This content originally appeared on Bits and Pieces - Medium and was authored by Ravidu Perera
Create simple Desktop notifications for React application
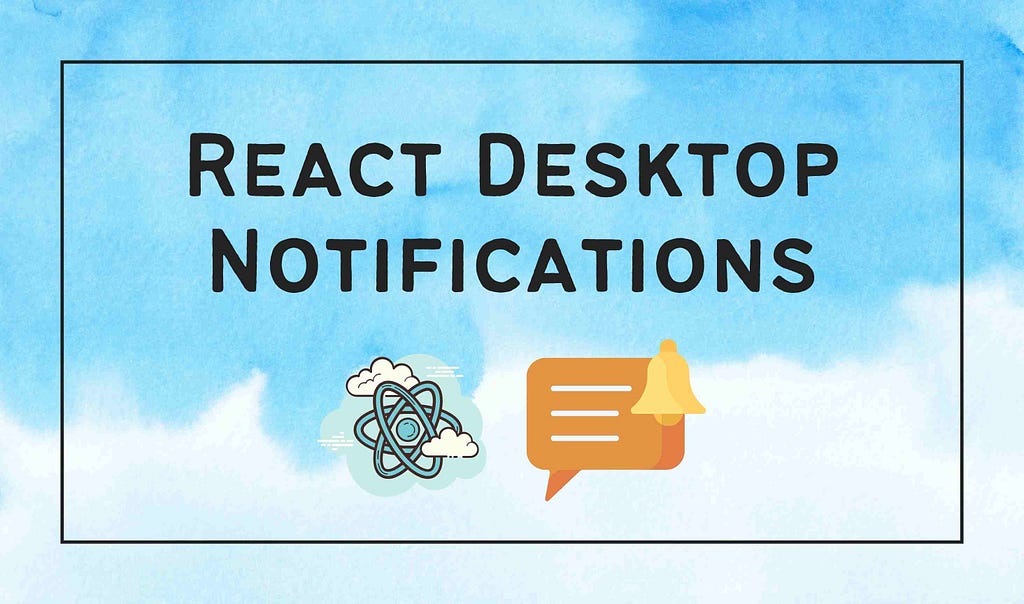
Web developers often use push notifications in web applications to display alerts. However, most web developers are unaware of desktop notifications and how we can use them in web applications.
Desktop notifications are also an important type of notification we can use in web applications. They allow developers to display alerts even when the user’s computer has been idle for too long. Also, desktop notifications are hard to miss.
So, in this article, I will discuss how to implement desktop notifications using React and why they’re important.
What are Notifications?
Notifications are a way to communicate with your users. You can display notifications to users in various contexts and situations. For example, you can use notifications to inform the user about new information or updates on their application or when you need immediate user attention for something.
What are Desktop Notifications?
I’m sure you have seen desktop notifications from multiple applications. They appear on the corner of your desktop in situations like when you get a new email or chat message. They can be shown as a popup or banner notification and used to communicate with users differently.
JavaScript offers a Notification API with various properties, methods and events to implement desktop notifications. So, in this article, I will use JavaScript Notification API for the implementations.
How to Use Desktop Notifications with React
JavaScript Notification API comes with 2 methods to facilitate desktop notification creation.
- Notification.requestPermission() - This method will request permission to the user's browser.
- Notification.close() - This will close the notification instance programmatically.
First, you must request permission from the user to display notifications using the requestPermission() method. Then display the notification and finally close the notification instance using close() method.
But, since we are implementing desktop notifications with React, you must check for browser support first. For that, you can use the componentDidMount()method as shown below :
componentDidMount() {
if (!("Notification" in window)) {
console.log("Browser does not support desktop notification");
} else {
console.log("Notifications are supported");
}
}
The above method will check whether the browser can access the notification object from the API.
