This content originally appeared on Bits and Pieces - Medium and was authored by Nathan Byers | Sr. Software Developer
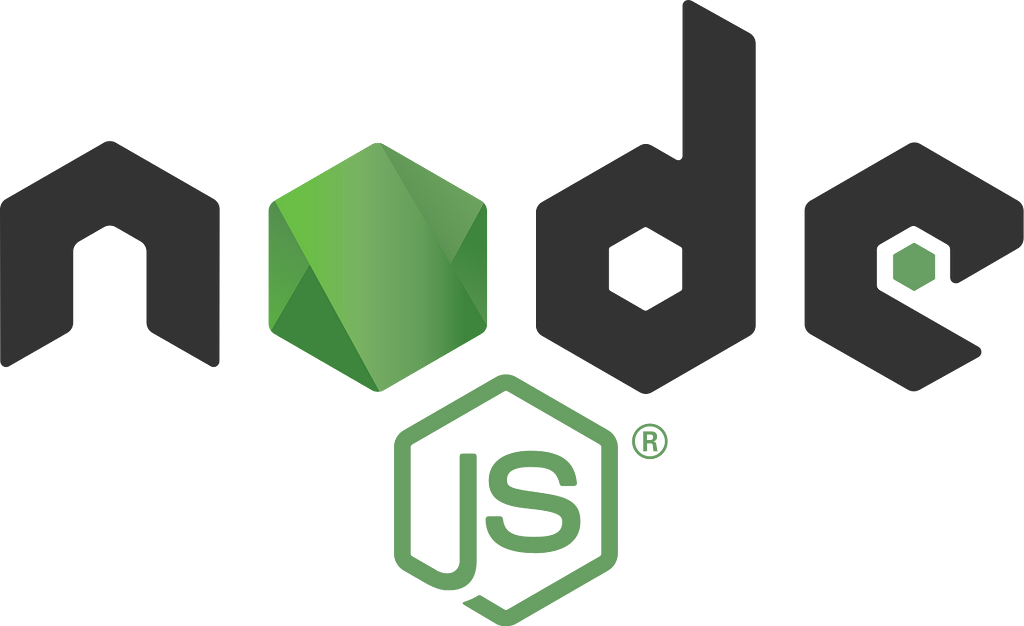
Microservices are a popular architectural style for building scalable, resilient, and maintainable applications. In this tutorial, we will learn how to create microservices using Node.js and the Express framework.
Prerequisites:
- Basic knowledge of Node.js and JavaScript
- Node.js and npm installed on your machine
Step 1: Set up the project
First, create a new directory for your project and navigate to it. Then, run the following command to initialize a new Node.js project:
npm init -y
This will create a package.json file for your project.
Next, install the Express framework and the nodemon development server by running the following command:
npm install --save express nodemon
Step 2: Create the microservice
Next, create a new file called index.js and add the following code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Microservice listening on port 3000');
});
This code creates a new Express application and sets up a simple route that responds with “Hello, World!” when a GET request is made to the root path. It then starts the server on port 3000.
Step 3: Test the microservice
Now, we can test our microservice by running the following command:
nodemon index.js
This will start the nodemon development server, which will automatically restart the server whenever you make changes to the code.
In your browser, visit http://localhost:3000 to see the “Hello, World!” message.
Step 4: Create additional routes
Next, let’s create additional routes for our microservice. Add the following code to your index.js file:
app.get('/users', (req, res) => {
res.send([
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' },
]);
});
app.get('/users/:id', (req, res) => {
const id = req.params.id;
res.send({ id, name: 'John' });
});
These routes simulate a simple API for a list of users. The /users route returns a list of users, and the /users/:id route returns a single user with the specified ID.
Step 5: Test the routes
Now, we can test our new routes by visiting http://localhost:3000/users and http://localhost:3000/users/1 in the browser.
You should see the JSON responses for the list of users and the individual user, respectively.
Step 6: Add error handling
It’s important to add error handling to your microservice to handle unexpected errors and provide helpful error messages to the client.
Add the following code to your index.js file:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send({ error: 'Something went wrong!' });
});
This code sets up a middleware function that catches any errors that occur in the application and sends a generic error message to the client.
Conclusion
In this tutorial, we learned how to create microservices using Node.js and the Express framework.
We set up a simple Express application and added additional routes to simulate an API.
We also added error handling to handle unexpected errors.
I hope you found this tutorial helpful! Let me know if you have any questions or suggestions in the comments below.
