This content originally appeared on Bits and Pieces - Medium and was authored by Nipuni Arunodi
Is CSS-in-JS Better than CSS?
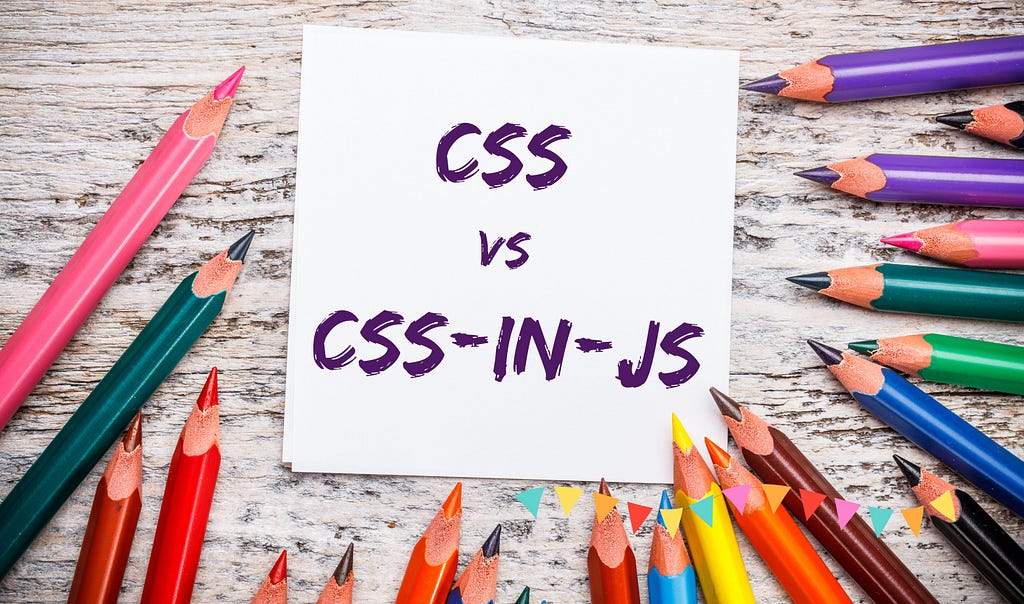
There are various methods for organizing application styles in modern web applications. One choice developers have to make is using CSS or CSS-in-JS to style their applications. Although pure CSS is one of the most common choices, techniques like CSS in JS have become popular among developers drastically. For example, the state of JS annual survey for 2021 found that 52% of developers use Styled Components, a well-known CSS-in-JS library.
So, in this article, I will compare and differentiate CSS and CSS in JS with their pros and cons to help you decide which one is more suitable for your project.
CSS
CSS is one the most popular style sheet language used to add styles to web pages written in markup languages. It makes it easier for developers to customize elements like colors, layout, font style, paragraph spacing, column size, text styles, and more.
There are 3 ways to include CSS in your web pages:
- Inline — Using the style attribute.
- Internal — Using the <style> tag.
- External — Using <link> tag to link external CSS files.
In general, writing CSS styles is pretty straightforward. You can use the element, class, or id to apply styles to HTML elements. For example, the below style changes the color and the font family of all the paragraphs in the application.
p {
font-family: verdana;
color: red;
}
Although CSS offers many benefits, it’s not a perfect solution. So, it is essential to understand its benefits and challenges.
Pros of CSS
Easy to use
With CSS, it’s now easier to maintain consistent styles throughout a web application. Earlier, there was no way to maintain or update styles through the application at once. With CSS, developers can easily use class names to update styles in multiple places at once. It also ensures that the style elements are consistent across multiple web pages.
Preprocessor Support
Preprocessors such as SASS and LESS enable developers to use variables and modularize their code for styles, making it easier to reuse code and maintain consistency in their projects.
High Performance
CSS allows developers to maintain styles for many web pages with few lines. This reduces the number of code lines and files that need to be loaded and improves the page rendering speed.
SEO Support
Using CSS can help reduce the HTML code in a web page, making it more organized and easier to rank in search indexes. This can be beneficial for search engine optimization because search engine spiders prefer clean, well-organized HTML code when ranking pages in search results.
