This content originally appeared on Level Up Coding - Medium and was authored by Nic Chong
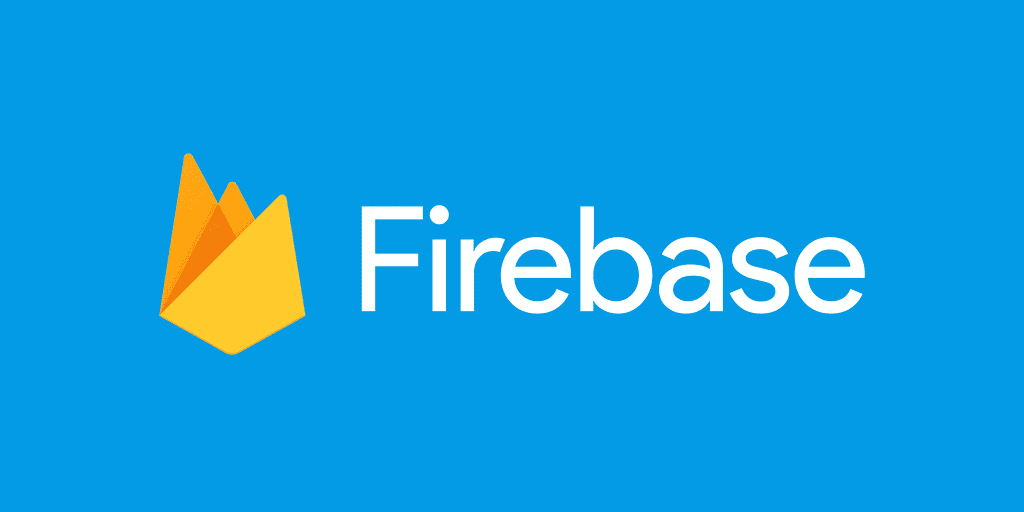
Before you continue, read:
Part 1: 10 tips for optimizing your Firestore database
Firestore is a powerful NoSQL database that is well-suited for storing and retrieving large amounts of data. However, it’s important to be mindful of the size of the data you store in your database, as large objects can impact performance and increase the amount of data you need to store and retrieve.
Here are some tips for avoiding storing large objects in your Firestore database:
- Store large binary objects (such as images and videos) in a separate storage service: Firestore is designed for storing structured data, such as documents and collections, rather than large binary objects. If you need to store large binary objects, it’s generally more efficient to use a separate storage service, such as Google Cloud Storage or Amazon S3.
- Use references instead of embedding data: If you need to store a large amount of data within a document, it can be more efficient to use references instead of embedding the data directly. A reference is a special type of field that stores the ID of another document in your database, rather than the entire document itself. When you need to access the data, you can use a query to retrieve the referenced document separately. This can reduce the size of your documents and improve performance, especially for data that is accessed infrequently or is not needed in real-time.
- Consider breaking large objects into smaller pieces: If you have a large object that needs to be stored within a document, you might consider breaking it into smaller pieces and storing each piece separately. This can make it easier to work with the data and reduce the size of your documents.
- Monitor the size of your documents: It’s a good idea to regularly monitor the size of your documents to ensure that they are not getting too large. If you notice that your documents are consistently exceeding a certain size, it might be time to reevaluate your data structure and consider ways to optimize it.
By following these tips and being mindful of the size of the data you store in your Firestore database, you can improve the performance and scalability of your application. It’s important to consider the specific needs and requirements of your application when deciding how to store and retrieve data, as well as the trade-offs of different data storage approaches.
Tip #7 Use Cloud Functions to automate backend tasks
Cloud Functions is a serverless platform provided by Google Cloud that allows you to run code in response to events or HTTP requests. You can use Cloud Functions to automate backend tasks and build scalable, real-time applications on top of Firestore and other Google Cloud services.
Here are some examples of how you can use Cloud Functions to automate backend tasks:
- Perform automatic data validation and cleansing: You can use Cloud Functions to automatically validate and cleanse data as it is written to your Firestore database. For example, you might use a Cloud Function to ensure that all data is in the correct format or to remove invalid data before it is stored in your database.
- Send notifications and emails: You can use Cloud Functions to send notifications or emails in response to events in your Firestore database. For example, you might use a Cloud Function to send an email to a user when their account is created or to send a notification to a group of users when a new item is added to a shared list.
- Trigger updates to other services: You can use Cloud Functions to trigger updates to other services in response to events in your Firestore database. For example, you might use a Cloud Function to update a search index whenever a new document is added to your database, or to send data to a analytics service whenever a user performs a certain action.
- Perform scheduled tasks: You can use Cloud Functions to perform tasks on a regular schedule, such as cleaning up old data or sending periodic reports.
To get started with Cloud Functions, you will need to install the Google Cloud Functions CLI and create a new function. You can then define the trigger for your function, such as a specific event or HTTP request, and write the code to perform the desired action.
Cloud Functions can be an extremely powerful tool for automating backend tasks and building scalable applications on top of Firestore. By taking advantage of the serverless architecture, you can focus on building your application and let Cloud Functions handle the underlying infrastructure.
