This content originally appeared on Level Up Coding - Medium and was authored by Nic Chong
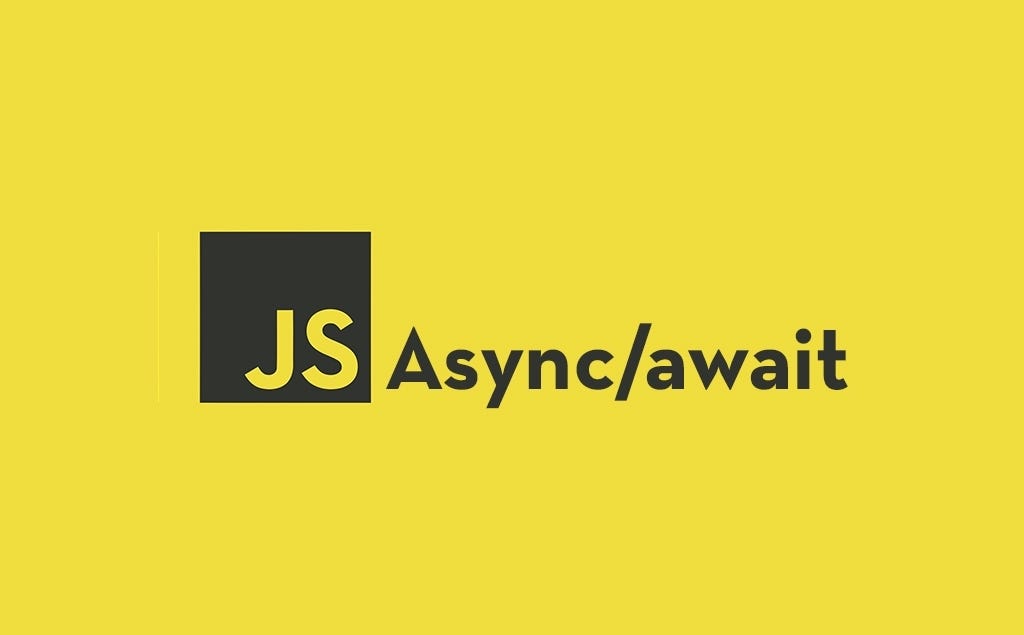
Introduction
In this blog post, we’re going to be talking about async/await — a powerful tool for writing asynchronous code in a variety of programming languages.
But first, let’s define async/await. Simply put, async/await is a way to write asynchronous code that looks and behaves like synchronous code. It allows you to pause the execution of a function, wait for a promise to resolve, and then pick up where you left off.
Here’s a quick example in JavaScript:
async function getData() {
const response = await fetch('https://example.com/data');
const data = await response.json();
return data;
}
Notice how the fetch and response.json calls are wrapped in await statements. This allows the function to pause and wait for the promise to resolve before moving on to the next line.
Why async/await is important
Asynchronous programming is a must in today’s world of high-concurrency applications. Whether you’re building a web app, a mobile app, or a backend service, chances are you’ll need to make use of async/await at some point.
- Improved performance and scalability
Async/await allows you to write non-blocking code, which means your application can handle more concurrent requests without running into performance bottlenecks.
- Enhanced user experience
Async/await can help you create more responsive and interactive applications, leading to a better user experience.
- Better code structure and readability
Async/await makes it easier to write clean and organized code, which is especially important as your application grows in size and complexity.
- Increased productivity
By simplifying asynchronous programming, async/await can help you get more done in less time.
Tip #1 Use async/await for all asynchronous code
There are a few reasons why it’s a good idea to use async/await for all asynchronous code. First, it promotes consistency in your codebase. By using async/await for all asynchronous code, you’ll have a consistent way of writing and organizing your code. This makes it easier for other developers to understand and maintain your codebase.
Async/await also makes it easy to catch and handle errors using try/catch blocks. This is especially useful when dealing with promises, which can be difficult to debug without proper error handling.
Finally, using async/await can lead to improved performance in some cases, especially when combined with the await keyword.
Here are a few examples of how to use async/await for all asynchronous code:
// Example 1: Using async/await with promises
async function getData() {
try {
const response = await fetch('https://example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error(error);
}
}
// Example 2: Using async/await with async/await functions
async function processData(data) {
try {
const processedData = await transformData(data);
await saveData(processedData);
} catch (error) {
console.error(error);
}
}
Tip #2 Use await inside a try/catch block to handle errors
Handling errors is an important part of writing reliable and maintainable code. When using async/await, it’s a good idea to use the await keyword inside a try/catch block to properly handle errors.
Here’s an example of how to use await inside a try/catch block:
async function getData() {
try {
const response = await fetch('https://example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error(error);
}
}
In this example, the fetch and response.json calls are wrapped in an await statement inside a try block. If an error occurs, it will be caught by the catch block and logged to the console.
Using await inside a try/catch block is a simple and effective way to handle errors in async/await code.
Tip #3 Avoid using .then() and .catch() with async/await
The .then() and .catch() methods are commonly used to handle promises in JavaScript. However, when using async/await, it's generally a good idea to avoid using .then() and .catch() in favor of try/catch blocks.
Here’s an example of how to use try/catch blocks instead of .then() and .catch():
async function getData() {
try {
const response = await fetch('https://example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error(error);
}
}
Tip #4 Avoid async void functions, except for event handlers
Async void functions are functions that are marked with the async keyword, but do not return a value. While it's possible to use async void functions in certain situations, they should generally be avoided.
Here’s an example of an async void function:
async void DoWork() {
// Async code here
}
Async void functions can be problematic because they don’t return a value, which makes it difficult to handle errors and determine when the function has completed.
