This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Kauna Hassan
Introduction
React is a popular JavaScript library for building user interfaces. However, as your application grows more complex, it can become challenging to manage the state of your application. That's where Redux comes in. Redux is a predictable state container that helps you manage the state of your application in a scalable and organized way. In this article, we'll explore how to use Redux for state management in your React application.
What is Redux?
Redux is a state management library that helps you manage the state of your application in a predictable way. It works by keeping your application state in a single, centralized store. Any changes to the state of your application are made through actions, which are dispatched to the store. The store then updates the state of your application based on the actions that were dispatched.
Advantages of Using Redux
There are several advantages to using Redux for state management in your React application:
Predictability: With Redux, the state of your application is predictable and easy to reason about. This makes it easier to debug your application and ensures that your application behaves consistently.
Scalability: Redux makes it easy to manage the state of your application as it grows more complex. You can add new features to your application without worrying about how they will affect the existing state of your application.
Reusability: Redux makes it easy to reuse stateful logic across your application. This can help reduce duplication and make your code more modular.
Getting Started with Redux
To use Redux in your React application, you'll need to install the redux and react-redux packages:
npm install redux react-redux
Next, you'll need to create a store to manage the state of your application:
import { createStore } from 'redux';
const initialState = {
count: 0,
};
function reducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
}
const store = createStore(reducer);
In this example, we've created a simple store with an initial state that includes a count property. We've also defined a reducer function that handles actions that are dispatched to the store. The reducer function takes the current state and an action as arguments and returns the new state of the application based on the action that was dispatched.
Next, we'll need to create a component that can interact with the store:
import { connect } from 'react-redux';
function Counter(props) {
const { count, increment, decrement } = props;
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
function mapStateToProps(state) {
return {
count: state.count,
};
}
function mapDispatchToProps(dispatch) {
return {
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
};
}
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
In this example, we've defined a Counter component that displays the current count from the store and provides buttons to increment and decrement the count. We've also defined mapStateToProps and mapDispatchToProps functions that map the state and actions of the store to props that can be used by the component. Finally, we've connected the component to the store using the connect function from the react-redux package.
Conclusion
Redux is a powerful tool for managing the state of your React application. It provides a predictable, scalable, and organized way to manage your application state, making it easier to reason about and debug your code.
By following the steps outlined in this article, you can get started with using Redux in your React application. With Redux, you can write clean, reusable code and manage the state of your application with ease. With practice, you can become proficient in using Redux and further enhance the functionality of your React applications.
In conclusion, state management in React with Redux is a great way to improve your React applications. Redux offers many advantages, including predictability, scalability, and reusability. By following the steps outlined in this article, you can get started with using Redux in your React application and start writing clean, reusable code. With Redux, you can easily manage the state of your application and improve the functionality of your React applications. By following best practices and using Redux, you can build better applications and become a more efficient React developer.
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Kauna Hassan
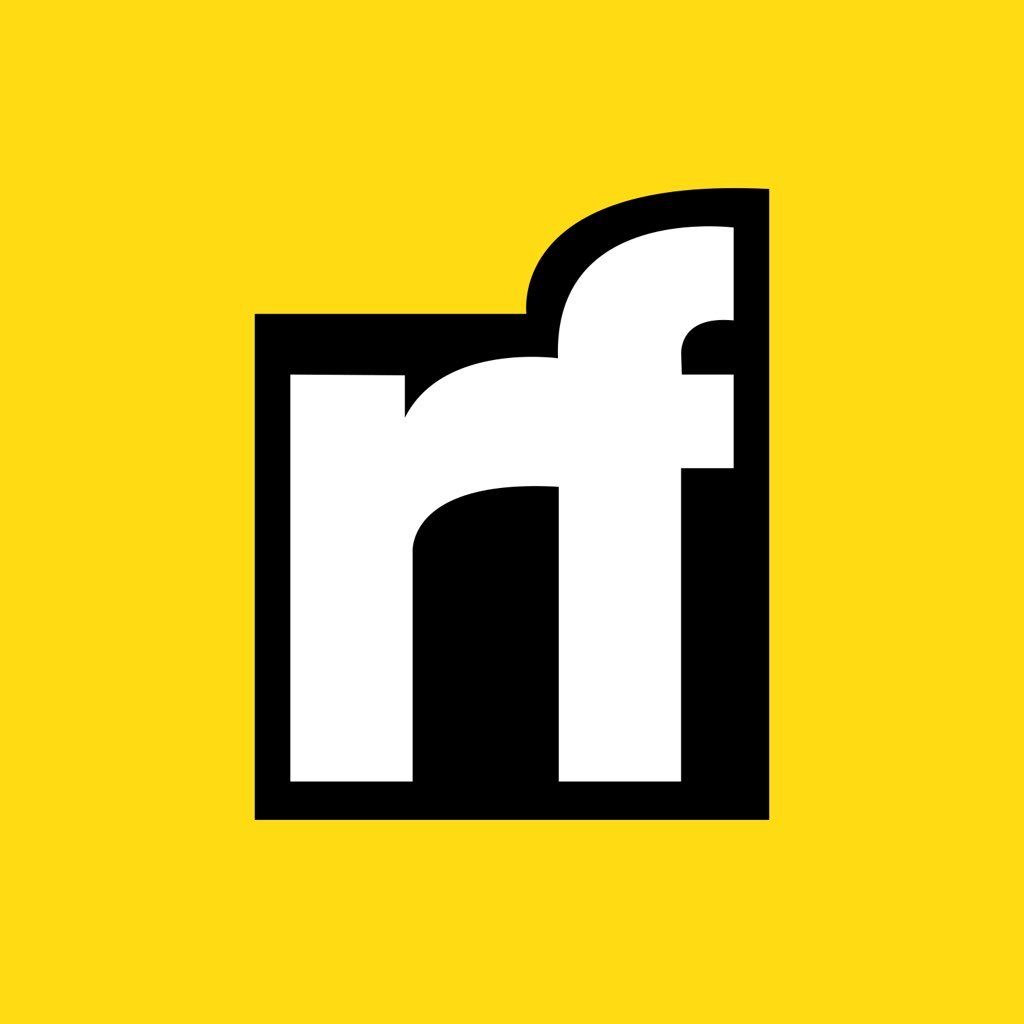
Kauna Hassan | Sciencx (2023-02-18T04:47:18+00:00) State Management in React with Redux. Retrieved from https://www.scien.cx/2023/02/18/state-management-in-react-with-redux/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.