This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Hardique Dasore
The delete keyword is used to remove a property from an object in JavaScript. When a property is deleted, it cannot be accessed or re-added to the object. The syntax for using the delete keyword to remove a property is as follows:
delete objectName.propertyName;
For example:
const myObject = { a: 1, b: 2 };
// Removing the 'a' property from the object
delete myObject.a;
console.log(myObject); // Output: { b: 2 }
Using delete on variable will remove variable from memory as well, so be careful and make sure that variable is not being referenced anywhere else before deletion.
Deleting property in JavaScript using destructuring
Using destructuring, you can easily create a new object without the property you want to remove. Destructuring allows you to extract properties from an object and assign them to variables. When you destructure an object, you can specify which properties you want to extract and which properties you want to ignore.
Here's an example of how you can use destructuring to create a new object without a specific property:
const myObject = { a: 1, b: 2, c: 3 };
// Using destructuring to create a new object without the 'b' property
const { b, ...updatedObject } = myObject;
In this example, the properties of myObject that are not b is assigned to updatedObject variable. This b variable will contain the value of b property from myObject. This will not mutate the original object myObject
.
You can also use destructuring in a function argument
function myFunction({ a, c }) {
// The 'b' property is not destructured and is therefore not accessible within this function
console.log(a,c)
}
myFunction(myObject);
In this way you can filter out the specific properties that you don't want and also you can use destructuring in function argument to only extract the specific properties you want.
Happy Coding!
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Hardique Dasore
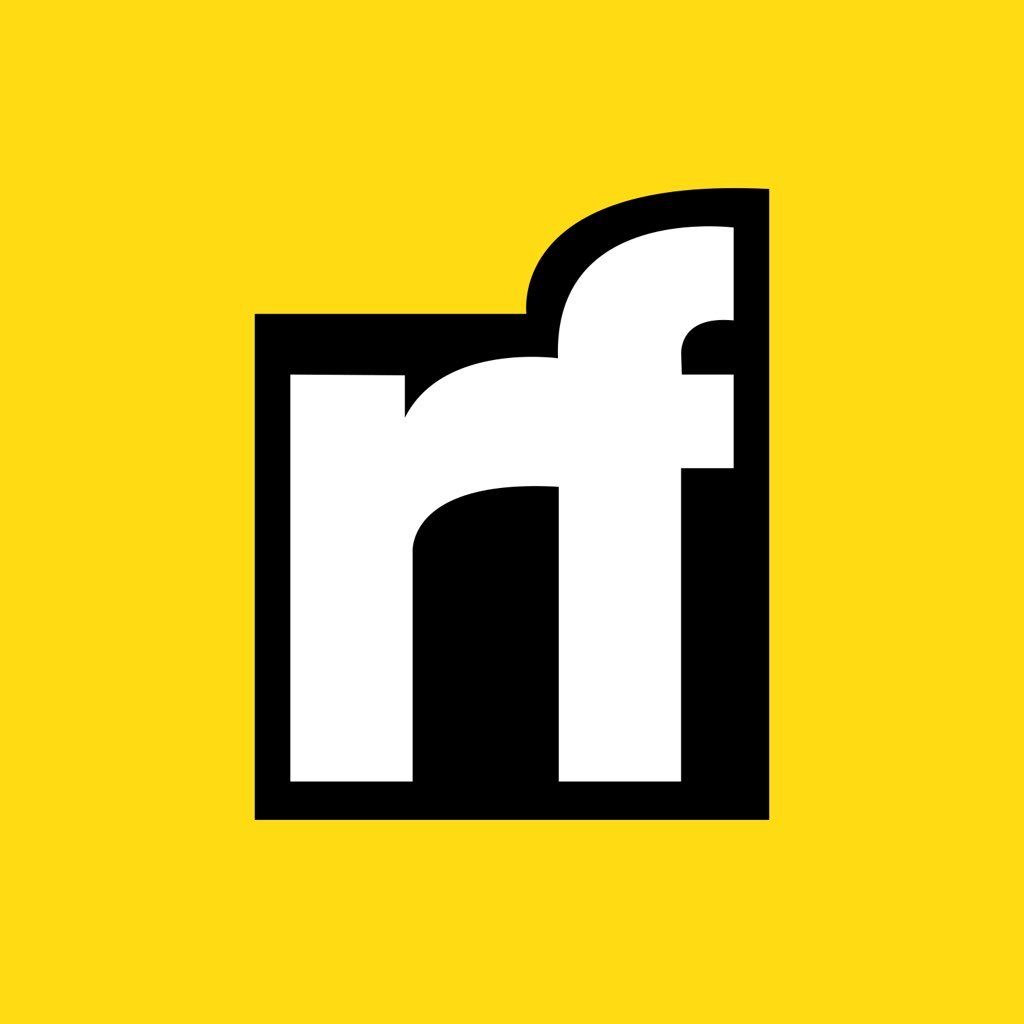
Hardique Dasore | Sciencx (2023-01-11T17:53:09+00:00) Avoid “delete” keyword in JavaScript to remove property. Retrieved from https://www.scien.cx/2023/01/11/avoid-delete-keyword-in-javascript-to-remove-property/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.