After that, you need to obtain consent from the user to display notifications using the requestPermission() method. For that, you can update the above code as below:
componentDidMount() {
if (!("Notification" in window)) {
console.log("Browser does not support desktop notification");
} else {
Notification.requestPermission();
}
}
When the page is loaded, it will prompt a pop-up with Allow and Block buttons to give consent to get the notifications. The below code shows a complete code example of a React component to send desktop notifications.
import React, { Component } from "react";
class DesktopNotification extends Component {
constructor() {
super();
this.showNotification = this.showNotification.bind(this);
}
componentDidMount() {
if (!("Notification" in window)) {
console.log("Browser does not support desktop notification");
} else {
Notification.requestPermission();
}
}
showNotification() {
new Notification('Hello World')
}
render() {
return (
<div>
<button onClick={this.showNotification}>Show notification</button></div>
);
}
}
export default DesktopNotification;
In this example, onClick event will call the this.showNotification() method. Then it will call the constructed method of the Notification API and display the message.
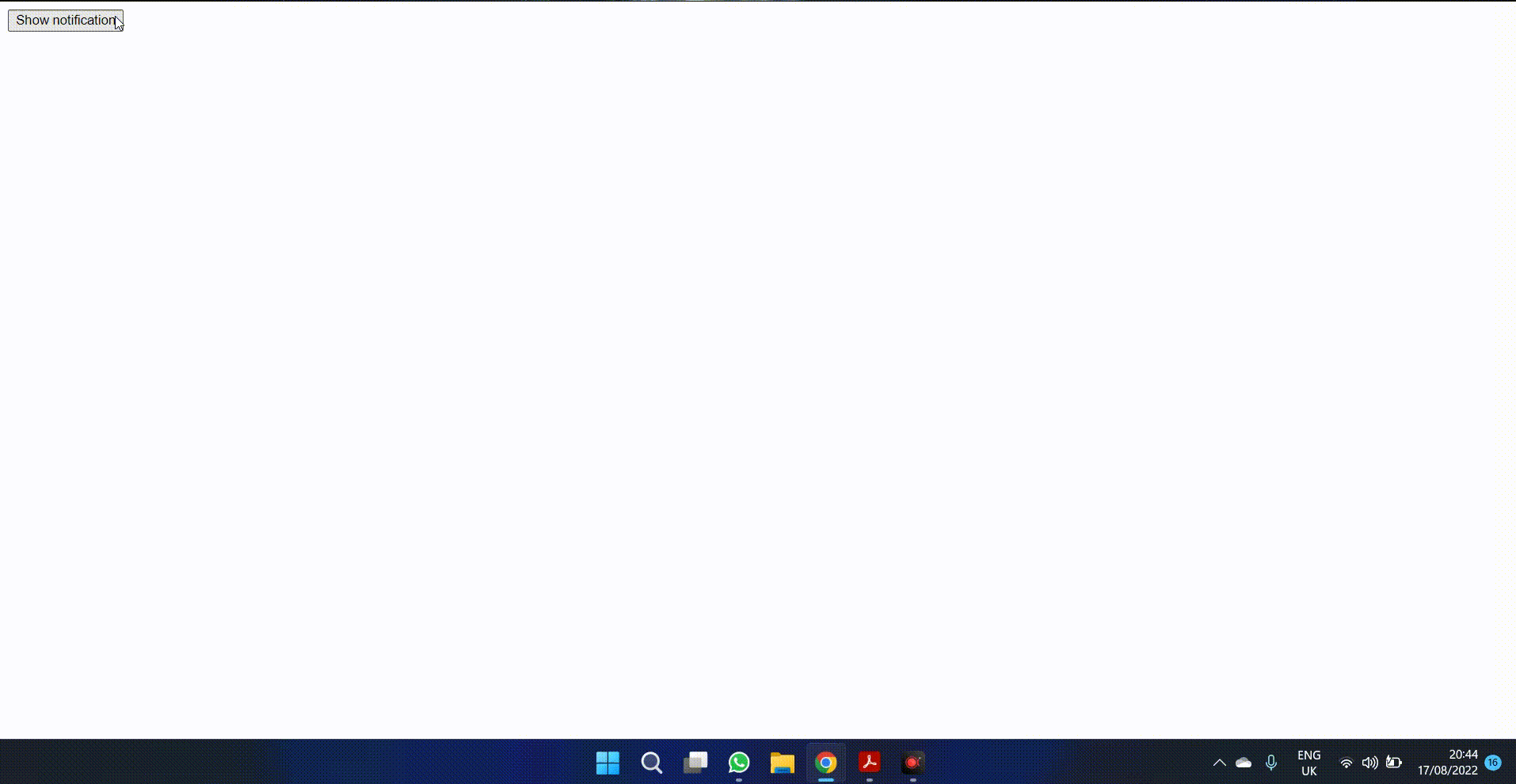
We can customize this showNotification() method and modify the notification body. For example, if you want to add an icon to the notification, you can create an object with the icon URL and pass it with the text to the Notification() method.