Device Compatibility
Today, users use multiple devices to access web applications. Hence, it is important to design applications to support any device’s specifications. In that case, CSS plays a major role by helping developers to design responsive designs. Ultimately, it increases the user experience and attracts more users to your application.
Cons of CSS
Overriding Issues
The biggest issue with the CSS is style overriding. as the applications scale, developers are forced to use inline styles or !important syntax to prevent style overriding.
Several CSS levels
There are different versions of CSS, from CSS1 to CSS3, which has caused confusion among web browsers and developers. Therefore, you should only use a single CSS level in a project.
Browser Support
Some styles that work well with one browser might not work with another. Hence, web developers must verify style compatibility by running the application in several browsers.
Also, Different web browsers interpret CSS data in various ways. If users use an outdated version, users will see the website as less appealing and negatively impact user experience.
Naming Issues
CSS class naming issues often happen as your application becomes complex. You may end up creating conflicting or even duplicate classes that can make it difficult to maintain and debug issues. Therefore, it is advised to follow a standard naming convention like BEM to help created well defined CSS classes in your application
CSS-in-JS
CSS in JS is also a pretty straightforward concept. It simply allows you to apply styles to your components using CSS props via JavaScript. The main purpose of introducing CSS in JS was to handle the style overriding issues in CSS, and you can apply CSS in JS to your components as inline styles or use third-party libraries like Styled Components.
Using inline styles
const MyComponent = props => (
<p style={{fontFamily: 'verdana', color: 'red'}}>My Component</p>
)
Using Styles Components
const StyledParagraph = styled.div`
font-family: verdana;
color: red;
`
const MyComponent = props => (
<StyledParagraph {...props}> My Component </StyledParagraph>
)
Although CSS is JS resolves the style overriding issue, it also comes with some challenges. So, let’s see the pros and cons of using CSS in JS.
Pros of CSS in JS
No Naming and Overriding Issues
As mentioned, you do not need to think about naming, or overriding issues since the styles you use are scoped to your component.
Functional flexibility
Since CSS-in-JS is essentially JavaScript code, you can use extensive logic for your style rules, such as loops, conditionals, variables, state-based styling, etc. Hence, it is a perfect choice for complex user interfaces that needs dynamic functionality.
Automatically Generates Browser-Specific Extensions
Most CSS in JS libraries provide inbuilt features to generate browser-specific style extensions like -webkit, and -moz.
Portability
You can move components around safely because they come with all the logic and styles. They function right out of the box and enable you to build programs with loosely linked components. In addition, you can easily remove styles from a component since they do not affect others.
Cons of CSS-in-JS
Learning Curve
There is a learning curve for CSS-in-JS, especially if you have never used web components or component-based frameworks. You must adopt a fresh thinking style in addition to the new syntax, which will take time and may slow down your development process.
No CSS Preprocessor Support
Unlike pure CSS, you can’t use preprocessors like SASS and LESS with CSS in JS.
Performance Issues
Using CSS in JS can increase the application rendering time since CSS in JS requires JavaScript to parse the CSS and inject them into the DOM.
Caching Issues
Can’t utilize CSS caching since there are no separate CSS files maintained.
Different CSS in JS Libraries
- Styled Components — Styled Components is the most popular CSS-in-JS library available. It utilizes ES6 template literals to specify the CSS styles of your components. It has more than 3M weekly NPM downloads and 38K+ GitHub stars. Learn how to use Styled Components with React here.
- JSS — JSS is a CSS-in-JS package independent of frameworks. It allows you to generate CSS styles on-the-fly based on the state of your components. It has more than 1.5M weekly NPM downloads and 6.9K+ GitHub stars.
- Emotion — Emotion is another CSS-in-JS library independent of frameworks. It has features like source maps, a cache provider, and snapshot tests. You can create Emotion scripts using JavaScript objects or ES6 template literals. It has more than 411K weekly NPM downloads and 15.9K+ GitHub stars.
- Radium — Radium inserts inline styles directly into the HTML rather than creating a unique class name for each component. As a result, the style global attribute is used to style each HTML element directly. Radium has more than 43K weekly NPM downloads and 7.4K+ GitHub stars.
