This content originally appeared on DEV Community and was authored by Mavis
I recently implemented Auth0 in my personal side project. If you're interested in learning how to implement it in React and Node, feel free to take a look.
pirate-shopping
The pirate shopping project is one of my side projects that allows me to keep adding some tech to advance my skillset.
To do list breakdown
- Deploy to AWS instead of Netlify, https://www.pirate-in-melbourne.com/ [Done]
- Setup github actions, to push docker images to AWS ECR [Done]
- Auth0 [Done]
Tech
Front-end
React 18
, vite
, TypeScript
, Redux
, Hooks
, Firebase
, Auth0 Login
, CSS-in-JS
Backend
Node/Express framework
Others
AWS
, Docker
, GitHub Action
, Netlify
How to run it
Please make sure docker have already installed.
Run ./run_docker.sh dev|prod
Production
docker build . -t xychenxy/pirate-shopping
docker run -it -p 5000:5000 -e "NODE_ENV=production" xychenxy/pirate-shopping
OAuth 2.0 is the industry-standard protocol for authorization. OAuth 2.0 focuses on client developer simplicity while providing specific authorization flows for web applications, desktop applications, mobile phones, and living room devices. Ref from https://oauth.net/2/
Auth0 is a software product that implement the OAuth 2.0 protocol.
The flow
From below graph we can easy to tell that client will get 2 types of token (access and refresh) from Authorization Server. Access token is used to protect your resources, refresh token is used to obtain new access token and refresh token.
Let's see how to implement auth0 in react and node
React side
In react codebase, install @auth0/auth0-react
<Auth0Provider
domain={import.meta.env.VITE_AUTH0_DOMAIN}
clientId={import.meta.env.VITE_AUTH0_CLIENT_ID}
authorizationParams={{
redirect_uri: window.location.origin,
audience: import.meta.env.VITE_AUTH0_AUDIENCE,
}}
onRedirectCallback={onRedirectCallback}
// scope="read:current_user"
>
{children}
</Auth0Provider>;
you also can use getAccessTokenSilently()
to get new access token.
for some protected resources requests, make sure add headers: {Authorization: Bearer token
} in your request
useEffect(() => {
try {
const fetchAccessToken = async () => {
const newAccessToken = await getAccessTokenSilently({
authorizationParams: {
audience: import.meta.env.VITE_AUTH0_AUDIENCE,
scope: "read:current_user",
},
});
localStorage.setItem("token", newAccessToken);
};
if (isAuthenticated) {
fetchAccessToken();
}
} catch (e: any) {
console.log("error", e.message);
}
}, [getAccessTokenSilently, isAuthenticated]);
Node side
In node codebase, express-jwt
and jwks-rsa
are useful to do the jwt check with auth0
const jwtCheck = jwt({
secret: jwks.expressJwtSecret({
cache: true,
rateLimit: true,
jwksRequestsPerMinute: 5,
jwksUri: `https://${process.env.auth0_domain}/.well-known/jwks.json`,
}),
audience: process.env.audience,
issuer: `https://${process.env.auth0_domain}/`,
algorithms: ["RS256"],
});
sometimes, if you want to get user info, it's not a good idea to put user info in your request, we can do it in this way
const userInfo = await axios
.get(`https://${process.env.auth0_domain}/userinfo`, {
headers: {
Authorization: req.headers.authorization,
},
})
.then((response) => {
return response.data;
});
Summary
Auth0 is a great product that allows us to implement authentication process quickly. Why not give it a try?
This content originally appeared on DEV Community and was authored by Mavis
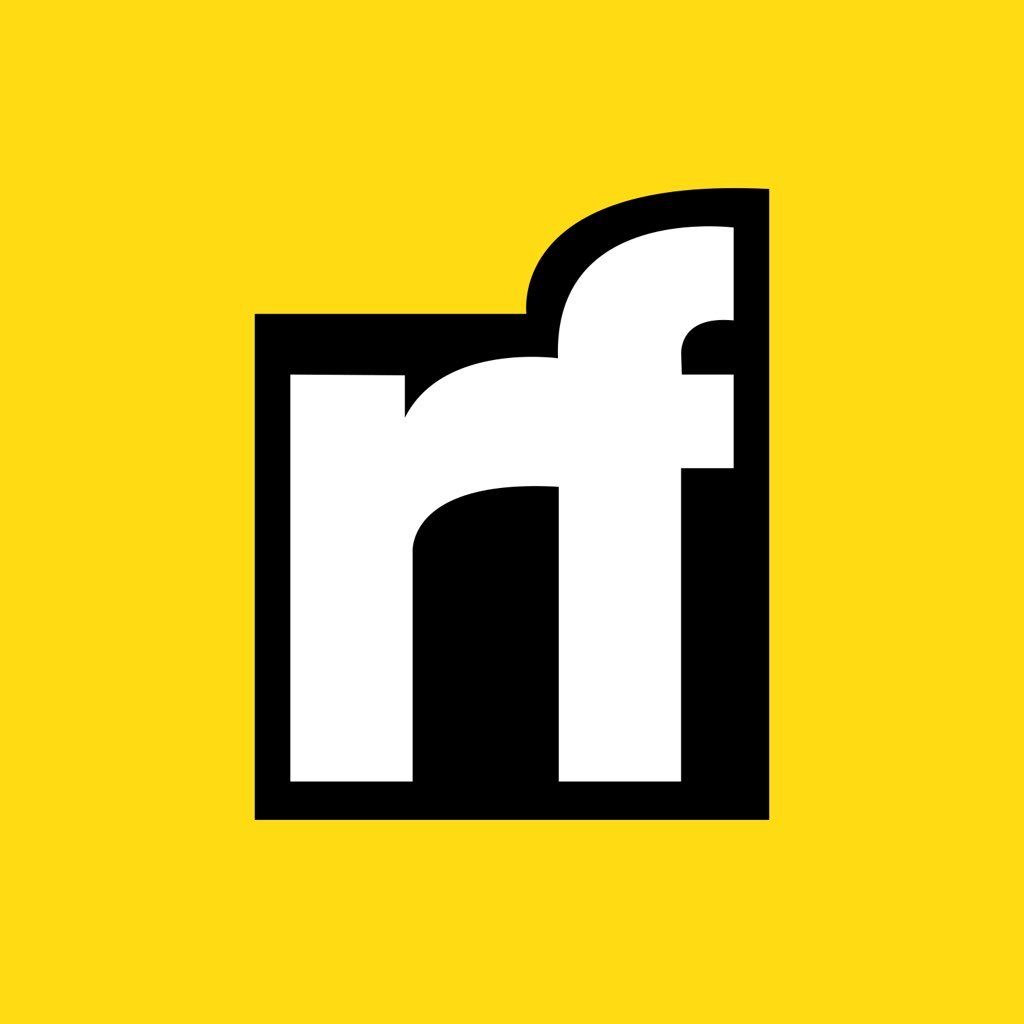
Mavis | Sciencx (2023-02-27T04:15:45+00:00) Auth0 in React and Node. Retrieved from https://www.scien.cx/2023/02/27/auth0-in-react-and-node/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.