Instead of using async void functions, it’s generally a better idea to use async Task or async Task<T> functions. These types of functions allow you to return a value and handle errors more easily.
Here’s an example of an async Task function:
async Task DoWork() {
// Async code here
}
There is one situation where it’s okay to use async void functions: event handlers. Async void event handlers are commonly used in UI programming to perform asynchronous operations without blocking the UI thread.
Here’s an example of an async void event handler:
private async void Button_Click(object sender, RoutedEventArgs e) {
// Async code here
}
Tip #5 Don’t mix sync and async code without proper consideration
Mixing sync and async code can lead to a number of problems, including performance issues, deadlocks, and race conditions. It’s generally a good idea to avoid mixing sync and async code unless you have a good reason to do so.
There are a few situations where it’s okay to mix sync and async code:
- When you need to call an async method from a sync method: In this case, you can use the await keyword to pause the sync method and wait for the async method to complete.
- When you need to call a sync method from an async method: In this case, you can use the Task.Run() method to execute the sync method on a separate thread.
Here’s an example of calling an async method from a sync method:
public void DoWork() {
// Sync code here
await DoAsyncWork();
// Sync code here
}
public async Task DoAsyncWork() {
// Async code here
}
And here’s an example of calling a sync method from an async method:
public async Task DoAsyncWork() {
// Async code here
await Task.Run(() => DoSyncWork());
// Async code here
}
public void DoSyncWork() {
// Sync code here
}
It’s generally a good idea to avoid mixing sync and async code unless you have a specific reason to do so. Mixing sync and async code can lead to performance issues, deadlocks, and race conditions. However, there are situations where it’s necessary to mix sync and async code, such as calling an async method from a sync method or calling a sync method from an async method. When mixing sync and async code, it’s important to properly handle errors and consider the potential impacts on performance and maintainability.
Don’t Miss my upcoming content and tech guides:
Get an email whenever Nic Chong publishes.
If you have any questions, I am here to help, waiting for you in the comments section :)
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
5 async/await best practices was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Nic Chong
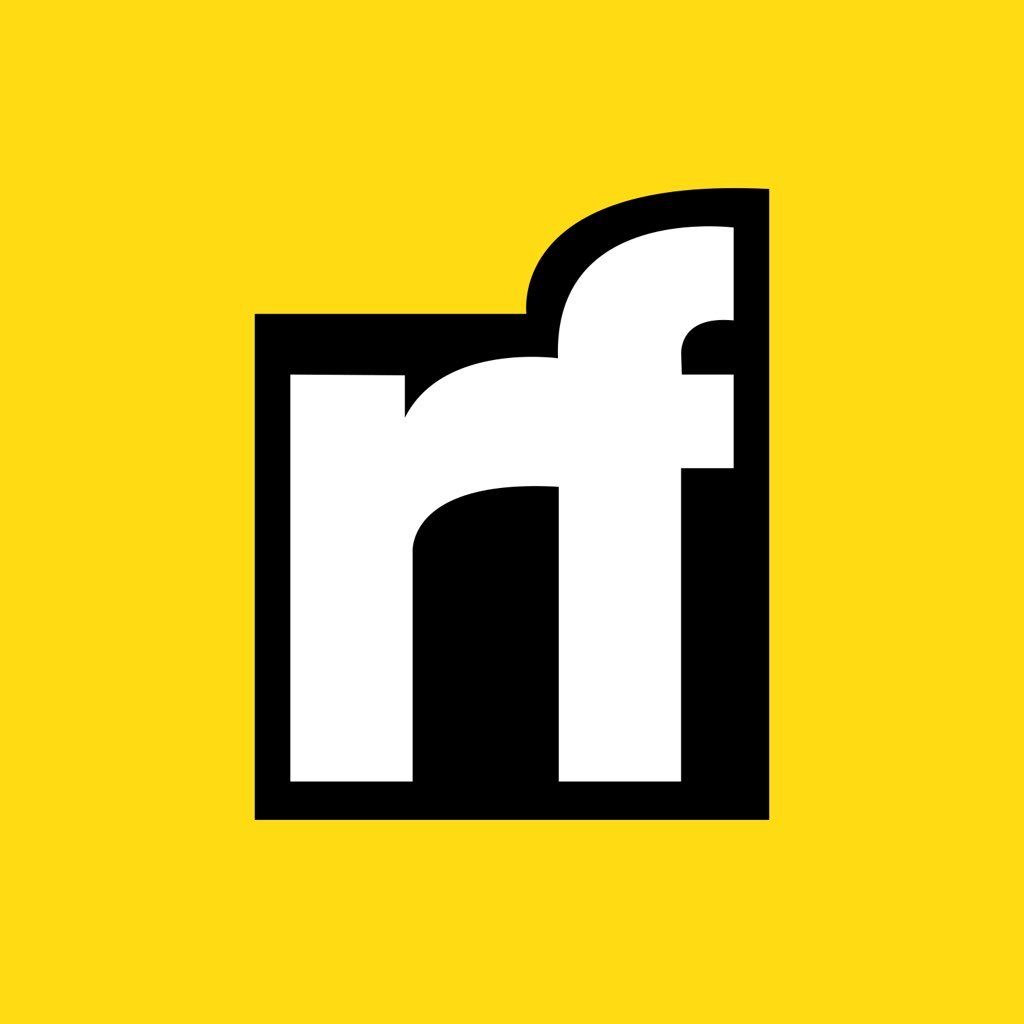
Nic Chong | Sciencx (2023-01-08T20:15:34+00:00) 5 async/await best practices. Retrieved from https://www.scien.cx/2023/01/08/5-async-await-best-practices/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.