This content originally appeared on Level Up Coding - Medium and was authored by Arjan Aswal
From reverse proxies to streams and caching, discover practical tips and coding examples to supercharge your Node.js API performance and keep your users happy.
Node.js has become one of the most popular technologies for developing web applications, particularly for building APIs. Node.js is built on top of Google’s V8 JavaScript engine, which makes it lightning-fast and scalable. However, even with Node.js, there are still ways to optimize and speed up your API’s performance.
In this article, we’ll discuss some hacks to speed up Node.js API performance and speed, including coding examples to help you understand each point thoroughly.
Use a Reverse Proxy
A reverse proxy is a server that sits between the client and the API server and forwards client requests to the API server. By using a reverse proxy, we can offload some of the work from the API server and improve its performance. Additionally, we can use a reverse proxy to load balance requests across multiple API servers, improving the scalability of our API.
Here’s an example of how to use a reverse proxy with Node.js:
In this example, we use the http-proxy library to create a reverse proxy that forwards requests to the API server running on port 3000. We use the app.all() method to intercept all requests to the /api/* endpoint and forward them to the API server using the proxy.web() method.
Use Caching
Caching is a technique used to store frequently accessed data in memory, reducing the amount of work the API server needs to do to retrieve the data. By using caching, we can improve the response time of our API and reduce the load on the database.
Here’s an example of how to use caching with Node.js:
In this example, we create a new NodeCache object and use it to store the ‘some-data’ key with a TTL of 5 minutes. We check if the key exists in the cache, and if so, return the cached data. If not, we fetch the data from an external API using the fetchData function and cache it for 5 minutes before returning it to the client.
Use Streams
Streams are a powerful feature in Node.js that can be used to process large amounts of data efficiently. Streams are like pipelines where data flows through different stages of processing. By using streams, we can avoid loading large files or data sets into memory, which can cause performance issues.
Here’s an example of how to use streams to process a large CSV file:
In this example, we use the fs module to create a read stream for a CSV file, and the csv-parser library to parse each row of data. As each row of data is processed, we push it into an array of results. Once all the data has been processed, we can do something with the results, such as writing them to a database or sending them to a client.
Use Compression
Compression is a technique used to reduce the size of data sent over the network, improving the performance of our API. By compressing our API responses, we can reduce the amount of data sent over the network, resulting in faster response times and lower bandwidth usage.
Here’s an example of how to use compression with Node.js:
In this example, we use the compression middleware provided by the compression library to compress our API responses. When a client requests data from our API, the data is fetched from the database and sent to the client. However, before sending the data, the compression middleware compresses it using gzip or deflate compression, reducing the size of the data sent over the network.
Use Cluster Module
The cluster module is a built-in Node.js module that allows us to create a cluster of Node.js processes. By creating a cluster, we can take advantage of multi-core CPUs and improve the performance of our API server.
Here’s an example of how to use the cluster module in Node.js:
In this example, we use the cluster module to create a cluster of Node.js processes. We use the os module to get the number of available CPUs and create a worker process for each CPU. When a worker process dies, the cluster module automatically creates a new worker process to replace it.
Use connection pooling
Connection pooling is a technique used to reuse database connections instead of creating a new connection for each request. This can significantly reduce the overhead of creating and tearing down connections, resulting in improved performance.
Here’s an example of how to use connection pooling with the pg module to connect to a PostgreSQL database:
In this example, we use the pg module to create a connection pool to our PostgreSQL database. We then use the pool.connect() method to acquire a client from the pool, execute our query, and release the client back to the pool when we’re done.
Use Promise.all for parallel processing
When you need to perform multiple asynchronous tasks at the same time, you can use Promise.all to execute them in parallel. This can improve the performance of your API by reducing the time it takes to complete multiple tasks.
Here’s an example of how to use Promise.all to fetch data from multiple sources in parallel:
In this example, we use axios to fetch data from three different endpoints in parallel using Promise.all. The Promise.all method takes an array of promises and returns an array of results when all the promises have resolved. We then return the data as an object with three properties: users, posts, and comments.
Using Promise.all can significantly reduce the time it takes to fetch data from multiple sources, improving the performance of your API. However, be careful not to overload your server by performing too many parallel tasks at once.
Optimize Database Queries
Database queries can be a significant bottleneck in our API performance. By optimizing our database queries, we can reduce the amount of time it takes to fetch data from the database and improve the response time of our API.
Here are some tips for optimizing database queries:
- Use indexes: Indexes can speed up database queries by allowing the database to quickly find the data it needs. Be sure to index columns that are frequently used in queries.
- Avoid OR conditions: OR conditions can be slow in database queries, especially when they involve multiple columns. Instead, use UNION to combine multiple queries.
- Use pagination: When fetching large amounts of data from the database, use pagination to limit the amount of data returned in each query.
- Use efficient joins: Joins can be slow in database queries, especially when they involve large tables. Be sure to use efficient join algorithms, such as nested loop join or hash join, and avoid cross joins whenever possible.
