This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Max
Python for loop is used to iterate over a sequence of elements such as a list, tuple, or string. The range
function can be used to create a sequence of numbers, and enumerate
can be used to iterate over a sequence while also keeping track of the index.
Python for Loop
The syntax of the for
loop in Python is as follows:
for variable in sequence:
# code to be executed for each element in sequence
Here, variable
is a new variable that takes on the value of each element in the sequence
. The code inside the loop is executed once for each element in the sequence
.
Example
Let's say we have a list of names and we want to print each name:
names = ['One', 'Two', 'Three', 'Four']
for name in names:
print(name)
Output:
One
Two
Three
Four
The range
Function
The range
function in Python is used to create a sequence of numbers. It can take up to three arguments: start
, stop
, and step
. The start
argument specifies the starting value of the sequence (default is 0), the stop
argument specifies the ending value (not inclusive), and the step
argument specifies the step size (default is 1).
Example
Let's say we want to print the numbers from 0 to 9:
for i in range(10):
print(i)
Output:
0
1
2
3
4
5
6
7
8
9
The enumerate
Function
The enumerate
function in Python is used to iterate over a sequence while also keeping track of the index. It returns a tuple containing the index and the element at that index.
Example
Let's say we have a list of names and we want to print each name along with its index:
names = ['One', 'Two', 'Three', 'Four']
for i, name in enumerate(names):
print(i, name)
Output:
0 One
1 Two
2 Three
3 Four
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Max
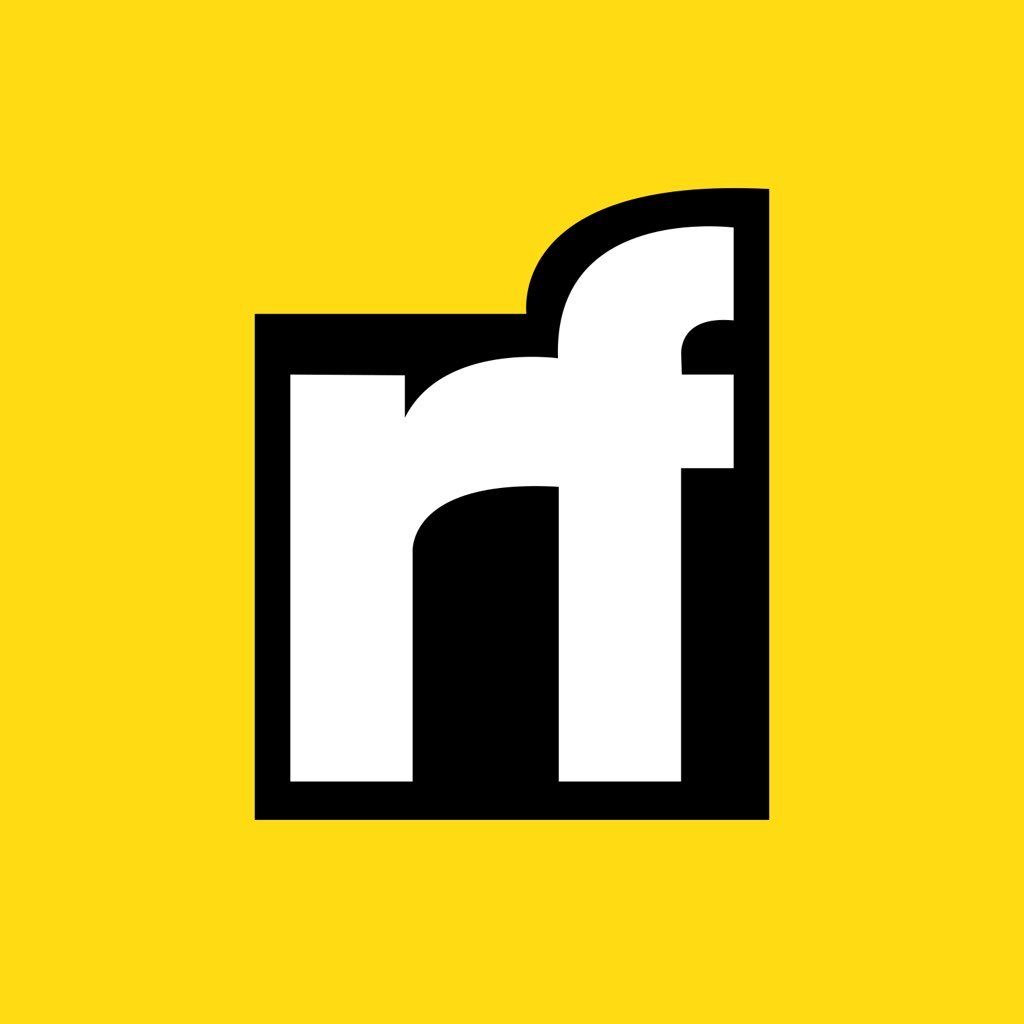
Max | Sciencx (2023-02-20T15:46:24+00:00) Python For Loops, Range, Enumerate Tutorial. Retrieved from https://www.scien.cx/2023/02/20/python-for-loops-range-enumerate-tutorial/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.