Tip #8 Monitor your database usage and performance
To ensure that your Firestore database is performing optimally, it’s important to regularly monitor your database usage and performance. This can help you identify any issues or bottlenecks, and take proactive steps to address them.
Here are some tips for monitoring your Firestore database:
- Use the Firebase console: The Firebase console provides a range of tools and metrics for monitoring your Firestore database. You can use the console to view real-time data on your database usage, including the number of read and write operations, the size of your data, and the number of active connections. You can also use the console to view performance and usage metrics over time, and to set up alerts for key performance indicators.
- Use the Firestore Profiling tool: The Firestore Profiling tool is a powerful tool that allows you to see how your Firestore database is being used in real-time. You can use the tool to see the most expensive queries and operations, and to identify any potential performance issues.
- Use Cloud Monitoring: Google Cloud Monitoring is a comprehensive monitoring service that allows you to monitor the performance and availability of your Firestore database and other Google Cloud services. You can use Cloud Monitoring to set up alerts for key performance indicators, and to view metrics and logs for your database over time.
- Use the Firestore REST API: You can use the Firestore REST API to retrieve usage and performance metrics for your database. The API provides a range of metrics, including the number of read and write operations, the size of your data, and the number of active connections.
By monitoring your Firestore database, you can ensure that it is performing optimally and identify any issues or bottlenecks before they become a problem. This can help you maintain the performance and scalability of your application and ensure that your users have a seamless experience.
Tip #9 Use caching to reduce network latency
Caching is a common technique for reducing network latency and improving the performance of an application. By storing frequently accessed data in a cache, you can avoid the need to retrieve the data from the database over the network, which can significantly improve the speed and responsiveness of your application.
There are several ways you can use caching to reduce network latency in a Firestore application:
- Use a client-side cache: You can use a client-side cache, such as the browser cache or a library like PouchDB, to store data locally on the client’s device. This can reduce the amount of data that needs to be transferred over the network and improve the performance of your application.
- Use a server-side cache: You can use a server-side cache, such as Redis or Memcached, to store data on a dedicated cache server. This can reduce the load on your Firestore database and improve the performance of your application.
- Use a cache-aside pattern: The cache-aside pattern is a common approach to caching in which you store data in a cache whenever it is retrieved from the database. This can help reduce the number of read operations you need to perform and improve the performance of your application.
- Use Cloud Functions: You can use Cloud Functions to automatically cache data in response to events in your Firestore database. For example, you might use a Cloud Function to cache data whenever it is updated or accessed, or to cache data on a regular schedule.
By using caching to reduce network latency, you can improve the performance of your Firestore application and provide a better user experience. It’s important to carefully consider your specific needs and requirements when deciding which caching approach to use, as each has its own trade-offs and limitations.
Tip #10 Use real-time listeners to get updates in real time
Firestore provides real-time listeners that allow you to get updates in real-time as data in your database changes. This can be useful for building real-time applications that need to stay up to date with the latest data.
Here are some examples of how you can use real-time listeners in a Firestore application:
- Chat applications: You can use real-time listeners to build a chat application that updates in real-time as new messages are added to the database.
- Collaborative editing: You can use real-time listeners to build a collaborative editing application that updates in real-time as users make changes to a shared document.
- Real-time dashboards: You can use real-time listeners to build a dashboard that updates in real-time as data changes in your database.
To use real-time listeners in your Firestore application, you will need to use the onSnapshot method provided by the Firestore JavaScript library. This method allows you to listen for changes to a document or query and receive updates as they occur.
Here’s an example of how you can use the onSnapshot method to listen for changes to a document:
db.collection("users").doc("u1").onSnapshot(function(doc) {
console.log("Document data:", doc.data());
});
This code listens for changes to the “u1” document in the “users” collection and logs the updated data to the console whenever the document changes.
Real-time listeners can be a powerful tool for building real-time applications with Firestore. By using these listeners, you can ensure that your application stays up to date with the latest data and provides a seamless user experience.
Conclusion and summary of key takeaways for optimizing your Firestore database
In this blog post, we covered several tips for optimizing your Firestore database and improving the performance of your application. Here are the key takeaways:
- Choose the right database mode for your use case: Firestore provides two modes for storing data: native mode and datastore mode. Native mode is optimized for storing and querying large amounts of data, while datastore mode is optimized for transactions and consistency. It’s important to choose the right mode for your use case to ensure that you get the best performance.
- Design an efficient and scalable data structure: Carefully designing your data structure can greatly impact the performance of your Firestore database. It’s important to consider the specific needs and requirements of your application when deciding how to structure your data, and to use techniques such as denormalization to reduce the need for joins.
- Use batch operations to improve performance: Batch operations allow you to group multiple read and write operations together and perform them as a single unit. This can significantly improve the performance of your Firestore database, especially for workloads that require a large number of read or write operations.
- Avoid storing large objects in your database: Large objects, such as images and videos, can impact performance and increase the amount of data you need to store and retrieve. It’s generally more efficient to store large binary objects in a separate storage service, such as Google Cloud Storage or Amazon S3.
