This content originally appeared on Envato Tuts+ Tutorials and was authored by George Martsoukos
In this tutorial, we’ll learn how to embed random content inside a grid layout, a popular technique used in different sites to catch visitors’ eyes and promote their products/services.
This is the perfect way to display ads, or promo blocks for your own content. It also gives your layouts an interesting visual break from repetitive grids.
As usual, to better understand what we’re going to build, there’s an accompanying demo. But, as this demo uses some PHP code, it needs a server to run.
You can download the project files from this GitHub repository.
As soon as you’re able to run the demo through a server, notice two things:
- The embedded banners
- and as you reload the page, the banner images change.
Understanding the Layout
Each instructor here at Tuts+ has their own archive page. In a previous tutorial, we recreated a tutorial list like this with our own markup.



The Markup
We used straightforward HTML to build the structure. This time, let’s automate things and store the posts inside a PHP array. Here we’ll go with PHP, but regardless of the language or the CMS/framework, the logic will remain the same. We should loop through the source and retrieve the posts.
Our posts’ format will look as follows:
1 |
$articles = array( |
2 |
array( |
3 |
'title' => 'Quick Tip: Create a Very Simple Parallax Effect With CSS & JavaScript', |
4 |
'image' => 'https://s3-us-west-2.amazonaws.com/s.cdpn.io/162656/parallax-pre.jpg', |
5 |
'link' => 'https://webdesign.tutsplus.com/tutorials/quick-tip-how-to-build-a-dead-simple-parallax-effect-with-css-javascript--cms-33061', |
6 |
'categories' => array( |
7 |
'CSS', |
8 |
'JavaScript', |
9 |
),
|
10 |
),
|
11 |
array( |
12 |
'title' => 'How to Build a Static Portfolio Page With CSS & JavaScript', |
13 |
'image' => 'https://s3-us-west-2.amazonaws.com/s.cdpn.io/162656/chart-pre.png', |
14 |
'link' => 'https://webdesign.tutsplus.com/tutorials/how-to-build-a-portfolio-page-with-css-javascript--cms-32933', |
15 |
'categories' => array( |
16 |
'CSS', |
17 |
'JavaScript', |
18 |
),
|
19 |
),
|
20 |
|
21 |
// more articles here
|
22 |
);
|
And here’s our loop logic:
1 |
<?php if ( ! empty( $articles ) ) : ?> |
2 |
<div class="container"> |
3 |
<ol class="posts"> |
4 |
<?php foreach ( $articles as $key => $article ) : ?> |
5 |
<li class="post"> |
6 |
<article>
|
7 |
<figure>
|
8 |
<a href="<?php echo $article['link']; ?>" target="_blank"> |
9 |
<img width="300" height="208" src="<?php echo $article['image']; ?>" alt="<?php echo $article['title']; ?>"> |
10 |
</a>
|
11 |
<figcaption>
|
12 |
<ol class="post-categories"> |
13 |
<?php foreach ( $article['categories'] as $cat ) : ?> |
14 |
<li>
|
15 |
<a href=""> |
16 |
<?php echo $cat; ?> |
17 |
</a>
|
18 |
</li>
|
19 |
<?php endforeach; ?> |
20 |
</ol>
|
21 |
<h2 class="post-title"> |
22 |
<a href="<?php echo $article['link']; ?>" target="_blank"> |
23 |
<?php echo $article['title']; ?> |
24 |
</a>
|
25 |
</h2>
|
26 |
</figcaption>
|
27 |
</figure>
|
28 |
</article>
|
29 |
</li>
|
30 |
<?php endforeach; ?> |
31 |
</ol>
|
32 |
</div>
|
33 |
<?php endif; ?> |
As said, depending on the language or CMS you’re going to use, things will change. For example, WordPress has a built-in loop for all primary queries.
1 |
<!-- Start the Loop. -->
|
2 |
<?php if ( have_posts() ) : while ( have_posts() ) : the_post(); ?> |
3 |
|
4 |
<!-- Add post info using WP built-in functions -->
|
5 |
|
6 |
<?php endwhile; else : ?> |
7 |
|
8 |
<!-- The very first "if" tested to see if there were any Posts to -->
|
9 |
<!-- display. This "else" part tells what do if there weren't any. -->
|
10 |
<p><?php esc_html_e( 'Sorry, no posts matched your criteria.' ); ?></p> |
11 |
|
12 |
<!-- REALLY stop The Loop. -->
|
13 |
<?php endif; ?> |
The Styles
Apart from the markup, we’ll also keep most of the styles from the previous tutorial. We’ll only make a few changes to make the layout responsive.