- Styletron — Styletron is a famous CSS-in-JS library that allows you to specify CSS styles using JavaScript objects regardless of the framework used. Styletron is compatible with React, standard JavaScript, and other frameworks. Styletron-styled components are transferable across different rendering engines.
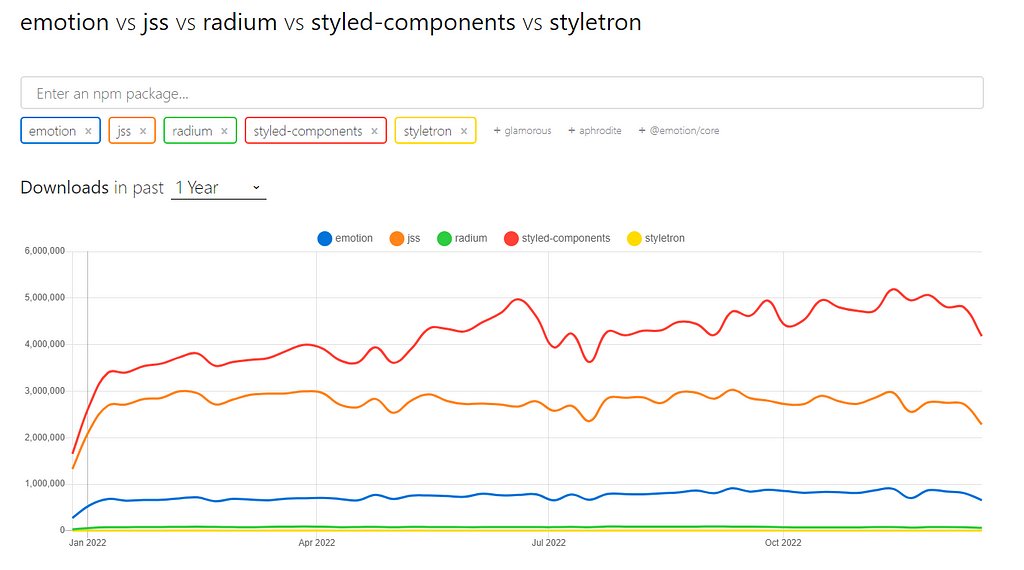
Source: https://npmtrends.com/emotion-vs-jss-vs-radium-vs-styled-components-vs-styletron
When to use What?
Based on what we discussed above, there is no doubt that both CSS and CSS-in-JS are great styling techniques. However, there can be scenarios where one is more suitable than the other.
Using CSS is a good choice if you have complex UIs and a performance-critical application. Traditional CSS removes a significant amount of code lines from JavaScript files and improves initial page loading time.
Also, it has been battle tested for many years, and most organizations believe that they can get the most out of techniques like CSS preprocessors and CSS modules. Furthermore, the learning curve of pure CSS is much simpler, and any new developer can quickly grasp it.
Overall, suppose you have an application that focuses on performance and does not require a design system. In that case, you can easily go with pure CSS or any associated techniques, like CSS modules.
On the other hand, the CSS-in-JS method is suitable when working with applications where performance is less important or for applications that require design systems.
However, you will have to put a lot of extra effort into maintaining the styling system since it can rapidly become complex as a program expands.
Conclusion
This article outlined CSS and CSS-in-JS with their pros and cons. Both CSS and CSS-in-JS are great for different use cases. Each has unique features, and you must choose one based on your requirements. Whatever approach you choose, you can use Bit to create highly adaptable and maintainable components that you can use across your entire organization to ensure consistency.
I hope you now have a better understanding of CSS and CSS-in-JS to select the best one for your project.