showNotification() {
var options = {
body: 'Notification Body',
icon: 'https://www.vkf-renzel.com/out/pictures/generated/product/1/356_356_75/r12044336-01/general-warning-sign-10836-1.jpg? auto=compress&cs=tinysrgb&dpr=1&w=500',
dir: 'ltr',
};
notification = new Notification('Hello World', options);
}
In the above example, options is an object which contains 3 properties:
- body - Contains the text message of the notification
- icon - Icon URL
- dir - Text direction (ltr = left to right)
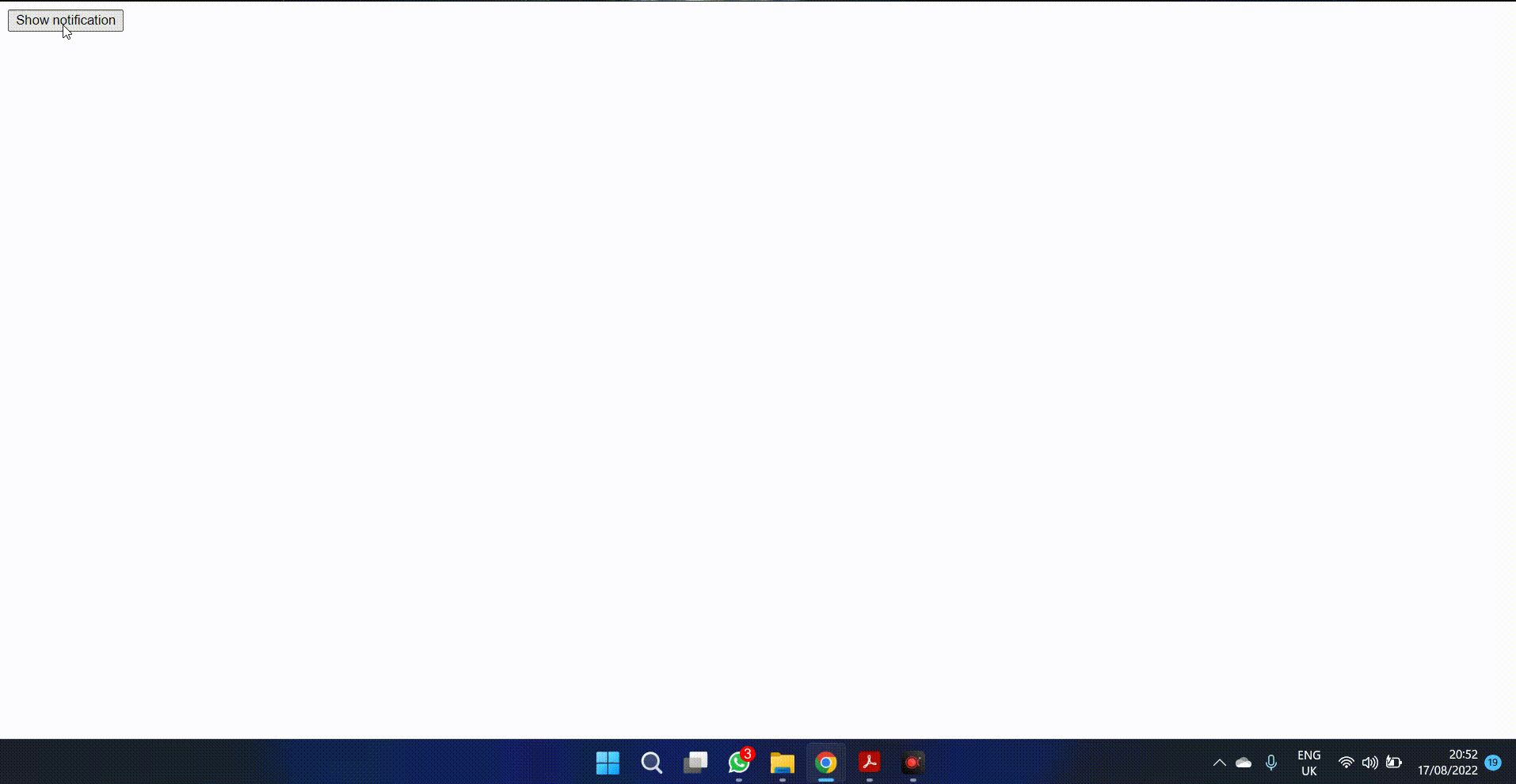
Finally, you need to close the notification after displaying it. For that, you can use the close() method provided by the Notification API.
closeNotification() {
notification.close();
}
You can either bind it to a button click similar to the below example or trigger it after a few seconds using a background worker.
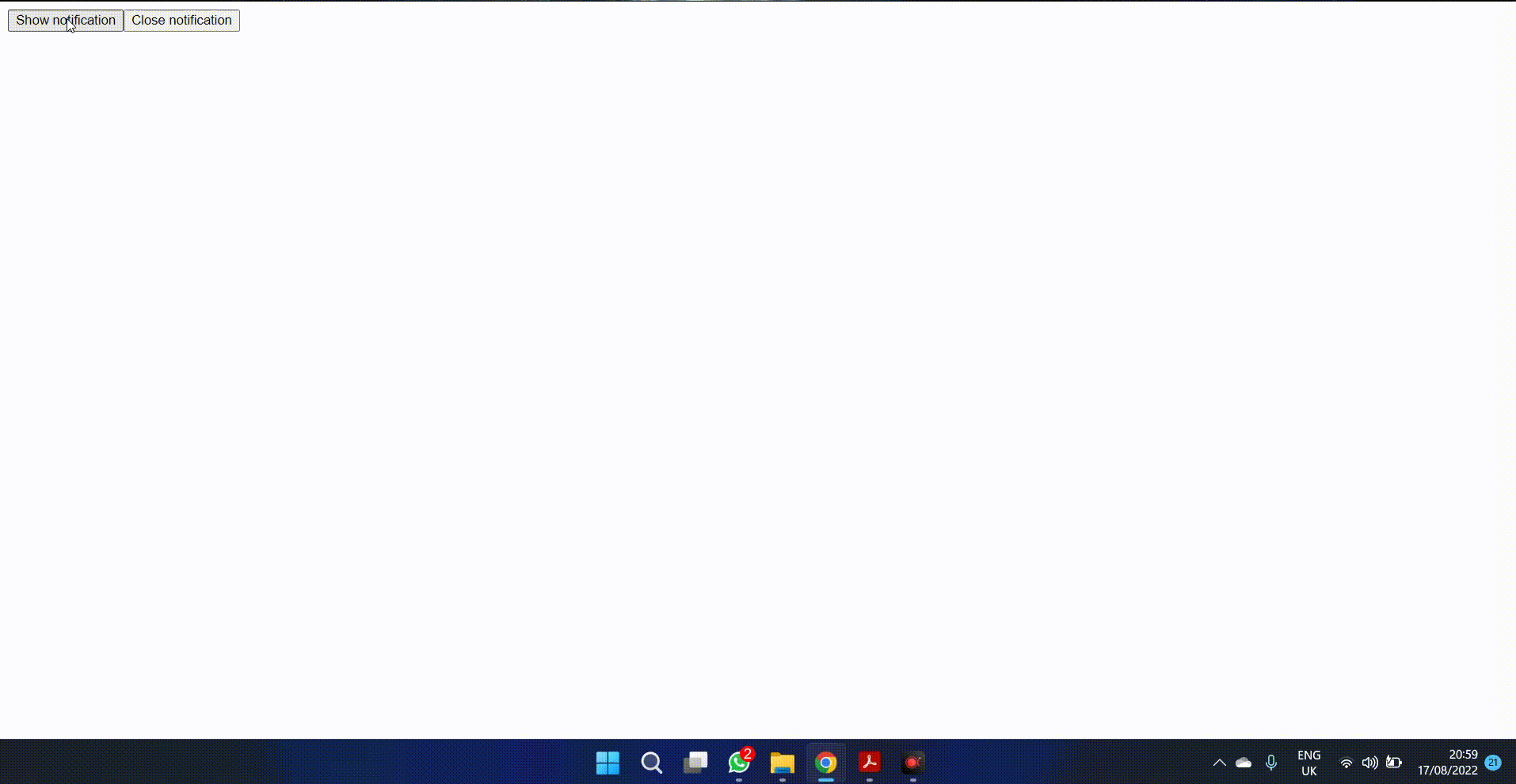
That’s it. You have successfully implemented desktop notifications with React using JavaScript Notification API.
Other Methods to Create Desktop Notifications
In addition to JavaScript Notification API, there are a few other ways to implement desktop notifications with React.
1. react-notifications library
The react-notifications library is great for creating new notifications and handling the events the user interacts with. You can configure it with various settings, including icon size and position on the screen (top left or top right).
Furthermore, it provides other options like content hiding based on device scaling factor and time-based notifications to improve battery life on users’ devices.
2. react-toast-notifications library
The react-toast-notifications is another library that can be used to implement desktop notifications. This library provides a toast notification to users with legible message content at the bottom of the screen or at a defined target. It automatically disappears after a brief time (time-out) with various animation effects. The control includes several built-in settings for adjusting visual components, animations, durations, and toast dismissal.
Clean reading content, optional graphics, icons, input fields, and buttons can all be included in a standard toast notification library. Users can load HTML information and alter it dynamically inside toast notifications using templates.
Users can quickly create standard React toast alerts using built-in classes like success, warning, information, and error.
react-toast-notifications are most suitable for:
- Less important messages where user actions are not important
- Confirmation messages.
- Information messages which do not need any follow-ups.
Conclusion
This article discussed implementing desktop notifications with React and JavaScript Notification API. Similar to push notifications, you can use desktop notifications to improve the user experience of your application.
