This content originally appeared on Level Up Coding - Medium and was authored by Maxwell
Master these JavaScript tool librarys to make your projects look great.
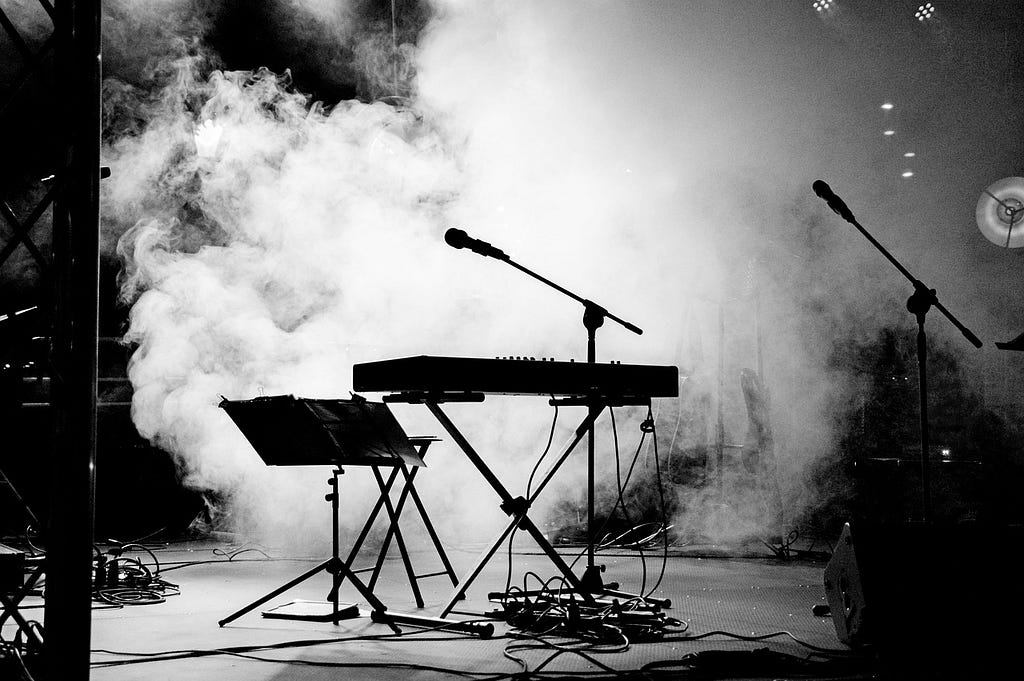
The important difference between experts and ordinary people is that they are good at using tools and leave more time for planning and thinking. The same is true for writing code. With the right tools, you have more time to plan the architecture and overcome difficulties. Today, I will share with you the most popular JavaScript library.
1. qs
A lightweight url parameter conversion JavaScript library.
npm install qs
Basic usage
import qs from 'qs'
qs.parse('user=maxwell&age=32');
// return { user: "maxwell", age: "32" }
qs.stringify({ user: "maxwell", age: "32" });
//return user=maxwell&age=32
Official website:https://www.npmjs.com/package/qs
2. js-cookie
A simple, lightweight JavaScript API for handling cookies.
npm install js-cookie
Basic usage
import Cookies from 'js-cookie'
Cookies.set('name', 'maxwell', { expires: 10 })
// cookies are valid for 10 days
Cookies.get('name') // return 'maxwell'
Official website:https://github.com/js-cookie/js-cookie
3.Day.js
A minimalist JavaScript library for handling time and date, with the same API design as Moment.js, but only 2KB in size.
npm install dayjs
Basic usage
import dayjs from 'dayjs'
dayjs().format('YYYY-MM-DD HH:mm')
dayjs('2022-11-1 12:06').toDate()
Official website:https://day.js.org/
4. Animate.css
A cross-browser css3 animation library with built-in many typical css3 animations, good compatibility and easy to use.
npm install animate.css
Basic usage
<h1 class="animate__animated animate__bounce">
An animated element
</h1>
import 'animate.css'
Official website: https://animate.style/
5. animejs
A powerful Javascript animation library. Can work with CSS3 properties, SVG, DOM elements, and JS objects to create a variety of high-performance, smooth transition animation effects.
npm install animejs
Basic usage
<div class="ball" style="width: 50px; height: 50px; background: blue"></div>
import anime from 'animejs/lib/anime.es.js'
// After the page is rendered, execute
anime({
targets: '.ball',
translateX: 250,
rotate: '1turn',
backgroundColor: '#F00',
duration: 800
})
Official website:https://animejs.com/
6. lodash.js
A modern JavaScript utility library delivering modularity, performance & extras.
npm install lodash
Basic usage
import _ from 'lodash'
_.max([4, 2, 8, 6]) // returns the maximum value in the array => 8
_.intersection([1, 2, 3], [2, 3, 4])
// returns the intersection of multiple arrays => [2, 3]
Official website:https://lodash.com/
7. vConsole
A lightweight, extendable front-end developer tool for mobile web pages. If you are still struggling with how to debug code on your phone, use it.
vConsole is framework-free, you can use it in Vue or React or any other framework application.
