This content originally appeared on Bits and Pieces - Medium and was authored by Farnam Homayounfard
A tutorial on writing unit tests with the React Testing Library in Next.js
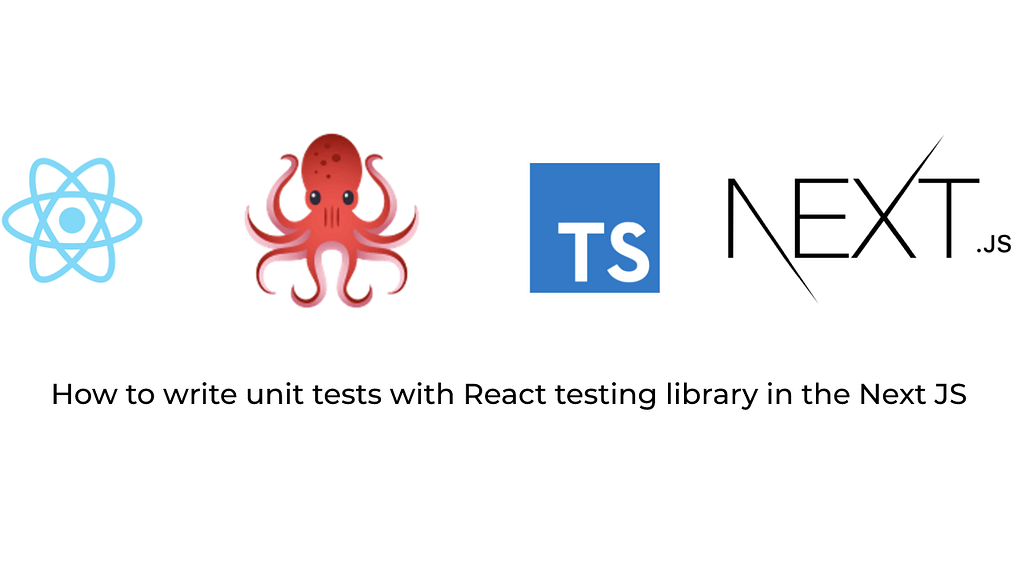
If you want to use the React testing library in Next.js for writing unit tests you have to make some configuration files for that and install some packages.
You can install packages with the below command:
npm install -D jest jest-environment-jsdom @testing-library/jest-dom @testing-library/react @testing-library/user-event
yarn add -D jest jest-environment-jsdom @testing-library/jest-dom @testing-library/react @testing-library/user-even
Then you need to create some files for jest configs:
./jest.config.js
const nextJest = require('next/jest')
const createJestConfig = nextJest({
// Provide the path to your Next.js app to load next.config.js and .env files in your test environment
dir: './',
})
// Add any custom config to be passed to Jest
const customJestConfig = {
setupFilesAfterEnv: ['<rootDir>/jest.setup.js'],
moduleNameMapper: {
// Handle module aliases (this will be automatically configured for you soon)
'^@/components/(.*)$': '<rootDir>/components/$1',
'^@/pages/(.*)$': '<rootDir>/pages/$1',
},
testEnvironment: 'jest-environment-jsdom',
}
// createJestConfig is exported this way to ensure that next/jest can load the Next.js config which is async
module.exports = createJestConfig(customJestConfig)
./jest.setup.js
// Optional: configure or set up a testing framework before each test.
// If you delete this file, remove `setupFilesAfterEnv` from `jest.config.js`
// Used for __tests__/testing-library.js
// Learn more: https://github.com/testing-library/jest-dom
import '@testing-library/jest-dom/extend-expect'
React router (optional)
if you use `useRouter` in your components you have to mock `next/router` and you have to create a mock file for that.
./__mocks__/routerMock.tsx
import * as ReactTesting from '@testing-library/react'
jest.mock('next/router', () => ({
push: jest.fn(),
back: jest.fn(),
events: {
on: jest.fn(),
off: jest.fn(),
},
beforePopState: jest.fn(() => null),
useRouter: () => ({
push: jest.fn(),
}),
}))
Then you need to add this line into `jest.setup.js` file.
import './__mocks__/routerMock'
Writing tests
Now you are able to write unit tests.
You create test files in this path: ./__tests__/
For example, if you want to create a snapshot test you can do it like this:
./__tests__/snapshot.tsx
import { render } from '@testing-library/react'
import Home from '@/pages/index'
it('renders homepage unchanged', () => {
const { container } = render(<Home />)
expect(container).toMatchSnapshot()
})
To define some custom paths in tsconfig you can add the following:
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@/components/*": [
"components/*"
],
"@/pages/*": [
"pages/*"
],
"@/styles/*": [
"styles/*"
]
}
}
Run the tests
In the end, you can add these commands and use them to run your tests.
"scripts": {
"test": "jest --watch",
"test:ci": "jest --ci"
},
And run them in your terminal like this:
yarn test
npm run test
Pro Tip: When you extract components into modular, shareable, reusable ones using a tool like Bit, unit tests are treated as first-class citizens. Using Bit’s Compositions feature, you can automatically create .spec. and recognize .test. files, and every component you publish and version on Bit will include tests as essentials, giving you confidence in your code.
