This content originally appeared on Bits and Pieces - Medium and was authored by Shamaz Saeed

JavaScript functions are a crucial part of the language, allowing developers to write reusable and modular code.
However, there are some advanced concepts related to functions that may not be familiar to all developers.
In this article, we'll explore some of the most powerful and advanced features of JavaScript functions, including closures, currying, and higher-order functions.
Understanding Closures
Closures are a fundamental concept in JavaScript that allows a function to remember and access its lexical scope, even when it's executed outside of that scope.
Closures are created when a function is defined inside another function, and the inner function references a variable from the outer function.
Here's an example of a closure:
function outerFunction() {
const message = 'Hello';
function innerFunction() {
console.log(message);
}
return innerFunction;
}
const myFunction = outerFunction();
myFunction(); // Output: Hello
In this example, the inner function innerFunction has access to the message variable, even though it's defined in the outer function outerFunction.
This is possible because innerFunction forms a closure over the message variable.
Using Currying
Currying is a technique that allows you to create a new function from an existing function by partially applying its arguments.
In other words, currying is a way of transforming a function that takes multiple arguments into a sequence of functions that each take a single argument.
Here’s an example of currying:
function add(a, b, c) {
return a + b + c;
}
function curry(fn) {
return function curried(...args) {
if (args.length >= fn.length) {
return fn(...args);
} else {
return function(...moreArgs) {
return curried(...args, ...moreArgs);
}
}
};
}
const curriedAdd = curry(add);
const addFive = curriedAdd(5);
const addTen = addFive(5);
console.log(addTen(10)); // Output: 20
In this example, we define a curried version of the add function that takes three arguments.
We then create a new function addFive by partially applying the first argument with the value 5. Finally, we create another new function addTen by partially applying the second argument with the value 5.
When we call addTen with the argument 10, it returns the value 20.
Leveraging Higher-Order Functions
A higher-order function is a function that takes one or more functions as arguments and/or returns a function as its result.
Higher-order functions are a powerful tool in functional programming and can be used for a wide variety of tasks, including filtering, mapping, and reducing arrays.
Here’s an example of a higher-order function:
function multiplyBy(factor) {
return function(value) {
return value * factor;
}
}
const double = multiplyBy(2);
const triple = multiplyBy(3);
console.log(double(5)); // Output: 10
console.log(triple(5)); // Output: 15
In this example, we define a higher-order function multiplyBy that returns a new function that multiplies its argument by a given factor. We then create two new functions double and triple by partially applying the multiplyBy function with the values 2 and 3, respectively. We can then use these new functions to multiply any value by 2 or 3, respectively.
Higher-order functions are also commonly used with array methods like filter, map, and reduce. Here's an example of using the map method with a higher-order function to transform an array of numbers:
const numbers = [1, 2, 3, 4, 5];
function square(number) {
return number * number;
}
const squaredNumbers = numbers.map(square);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
In this example, we define a function square that takes a number and returns its square. We then use the map method with the square function to transform an array of numbers into an array of their squares.
Real-World Applications
Now that we’ve explored closures, currying, and higher-order functions, let’s take a look at some real-world applications of these advanced concepts.
One common use case for closures is to create private variables in JavaScript. By defining a variable inside a function and returning a function that has access to that variable, we can create a private variable that’s not accessible outside of the function.
Currying is often used in functional programming to create reusable functions. By partially applying a function’s arguments, we can create new functions that have some of the arguments already set.
This makes it easy to create reusable functions that can be used in a variety of contexts.
Finally, higher-order functions are a key tool in functional programming for transforming and working with data.
By combining higher-order functions with array methods like filter, map, and reduce, we can create powerful and expressive code for manipulating data.
Conclusion
In this article, we’ve explored some of the most advanced concepts related to JavaScript functions, including closures, currying, and higher-order functions.
By understanding these concepts and their real-world applications, you can become a more skilled and effective JavaScript developer.
Whether you’re building web applications, creating APIs, or working on other projects, advanced JavaScript functions can help you write more modular, reusable, and expressive code.
If you want to stay updated on more tutorials and projects, be sure to follow me on GitHub and Medium.
Thanks for reading, and happy coding!