npm install vconsole
Basic usage
import VConsole from 'vconsole';
const vConsole = new VConsole();
// or init with options
const vConsole = new VConsole({ theme: 'dark' });
// call `console` methods as usual
console.log('Hello world');
// remove it when you finish debugging
vConsole.destroy();
Official website:https://github.com/Tencent/vConsole
8. Chart.js
A simple, clean and attractive JavaScript charting library based on HTML5
Simple yet flexible JavaScript charting for designers & developers
npm install chart.js
Basic usage
<canvas id="myChart" width="500" height="500"></canvas>
import Chart from 'chart.js/auto'
// executed after page rendering is complete
const ctx = document.getElementById('myChart')
const myChart = new Chart(ctx, {
type: 'bar',
data: {
labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'],
datasets: [
{
label: '# of Votes',
data: [12, 19, 3, 5, 2, 3],
backgroundColor: [
'rgba(255, 99, 132, 0.2)',
'rgba(54, 162, 235, 0.2)',
'rgba(255, 206, 86, 0.2)',
'rgba(75, 192, 192, 0.2)',
'rgba(153, 102, 255, 0.2)',
'rgba(255, 159, 64, 0.2)'
],
borderColor: [
'rgba(255, 99, 132, 1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(75, 192, 192, 1)',
'rgba(153, 102, 255, 1)',
'rgba(255, 159, 64, 1)'
],
borderWidth: 1
}
]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
})
Each of the above libraries is tested by myself, and the company’s projects are basically in use. If you have any questions, please feel free to communicate in the comment area. If you have other good tool libraries, please share them and improve work efficiency together. if you are interested in my articles, you can follow me on Medium or Twitter.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Placing developers like you at top startups and tech companies
8 Commonly Used JavaScript Libraries, Become a Real Master was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Maxwell
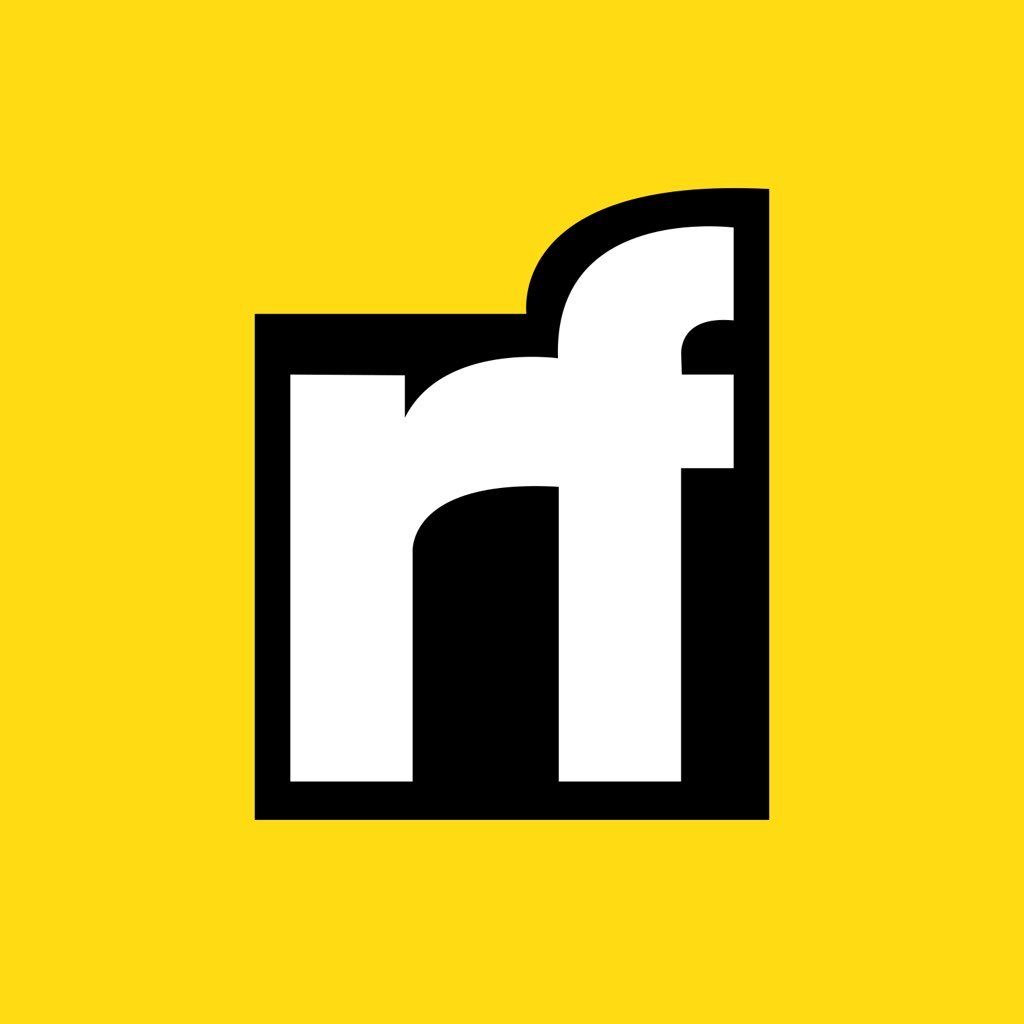
Maxwell | Sciencx (2022-11-02T12:10:06+00:00) 8 Commonly Used JavaScript Libraries, Become a Real Master. Retrieved from https://www.scien.cx/2022/11/02/8-commonly-used-javascript-libraries-become-a-real-master/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.