This content originally appeared on Level Up Coding - Medium and was authored by Amit Kumar Manjhi
What is a Pointer?
- A pointer is a variable that is used to store the memory address of another variable.
Declaration and initialization of Pointers
- * Operator also termed as the dereferencing operator used to declare pointer variable and access the value stored in the address.
- & operator termed as address operator used to returns the address of a variable or to access the address of a variable to a pointer.
Declaring a pointer:
var pointer_name *Data_Type
//Example: var s *string
Initialization of Pointer:
//normal variable declaration
var a = 45
// Initialization of pointer s with memory address of variable a
var s *int = &a
Create Pointers using Golang new()
package main
import "fmt"
func main() {
// create a pointer using new()
var ptr = new(int)
*ptr = 20
fmt.Println(ptr) // 0xc000016058
fmt.Println(*ptr) // 20
}
Nil Pointers in Golang
When we declare a pointer variable but do not initialize it, the value of the pointer is always nil.
package main
import "fmt"
func main() {
// declare a pointer variable
var ptr *int
fmt.Println("Value of pointer:", ptr)
}
Dereferencing the Pointer
As we know that * operator is also termed as the dereferencing operator. It is not only used to declare the pointer variable but also used to access the value stored in the variable which the pointer points to which is generally termed as indirecting or dereferencing.
package main
import "fmt"
func main() {
var y = 500
var p = &y
fmt.Println("Value stored in y = ", y)
fmt.Println("Address of y = ", &y)
fmt.Println("Value stored in pointer variable p = ", p)
fmt.Println("Value stored in y(*p) = ", *p)
}
Output:
Value stored in y = 500
Address of y = 0x414020
Value stored in pointer variable p = 0x414020
Value stored in y(*p) = 500
Thank you for reading :)
Reference : https://go.dev/tour/moretypes/1
Pointers In GoLang was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Amit Kumar Manjhi
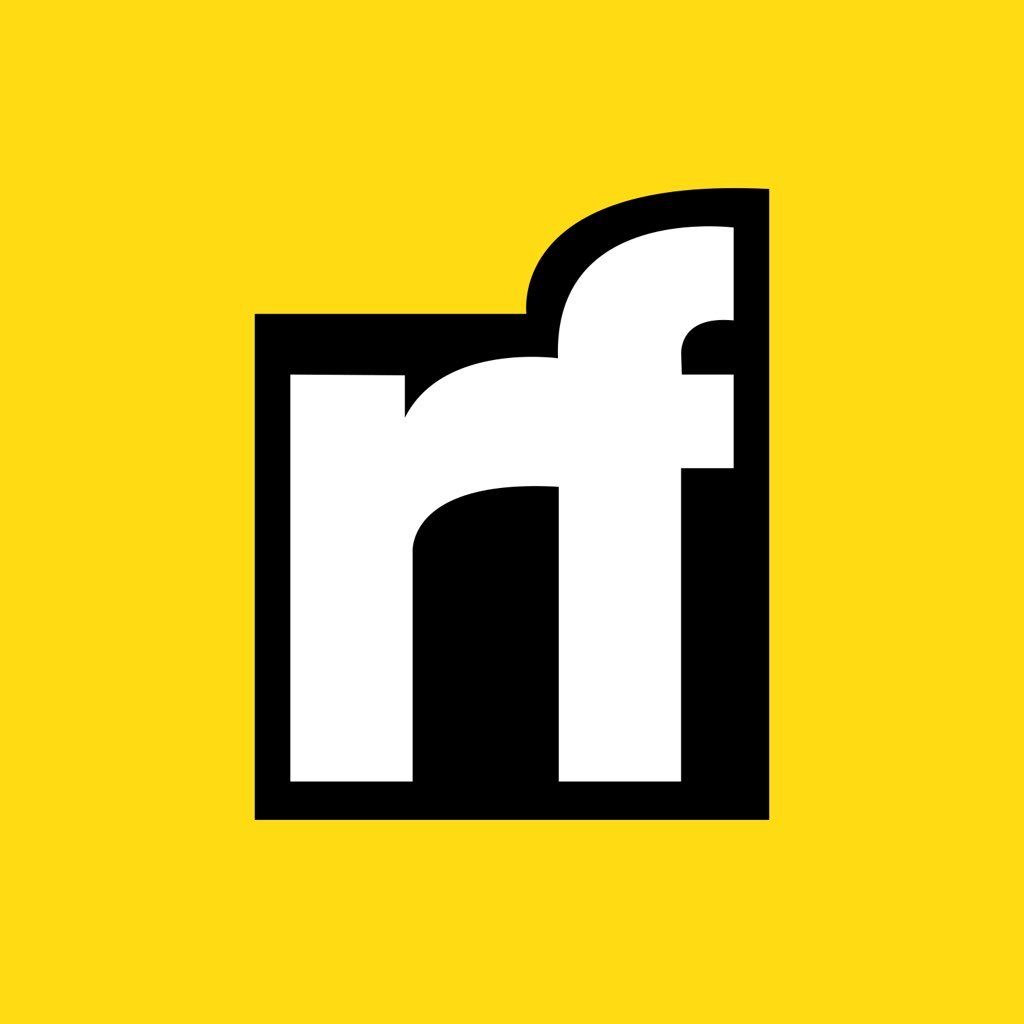
Amit Kumar Manjhi | Sciencx (2023-02-03T16:13:13+00:00) Pointers In GoLang. Retrieved from https://www.scien.cx/2023/02/03/pointers-in-golang-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.