Build apps with reusable components like Lego
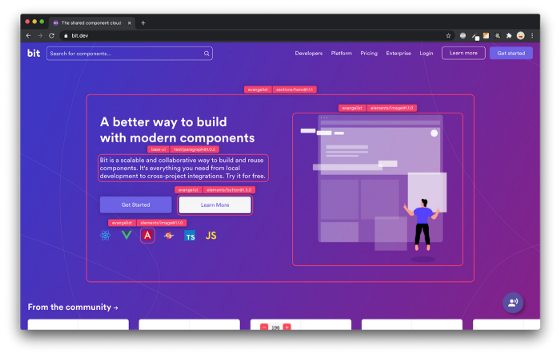
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn More
- How We Build Micro Frontends
- Theming React Apps with Styled Components and Bit
- How we Build a Component Design System
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
CSS vs. CSS-in-JS: What You Should Choose in 2023 was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Nipuni Arunodi
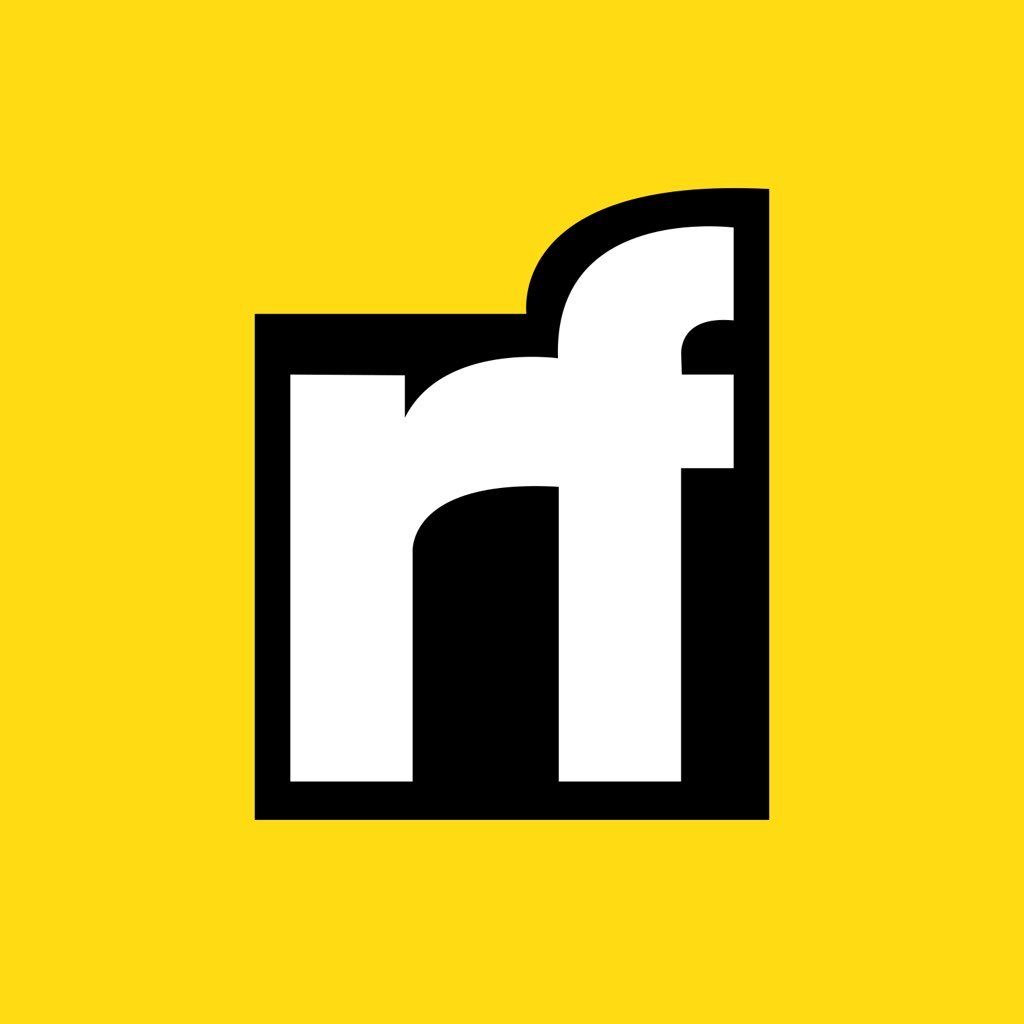
Nipuni Arunodi | Sciencx (2023-02-03T07:02:30+00:00) CSS vs. CSS-in-JS: What You Should Choose in 2023. Retrieved from https://www.scien.cx/2023/02/03/css-vs-css-in-js-what-you-should-choose-in-2023/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.