This content originally appeared on Bits and Pieces - Medium and was authored by Fernando Doglio
An overview of the final implementation of this feature
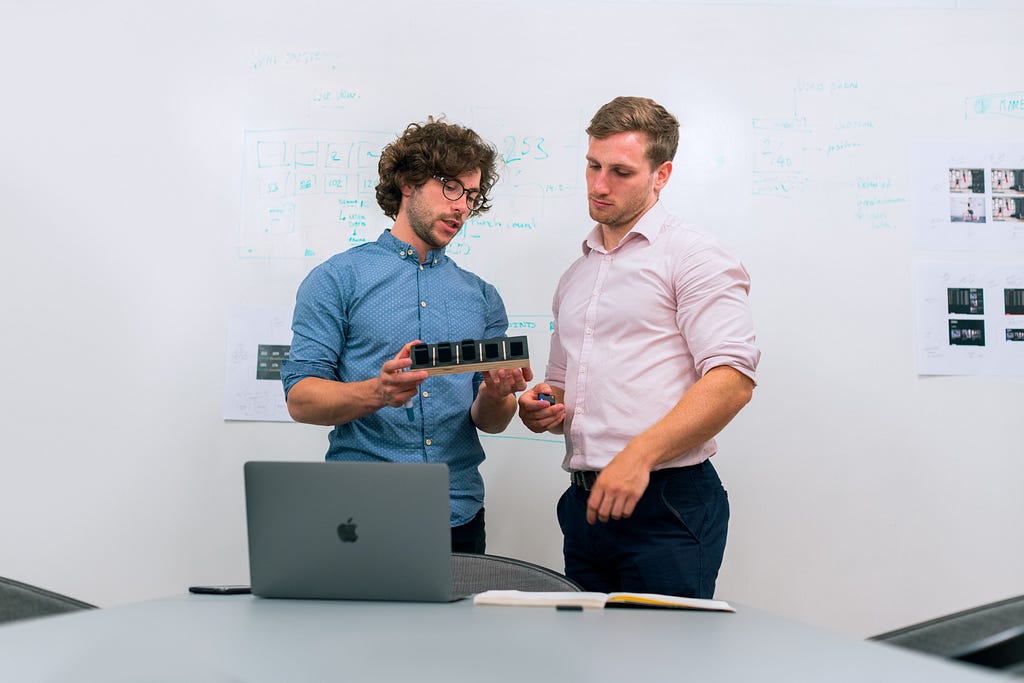
Server components is a feature of React that has been coming for a while now. We’ve all seen preliminary impressions about them and how they promise to change the way we design our apps.
With the release of Next 13, we also got access to this feature. It seems they’ve integrated it into the framework and now with a simple directive we can specify if a component is a server or a client component.
So let’s take a look at what server components are, what they look like and why you’d want to use them with Next 13!
What exactly are server components?
Server components, put simply, are components that get rendered on the server, as opposed to regular components (client components) that need the browser for that.
Of course there are more layers to the onion that are server components, but the gist of it is that.
You’re now able to specify which components get rendered on the server and which ones get rendered on the client.
Wait, are they the same as Server-Side Rendering?
That’s a good question, one I asked myself as well.
After all, we are talking about rendering components on the server. However, a small but very significant difference is that while SSR will render the entire page, resulting in the server returning all the HTML you need, server components will only render a single component and they’ll make the server return only THAT HTML.
Again, small, but definitely important.
That difference means that for pages that have tons of components and some of them are either heavy or do a lot of processing (such as data loading), you can split the load, and have both, the server and the client working in parallel to render all components as fast as possible.
Of course, this is all almost completely transparent to you as the developer. There are some rules you can’t break, but all in all, you’re free to mix and match components.
The only limitations:
- Server-side components can’t be imported by client-side components. After all, the server-side one might have servers-specific code that won’t work on the browser
- You can pass props between server and client components, but the data in them needs to be serializable. This is obvious if you think about it, after all there is no easy way to transfer a class or a date object between server and client. And whether you realize it or not, that’s what you’re doing by sharing props, you’re transferring data between both environments.
As long as you keep that in mind, you can code as if you were just writing a normal React application. If you ask me, that transparency (or rather, “almost” complete transparency) is a fantastic piece of Developer Experience. We’re slowly seeing a blending of both environments where frameworks really promote one of the least useful benefits of JavaScript until today: isomorphism. Or the ability to write code that works well both on the front-end and the back-end.
While the language has permitted that for a while now, we’ve lacked the tools to do it. There are always differences between the runtime APIs of both front and back end. This makes it impossible to truly write isomorphic code.
