This content originally appeared on Level Up Coding - Medium and was authored by Lorenzo Zarantonello
Difference Between LocalStorage And SessionStorage — How To Use Them?
Learn how to store data in localStorage and sessionStorage using JavaScript
Both localStorage and sessionStorage are objects in the browser. You can use the two objects to store data.
TL;DR:
- localStorage data has no expiration time, except when the user is browsing in incognito mode. In that case, data gets removed when the page is closed. The user can also remove data by clearing the browser cache.
- sessionStorage data gets removed when the page is closed.
How To Use LocalStorage?
Here is the generic syntax to save data in localStorage.
localStorage.setItem(variableKey, variableValue);
Store Data
Let’s say you have a string variable called dataValue.
You can save dataValue in localStorage by using the following syntax.
localStorage.setItem("dataKey", "dataValue");
In this example, dataKey represents the key that will be associated with the value dataValue. You can name dataKey as you want.
If dataValue is not a string, you may want to convert it by using JSON.stringify().
Read Data
You can then read data from the store by using
const data = localStorage.getItem("dataKey");
console.log("Data in storage: ", data);
If you previously used JSON.stringify(), you will now need to use the JSON.parse() method on data. However, this is not strictly necessary and it depends on what you want to do with that data.
You can use localStorage in React and Angular. However, you should pay attention to the component lifecycle with the former.
Delete Data
Finally, you may want to delete data fromlocalStorage.
There are two ways: removeItem() and clear().
By using removeItem() you only delete values associated with the key you pass in, e.g. 'dataKey' in the example below.
localStorage.removeItem('dataKey');
By using clear() you will delete everything in the localStorage area associated with the app you are using.
localStorage.clear();
How To Use SessionStorage?
A few remarks on sessionStorage. As reported on MDN:
- One tab = One sessionStorage — Whenever a document is loaded in a particular tab in the browser, a unique page session gets created and assigned to that particular tab. That page session is valid only for that particular tab.
- Tab open = sessionStorage alive — A page session lasts as long as the tab or the browser is open, and survives over page reloads and restores.
- Tab close = clear sessionStorage — Closing a tab/window ends the session and clears objects in sessionStorage.
Having said that, using sessionStorage is very similar to localStorage.
Store Data
Let’s say you have a string variable called dataValue2.
You can save dataValue2 in sessionStorage by using the following syntax.
sessionStorage.setItem("dataKey", "dataValue2");
As in the previous example, dataKey represents the key that will be associated with the value dataValue2. You can name dataKey as you want.
If dataValue2 is not a string, you may want to convert it by using JSON.stringify().
Read Data
You can then read data from the store by using
const data = sessionStorage.getItem("dataKey");
console.log("Data in storage: ", data);
If you previously used JSON.stringify(), you will now need to use the JSON.parse() method on data. However, this is not strictly necessary and it depends on what you want to do with that data.
Delete Data
Finally, you may want to delete data sessionStorage.
There are two ways: removeItem() and clear().
By using removeItem() you only delete values associated with the key you pass in, e.g. 'dataKey' in the example below.
sessionStorage.removeItem('dataKey');
By using clear() you will delete everything in the sessionStorage area associated with the app you are using.
sessionStorage.clear();
While here you can only see an example of localStorageusing React and Angular, using sessionStorage follows a similar pattern.
Difference Between LocalStorage And SessionStorage — How To Use Them? was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Lorenzo Zarantonello
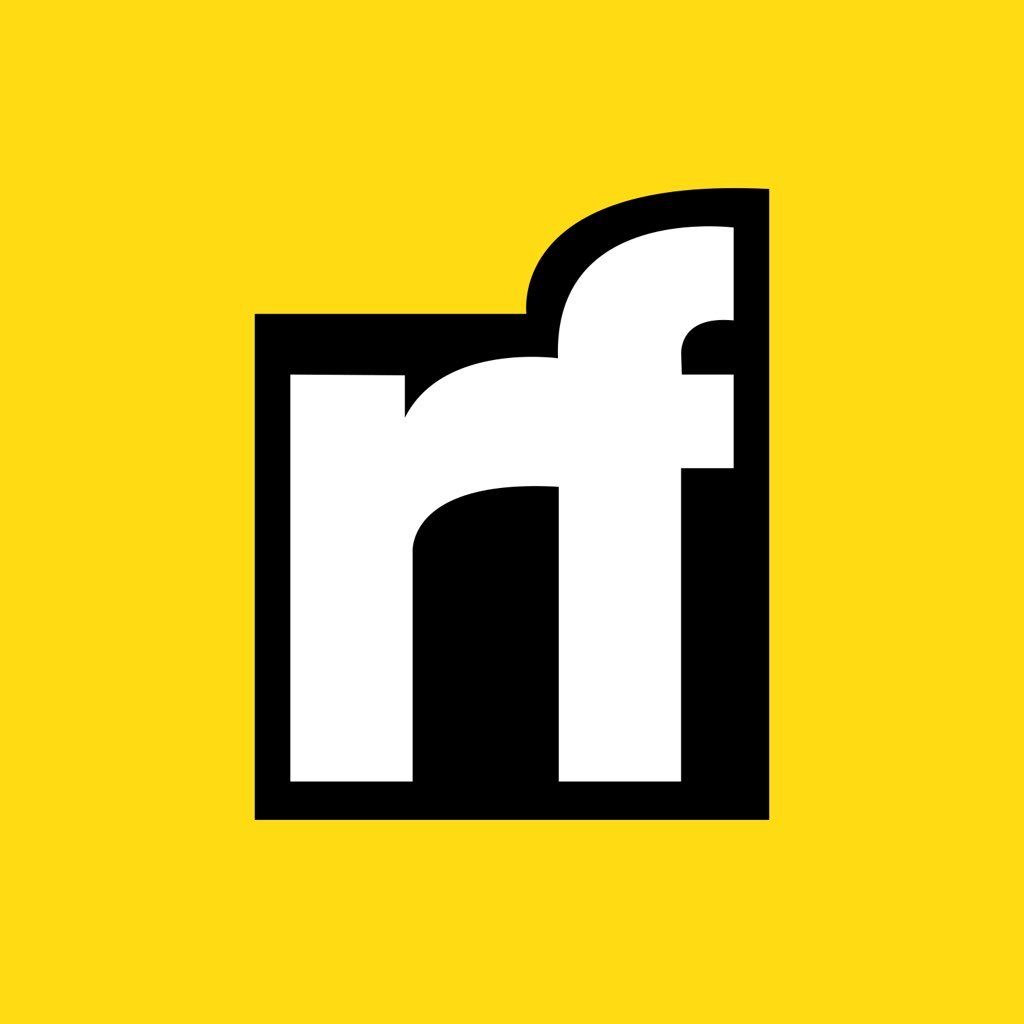
Lorenzo Zarantonello | Sciencx (2022-10-25T02:50:15+00:00) Difference Between LocalStorage And SessionStorage — How To Use Them?. Retrieved from https://www.scien.cx/2022/10/25/difference-between-localstorage-and-sessionstorage-how-to-use-them/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.