Here are all the styles:
1 |
:root { |
2 |
--black: #3a3a3a; |
3 |
--white: #fff; |
4 |
--green: #49b293; |
5 |
}
|
6 |
|
7 |
* { |
8 |
margin: 0; |
9 |
padding: 0; |
10 |
}
|
11 |
|
12 |
img { |
13 |
display: block; |
14 |
max-width: 100%; |
15 |
height: auto; |
16 |
}
|
17 |
|
18 |
ol { |
19 |
list-style: none; |
20 |
}
|
21 |
|
22 |
a { |
23 |
text-decoration: none; |
24 |
color: inherit; |
25 |
}
|
26 |
|
27 |
body { |
28 |
margin: 50px 0; |
29 |
color: var(--black); |
30 |
font: 1rem/1.3 sans-serif; |
31 |
}
|
32 |
|
33 |
.container { |
34 |
max-width: 1200px; |
35 |
padding: 0 15px; |
36 |
margin: 0 auto; |
37 |
}
|
38 |
|
39 |
h1 { |
40 |
text-align: center; |
41 |
margin-bottom: 2rem; |
42 |
}
|
43 |
|
44 |
h1 a { |
45 |
text-decoration: underline; |
46 |
}
|
47 |
|
48 |
.posts { |
49 |
display: grid; |
50 |
grid-gap: 1.5rem; |
51 |
}
|
52 |
|
53 |
.posts .post { |
54 |
width: 300px; |
55 |
margin: 0 auto; |
56 |
border: 1px solid rgba(0, 0, 0, 0.1); |
57 |
}
|
58 |
|
59 |
.posts > li { |
60 |
background: #fafafa; |
61 |
}
|
62 |
|
63 |
.posts .post-title { |
64 |
font-size: 1.3rem; |
65 |
}
|
66 |
|
67 |
.posts .post-title:hover { |
68 |
text-decoration: underline; |
69 |
}
|
70 |
|
71 |
.posts figcaption { |
72 |
padding: 1rem; |
73 |
}
|
74 |
|
75 |
.posts .post-categories { |
76 |
margin-bottom: 0.75rem; |
77 |
font-size: 0.75rem; |
78 |
}
|
79 |
|
80 |
.posts .post-categories * { |
81 |
display: inline-block; |
82 |
}
|
83 |
|
84 |
.posts .post-categories li { |
85 |
margin-bottom: 0.2rem; |
86 |
}
|
87 |
|
88 |
.posts .post-categories a { |
89 |
padding: 0.2rem 0.5rem; |
90 |
border-radius: 1rem; |
91 |
border: 1px solid; |
92 |
line-height: normal; |
93 |
transition: all 0.1s; |
94 |
}
|
95 |
|
96 |
.posts .post-categories a:hover { |
97 |
background: var(--green); |
98 |
color: var(--white); |
99 |
}
|
100 |
|
101 |
@media (min-width: 500px) { |
102 |
.posts { |
103 |
grid-template-columns: repeat(2, 1fr); |
104 |
}
|
105 |
|
106 |
.posts .post { |
107 |
width: auto; |
108 |
}
|
109 |
}
|
110 |
|
111 |
@media (min-width: 600px) { |
112 |
.posts { |
113 |
grid-template-columns: repeat(3, 1fr); |
114 |
}
|
115 |
}
|
116 |
|
117 |
@media (min-width: 900px) { |
118 |
.posts { |
119 |
grid-template-columns: repeat(4, 1fr); |
120 |
}
|
121 |
}
|
Embed Banners
Let’s now assume that we want to place banners inside our grid. In this case, we’ll just use image banners, but in your case, you can insert ads coming from different sources, carousels, videos, or anything else you like.
Our banners have to satisfy the following requirements:
- They should come after every fifth column. In our case, there are 12 posts, so our grid will contain two banners. Of course, in your case, you can have many more.
- On each page load, they should appear randomly, meaning that there won’t be any fixed places for certain banners.
- Plus, the embedded banners should be unique, meaning that a single banner won’t ever appear twice inside the same page.
Here’s an example of the layout we want to produce:



As mentioned, banners will appear randomly, so on another page load, we might see different ones, like this:



To accomplish this behavior, we’ll use different PHP array functions (array_keys
, array_diff
, array_rand
, array_push
).
Let’s take note of the steps we’ll follow:
- Store inside the
$images
array all the banners (coming from Unsplash) and grab the keys from this array. - Initialize the
$exclude_images_keys
array, where we’ll add the key of each banner that is being added to the grid. By default, it’ll be empty. - Inside the loop, we’ll check to see if the current element's index isn’t 0 and is divisible by 5.
- If that happens, we’ll compare the
$images_keys
and$exclude_images_keys
arrays. We’ll return their unique values, if any, and store them inside the$in_items
array. - Grab a random key from the
$in_items
array. - Add this key to the
$exclude_images_keys
array to exclude the associated banner from future selections. - Pick the banner with this key and place it in the grid.
