This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Christian Heilmann
GitHub Copilot is incredible, and if you check what's happening in the preview released as the Copilot Labs extension it will only get more amazing.
Check this out:
Let's start with a basic JavaScript that checks a string length and reports when it is empty. One with an obvious mistake, as we use a single =
instead of the type safe ===
. This will always report the string as empty.
if (str.length = 0) {
console.error('String is empty');
}
Using the Fix bugs
brush, this is rectified.
if (str.length === 0) {
console.error('String is empty');
}
Using the Clean
brush also adds reporting when the string is not empty.
if (str.length === 0) {
console.error('String is empty');
} else {
console.log('String is not empty');
}
Using the List steps
brush adds comments for step (sure, this is redundant, but it also works for much more complex code).
// check the length of the string
if (str.length === 0) {
// if length is 0, print 'String is empty'
console.error('String is empty');
} else {
// if length is not 0, print 'String is not empty'
console.log('String is not empty');
}
Using Make robust
adds error reporting instead of logging and an extra check to make sure that str
is really a String and not an empty Array.
function test(str) {
if (str.length === 0) {
throw new Error('String is empty');
} else if (typeof str !== 'string') {
throw new Error('It is not a string');
} else {
console.log('String is not empty');
}
}
The Add types
brush does exactly that.
/**
* @param {string} str
* @returns {string}
*/
function test(str) {
if (str.length === 0) {
throw new Error('String is empty');
} else if (typeof str !== 'string') {
throw new Error('It is not a string');
} else {
console.log('String is not empty');
}
}
You can also define custom brushes and there are more to come.
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Christian Heilmann
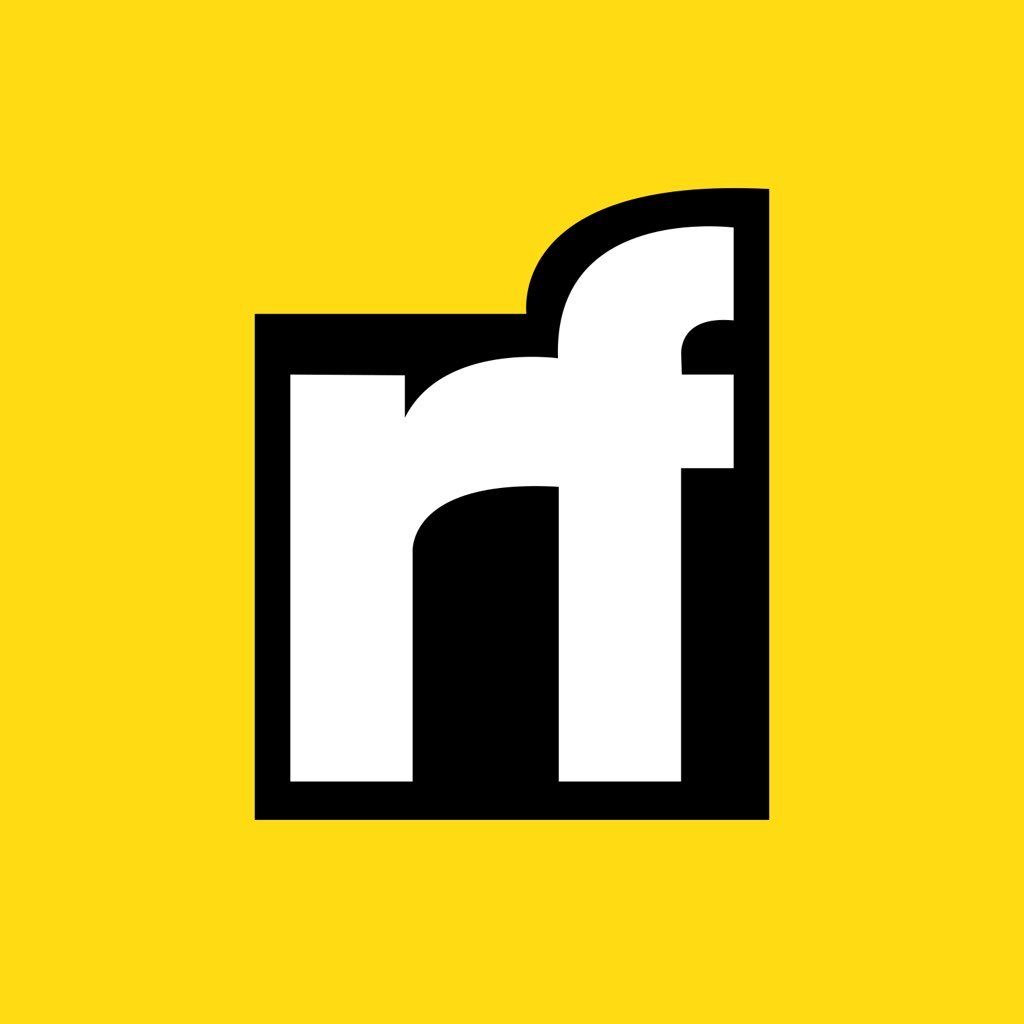
Christian Heilmann | Sciencx (2022-12-13T15:45:47+00:00) Code brushes for GitHub Copilot. Retrieved from https://www.scien.cx/2022/12/13/code-brushes-for-github-copilot/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.