Let’s Connect
Thanks for reading my article. If you like my content, please consider buying me a coffee ☕.
Consider becoming a Medium member if you appreciate reading stories like this and want to help me as a writer. It costs $5 per month and gives you unlimited access to Medium content. I’ll get a little commission if you sign up via my link.
Build React apps with reusable components, just like Lego
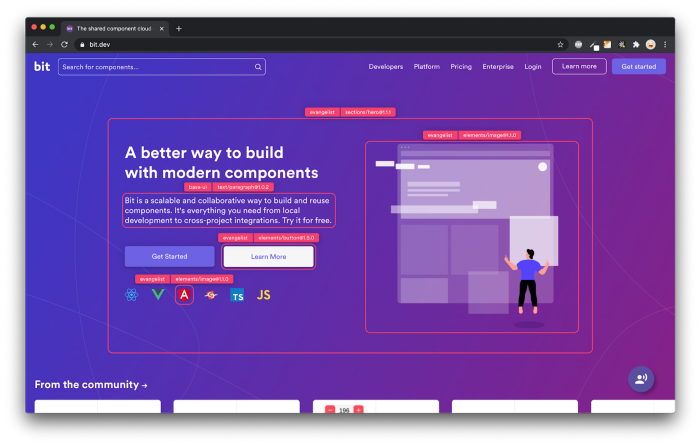
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
How to Write Unit Tests with React Testing Library in Next.js was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Farnam Homayounfard
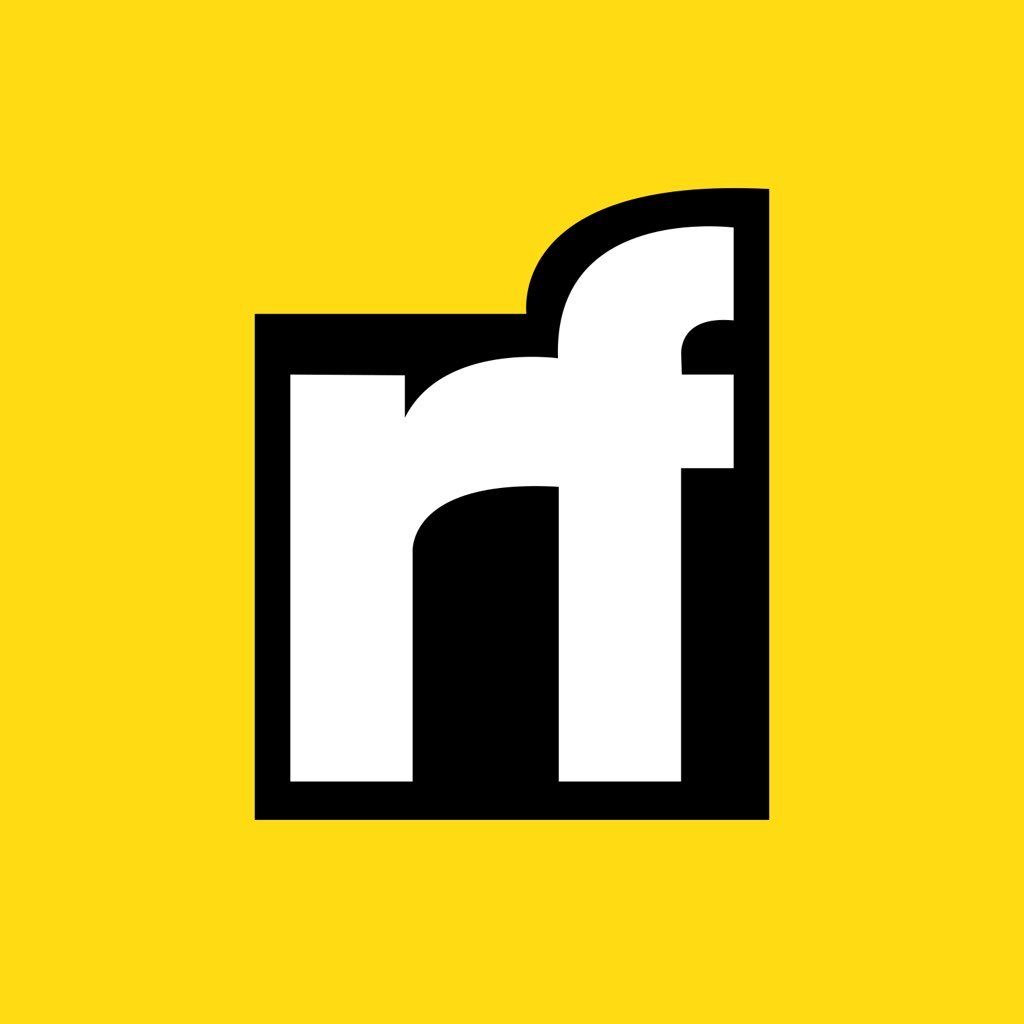
Farnam Homayounfard | Sciencx (2023-02-17T15:29:57+00:00) How to Write Unit Tests with React Testing Library in Next.js. Retrieved from https://www.scien.cx/2023/02/17/how-to-write-unit-tests-with-react-testing-library-in-next-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.