Here’s the PHP code responsible for this functionality:
1 |
<?php
|
2 |
// 1
|
3 |
$images = array( |
4 |
'banner1.jpg', |
5 |
'banner2.jpg', |
6 |
'banner3.jpg', |
7 |
'banner4.jpg', |
8 |
'banner5.jpg', |
9 |
'banner6.jpg', |
10 |
);
|
11 |
$images_keys = array_keys( $images ); |
12 |
$exclude_images_keys = array(); |
13 |
|
14 |
foreach ( $articles as $key => $article ) : |
15 |
// 3
|
16 |
if ( 0 !== $key && 0 === $key % 5 ) : |
17 |
// 4
|
18 |
$in_items = array_diff( $images_keys, $exclude_images_keys ); |
19 |
// 5
|
20 |
$random_image_key = array_rand( $in_items ); |
21 |
// 6
|
22 |
array_push( $exclude_images_keys, $random_image_key ); |
23 |
// 7
|
24 |
$random_image = $images[ $random_image_key ]; |
25 |
?>
|
26 |
<li class="banner"> |
27 |
<img width="800" height="533" src="img/<?php echo $random_image; ?>" alt="banner"> |
28 |
</li>
|
29 |
<?php
|
30 |
endif; |
31 |
...
|
32 |
endforeach; |
And the additional CSS for our banners:
1 |
.posts .banner img { |
2 |
width: 100%; |
3 |
height: 100%; |
4 |
object-fit: cover; |
5 |
}
|
6 |
|
7 |
@media (min-width: 900px) { |
8 |
.posts .banner { |
9 |
grid-column: span 2; |
10 |
}
|
11 |
}
|
Conclusion
That’s all, folks! I hope you enjoyed this little exercise as much as I did and that it gave you some ideas on how to embed advertising items just within a grid layout. Also, by using the modulo operator (%
) in the way we covered here, you can create multi-column layouts with dynamic patterns.
As always, thanks a lot for reading!
This content originally appeared on Envato Tuts+ Tutorials and was authored by George Martsoukos
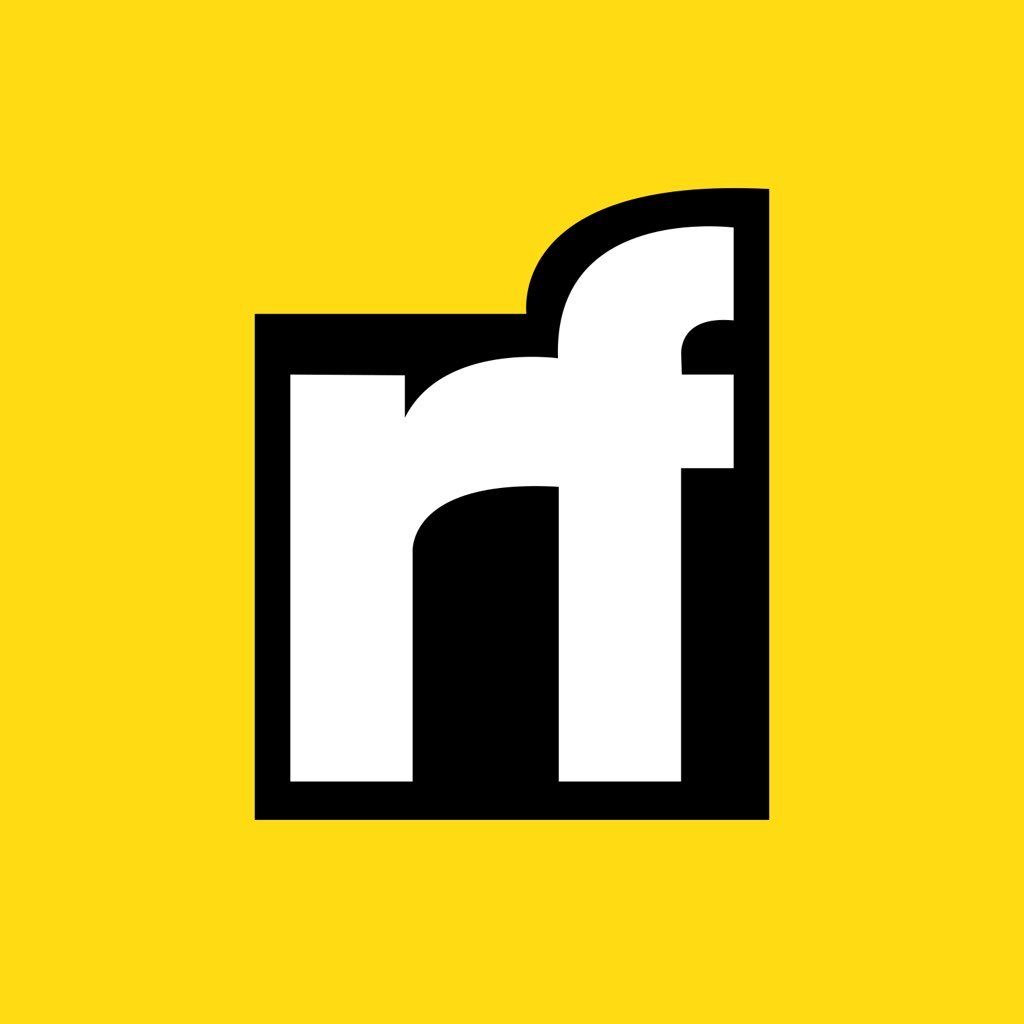
George Martsoukos | Sciencx (2022-10-25T18:04:36+00:00) How to Embed Random Content Inside a Grid Layout (With PHP). Retrieved from https://www.scien.cx/2022/10/25/how-to-embed-random-content-inside-a-grid-layout-with-php/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.