Here’s an example of how to use pagination to optimize a database query in Node.js:
In this example, we use the OFFSET and LIMIT clauses in the SQL query to implement pagination. The page and limit parameters are passed in the query string, and the offset is calculated based on the current page and limit. By limiting the number of rows returned in each query, we can reduce the amount of time it takes to fetch data from the database and improve the performance of our API.
Conclusion
In conclusion, optimizing the performance and response time of Node.js APIs is essential for delivering a fast and efficient web application. The various hacks and techniques discussed in this article, such as caching, compression, connection pooling, and clustering, can greatly improve the performance and scalability of Node.js APIs. By implementing these hacks and monitoring the performance metrics, developers can fine-tune their Node.js applications to handle more requests and provide a seamless user experience. It is important to remember that every application is unique and requires a tailored approach to optimize its performance. Therefore, continuous monitoring, testing, and experimentation are crucial to achieve the best possible results. With the right tools and techniques, developers can ensure that their Node.js APIs are running at peak performance, delivering lightning-fast response times to their users.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Boost Your Node.js API Speed: Top Hacks to Optimize Performance and Accelerate Response Time was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Arjan Aswal
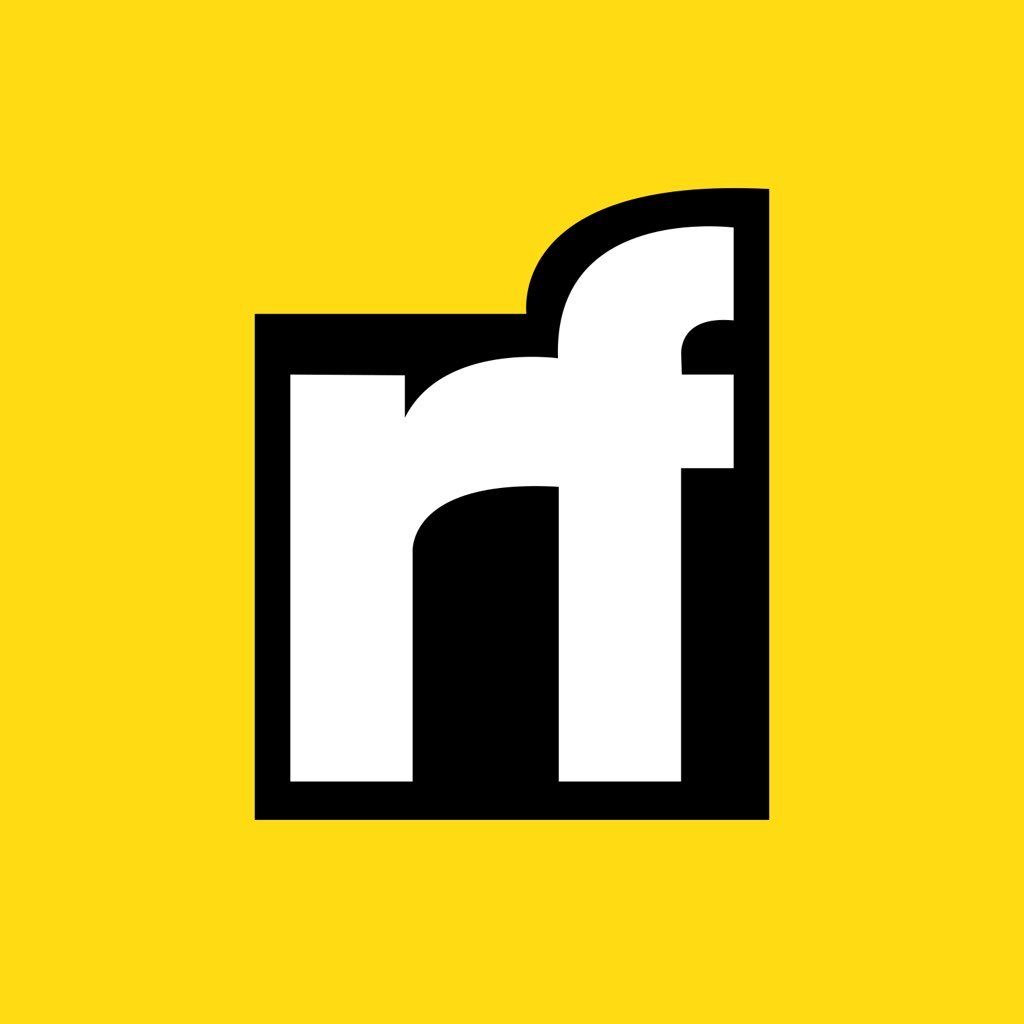
Arjan Aswal | Sciencx (2023-02-27T01:38:11+00:00) Boost Your Node.js API Speed: Top Hacks to Optimize Performance and Accelerate Response Time. Retrieved from https://www.scien.cx/2023/02/27/boost-your-node-js-api-speed-top-hacks-to-optimize-performance-and-accelerate-response-time/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.