This content originally appeared on DEV Community and was authored by Kauna Hassan
Introduction
React is a well-known front-end JavaScript library for creating dynamic user interfaces. Its popularity stems from its simplicity, modularity, and reusability. However, as your codebase grows, you may encounter scalability and maintainability issues. Code splitting and lazy loading are two approaches to overcoming these challenges. In this article, we'll look at how to use code splitting and lazy loading to create a scalable and maintainable React codebase.
What is code splitting?
Code splitting is a technique for dividing your application code into smaller chunks that can be loaded as needed. This technique aids in reducing your application's initial load time, which improves the user experience. When your application's codebase is large and complex, code splitting comes in handy.
The dynamic import() function can be used to implement code splitting in React. This function allows you to load modules on the fly. Here's an example of how to load a module using dynamic import():
import('./MyComponent').then((module) => {
// use module.default to access the default export of the module
});
What is lazy loading?
Lazy loading is a method of deferring the loading of non-critical resources until they are required. This technique improves the initial load time of your application by loading only the necessary resources at the start.
The React.lazy() function is used to implement lazy loading in React. This function allows you to load a component slowly. Here's an example of using React.lazy():
const MyComponent = React.lazy(() => import('./MyComponent'));
Best practices for building a scalable and maintainable React codebase
use code splitting and lazy loading.
You can improve the performance of your application by reducing the initial load time by using code splitting and lazy loading. This enhances the user experience and ensures that your app is fast and responsive.
Maintain a small and focused set of components.
Keep your components small and focused to ensure that your codebase is maintainable. Each component should be simple to understand and have a single responsibility.
To manage state, use React Hooks.
React Hooks are a powerful React feature for managing state in a functional component. Hooks make it simple to share stateful logic between components, reducing code complexity.
Use TypeScript to improve the type safety of your code.
TypeScript is a JavaScript superset that adds static typing to your code. You can improve the type safety of your code and reduce the likelihood of bugs by using TypeScript.
To ensure the quality of your code, write unit tests.
Unit tests are critical for ensuring that your code is of high quality. You can catch bugs early and ensure that your codebase is maintainable by writing unit tests.
Conclusion
Building a scalable and maintainable React codebase is critical for ensuring your application's long-term success. You can improve the performance of your application and ensure that it remains fast and responsive as it grows by using code splitting and lazy loading. You can ensure that your codebase is maintainable and of high quality by following best practices such as keeping your components small and focused, using React Hooks to manage state, TypeScript to improve type safety, and writing unit tests.
This content originally appeared on DEV Community and was authored by Kauna Hassan
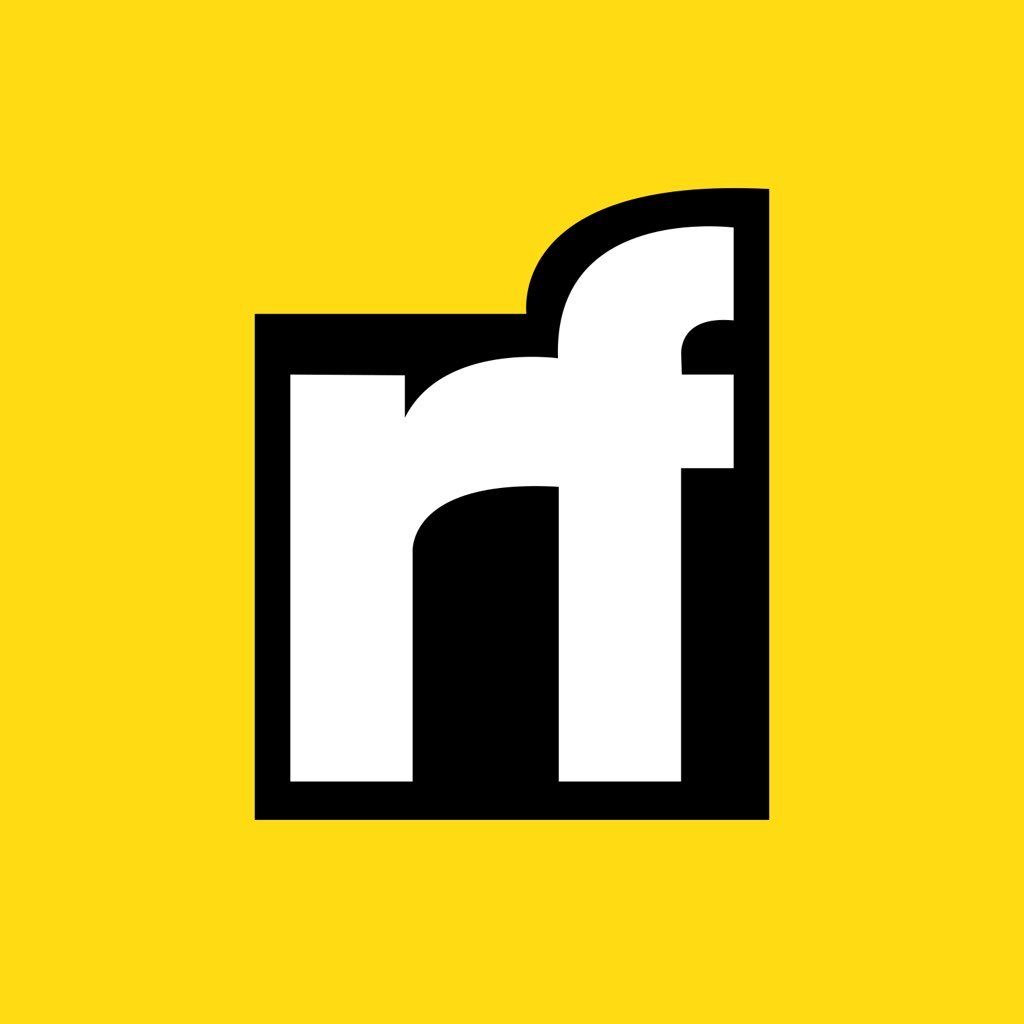
Kauna Hassan | Sciencx (2023-03-06T00:13:09+00:00) Best Practices for Building React Apps with Code Splitting and Lazy Loading to Improve Performance and Maintainability. Retrieved from https://www.scien.cx/2023/03/06/best-practices-for-building-react-apps-with-code-splitting-and-lazy-loading-to-improve-performance-and-maintainability/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.