This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Yuya Hirano
TypeScript is a statically typed language, meaning that every variable in TypeScript has a type. It is important to understand these types to write clean, efficient, and error-free code. This article covers the basic and most commonly used data types in TypeScript, as well as a few additional types that are useful to know.
Number: Used to represent numeric values, such as integers or floating-point numbers.
let a: number = 42;
let b: number = 3.14;
String: Used to represent a sequence of characters.
let name: string = "John Doe";
Boolean: Used to represent true or false values.
let isTrue: boolean = true;
let isFalse: boolean = false;
Array: Used to represent a collection of values of the same type.
let numbers: number[] = [1, 2, 3, 4];
let names: string[] = ["John", "Jane", "Jim"];
Tuple: Used to represent a data structure similar to an array with multiple types.
let user: [string, number] = ["John Doe", 32];
Enum: Used to give named identifiers to numbers or strings.
enum Color {Red, Green, Blue};
let c: Color = Color.Green;
Any: Used to represent any TypeScript type.
let value: any = 42;
value = "Hello";
Void: Used to indicate a function that does not return a value.
function sayHello(): void {
console.log("Hello!");
}
Never: Used to indicate an error or termination state.
function throwError(): never {
throw new Error("Something went wrong");
}
These are just a few of the additional TypeScript data types that are available. It's important to understand these types and choose the appropriate one for your specific use case to write efficient and maintainable code.
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Yuya Hirano
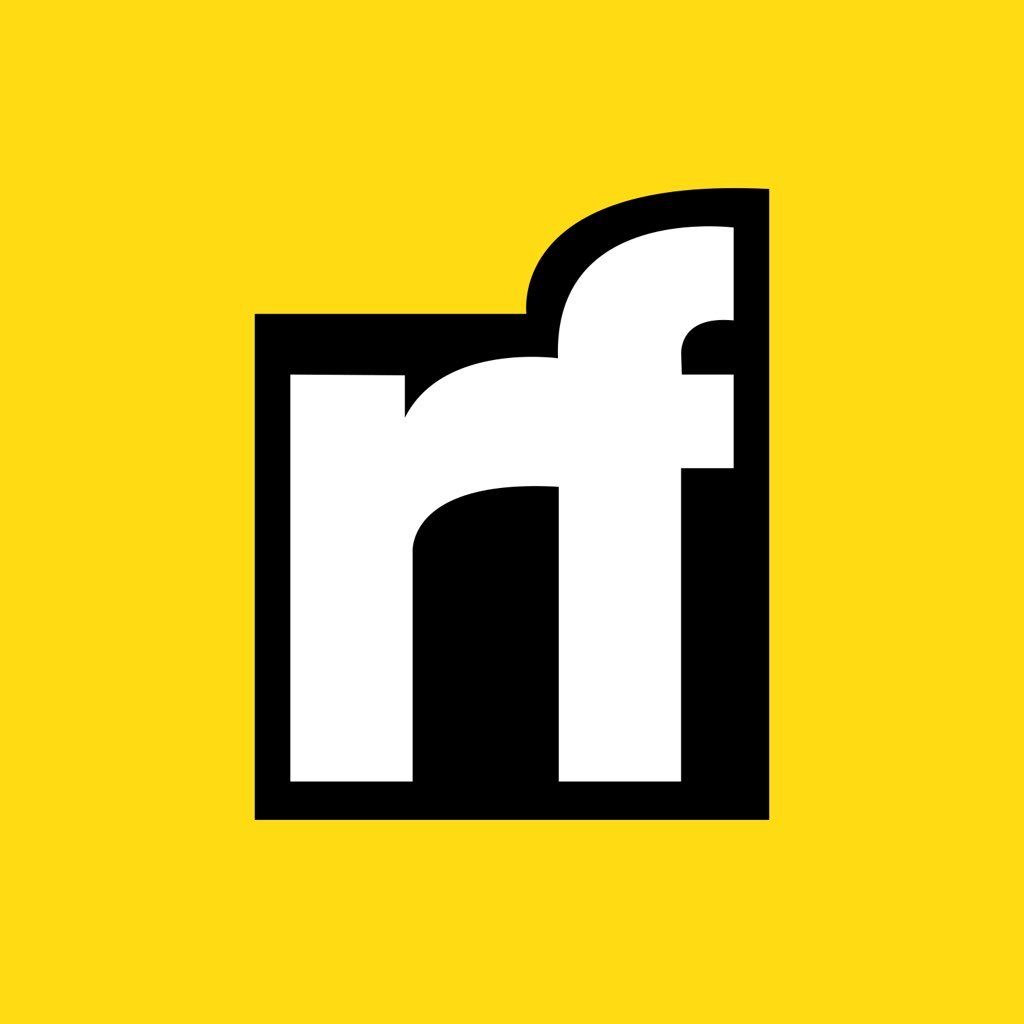
Yuya Hirano | Sciencx (2023-02-06T08:03:29+00:00) Understanding TypeScript Data Types. Retrieved from https://www.scien.cx/2023/02/06/understanding-typescript-data-types/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.