This content originally appeared on Level Up Coding - Medium and was authored by Maxwell
Good code is refreshing and easy to understand, and also gives you a sense of accomplishment.

As programmers, we need a lot of writing skills to write code as well. A good code can be refreshing, easy to understand, comfortable and natural, and at the same time make you feel full of achievement. So, I’ve put together some JS development tips that I’ve used to help you write code that is refreshing, easy to understand, and comfortable.
String Tips
- Generate random ID
const RandomId = len => Math.random().toString(36).substr(3, len);
const id = RandomId(10);
console.log(id); //l5fy28zzi5
2. Formatting Money
const ThousandNum = num => num.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
const money = ThousandNum(2022112213);
console.log(money); //2,022,112,213
3. Generate star ratings
const StartScore = rate => "★★★★★☆☆☆☆☆".slice(5 - rate, 10 - rate);
const start = StartScore(3);
console.log(start); // ★★★☆☆
4. Generate random HEX color values
const RandomColor = () => "#" + Math.floor(Math.random() * 0xffffff).toString(16).padEnd(6, "0");
const color = RandomColor();
console.log(color); // #bd1c55
5. Operation URL query parameters
const params = new URLSearchParams(location.search.replace(/\?/ig, ""));
// location.search = "?name=young&sex=male"
params.has("young"); // true
params.get("sex"); // "male"
Number Tips
- rounding
Math.floor() for positive numbers, Math.ceil() for negative numbers.
const num1 = ~~2.69;
const num2 = 3.69 | 0;
const num3 = 5.69 >> 0;
console.log(num1); // 2
console.log(num2); // 3
console.log(num3); // 5
2. Complementary zero
const FillZero = (num, len) => num.toString().padStart(len, "0");
const num = FillZero(187, 6);
console.log(num); //000187
3. Transfer Value
Valid only for null, “”, false, numeric strings
const num1 = +null;
const num2 = +"";
const num3 = +false;
const num4 = +"189";
console.log(num1); //0
console.log(num2); //0
console.log(num3); //0
console.log(num4); //189
4. Timestamp
const timestamp = +new Date("2022-11-21");
console.log(timestamp); //1668988800000
5. Precise decimals
const RoundNum = (num, decimal) => Math.round(num * 10 ** decimal) / 10 ** decimal;
const num = RoundNum(5.78602, 2);
console.log(num); //5.79
6. Determining parity
const OddEven = num => !!(num & 1) ? "odd" : "even";
const num = OddEven(6);
console.log(num); //even
7. Take the minimum and maximum value
const arr = [31,22, 21,18,12,78,25];
const min = Math.min(...arr);
const max = Math.max(...arr);
console.log(min); //12
console.log(max); //78
Array Tips
- Clone array
const _arr = [0, 1, 2];
const arr = [..._arr];
// arr => [0, 1, 2]
2. Merge Arrays
const arr1 = [0, 1, 2];
const arr2 = [3, 4, 5];
const arr = [...arr1, ...arr2];
