This content originally appeared on Level Up Coding - Medium and was authored by Zayn Korai
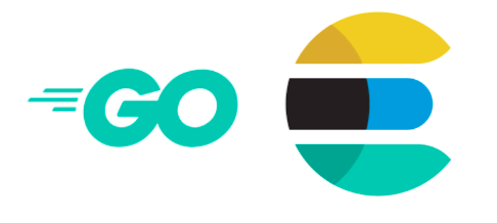
Elasticsearch is a powerful search and analytics engine that is used for storing, searching, and analyzing large volumes of data. It is built on top of the Lucene library and uses a flexible JSON-based query language called Elasticsearch Query DSL. One of the main advantages of Elasticsearch is its ability to handle structured and unstructured data, making it a popular choice for a wide range of use cases such as text search, log analysis, and data visualization.
Golang, also known as Go, is a modern programming language that is known for its simplicity, performance, and scalability. It is often used for building high-performance and concurrent systems, making it a great choice for working with Elasticsearch.
To interact with Elasticsearch from Golang, you can use an official Elasticsearch client library called elastic. This library provides a convenient and powerful API for interacting with Elasticsearch, including the ability to index, search, and update documents, as well as perform more complex operations such as aggregations and geospatial queries.
Here is an example of how to use the elastic library to index a document in Elasticsearch:
package main
import (
"context"
"fmt"
"github.com/olivere/elastic"
)
func main() {
// Connect to Elasticsearch
client, err := elastic.NewClient(elastic.SetURL("http://localhost:9200"))
if err != nil {
panic(err)
}
// Create a new document
doc := struct {
Title string `json:"title"`
Body string `json:"body"`
}{
Title: "My first Elasticsearch document",
Body: "Hello, Elasticsearch!",
}
// Index the document
_, err = client.Index().
Index("myindex").
Type("mytype").
BodyJson(doc).
Do(context.Background())
if err != nil {
panic(err)
}
fmt.Println("Document indexed!")
}
This example shows how to use the elastic library to create a new Elasticsearch client, create a new document, and then index it in Elasticsearch. You can also use this library to perform other operations such as searching and updating documents, as well as more advanced operations like aggregations and geospatial queries.
An advanced example of using Elasticsearch and Golang would be to build a real-time data pipeline that ingests, processes, and analyzes data in near real-time. This could involve the following steps:
- Ingesting data into Elasticsearch: Data can be ingested into Elasticsearch using the bulk API, which allows for indexing and updating multiple documents in a single request. This can be done using the elastic library in Golang, as shown in the previous example.
- Processing data using Elasticsearch: Once the data is indexed in Elasticsearch, it can be processed using the Elasticsearch Query DSL. This allows for powerful search and aggregation operations to be performed on the data, such as filtering and grouping documents by certain fields, calculating statistics and metrics, and more.
- Analyzing data using Kibana: Kibana is a visualization tool that can be used in conjunction with Elasticsearch to create interactive dashboards and charts. It allows for the data to be visualized and analyzed in real-time, providing insight into trends, patterns, and anomalies in the data.
Here is an example of a Go program that ingests JSON data into Elasticsearch, processes it using the Elasticsearch Query DSL, and then visualizes it in Kibana:
package main
import (
"context"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
"time"
"github.com/olivere/elastic"
)
func main() {
// Connect to Elasticsearch
client, err := elastic.NewClient(elastic.SetURL("http://localhost:9200"))
if err != nil {
panic(err)
}
// Ingest data into Elasticsearch
go func() {
for {
resp, err := http.Get("http://your-data-source")
if err != nil {
panic(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
var docs []interface{}
json.Unmarshal(body, &docs)
bulk := client.Bulk()
for _, doc := range docs {
req := elastic.NewBulkIndexRequest().Index("myindex").Type("mytype").Doc(doc)
bulk.Add(req)
}
_, err = bulk.Do(context.Background())
if err != nil {
panic(err)
}
fmt.Println("Data ingested into Elasticsearch!")
time.Sleep(10 * time.Second)
}
}()
// Process data using Elasticsearch
go func() {
for {
// Perform search and aggregation
res, err := client.Search().
Index("myindex").
Query(elastic.NewMatchAllQuery()).