- Use Cloud Functions to automate backend tasks: Cloud Functions is a serverless platform that allows you to run code in response to events or HTTP requests. You can use Cloud Functions to automate backend tasks and build scalable, real-time applications on top of Firestore.
- Monitor your database usage and performance: Regularly monitoring your database usage and performance can help you identify any issues or bottlenecks, and take proactive steps to address them.
- Use caching to reduce network latency: Caching is a common technique for reducing network latency and improving the performance of an application. By storing frequently accessed data in a cache, you can avoid the need to retrieve the data from the database over the network.
- Use real-time listeners to get updates in real-time: Firestore provides real-time listeners that allow you to get updates in real-time as data in your database changes. This can be useful for building real-time applications that need to stay up to date with the latest data.
By following these tips and being mindful of the performance of your Firestore database, you can ensure that your application is scalable and efficient. It’s important to consider the specific needs and requirements of your application when deciding how to optimize your database, and to regularly monitor and test the performance of your database to ensure that it is performing optimally.
Don’t Miss my upcoming content and tech guides:
Get an email whenever Nic Chong publishes.
If you have any questions, I am here to help, waiting for you in the comments section :)
Part 2: 10 tips for optimizing your Firestore database was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Nic Chong
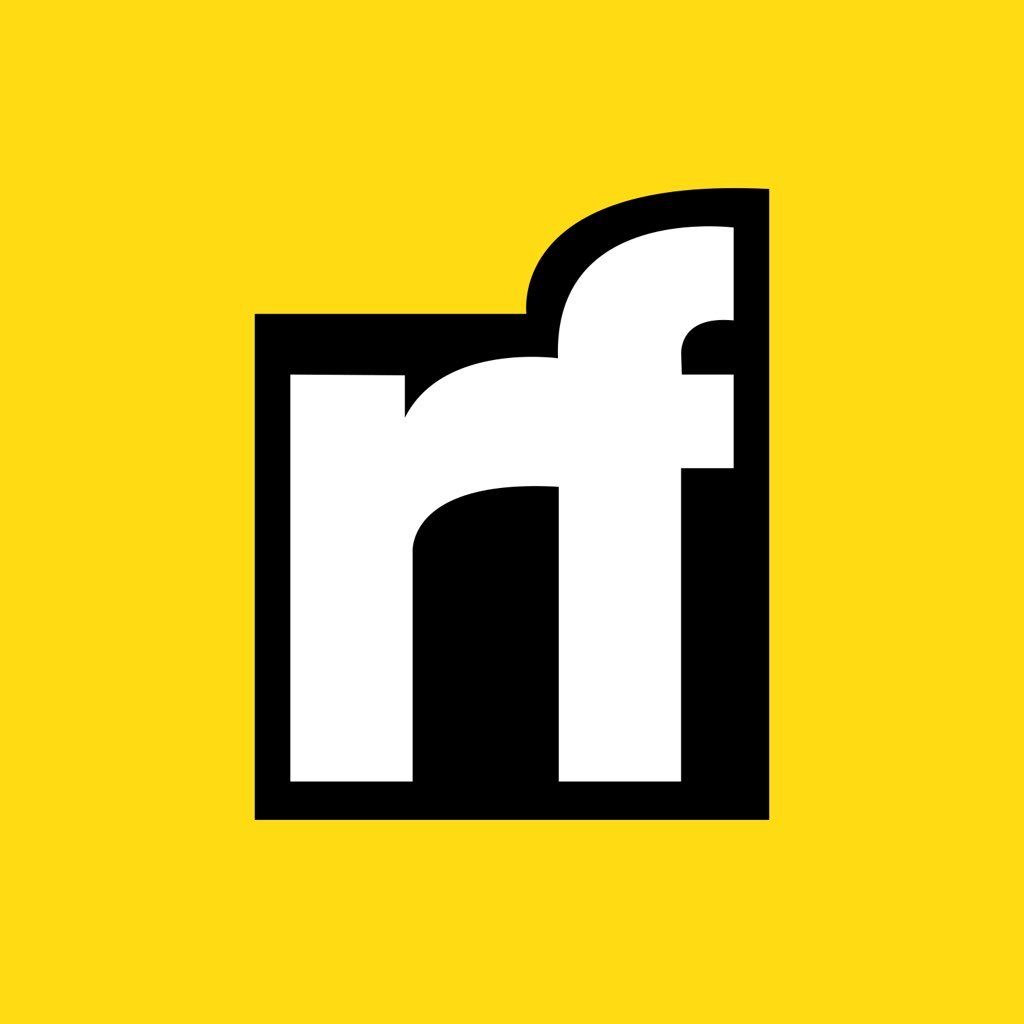
Nic Chong | Sciencx (2023-01-03T13:49:27+00:00) Part 2: 10 tips for optimizing your Firestore database. Retrieved from https://www.scien.cx/2023/01/03/part-2-10-tips-for-optimizing-your-firestore-database/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.