Build apps with reusable components like Lego
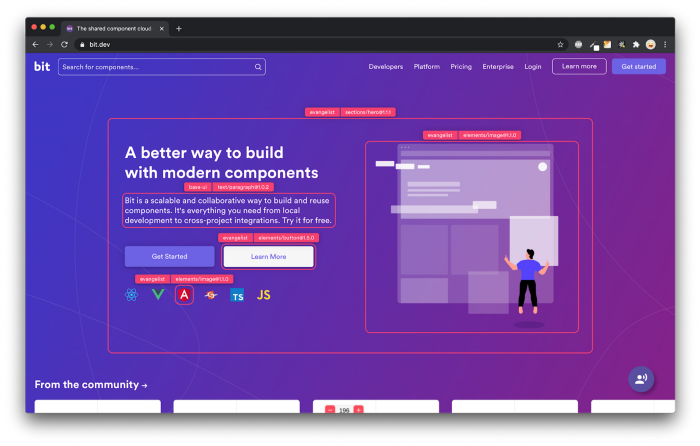
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- Bit - Component driven development
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Mastering Advanced JavaScript Functions: Closures, Currying, and Higher-Order Functions was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Shamaz Saeed
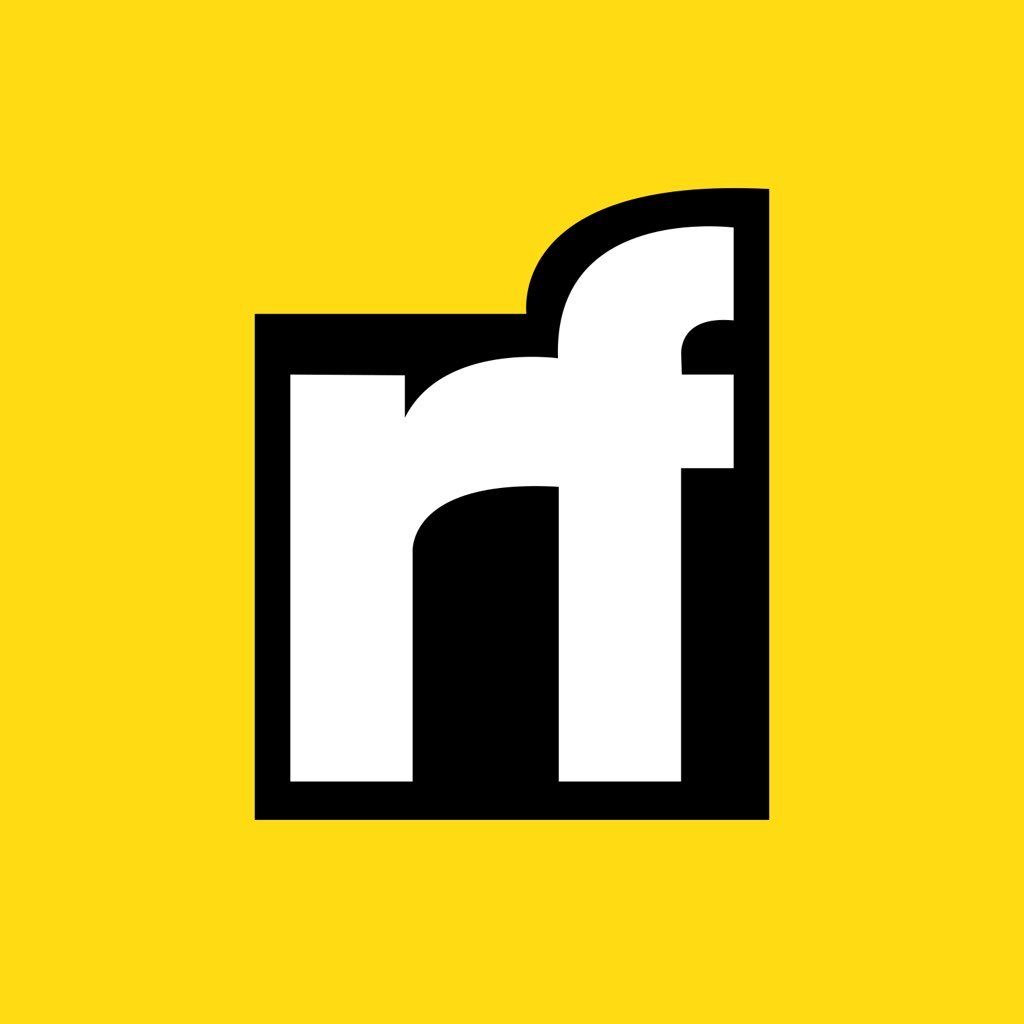
Shamaz Saeed | Sciencx (2023-02-23T07:02:40+00:00) Mastering Advanced JavaScript Functions: Closures, Currying, and Higher-Order Functions. Retrieved from https://www.scien.cx/2023/02/23/mastering-advanced-javascript-functions-closures-currying-and-higher-order-functions/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.