This content originally appeared on DEV Community and was authored by Kauna Hassan
React is a powerful JavaScript library that enables developers to easily create complex user interfaces. However, it can be difficult for newcomers to distinguish between props and states. Props and state are both important React concepts, but they serve different purposes and should be used differently.
What Are props and state?
Both 'props' and'state' are JavaScript objects that hold information that React components can use. The distinction is that props are passed down from a parent component to a child component, whereas state is managed within the component itself.
props
props
stands for "properties" and is short for "component properties". Props are unchangeable and are passed down from parent to child components. Props, in other words, are read-only and should not be modified by the child component.
Here's an example of a parent component passing props to a child component:
// ParentComponent.js
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const message = 'Hello, World!';
return (
<div>
<ChildComponent message={message} />
</div>
);
}
// ChildComponent.js
import React from 'react';
function ChildComponent(props) {
return <div>{props.message}</div>;
}
The ParentComponent
in this example passes the message
prop to the ChildComponent
. The ChildComponent
object is passed as an argument to the 'ChildComponent,' which then renders the message
prop.
state
Astate
object is one that is managed within a component. It enables a component to keep track of its own data and automatically update its rendering when that data changes. Unlike props
,state
can be changed by the component.
Here's an example of a component that makes use of state
:
// MyComponent.js
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
<div>
<p>You clicked the button {count} times.</p>
<button onClick={handleClick}>Click me</button>
</div>
);
}
In this example, the MyComponent
component manages its own count
state using the useState
hook. When the button is clicked
, the handleClick
function updates the count state, and the component re-renders to reflect the updated state.
When Should You Use props or state?
Depending on the situation, props
and state
should be used in different ways.
When data is passed down from parent to child, use props
.
When passing data from a parent component to a child component, use props
. Because props
are immutable, the child component should not modify them.
When Data Is Managed Within a Component, Use state
When you need to manage data within a component, useState
. The component can change the state using setState
or hooks like useState
, useReducer
, or useContext
.
Conclusion
In conclusion, props
and state
are both important concepts in React, but they serve different functions. Props
are used to pass data from parent to child components, whereas state' is used to manage data within a component.
When using props, keep in mind that they are read-only and should not be changed by the child component. If you need to change the data, use state
instead.
Similarly, when working with state
, keep in mind that it should only be changed using the setState
method or hooks like useState
, useReducer
, or useContext
. Directly modifying state can result in unexpected behavior and should be avoided.
You can write more efficient and maintainable React code if you understand the difference between props
and state
. Props
are used to pass data from parent to child components, and state is used to manage data within a component. Always use setState
or hooks to modify state
and avoid directly modifying props
.
With a better understanding of props
and state
, you can use React's powerful capabilities to create dynamic and responsive user interfaces.
This content originally appeared on DEV Community and was authored by Kauna Hassan
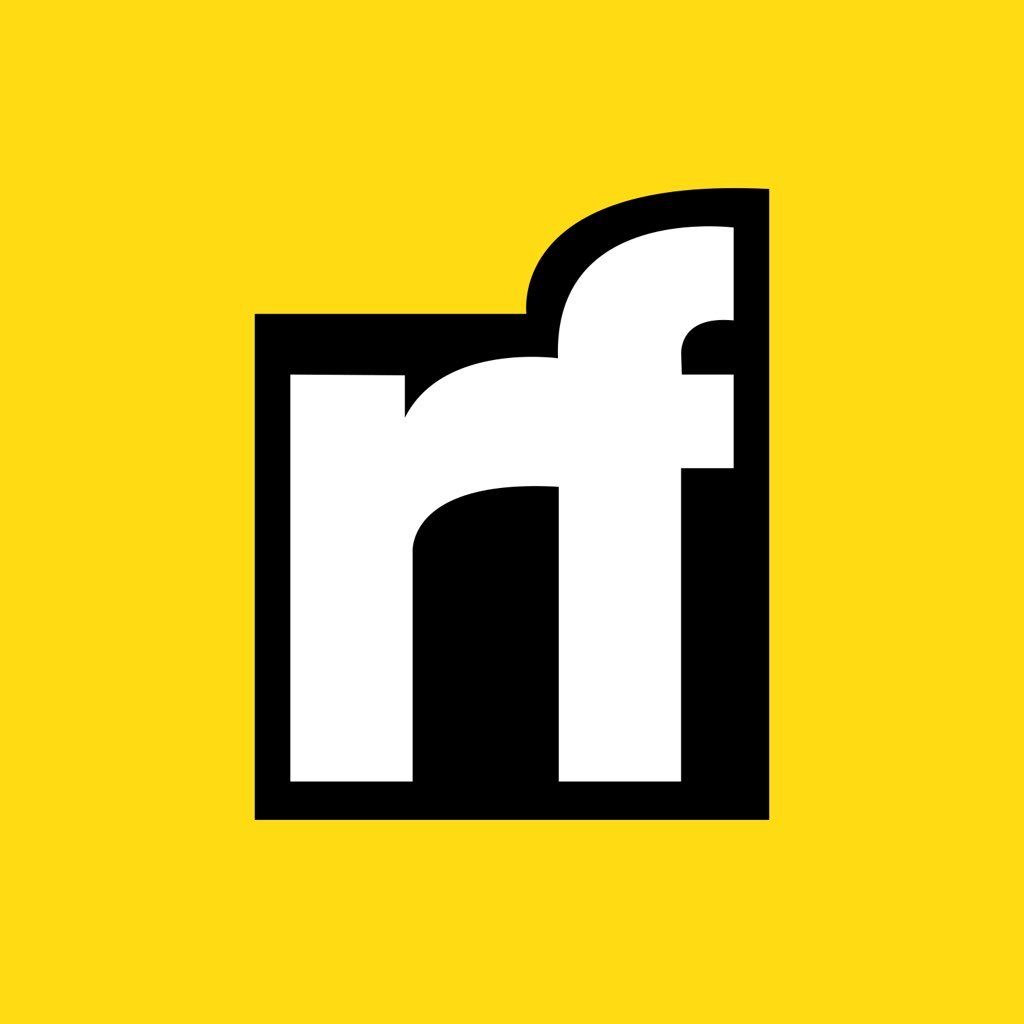
Kauna Hassan | Sciencx (2023-03-05T23:51:28+00:00) Understanding the Difference Between props and state in React. Retrieved from https://www.scien.cx/2023/03/05/understanding-the-difference-between-props-and-state-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.