I hope this guide helped you to understand how desktop notifications work in React applications. Thank you for reading.Build apps with reusable components like Lego
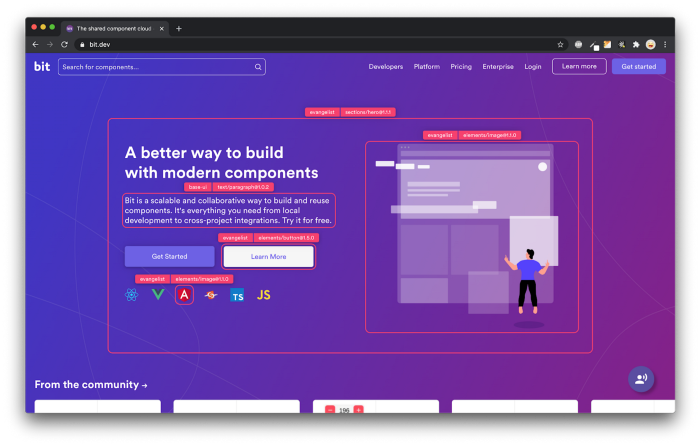
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- The Bit Blog
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
How to Use Desktop Notifications with React was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Ravidu Perera
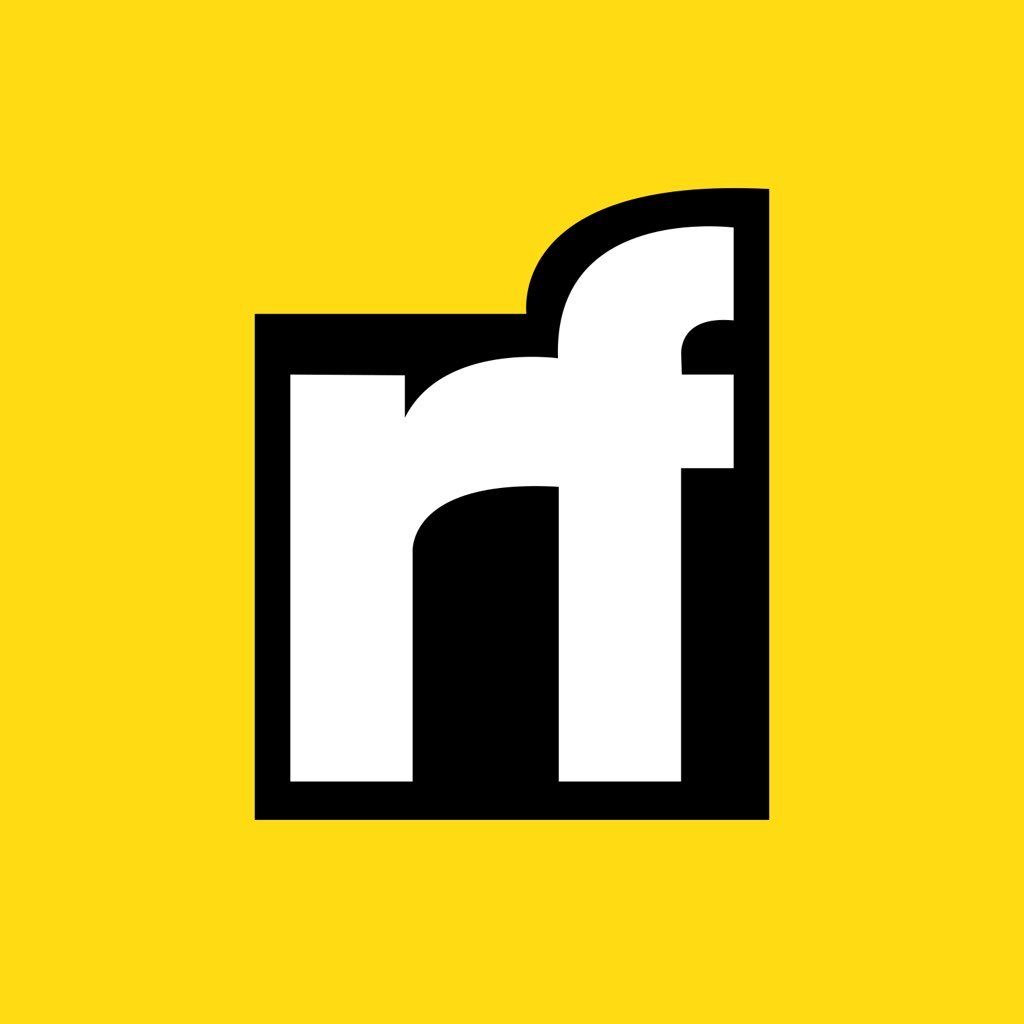
Ravidu Perera | Sciencx (2022-10-20T06:21:39+00:00) How to Use Desktop Notifications with React. Retrieved from https://www.scien.cx/2022/10/20/how-to-use-desktop-notifications-with-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.