And while we’re not there yet with Server Components, we’re definitely getting closer.
When would you want to create server components?
So far, we’ve been living just fine without server components, so what makes them so great that developers will now want to start using them?
Let me answer that question with an example:
Pretend you’re using React without server/client components. You’re actually building a very simple Weather app with NextJS 12, and you need to get the data from the weather API.
We’ve all been through this example, you’ll build a component that sends a request to your server, where you’ve built an API endpoint that takes care of sending a request to the actual weather API. That endpoint will then clean the data, and return it to the client.
The client will then parse it and display it on the UI.
Sounds familiar?
You can of course write a React component that directly queries the weather API, but you might run into issues like CORS, security concerns if the API requires a key to be used, etc. So you usually go with the first approach.
However, with server components you can now actually build a component that directly queries the API, and if you make it a server component, it’ll be executed on the server.
This means:
- No CORS issues to worry about.
- No security concerns,. All API keys and any other type of secrets never get sent to the client.
- There is less code involved. You don’t have to write the component AND your own API endpoint, you can simply have the component do all the work.
Overall, the process is much smoother and results in cleaner code.
How do you define a server component in NextJS 13?
This is the best part, doing this is trivial.
To define a server component, simply add the 'use client' directive at the top of the file.
And you only have to do that with the components you specifically want to be rendered on the client, otherwise Next will decide where to render them based on the code you’re using.
For example, if you don’t specify a directive, and inside your component you use useEffect or useState , then Next will still render it inside the browser.
However, if you write it like this, the component will be rendered on the back-end:
This code is defining an async function that will render the component. It also performs an async fetch operation to get some data from an external API.
The rendered output from this component and the app using it, is the following:
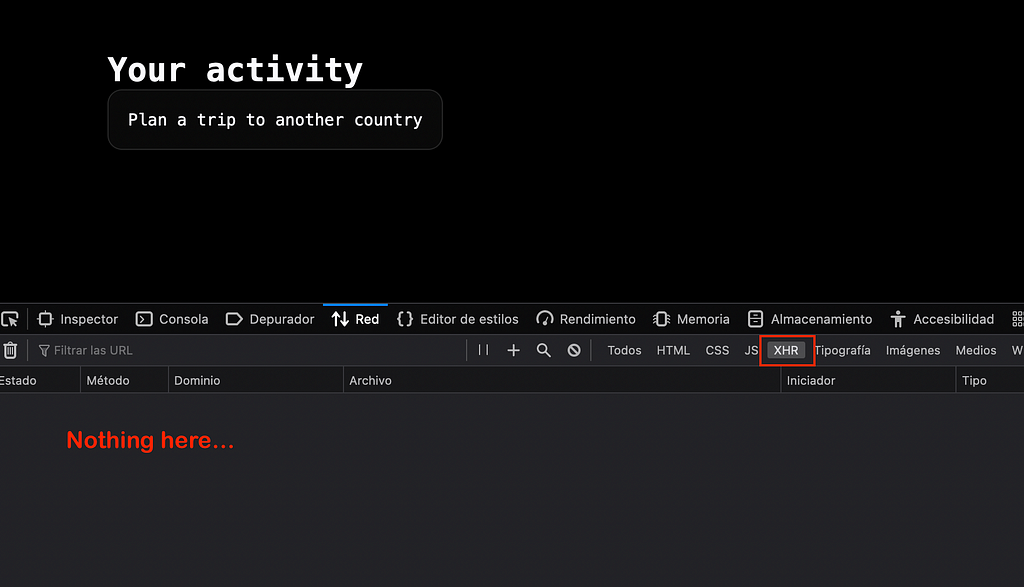
The screenshot shows the devtools on Firefox, and you can see there are no XHR requests being done for the site. That’s because this component was rendered on the server.
You can’t even get the source code for this component on dev mode, because all we get on the browser is the result.
How cool is that?!
Server components are a very nice addition to the React ecosystem, it simplifies the process of building complex apps, and also solidify the bond between JavaScript and React. Until now you could very well use any back-end language, and still get all the benefits from React. Now however, there is an extra addon that will only be there if you decide to go with JavaScript for both environments.
There is still more to server components, so if you liked what you read so far, check out the rest of the docs!
What are your thoughts on server components? Are you using them already?