// arr => [0, 1, 2, 3, 4, 5];
3. De-duplicated array
const arr = [...new Set([0, 1, 1, null, null])];
// arr => [0, 1, null]
4. Async Accumulation
async function Func(deps) {
return deps.reduce(async(t, v) => {
const dep = await t;
const version = await Todo(v);
dep[v] = version;
return dep;
}, Promise.resolve({}));
}
const result = await Func(); // Needs to be used under async bracket
5. Insert member at the beginning of the array
let arr = [1, 2];
// Choose any one of the following methods
arr.unshift(0);
arr = [0].concat(arr);
arr = [0, ...arr];
// arr => [0, 1, 2]
6. Insert member at the end of the array
let arr = [0, 1]; // Choose any one of the following methods
arr.push(2);
arr.concat(2);
arr[arr.length] = 2;
arr = [...arr, 2];
// arr => [0, 1, 2]
7. Create an array of specified length
const arr = [...new Array(3).keys()];
// arr => [0, 1, 2]
8. reduce instead of map and filter
const _arr = [0, 1, 2];
// map
const arr = _arr.map(v => v * 2);
const arr = _arr.reduce((t, v) => {
t.push(v * 2);
return t;
}, []);
// arr => [0, 2, 4]
// filter
const arr = _arr.filter(v => v > 0);
const arr = _arr.reduce((t, v) => {
v > 0 && t.push(v);
return t;
}, []);
// arr => [1, 2]
// map和filter
const arr = _arr.map(v => v * 2).filter(v => v > 2);
const arr = _arr.reduce((t, v) => {
v = v * 2;
v > 2 && t.push(v);
return t;
}, []);
// arr => [4]
Object Tips
- Clone object
const _obj = { a: 0, b: 1, c: 2 };
// Choose any one of the following methods
const obj = { ..._obj };
const obj = JSON.parse(JSON.stringify(_obj));
// obj => { a: 0, b: 1, c: 2 }
2. Merge objects
const obj1 = { a: 0, b: 1, c: 2 };
const obj2 = { c: 3, d: 4, e: 5 };
const obj = { ...obj1, ...obj2 };
// obj => { a: 0, b: 1, c: 3, d: 4, e: 5 }
3 . Object Literal
const env = "prod";
const link = {
dev: "Development Address",
test: "Testing Address",
prod: "Production Address"
}[env];
// link => "Production Address"
4. Create empty object
const obj = Object.create(null);
Object.prototype.a = 0;
// obj => {}
5. Remove useless properties of an object
const obj = { a: 0, b: 1, c: 2 }; // Only want to take b and c
const { a, ...rest } = obj;
// rest => { b: 1, c: 2 }
Thank you for reading, and we will continue to compile more Javascript development tips for you in subsequent articles. If you are interested in my articles, you can follow me.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Some JavaScript Tips To Make You Look More Like a Master was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Maxwell
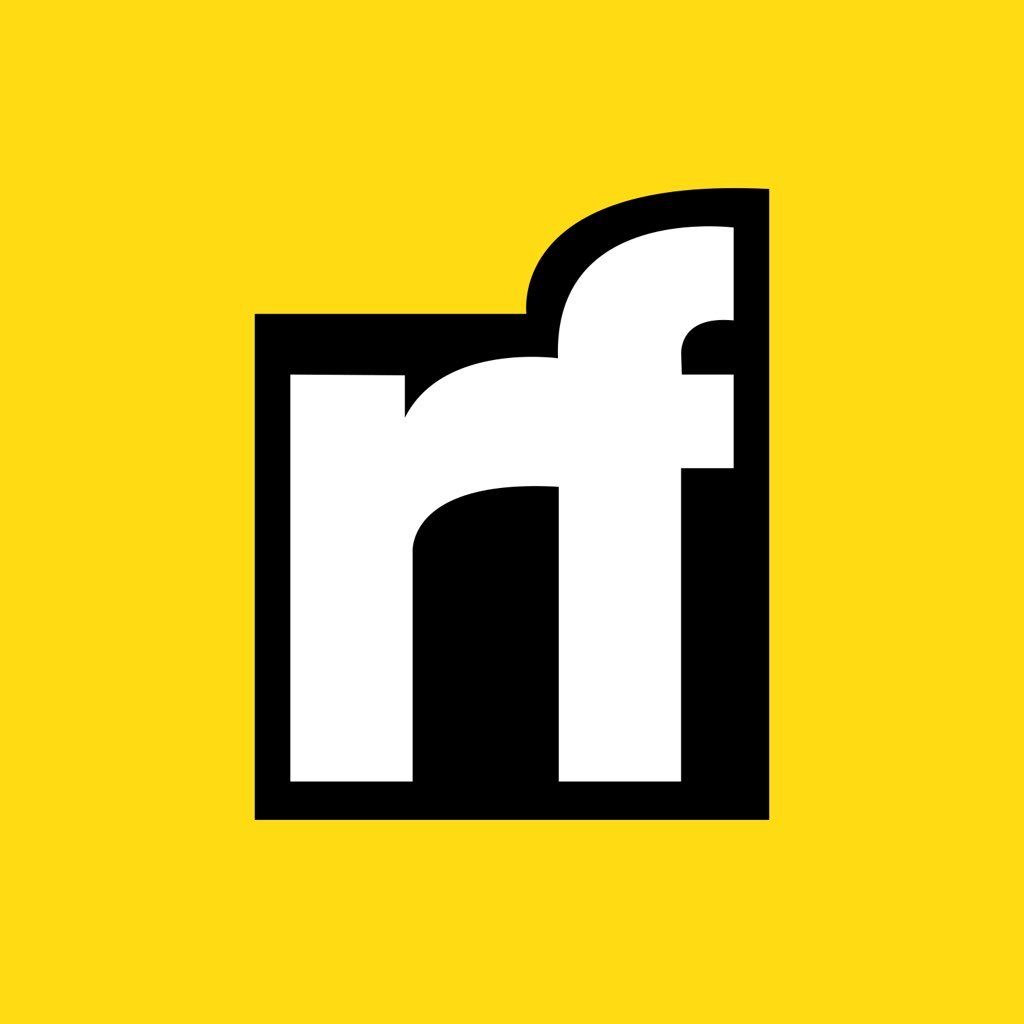
Maxwell | Sciencx (2022-11-23T02:31:48+00:00) Some JavaScript Tips To Make You Look More Like a Master. Retrieved from https://www.scien.cx/2022/11/23/some-javascript-tips-to-make-you-look-more-like-a-master/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.