operator for multiple conditions
To call a function only if the variable is true, use the && operator.
This is useful for short-circuiting with (&&) when you want to conditionally render a component in React. E.g:
<div> {this.state.isLoading && <Loading />} </div>
9. switch simplified
We can store conditions in key-value objects and call them based on the conditions.
10. Default parameter value
11. Conditional lookup simplified
If we want to call different methods based on different types, we can use multiple else if statements or switches, but is there a better simplification trick than this? In fact, it is the same as the previous switch simplification.
12. Object property assignment
13. Destructuring Assignment
14. Template String
If you’re tired of using + to concatenate multiple variables into a single string, this simplification trick will save you headaches.
15. Spanning String
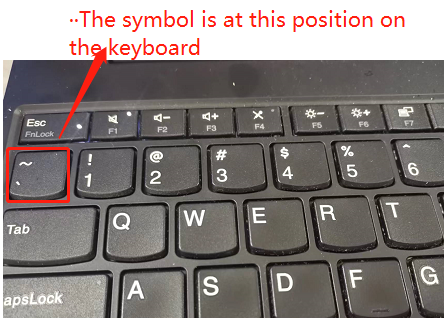
16. Bitwise simplification of indexOf
When looking up a certain value in an array, we can use the indexOf() method. But there is a better way, let’s look at this example.
17. Convert string to number
There are built-in methods such as parseInt and parseFloat that can be used to convert strings to numbers. We can also do this simply by supplying a unary operator (+) in front of the string.
18. Execute Promises Sequentially
What if you have a bunch of async or normal functions all returning promises that require you to execute them one by one?
Wait for All Promises To Complete
The Promise.allSettled() method accepts a set of Promise instances as a parameter, wrapped into a new Promise instance. The wrapper instance will not end until all of these parameter instances have returned results, either fulfilled or rejected.
Sometimes, we don’t care about the results of asynchronous requests, we only care about whether all requests have ended. At this time, the Promise.allSettled() method is very useful.
19. Swap the positions of array elements
20. Random number generator with range
Sometimes you need to generate random numbers, but you want the numbers to be within a certain range, then you can use this tool.
Generate random colors
Ok, that’s all, the 20 JavaScript Tips compiled above hope to be of practical help to your development work, thank you for reading.
- JavaScript Try-Catch Error and Exception Handling Guide
- 12 Lines of JavaScript To Make You Look Like a Pro
- Summarize the Common Loop Traversal Methods in JavaScript — How Many Have You Used?
20 Super Useful JS Tips To Make Your Job Easier was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Maxwell
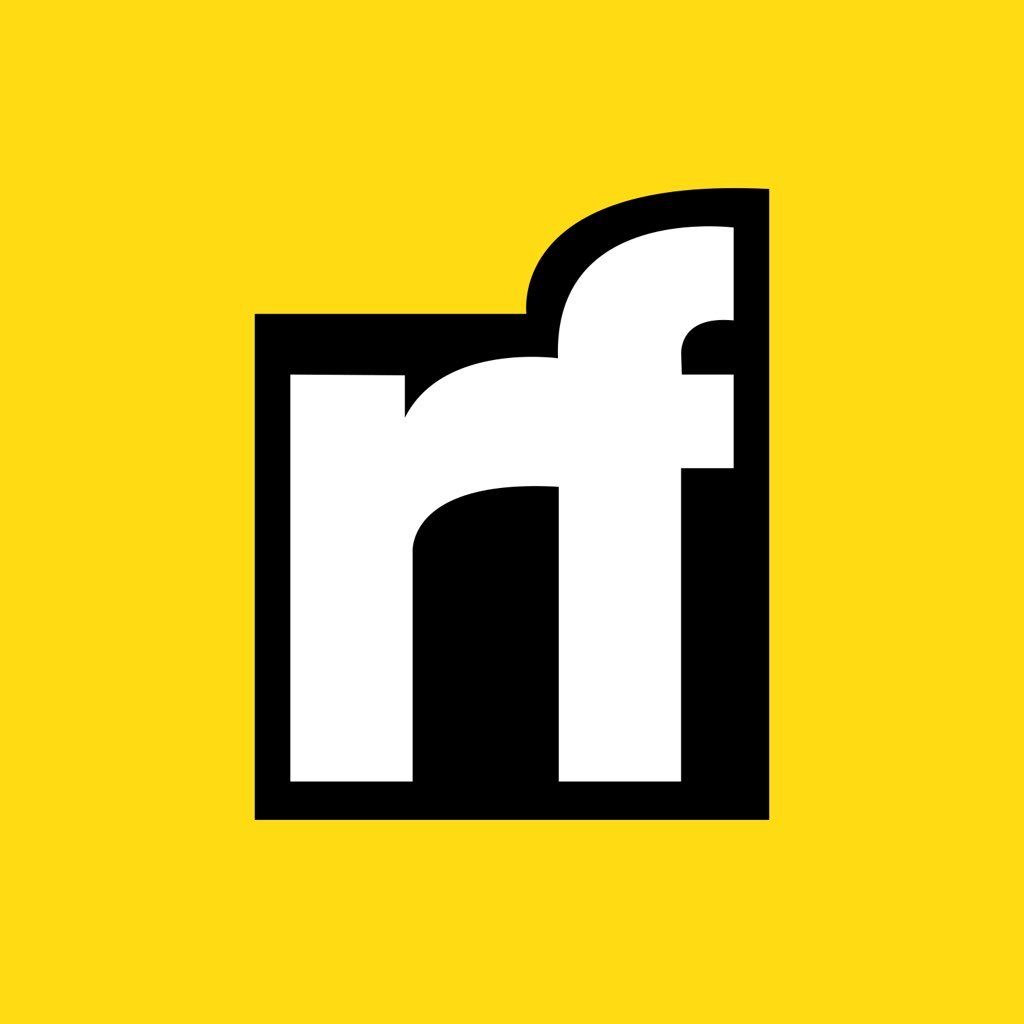
Maxwell | Sciencx (2022-10-20T16:12:37+00:00) 20 Super Useful JS Tips To Make Your Job Easier. Retrieved from https://www.scien.cx/2022/10/20/20-super-useful-js-tips-to-make-your-job-easier/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.