This content originally appeared on Level Up Coding - Medium and was authored by Amit Kumar Manjhi
What is Function?
- Functions are generally the block of codes or statements in a program that gives the user the ability to reuse the same code which ultimately saves the excessive use of memory, acts as a time saver and more importantly, provides better readability of the code.
Function Declaration
func function_name(Parameter-list)(Return_type){ // function body..... }
The declaration of the function contains:
- func: It is a keyword in Go language, which is used to create a function.
- function_name: It is the name of the function.
- Parameter-list: It contains the name and the type of the function parameters.
- Return_type: It is optional and it contains the types of the values that the function returns.
Function Parameters
- Without return statement
// Program to illustrate function parameters
package main
import "fmt"
// define a function with 2 parameters
func addNumbers(n1 int, n2 int) {
sum := n1 + n2
fmt.Println("Sum:", sum)
}
func main() {
// pass parameters in function call
addNumbers(21, 13)
}
- With return statement
package main
import "fmt"
func main() {
fmt.Println("Welcome to Functions in GoLang")
greeter()
result := adder(5,3)
fmt.Println("sum of two number is:",result)
proRes:= proadder(2,3,4,0,5,6,7)
fmt.Println("Sum of n number is :",proRes)
}
func adder(val1 int, val2 int)int{
return val1 + val2
}
func proadder(values ...int)int{
total :=0
for _,val := range values{
total += val
}
return total
}
func greeter(){
fmt.Println("Namste from GoLang")
}
Thank you for reading :)
Reference : https://go.dev/tour/basics/4
Functions In GoLang was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Amit Kumar Manjhi
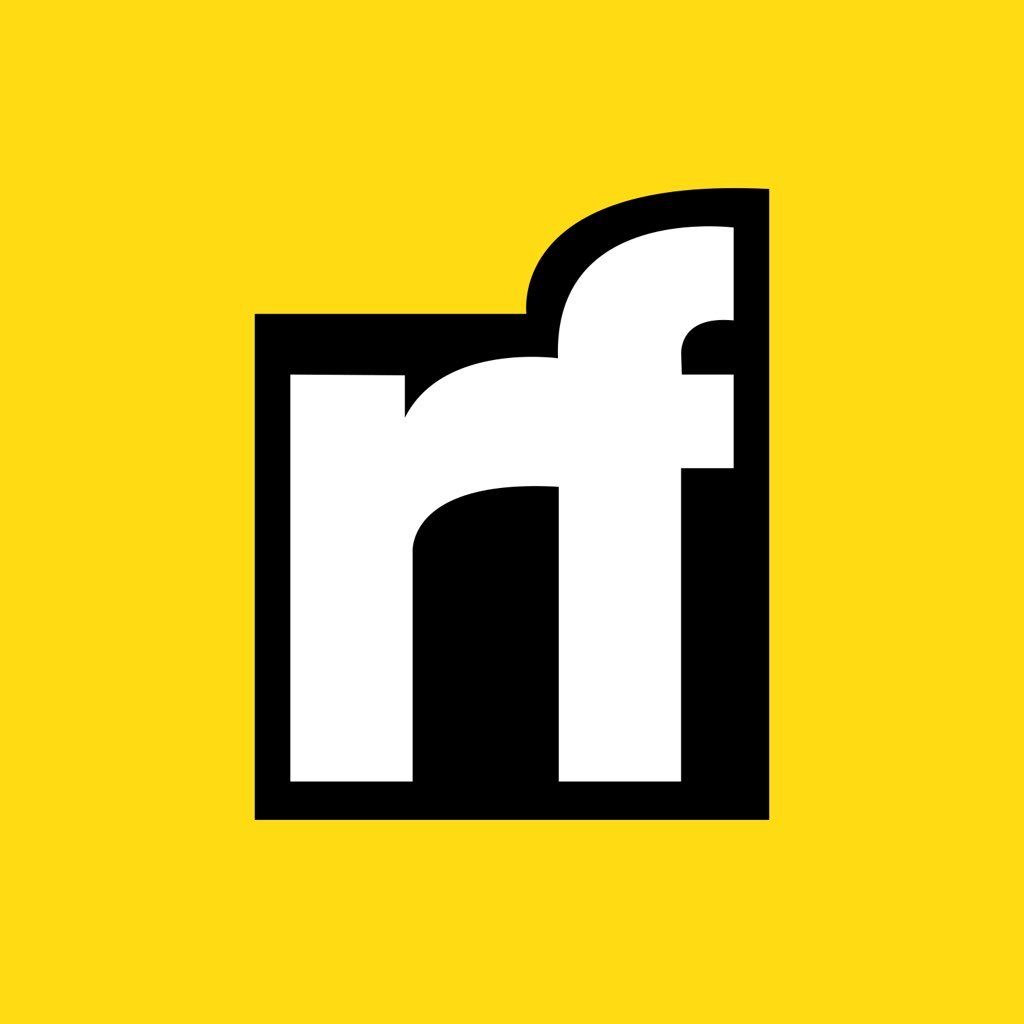
Amit Kumar Manjhi | Sciencx (2023-02-03T16:12:35+00:00) Functions In GoLang . Retrieved from https://www.scien.cx/2023/02/03/functions-in-golang-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.