Aggregation("myagg", elastic.NewTermsAggregation().Field("field1")).
Do(context.Background())
if err != nil {
panic(err)
}
fmt.Println("Data processed using Elasticsearch!")
// Visualize data in Kibana
// ...
time.Sleep(60 * time.Second)
}
In conclusion, Elasticsearch and Golang are a great combination for building powerful and efficient search and analytics systems. The elastic library provides a convenient and powerful API for interacting with Elasticsearch, making it easy to work with this powerful search engine from Golang.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Elasticsearch with Golang 101 was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Zayn Korai
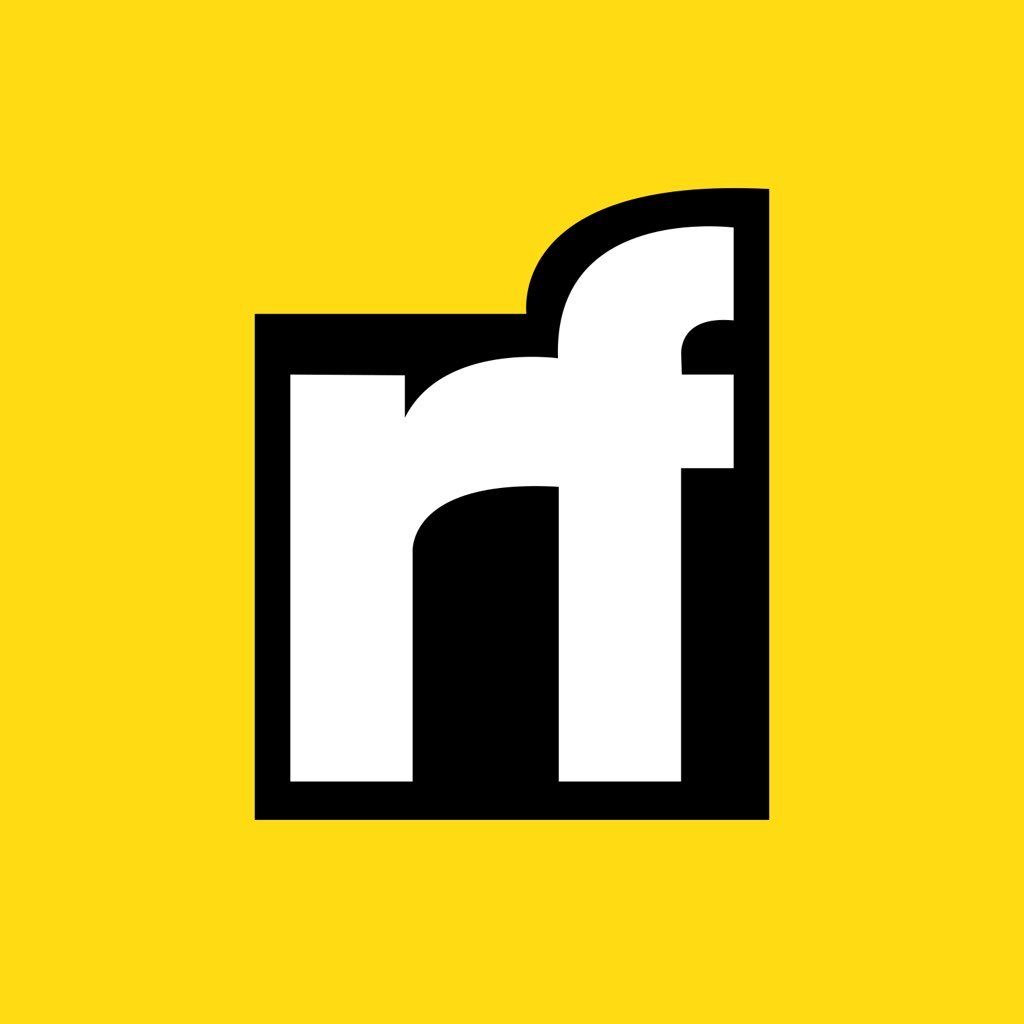
Zayn Korai | Sciencx (2023-01-30T17:21:21+00:00) Elasticsearch with Golang 101. Retrieved from https://www.scien.cx/2023/01/30/elasticsearch-with-golang-101/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.