This content originally appeared on DEV Community and was authored by Kauna Hassan
Introduction
Both React and TypeScript are well-known technologies in the world of software development. React is a popular front-end library for creating user interfaces, and TypeScript is a type-safe superset of JavaScript that aids in error detection during compilation. This article will go over how to use React with TypeScript, as well as best practices and code examples.
Setting up a TypeScript React Project:
To begin, we'll make a new React project with Create React App and TypeScript. This can be accomplished by entering the following command into our terminal:
npx create-react-app my-app --template typescript
This command generates a new React project with TypeScript pre-installed. Then, using the cd command, we can navigate to the project directory and start the development server by running npm start.
Writing TypeScript Components
Now that our project is set up, let's write a simple React component in TypeScript. In the src/components
directory, we'll create a new file called MyComponent.tsx
and add the following code:
import React from 'react';
interface Props {
name: string;
}
const MyComponent: React.FC<Props> = ({ name }) => {
return (
<div>
Hello, {name}!
</div>
);
};
export default MyComponent;
In this code, we're defining a new component called MyComponent
, which accepts a type string
of name prop
. We're also defining our component function with the React.FC
type, which provides type checking for our component's props
.
Using the Component
Now that we've defined our component, let's put it to use in our app. The following code will be added to the App.tsx
file:
import React from 'react';
import MyComponent from './components/MyComponent';
function App() {
return (
<div>
<MyComponent name="React with TypeScript" />
</div>
);
}
export default App;
We're importing our MyComponent
component and using it in our App
component with the name
prop "React with TypeScript" in this code.
Benefits of Using React with TypeScript
There are several advantages to using TypeScript with React. TypeScript catches errors at compile time, saving us time and preventing bugs in our code. Furthermore, TypeScript improves code completion and documentation, making it easier for developers to understand and use our code. Overall, combining TypeScript and React can assist us in writing more robust and maintainable code.
Conclusion
In this article, we looked at how to use React with TypeScript, including how to create a project, write components in TypeScript, and use those components in our app. We can write more robust and maintainable code by combining TypeScript and React. Consider using TypeScript to improve your development process the next time you start a new React project.
This content originally appeared on DEV Community and was authored by Kauna Hassan
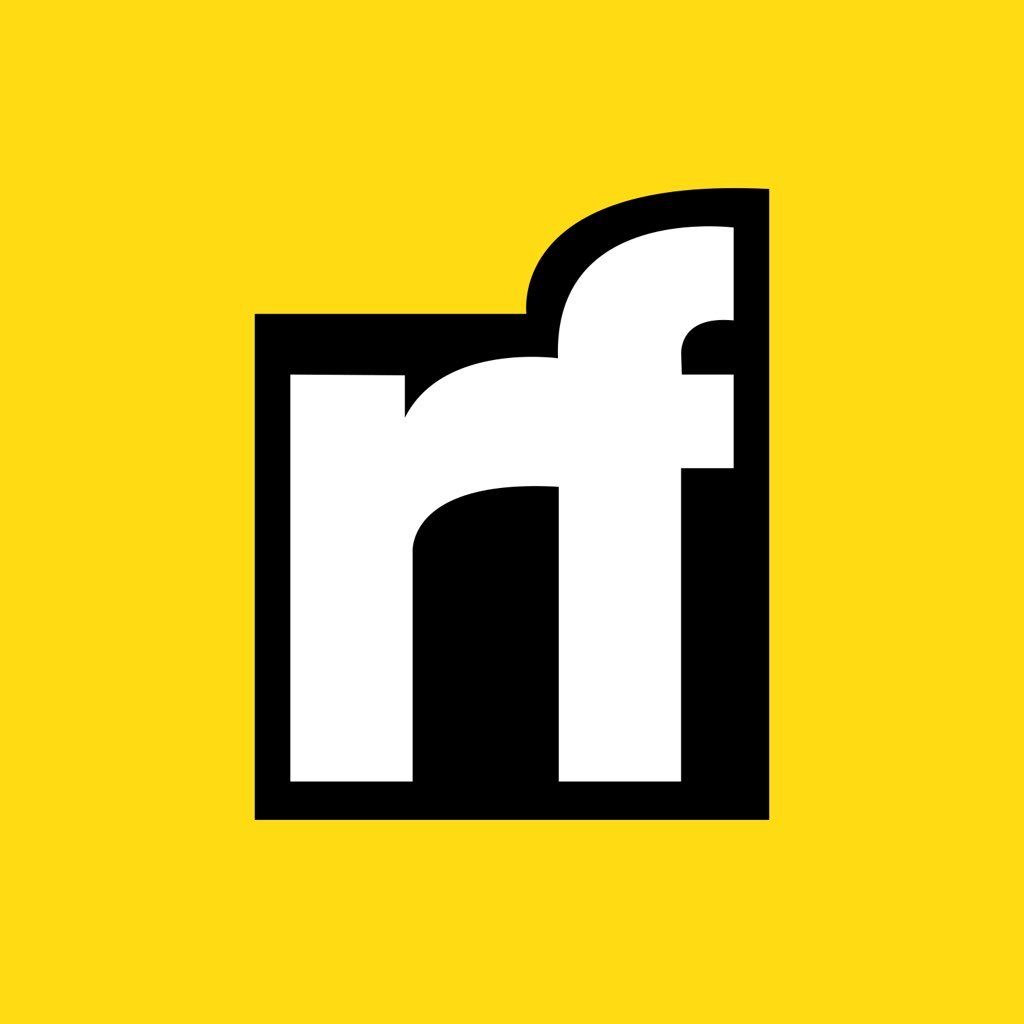
Kauna Hassan | Sciencx (2023-03-06T00:38:43+00:00) Building Maintainable User Interfaces with React and TypeScript. Retrieved from https://www.scien.cx/2023/03/06/building-maintainable-user-interfaces-with-react-and-typescript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.