Bonus: Learn how to build microservices with reusable components, just like Lego
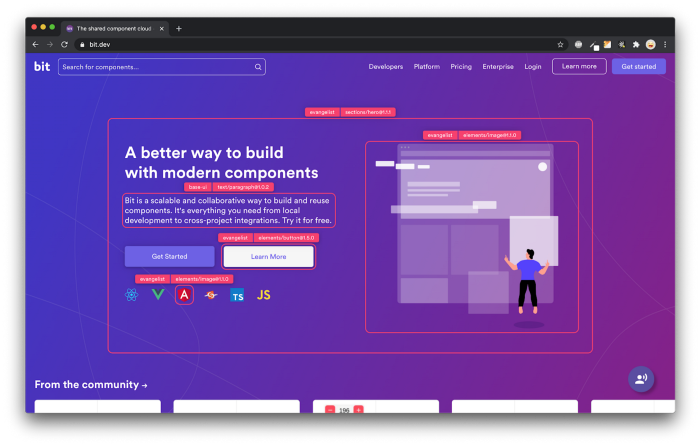
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
- Sharing JavaScript Utility Functions Across Projects
Creating Microservices with Node.js and Express was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Nathan Byers | Sr. Software Developer
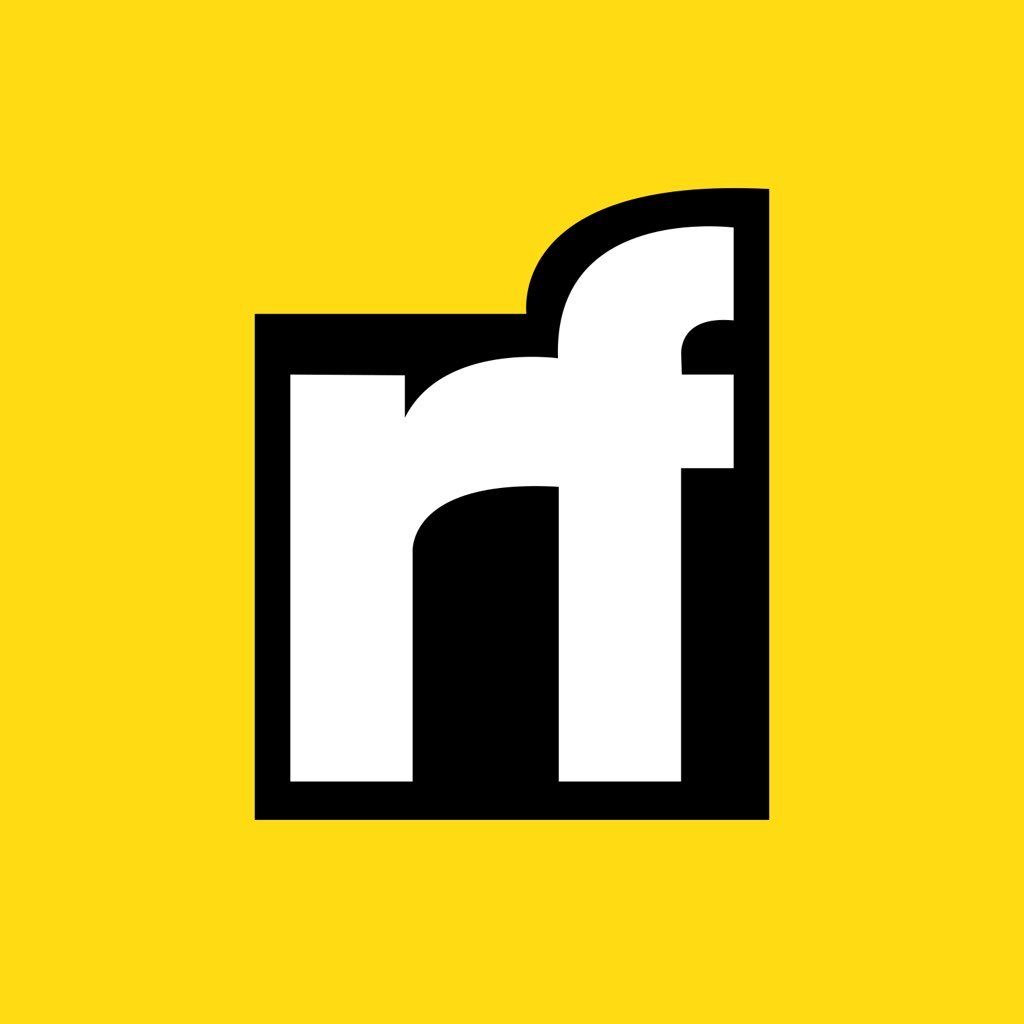
Nathan Byers | Sr. Software Developer | Sciencx (2023-01-24T11:05:16+00:00) Creating Microservices with Node.js and Express. Retrieved from https://www.scien.cx/2023/01/24/creating-microservices-with-node-js-and-express/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.