Build apps with reusable components like Lego
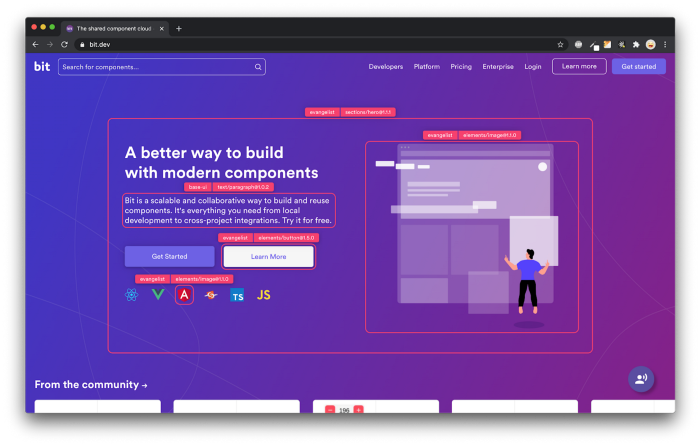
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- Bit - Component driven development
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Unleash the Power of Server-Side Rendering with React Server Components and Next.js 13 was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Fernando Doglio
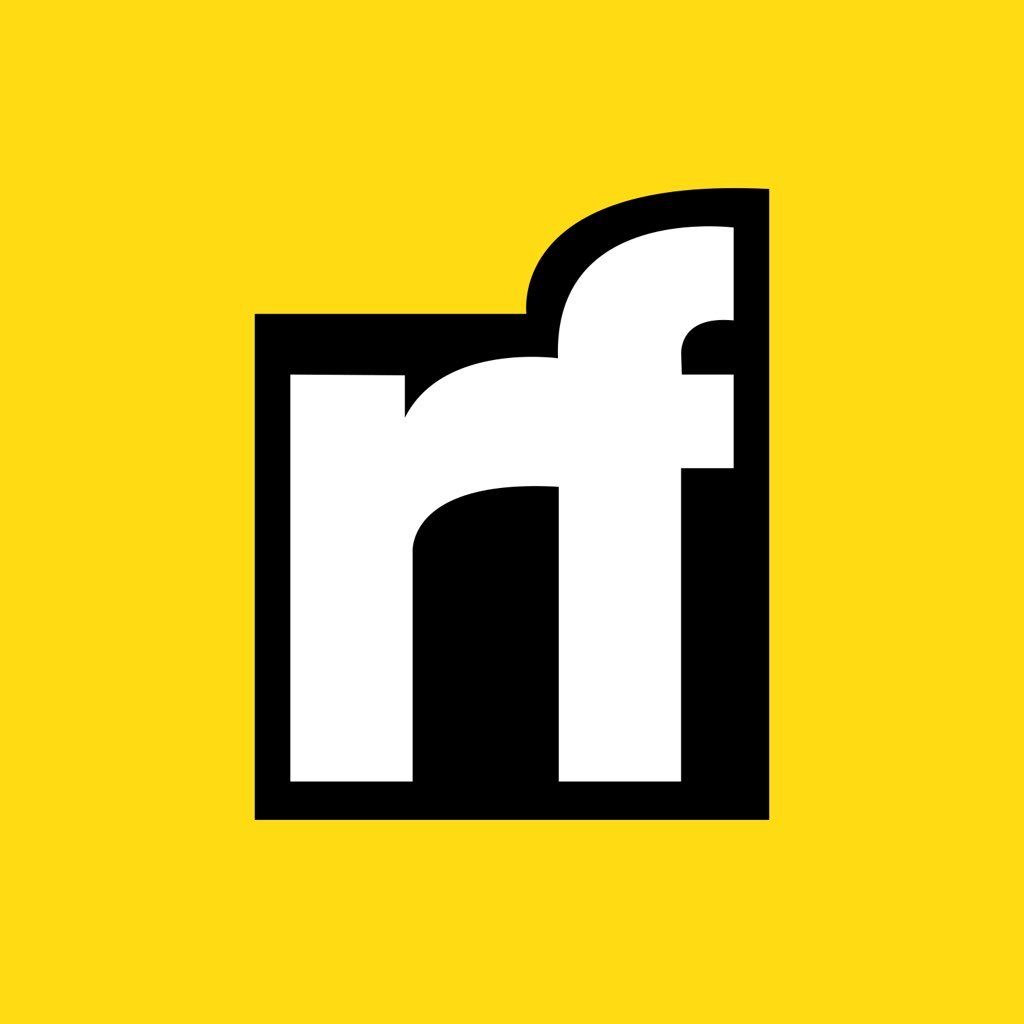
Fernando Doglio | Sciencx (2023-01-04T07:31:39+00:00) Unleash the Power of Server-Side Rendering with React Server Components and Next.js 13. Retrieved from https://www.scien.cx/2023/01/04/unleash-the-power-of-server-side-rendering-with-react-server-components-and-next-js-13/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.