This content originally appeared on Level Up Coding - Medium and was authored by D Varghese
Usage of Python map and reduce
Test your Python skills to use map and reduce functions to compute length of words in a sentence and aggregate it to find the total length
This article showcases the usage of Python map and reduce functions. This is done by showing how map and reduce could be used to compute the average length of words in a sentence.
The sentence used in the program is “I see the cat and mouse!”.
The sentence has 6 words. The total length of the words is 18 excluding the exclamation — I(1) see(3) the(3) cat(3) and(3) mouse!(5). So the average length should be computed as 3 i.e. 18/6. Let’s see how its getting done.
The program begins here by entering the sentence.
sentence = 'I see the cat and mouse!'
print('The sentence is \'{}\' and object type is {}'.format(sentence, type(sentence))
The sentence is 'I see the cat and mouse!' and object type is <class 'str'>
Next, the special characters like ! are removed from the sentence and words stored in a list.
import re
words = re.split('\s', re.sub('[\.\?!,:;\'\-\{\}\[\]\(\)]{1}', '', sentence))
print('The words in the sentence are {} and object type is {}'.format(words, type(words)))
The words in the sentence are ['I', 'see', 'the', 'cat', 'and', 'mouse'] and object type is <class 'list'>
Next, use the map function to compute the word lengths, and then store the output in a list
word_lens = list(map(lambda word: len(word), words))
print('The word lengths are {}'.format(word_lens))
The word lengths are [1, 3, 3, 3, 3, 5]
Next, use the reduce function to sum the word lengths
import functools
tot_len = functools.reduce(lambda a, b: a + b, word_lens)
print('The total length is {}'.format(tot_len))
The total length is 18
Alternatively, the above map and reduce can be invoked with one line of code
tot_len = functools.reduce(lambda a, b: a + b, list(map(lambda word: len(word), words)))
print('The total length is {}'.format(tot_len))
The total length is 18
Finally, the average length of the words in the sentence is computed
print('Average word length is: {}'.format(round(tot_len / len(words)*1.0, 2)))
Average word length is: 3.0
Happy coding!
Average length of words was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by D Varghese
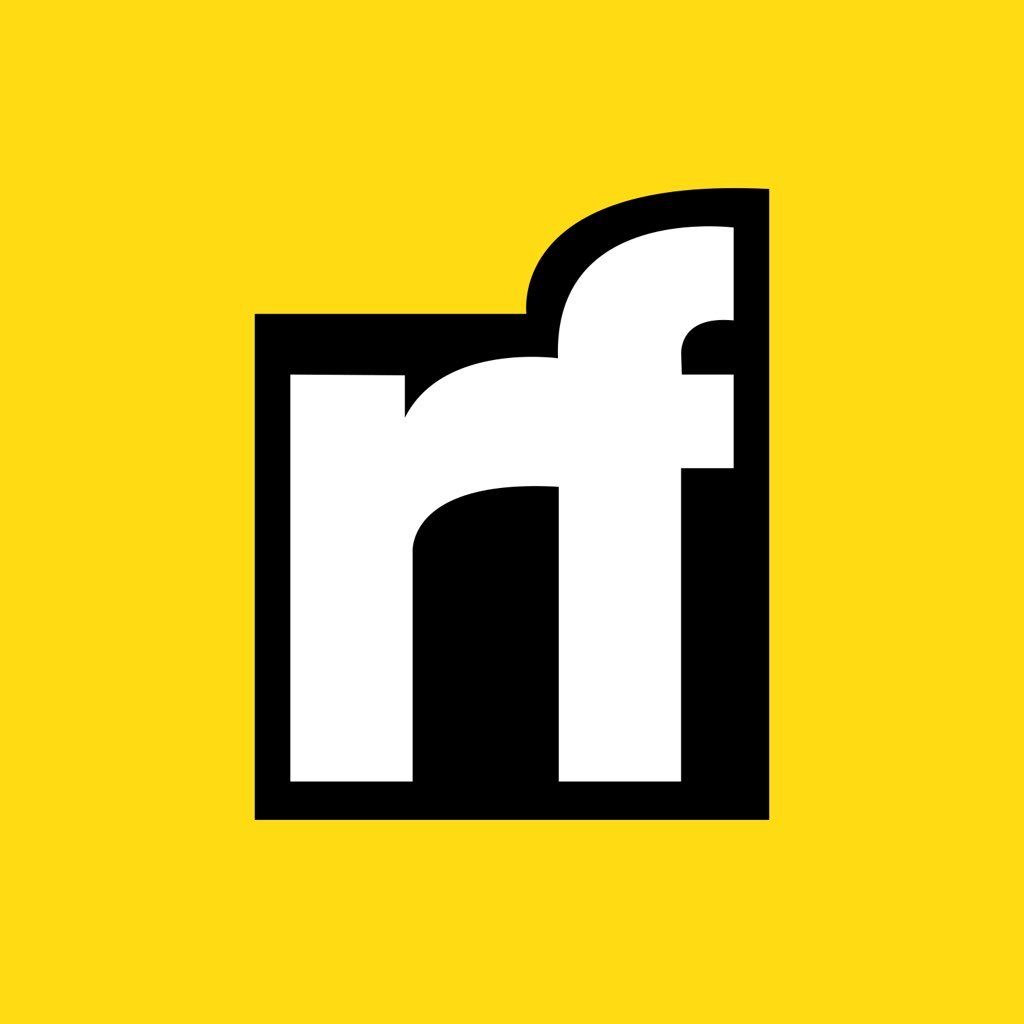
D Varghese | Sciencx (2023-01-26T21:38:32+00:00) Average length of words. Retrieved from https://www.scien.cx/2023/01/26/average-length-of-words/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.